I created my self-portrait using different symbols that are linked to the pixel colors on the image. When the mouse is pressed, the shapes are drawn to create the image.
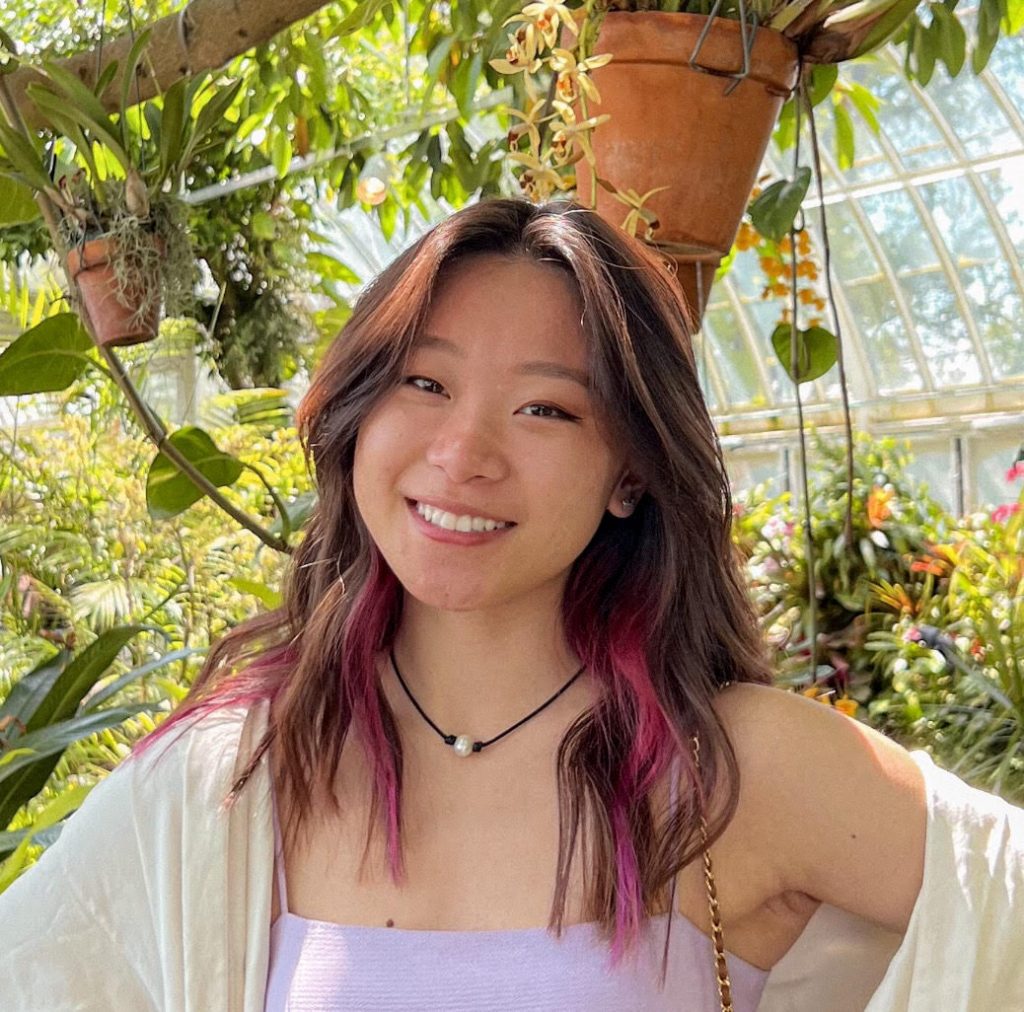
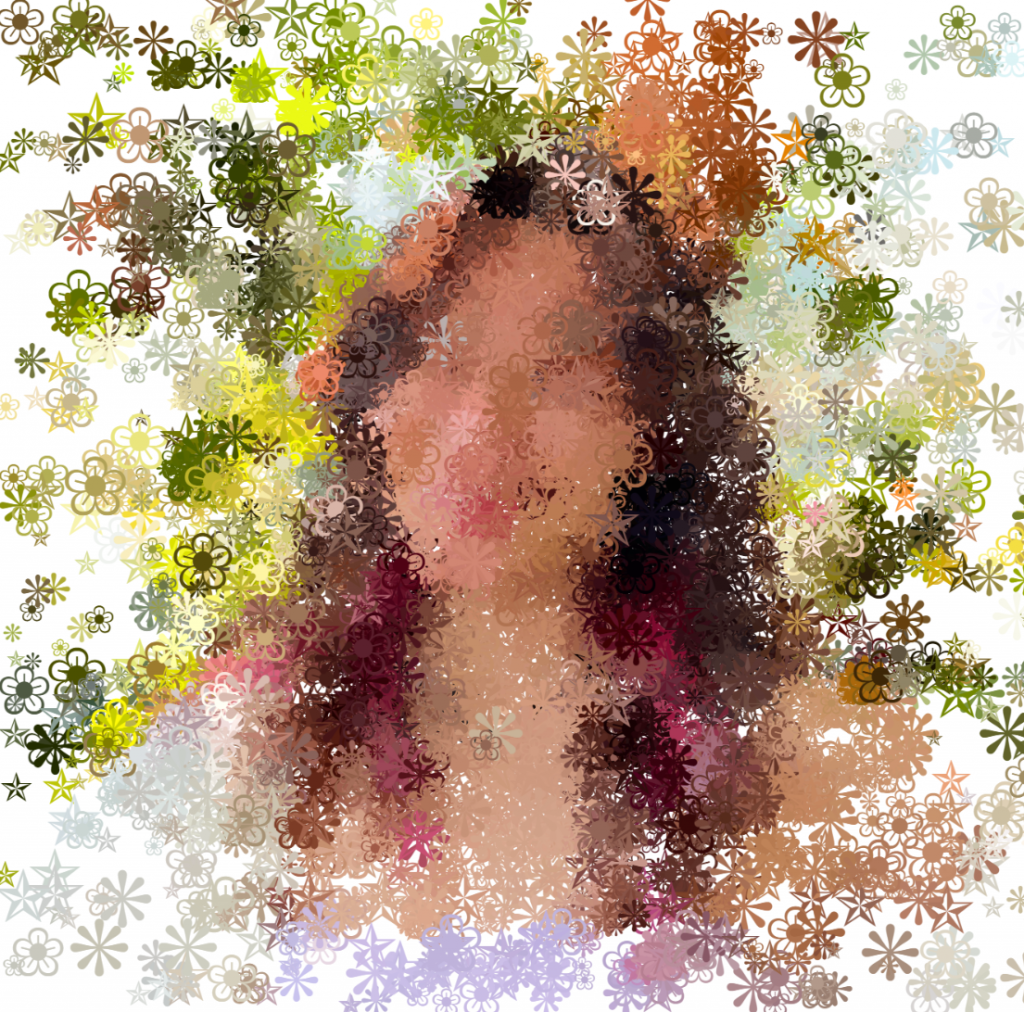
//amy hu
//amyhu
//section d
var img;
var smallPoint, largePoint;
var symbols = ["❀", "❋", "✯"]
function preload() {
img = loadImage('https://i.imgur.com/2ZzOdG6.png');
}
function setup() {
createCanvas(450,450);
// smallPoint = 5;
// largePoint = 20;
imageMode(CENTER);
noStroke();
background(255);
img.loadPixels();
}
function draw() {
//mouse location determines point size
var pointillize = map(mouseX, 0, width, smallPoint, largePoint);
//picks random x y value
var x = floor(random(img.width));
var y = floor(random(img.height));
//gets pixel color form a xy value
var pix = img.get(mouseX, mouseY);
//fills pixel color
fill(pix, 128);
ellipse(x, y, pointillize, pointillize);
//draws symbols when mouse is pressed
if(mouseIsPressed){
textSize(random(10,35));
text(random(symbols), mouseX, mouseY);
}
}