// Your name: Ashley Su
// Your andrew ID: ashleysu
// Your section (C):
var Man;
var grass = 0.4;
var grassSpeed = 0.07;
var mountainFront = 0.008;
var mountainFrontSpeed = 0.0005;
var mountainBack = 0.01;
var mountainBackSpeed = 0.0002;
var bubbles = [];
function setup() {
createCanvas(480, 480);
frameRate(20);
// setting up bubble array
for (var i = 0; i < 9; i++){
var bubblesX = random(width);
var bubblesY = random(0, 200);
bubbles[i] = makebubbles(bubblesY, bubblesX);
}
}
function draw(){
background(221,191,218);
Sun();
MountainBack();
MountainFront();
Grass();
image(Man,mouseX,300,80,80);
updatebubbles();
addbubbles();
}
// draw sun
function Sun(){
noStroke();
fill(250,70,22,120);
ellipse(350, 200, mouseX+500);
fill(250,70,22,40);
ellipse(350, 200, mouseX+350);
fill(250,70,22,100);
ellipse(350, 200, mouseX+200);
}
//pizza man loading
function preload(){
Man = loadImage("https://i.imgur.com/9AUtJm7.png");
}
//draws first mountain
function MountainBack(){
noStroke();
fill(0,109,87);
beginShape();
for (var i = 0; i < width; i ++){
var x = (i * mountainBack) + (millis() * mountainBackSpeed);
var y = map(noise(x), 0, 1.5, 50, 300);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
// drawing second mountain in front of first mountain
function MountainFront(){
noStroke();
fill(208,240,192);
beginShape();
for (var i = 0; i < width; i ++){
var x = (i * mountainFront) + (millis() * mountainFrontSpeed);
var y = map(noise(x), 0, 1.2, 150, 250);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
// draws the grass
function Grass(){
noStroke();
fill(156,176,113);
beginShape();
for (var i = 0; i < width; i ++){
var x = (i * grass) + (millis() * grassSpeed);
var y = map(noise(x), 0, 1.1, 350, 370);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
//constructing the bubbles as objects
function makebubbles(bubblesX, bubblesY){
var bubbles = {
x: bubblesX,
y: bubblesY,
velocity: random(3, 8),
size: random(5, 15),
move: movebubbles,
show: showbubbles,
}
return bubbles;
}
// defining the speed of bubbles
function movebubbles(){
this.x -= this.velocity;
this.y -= this.velocity / random(5, 20);
}
// defning bubbles characteristics
function showbubbles(){
strokeWeight(1);
stroke('LightBlue');
fill(101,208,255,80);
circle(this.x, this.y, this.size);
curve(this.x + this.size, this.y, this.size, this.size/2);
}
//telling bubbbles to move on the screen
function updatebubbles(){
for(i = 0; i < bubbles.length; i++){
bubbles[i].move();
bubbles[i].show();
}
}
// making bubbles contiuesly move accross the screen
function addbubbles(){
if (random(0,2) < 0.5){
var bubblesX = width;
var bubblesY = random(0, height/2);
bubbles.push(makebubbles(bubblesX, bubblesY));
}
}
Author: asu
Looking Outwards Blog 11 – Societal Impacts of Digital Art
The article I read this week discussed the copyrighting of NFTs. What I found interseting about this article’s conclusion was that it directed most of the issues towards the lack of secruity systems. The author states in the end of the article that the digital platforms where NFTs are passed around are not advanced enough to ensure that a peice of work is is properly insured. However, there was little to no discussion over the ethical and moral aspect of the impacts of copyrighting in the digtial world. I think that there is a sense of pushing around the responsiblity from the people who are taking artwork to the people creating systems to prevent the copyrighting of artwork. This issue not only applies in this situation, but many other societal issues as well. Instead of looking at solutions to stop copyrighting, the article discusses possible solutions for copyrighting to not make it past their system rather than stopping it from ever happening.
Project 09: Computational Portrait
For my image, I noticed that the background lights were blurry, and I wanted to implement that in the code with mousPressesed. So each time you use you press the mouse, a filter is applied to the whole canvas and the whole canvas gets a bit blurry. Additionally, I wanted there to be a difference in shapes for the background and the person.
