project3
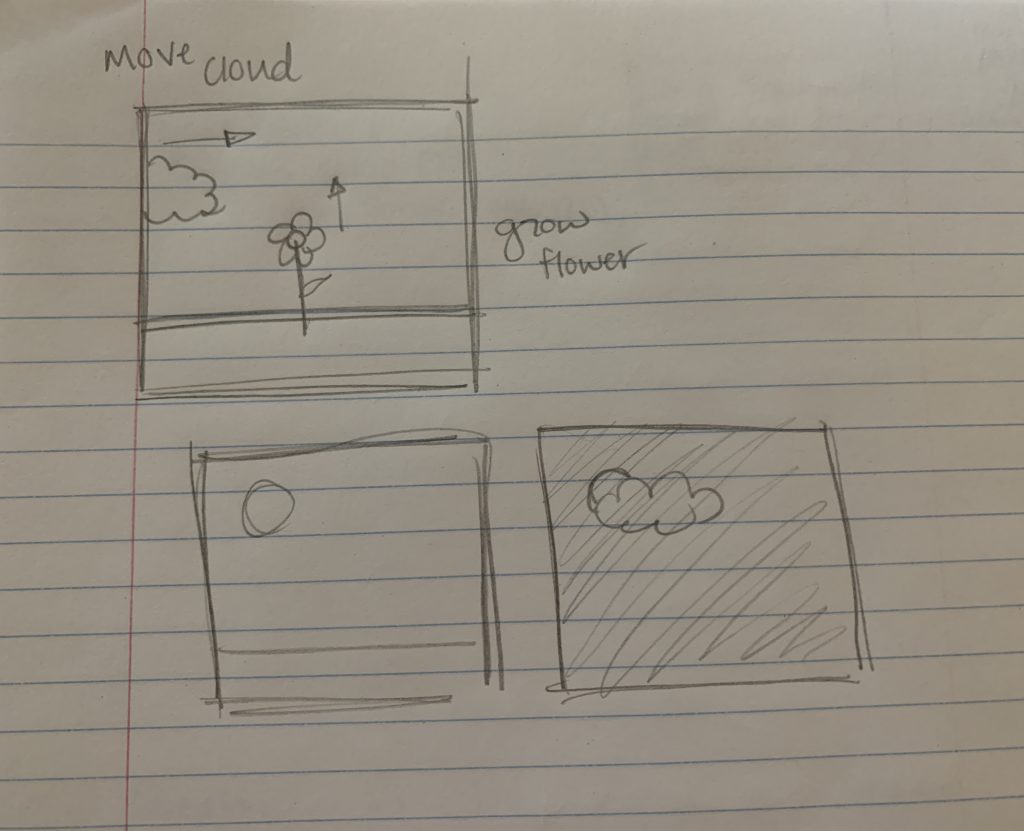
Initial idea of moving flower and cloud with mouseX and mouseY. Also, figuring out how to make scene darker when cloud covers the sun.
For this project, I wanted some kind of flower scene. I had a lot of fun figuring out how to make it seem like they can grow based on the position of the mouse, along with making the cloud interactive both with the sun and the mouse. I added the spinning parts to try the rotate and push & pop methods and figure out how they work.
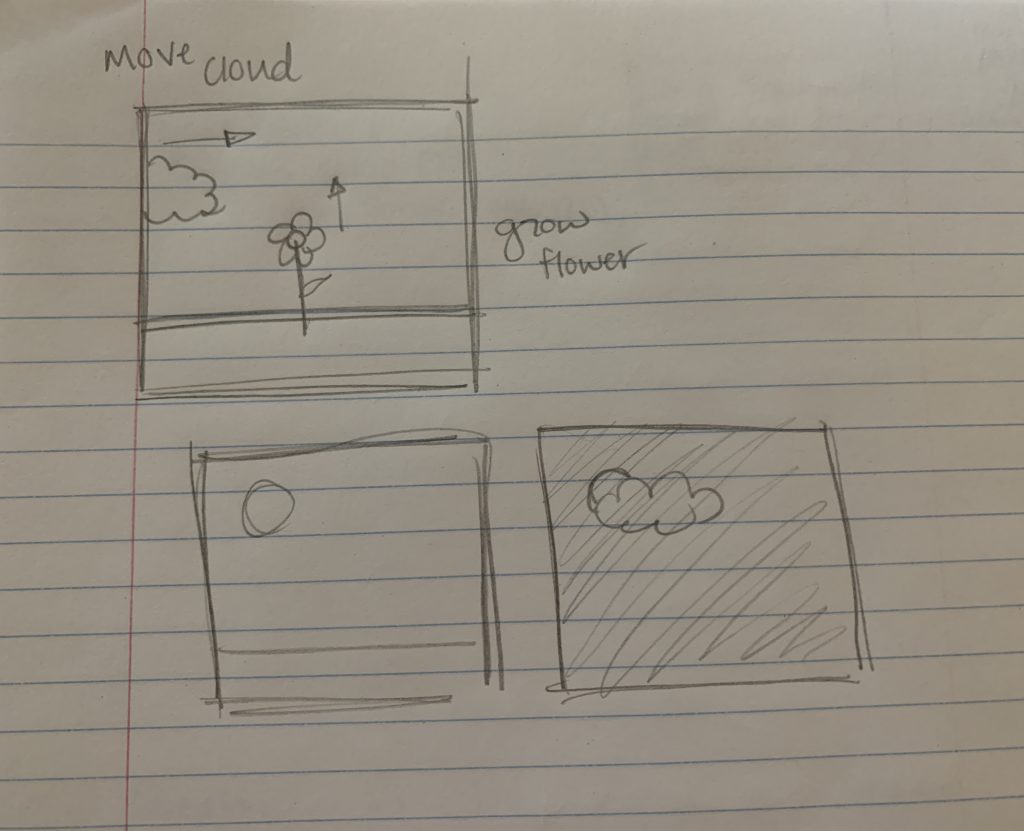
// Rachel Legg / rlegg / Section C
var x;
var y;
var offset = 5;
var fOne;
var fThree;
var edge = 599;
var angle = 0;
function setup() {
createCanvas(600, 450);
background(255);
x = 1/5 * width;
}
function draw() {
//if mouse is on right side, it is night , or else it is day
if (mouseX <= width / 2){
background(135, 206, 235); //dark blue
noStroke();
ellipse(75, 75, 40);
fill("yellow");
noStroke();
ellipse(75, 75, 40);
}else{
background(0, 0, 102);
fill(255);
noStroke();
ellipse(75, 75, 40);
fill(0, 0, 102);
ellipse(80, 70, 40);
}
//ground
fill(111, 78, 55); //brown
noStroke();
rect(0, 4/5 * height, width, 4/5 * height);
fill("green"); //grass
rect(0, 4/5 * height, width, 20);
//flower 1
//flower stem grows and shrink based on mouse location
if(mouseX < 200 & mouseY <= (4/5 *height)){
stroke("green");
line(1/4 * width, 4/5 * height, 1/4 * width, mouseY);
fill(255);
noStroke();
fOne = 1/4 * width;
circle(fOne, mouseY - 17, 30);
circle(fOne - 20, mouseY - 5, 30);
circle(fOne + 20, mouseY - 5, 30);
circle(fOne - 12, mouseY + 13, 30);
circle(fOne + 12, mouseY + 13, 30);
fill("yellow");
stroke("pink");
strokeWeight(4);
circle(1/4 * width, mouseY, 20);
//flower spinds when not interacting
}else{
strokeWeight(4);
stroke("green");
line(1/4 * width, 4/5 * height, 1/4 * width, 2/3 * height);
fill(255);
noStroke();
push();
translate(150, 300);
rotate(radians(angle));
circle(0, -17, 30);
circle(-20, -5, 30);
circle(20, -5, 30);
circle(-12, 13, 30);
circle(12, 13, 30);
fill("yellow");
stroke("pink");
strokeWeight(4);
circle(0, 0, 20);
pop();
angle += 2
}
//flower 2
