One Night on the Farm
One night on the farm, the farmer left the animals out. The crickets were chirping and the chickens were clucking. A whirring hum was heard and an alien space ship appeared. The alien first abducted the sheep who let out a surprised “baa” as he rose into the air and shrunk. The pig was next. He let out a startled “oink” as he was lifted away from his mud. Then it was the cows turn. He let out a shocked “moo” before being whisked away. The space ship left with its new specimens, and the crickets chirped and the chickens clucked some more.
The farm images are licensed from Adobe stock images. The sounds used are from freesound.org and edited with Audacity. The sounds are used under the Creative Commons license. The owners did not want attribution. The sounds are: crickets.wav – crickets chirping, cluckcluck.wav – chickens clucking, spaceShip.wav – space ship sounds, moo.wav – a cow, baa.wav – a sheep, oink.wav – a pig.
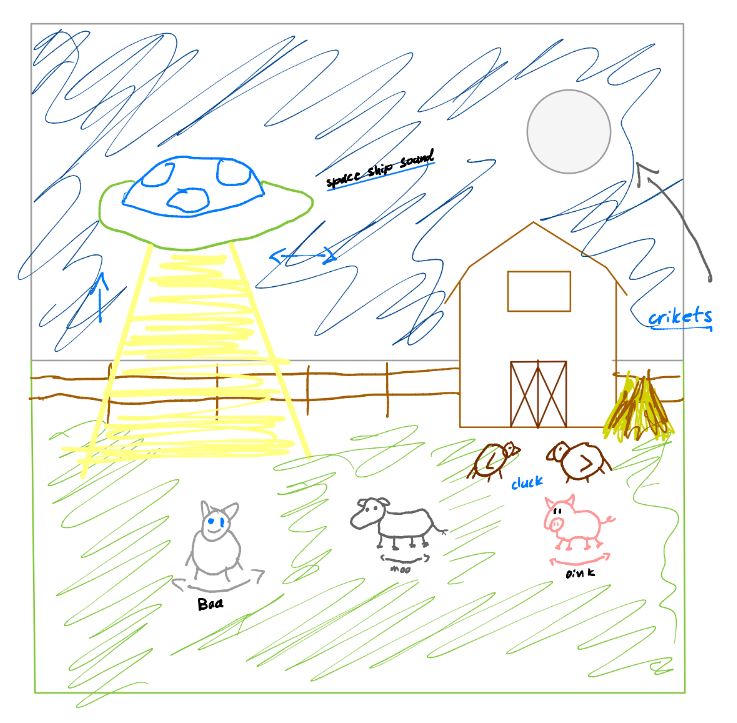
/* Evan Stuhlfire
* estuhlfi@andrew.cmu.edu Section B
* Project-10: Sonic Story - One Night on the Farm
Assignment-10: Sonic Story
One Night on the Farm
One night on the farm, the farmer left the animals
out. The crickets were chirping and the chickens were
clucking. A whiring hum was heard and an alien space ship appeared.
The alien first abducted the sheep who let out a surprised "baa" as he
rose into the air and shrunk. The pig was next. He let out a startled
"oink" as he was lifted away from his mud. Then it was the cows turn.
He let out a shocked "moo" before being whisked away. The
space ship left with its new specimens, and the crickets chirped
and the chickens clucked some more.
The farm images are liscensed from Adobe stock images. The sounds used are from freesound.org and edited with Audacity.
The sounds are used under the Creative Commons liscense. The owners did not
want attribution. The sounds are:
crickets.wav - crickets chirping, cluckcluck.wav - chickens clucking,
spaceShip.wav - space ship sounds, moo.wav - a cow, baa.wav - a sheep,
oink.wav - a pig.