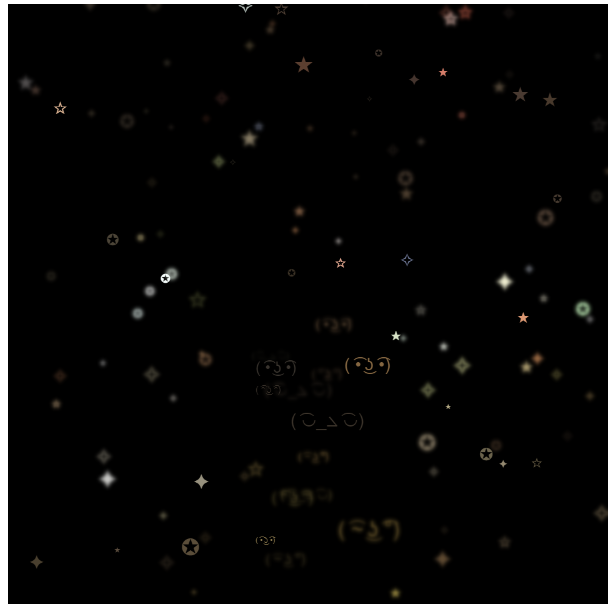
Small Blur
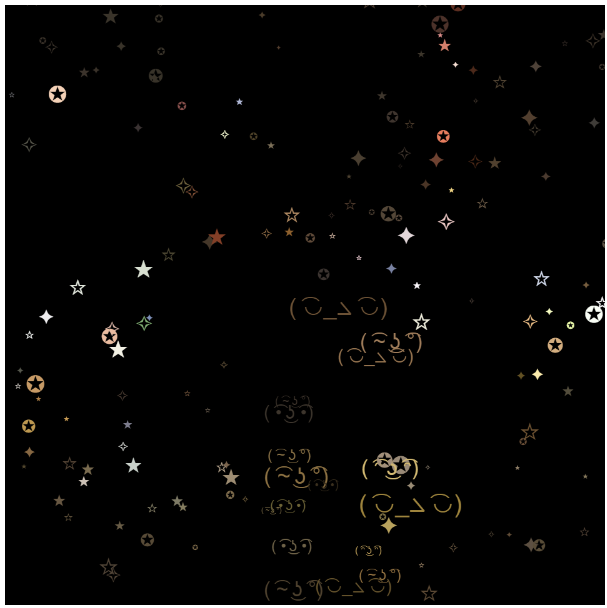
No Blur
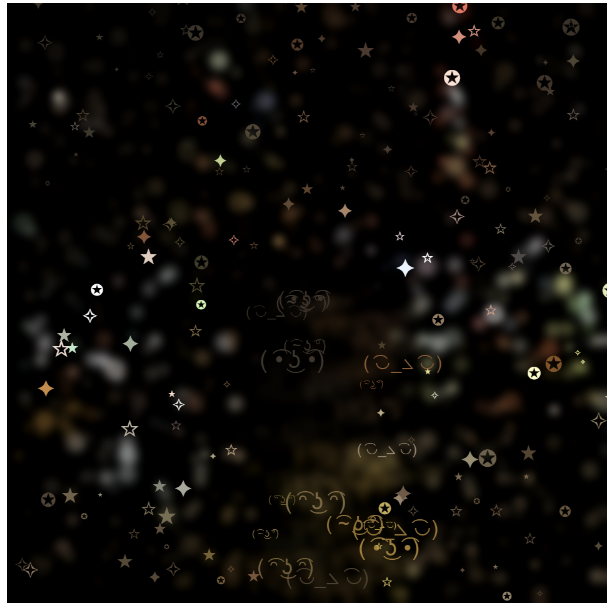
Medium Blur
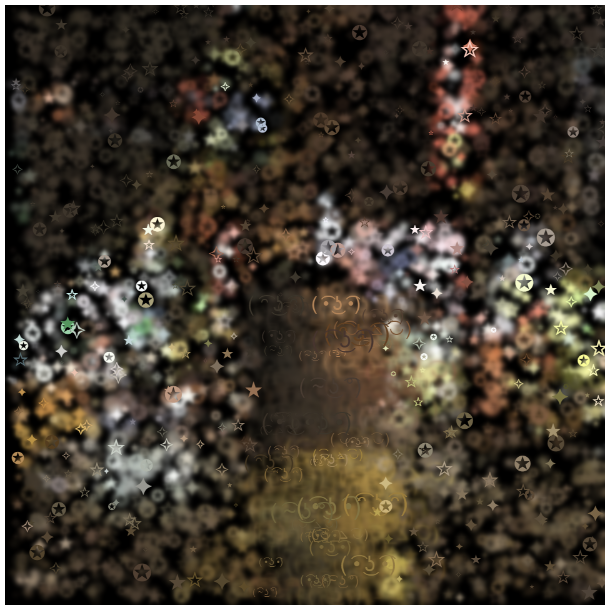
Lots of Blur
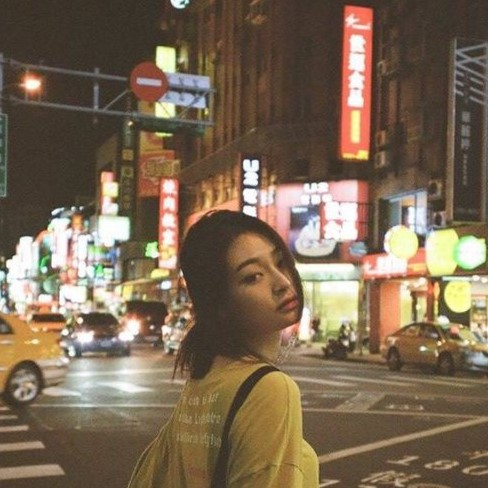
Image
// Your name: Ashley Su
// Your andrew ID: ashleysu
// Your section (C):
var img;
var stars = [" ★ ", " ☆ "," ✪ "," ✦ ", " ✧ "]
var faces = ['( ͡• ͜ʖ ͡•)', '( ͡ᵔ ͜ʖ ͡ᵔ)', '( ͡~ ͜ʖ ͡°)', '( ͡◡_⦣ ͡◡)']
function preload() {
img = loadImage("https://i.imgur.com/spqIDsb.jpeg")
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
img.loadPixels();
img.resize(480, 480); //resize image to canvas
frameRate(50000);
background(0);
}
function draw() {
//pick random x and y
var x = floor(random(img.width));
var y = floor(random(img.height));
//pull pixel color from that x and y
var pixelColor = img.get(x, y);
//fill using the pixel color
fill(pixelColor);
if (x>((width/2)-50) & x<((width/2)+50) && y>height/2 && y < height) { //setting the faces smilies to be next where the actaul face is
strokeWeight(5);
textSize(random(5, 20)); // smaller near face
text(random(faces), x, y);
} else {
textSize(random(5, 20));
text(random(stars), x, y); //draws stars everywhere else
}
}
function mousePressed() {
filter(BLUR,3); // when mouse is pressed, it blurs the layer
}
Looking Outwards 09 : A Focus on Women and Non-binary Practitioners in Computational Art
The work I chose to look into for this week was the project called ‘Reverb’ by Madlab.CC. MadLab.CC is a research studio that aims to invent better ways to communicate with machines that make wearable crafts. MadLab.CC is headed by Madeline Gannon who is a researcher, designer, and educator that gradated with a PhD in Computational Design from CMU. She is also the founder and principal researcher at Atonation which is separate studio that aims to combine research, and functional alternate futures. Madlab.CC is headquartered in Pittsburgh, but she also gives lectures and talks around the whole world at various conferences.
The project, Reverb, is a context-aware 3D modeling environment that lets one personalize a piece of wearable print to their own body. The technology behind it consist of computer vision, digital design, and digital fabrication that ultimately translates the physical assets into printable geometry. Something I admire about the aspects of this project is the way the computational geometries are informed by human contours and gestures. This makes the technology feel as an integrated conversation with the real-world. For the general person, there is a sense of customization and having something “one of a kind”. Rather than fitting the human into some kind of computational form, the form is the one fitting around the human.