if(mouseX < 400 & mouseX > 200 && mouseY <= (4/5 *height)){
stroke("green");
line(width / 2, 4/5 * height, width / 2, mouseY);
fill(250, 172, 382);
noStroke();
circle(width / 2, mouseY, 40, 40);
fill(77, 208, 99);
circle(width / 2, mouseY, 25, 25);
fill(255, 51, 51);
circle(width / 2, mouseY, 10, 10);
}else{
strokeWeight(4);
stroke("green");
line(width / 2, 4/5 * height, width / 2, 2/3 * height);
fill(250, 172, 382);
noStroke();
circle(width / 2, 2/3 * height, 40, 40);
fill(77, 208, 99);
circle(width / 2, 2/3 * height, 25, 25);
fill(255, 51, 51);
circle(width / 2, 2/3 * height, 10, 10);
}
//flower 3
if(mouseX > 400 & mouseY <= (4/5 *height)){
stroke("green");
line(3/4 * width, 4/5 * height, 3/4 * width, mouseY);
noStroke();
fThree = 3/4 * width;
fill(0, 128, 255);
circle(fThree + 20, mouseY - 17, 25);
circle(fThree - 20, mouseY - 17, 25);
circle(fThree + 20, mouseY + 17, 25);
circle(fThree - 20, mouseY + 17, 25);
fill(0, 51, 102);
ellipse(fThree, mouseY - 17, 20, 30);
ellipse(fThree - 20, mouseY, 30, 20);
ellipse(fThree + 20, mouseY, 30, 20);
ellipse(fThree, mouseY + 17, 20, 30);
fill(255, 153, 204);
circle(3/4 * width, mouseY, 30);
fill(255, 51, 153);
stroke(204, 204, 255);
strokeWeight(4);
circle(3/4 * width, mouseY, 20);
}else{
strokeWeight(4);
stroke("green");
line(3/4 * width, 4/5 * height, 3/4 * width, 2/3 * height);
fill(255);
noStroke();
noStroke();
push();
translate(450, 300);
rotate(radians(angle));
fill(0, 128, 255);
circle(20, -17, 25);
circle(-20, -17, 25);
circle(20, 17, 25);
circle(-20, 17, 25);
fill(0, 51, 102);
ellipse( 0, -17, 20, 30);
ellipse(-20, 0, 30, 20);
ellipse(20, 0, 30, 20);
ellipse(0, 17, 20, 30);
fill(255, 153, 204);
circle(0, 0, 30);
fill(255, 51, 153);
stroke(204, 204, 255);
strokeWeight(4);
circle(0, 0, 20);
pop();
angle += 2
}
//cloud follows mouse back and forth
if (mouseX > x) {
x += 0.5;
offset = -5;
}
if (mouseX < x) {
x -= 0.5;
offset = 5;
}
if (x > 599){ //constrain to frame
x = 599;
}
fill(255);
noStroke();
ellipse(x, 100, 100);
ellipse(x + 50, 110, 70);
ellipse(x - 50, 110, 70);
//if cloud covers sun, screen gets darker
var shadeCover = 20;
if (x <= 125 & x >= 30){
background(0, 0, 102, shadeCover);
}
}