With this project, I mainly wanted to create different color modes from the pixels. The background is black and white, the 1st set of selected pixels are the original colors, and when clicked the pixels become inverted.
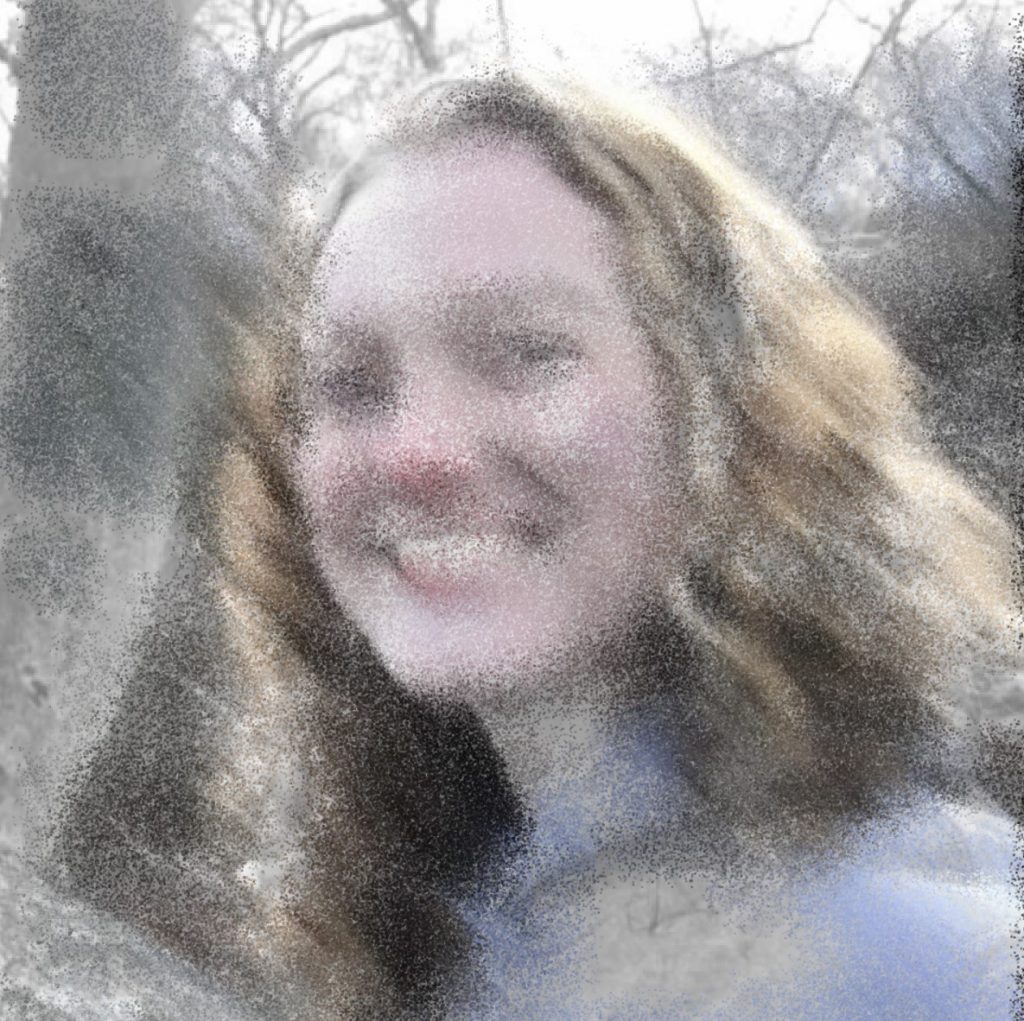
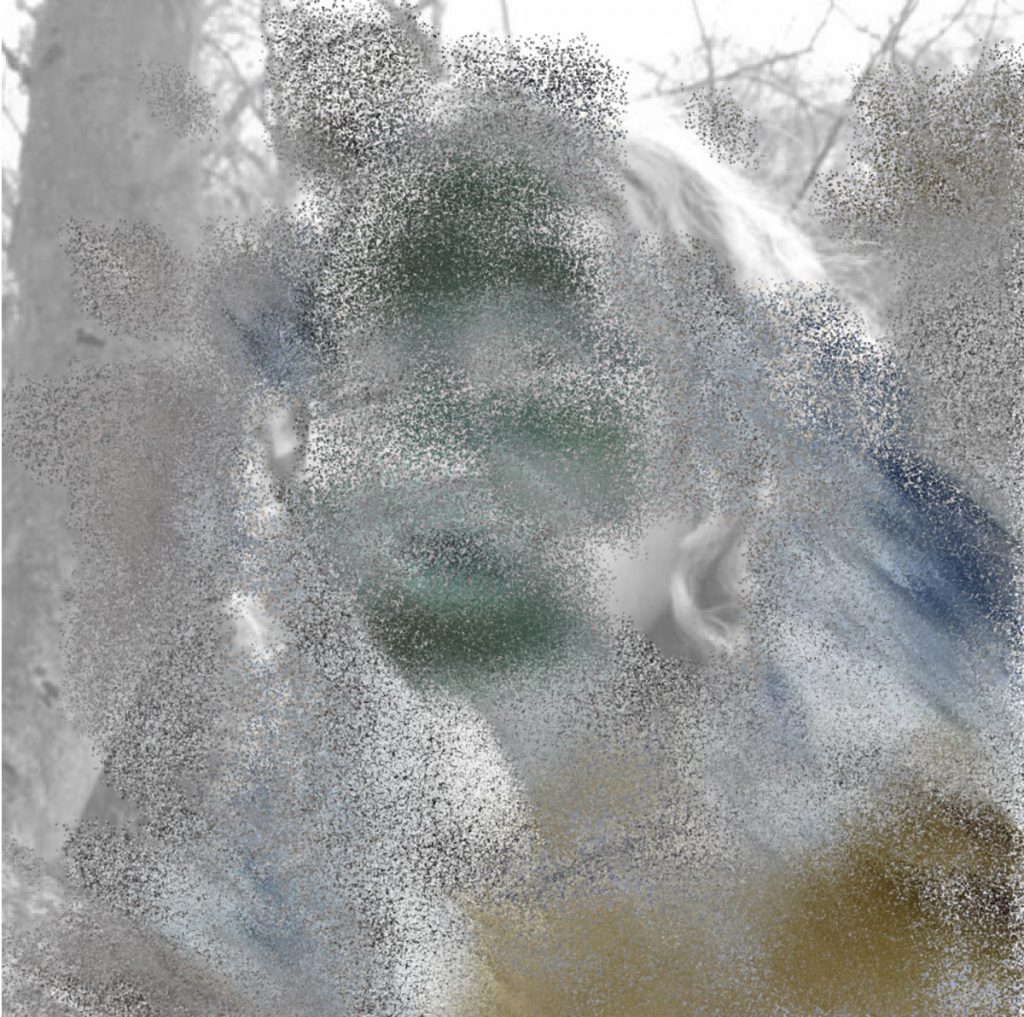
sketch
// Emily Franco
// Section C
// efranco@andrew.cmu.edu
// Assignment-09
var sqr = [];
var sqrArrayLen = 150;
//true = original colors false = inverted
var colorStyle = true;
var count = 0;
function preload(){
face = loadImage("https://i.imgur.com/bu90lcN.jpg");
}
//constructor for square objects
function makeSquare(cx, cy, wdth){
var c = {x:cx, y:cy, w:wdth}
return c;
}
function setup(){
createCanvas(480,480);
background("white");
face.loadPixels();
face.resize(480,600);
greyscale();
//define objects in sqr array
for(var i=0;i<=sqrArrayLen-1;i++){
var side1 = random(10,100);
var x = random(width);
var y = random(height);
var square = makeSquare(x,y,side1);
sqr.push(square);
}
}
function draw() {
noFill();
stroke("white");
//draw 1st image only once
if(count<=0){
drawAlterations()
}
count++;
noLoop();
}
//draw changed made to image
function drawAlterations(){