Link : http://www.madlab.cc/reverberating-across-the-divide
Looking Outwards 08: The Creative Practice of an Individual
The work I chose to look into for this week is from Mike Tucker. Specifically the project he did at Magic Leap. Mike Tucker describes himself as an interactive director, designer & developer. The project was an interactive an immersive space that combined art and music. More specifically, Mike teamed up with Sigur Ros to manifest the DNA of a new sound in a new reality. The website write, “Tónandi, which translates to sound spirit in Icelandic, is an interactive audio-visual exploration of the sounds and spirit of Sigur Rós.” What I admire most about Tucker’s project is that it combines art from three different sensory fields. As art and music can be seen as forms that fill and take up space, using touch to generate and change that space makes this project both complex but also grounded in the everyday. Although it is taking a big step into a world that is heavily based on computer generated data, the way the work is both immersive and grounded in the human experiences of the senses makes the piece feel more real then a lot of the other VR projects I’ve seen and read about. Mike Tucker, seen in his lecture, presented his work very pragmatically. He first begun by explaining the ‘why’ in his project followed by the ‘how’. Making sure every detail of the work was explained including the specifics of the technology. What I think made his presentation effective was the way he reached into the world of possibilities within the listener’s mind. He combined visual “proof” that essentially backed up what he was trying o achieve on the philosophical level. In addition, Tucker used key words such as “new reality” and “tomorrow” which hint at this sense of reachability and accessibility to the everyday person. Almost as if he was saying that dreams do come true. Making his work that much more appealing to the public.
Website link: https://mike-tucker.com/
Project link: https://world.magicleap.com/en-us/details/com.magicleapstudios.tonandi
Pacman Clock
For this assignment, I thought it would be cool to build off last week’s wallpaper and make my Pacman a dynamic clock. It is supposed to be read left to right, then down. So the two horizontal Pacmans are the hour and minute (top and bottom) and the vertical Pacman is the seconds.