*/
var farmImg = []; // an array to store the images
var farmObjArray = []; // array to store images
// sound variables
var crickets;
var cluck;
var baa;
var moo;
var oink;
var spaceShip;
// starting sky and foreground colors
var rNight = 50;
var gNight = 100;
var bNight = 150;
var rFore = 50;
var gFore = 160;
var bFore = 80;
var moon = 100;
// position of the moon
var xMoon;
var yMoon;
// map to array indexes
var fence = 0;
var barn = 1;
var hay = 2;
var cow = 3;
var pig = 4;
var sheep = 5;
var chicken = 6;
var rooster = 7;
var ship = 8;
function preload(){
// These URLs are images stored on imgur
// images liscensed from Adobe Stock images
// preload farm images
var filenames = [];
filenames[fence] = "https://i.imgur.com/bbBOrZ7.png";
filenames[barn] = "https://i.imgur.com/inS5Xdt.png";
filenames[hay] = "https://i.imgur.com/IXPEVak.png";
filenames[cow] = "https://i.imgur.com/8XjQyMt.png";
filenames[pig] = "https://i.imgur.com/7VPvVRB.png";
filenames[sheep] = "https://i.imgur.com/vIFlqDY.png";
filenames[chicken] = "https://i.imgur.com/vdTxUKf.png";
filenames[rooster] = "https://i.imgur.com/SCYoQoX.png";
filenames[ship] = "https://i.imgur.com/lAHddxj.png"
// load farm images
for (var i = 0; i < filenames.length; i++) {
farmImg[i] = loadImage(filenames[i]);
}
// load sounds
crickets = loadSound("https://courses.ideate.cmu.edu/15-104/f2022/wp-content/uploads/2022/11/crickets2.wav");
cluck = loadSound("https://courses.ideate.cmu.edu/15-104/f2022/wp-content/uploads/2022/11/cluckcluck.wav");
baa = loadSound("https://courses.ideate.cmu.edu/15-104/f2022/wp-content/uploads/2022/11/baa.wav");
moo = loadSound("https://courses.ideate.cmu.edu/15-104/f2022/wp-content/uploads/2022/11/moo.wav");
oink = loadSound("https://courses.ideate.cmu.edu/15-104/f2022/wp-content/uploads/2022/11/oink.wav");
spaceShip = loadSound("https://courses.ideate.cmu.edu/15-104/f2022/wp-content/uploads/2022/11/spaceShip.wav");
}
function stepImage() {
// // move and grow the space ship to get the sheep
if(this.imageNum == ship & this.moving) {
if(this.target == sheep) {
if(this.x < width/4) {
this.x += this.speed;
}
if(this.y < height/2.5) {
this.y += this.speed;
}
if(this.ySize <= 100) {
this.ySize += this.speed;
}
if(this.xSize <= 200) {
this.xSize += this.speed;
} else {
if(this.drawBeam) {
drawBeam(this.x, this.y, this.target);
}
}
} else if(this.target == pig) {
// move the space ship to get the pig
this.drawBeam = true; // turn the beam back on
if(this.ySize >= 80) {
this.ySize -= this.speed;
}
if(this.xSize >= 180) {
this.xSize -= this.speed;
}
if(this.y > height/3) {
this.y -= this.speed;
}
if(this.x <= width/2) {
this.x += this.speed;
} else {
if(this.drawBeam) {
drawBeam(this.x, this.y, this.target);
}
}
} else if(this.target == cow) {
// move the space ship to get the cow
this.drawBeam = true; // turn the beam back on
if(this.ySize <= 120) {
this.ySize += this.speed;
}
if(this.xSize <= 240) {
this.xSize += this.speed;
}
if(this.y < height/1.8) {
this.y += this.speed;
}
if(this.x >= width/4) {
this.x -= this.speed;
} else {
// the ship is in the correct location for the beam
if(this.drawBeam) {
drawBeam(this.x, this.y, this.target);
}
}
} else if(this.target < cow) {
// fly away
if(this.ySize >= 0) {
this.ySize -= this.speed;
}
if(this.xSize >= 0) {
this.xSize -= this.speed;
}
if(this.y > 0) {
this.y -= this.speed;
}
if(this.x <= width) {
this.x += this.speed;
}
}
}
// when an animal is being abducted it shrinks and rises into the ship
if(this.imageNum == farmObjArray[ship].target & this.moving) {
// decrease y to rise into the ship
if(this.y >= farmObjArray[ship].y) {
this.y -= this.speed;
} else {
// turn the beam off and set target to pig
farmObjArray[ship].drawBeam = false;
// don't decrease the target below the cow index
if(farmObjArray[ship].target >= cow) {
farmObjArray[ship].target--;
}
// stop drawing this image
this.moving = false;