var opacity;
var shift;
var c;
for(var i=0; i<=sqr.length-1;i++){
noStroke();
if(colorStyle){
showColor(i);
}else{
invertColors(i);
}
print(colorStyle);
}
}
//make image greyscale
function greyscale(){
for (var i=0;i<=face.width;i++){
for(var j=0;j<=face.height;j++){
c = face.get(i,j);
var grey = c[0];
push();
noStroke();
fill(grey,150);
square(i,j,1);
pop();
}
}
}
//change pixel color back to original only in boxed area
function showColor(index){
var maxX = sqr[index].x + sqr[index].w;
var maxY = sqr[index].y + sqr[index].w;
for (var i=sqr[index].x;i<=maxX;i++){
for(var j=sqr[index].y;j<=maxY;j++){
c = face.get(i,j);
opacity = random(50,150);
shift = random(-10,10);
fill(c[0],c[1],c[2],opacity);
square(i+shift,j+shift,1);
}
}
}
function invertColors(index){
var maxX = sqr[index].x + sqr[index].w;
var maxY = sqr[index].y + sqr[index].w;
for (var i=sqr[index].x;i<=maxX;i++){
for(var j=sqr[index].y;j<=maxY;j++){
c = face.get(i,j);
var r = 255-c[0];
var g = 255-c[1];
var b = 255-c[2];
opacity = random(50,150);
shift = random(-10,10);
fill(r,g,b,opacity);
square(i+shift,j+shift,1);
}
}
}
function mousePressed(){
colorStyle = !colorStyle;
drawAlterations();
}