this.drawOn = false;
}
// shrink
if(this.xSize > 0) {
this.xSize *= .95;
}
if (this.ySize > 0) {
this.ySize *= .95;
}
if(this.imageNum == sheep & frameCount % 15 == 0) {
baa.play();
} else if(this.imageNum == pig & frameCount % 15 == 0) {
oink.play();
} else if(this.imageNum == cow & frameCount % 12 == 0) {
moo.play();
}
}
}
function drawImage() {
// draw image
if(this.drawOn){
image(farmImg[this.imageNum], this.x, this.y, this.xSize, this.ySize);
}
}
// Constructor for each farm image
function makeObj(cx, cy, imNum, xs, ys) {
var c = {x: cx, y: cy, xSize: xs, ySize: ys,
imageNum: imNum,
moving: false,
drawBeam: true,
target: sheep,
speed: 5,
drawOn: true,
stepFunction: stepImage,
drawFunction: drawImage
}
return c;
}
function setup() {
createCanvas(480, 480);
// set farm colors
background(rNight, gNight, bNight);
foreground(rFore, gFore, bFore);
// set initial moon position
xMoon = width + 25;
yMoon = height/2.25;
xShip = width/4;
yShip = height/2 + 50;
imageMode(CENTER);
// create array of farm objects
createFarmObjs();
frameRate(10);
useSound();
}
function soundSetup() { // setup for audio generation
crickets.setVolume(0.1);
cluck.setVolume(0.3);
baa.setVolume(0.3);
moo.setVolume(0.5);
oink.setVolume(0.3);
spaceShip.setVolume(0.5);
}
function draw() {
noStroke();
background(rNight, gNight, bNight);
foreground(rFore, gFore, bFore);
makeSounds();
var currentImage;
// loop over farm images and draw them
for(var i = 0; i < farmObjArray.length; i++) {
currentImage = farmObjArray[i];
// draw the farm
currentImage.stepFunction();
currentImage.drawFunction();
}
// darken to night sky
fadeNightSky();
}
function makeSounds() {
// make sounds at frameCount intervals
// play crickets
if(frameCount >5 & frameCount < 350 && frameCount % 25 == 0) {
crickets.play();
}
// play cluck cluck
if ((frameCount > 24 & frameCount < 350 && frameCount % 50 == 0)) {
cluck.play();
}
if(frameCount > 24 & frameCount < 200 && frameCount % 25 == 0) {
spaceShip.play();
}
if(frameCount > 400){
// stop all sounds
crickets.stop();
cluck.stop();
spaceShip.stop();
baa.stop();
moo.stop();
oink.stop();
// stop looping
noLoop();
}
}
function createFarmObjs() {
// create objects for farm: fence, barn, hay
// set initial positions xPos and yPos
var xPos = width/1.25;
var yPos = height/2;
// set initial size
var xs = 125;
var ys = 125;
// create farm objects
farmObjArray[fence] = makeObj(width/2, yPos + 20, fence, xs * 5,
ys * .25);
farmObjArray[barn] = makeObj(xPos, yPos, barn, xs, ys);
farmObjArray[hay] = makeObj(xPos + 50, yPos + 50, hay, xs/2, ys/2);
farmObjArray[cow] = makeObj(width/4, height/1.2, cow, 100, 70);
farmObjArray[pig] = makeObj(width/2, height/1.68, pig, 55, 45);
farmObjArray[sheep] = makeObj(width/4, height/1.65, sheep, 30, 50);
farmObjArray[chicken] = makeObj(width/1.2, height/1.35,
chicken, 25, 35);
farmObjArray[rooster] = makeObj(width/1.3, height/1.45,
rooster, 30, 40);
farmObjArray[ship] = makeObj(0, height/8, ship, 20, 1);
}
function drawBeam(x, y, target) {
// draw beam
fill(240, 250, 240, 80);
triangle(x, y, x + 65,
farmObjArray[target].y + 40,
x - 65,
farmObjArray[target].y + 40);
// set target to moving
farmObjArray[target].moving = true;
}
function fadeNightSky() {
// decrease the background colors to darken the sky to night
var offset = 5;
rNight -= offset;
gNight -= offset;
bNight -= offset;
rNight = max(0, rNight);
gNight = max(0, gNight);
bNight = max(0, bNight);
// draw moon
if(rNight == 0) {
fill(moon);
ellipse(xMoon, yMoon, 50);
moon++;
moon = min(moon, 245);
xMoon -= offset;
yMoon -= offset;
xMoon = max(width/1.35, xMoon);
yMoon = max(height/7.75, yMoon);
}
// when it is dark start moving space ship
if(bNight == 0) {
farmObjArray[ship].moving = true;
}
}
function foreground(r, g, b) {
// create lawn and mud puddle for pig
fill(r, g, b);
rect(0, height/2, width, height/2);
fill(90, 80, 15);
ellipse(width/2 - 10, height/1.6, 150, 25);
}