// Your name: Ashley Su
// Your andrew ID: ashleysu
// Your section (C):
function setup() {
createCanvas(450,590);
}
function dotGrid() {
var PADDING = 20;
var ROWS =19;
var COLUMNS =13;
var CELL_COLOR = 'Blue'
for (var col=0;col<COLUMNS;col++) {
for (var row=0;row<ROWS;row++) {
var left = PADDING+(col*34);
var top = PADDING+(row*34.3);
fill(random(255),random(255),random(255))
circle(left,top,10);
}
}
}
function blueBaracades() {
var PADDING = 40;
var ROWS = 4;
var COLUMNS = 3;
var CELL_SIZE = 140;
var CELL_COLOR = 'Blue'
fill(0);
stroke('Blue')
strokeWeight(3);
for (var col=0;col<COLUMNS;col++) {
for (var row=0;row<ROWS;row++) {
var left = PADDING+(col*CELL_SIZE);
var top = PADDING+(row*CELL_SIZE);
var size = CELL_SIZE - 50;
rect(left,top,size,size);
}
}
}
function pacmanH(h){
fill('yellow');
noStroke();
push() //hour
translate(10,height/3.2);
rotate(radians(310));
arc(h*10,h*10,35,35,radians(80),radians(35)/2); // increments by *10 pixels to the right
pop();
}
function pacmanM(m){
fill('yellow');
noStroke();
push(); // minute
translate(width,height/1.33);
rotate(radians(140));
arc(m*4,m*4,35,35,radians(80),radians(20)/2); // increments by *4 pixels to the left
pop();
}
function pacmanS(s){
fill('yellow');
noStroke();
push(); // second
translate(2*width/3-7,height/26);
rotate(radians(45));
arc(s*6,s*6,35,35,radians(80),radians(20)/2); // increments by *6 pixels down
pop();
}
function draw(){
background('Black');
dotGrid();
blueBaracades();
var s = second();
var h = hour();
var m = minute();
pacmanH(h);
pacmanM(m);
pacmanS(s);
}
Looking Outwards 06 – Mr.Doodle
The artwork I immediately thought of was probably anything by Mr. Doodle. The earliest memory I have of seeing his work was when he had a small Instagram following about 7 years ago. His artwork is comprised of drawing lines and characters seemingly randomly across a page, or really any surface. The only rule he seems to follow is that every inch of the surface must be covered. But I think is interesting about his work is how in his randomness, you are searching for something. Almost like you are looking for a character or a pattern or any type of regulation. I think this differs from other artworks because although his work has little characters and themes, the way he approaches these pieces is still very random. He has no plan or logic of what goes where and why. I think randomness can be blurry in the art world in terms of whether or not it is a justifiable reason for a certain design choice, but in Mr. Doodle’s case, he is using that randomness to his advantage.
Link : https://www.designboom.com/art/watch-mr-doodle-mansion-black-white-cartoonish-signature-10-05-2022/
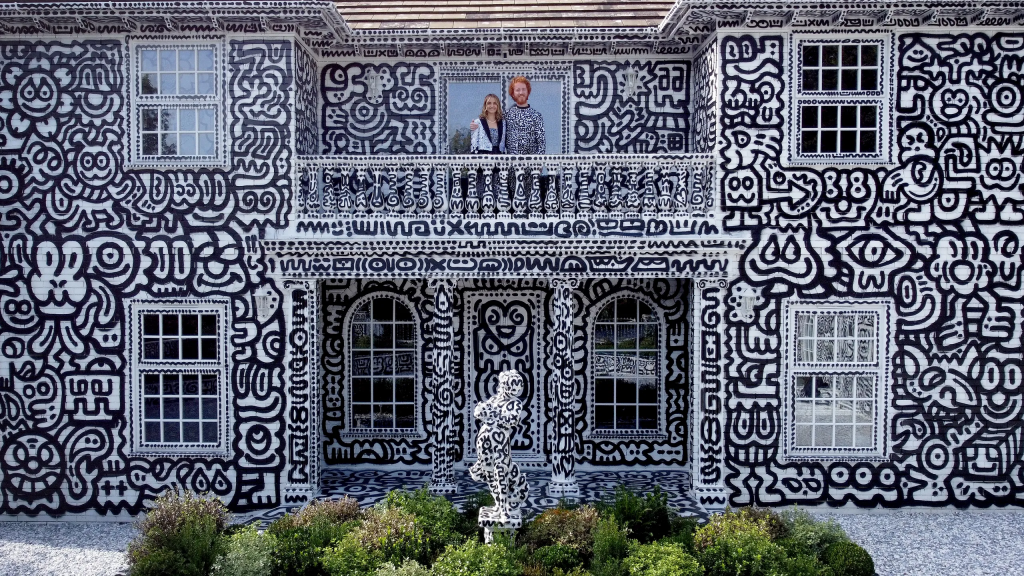
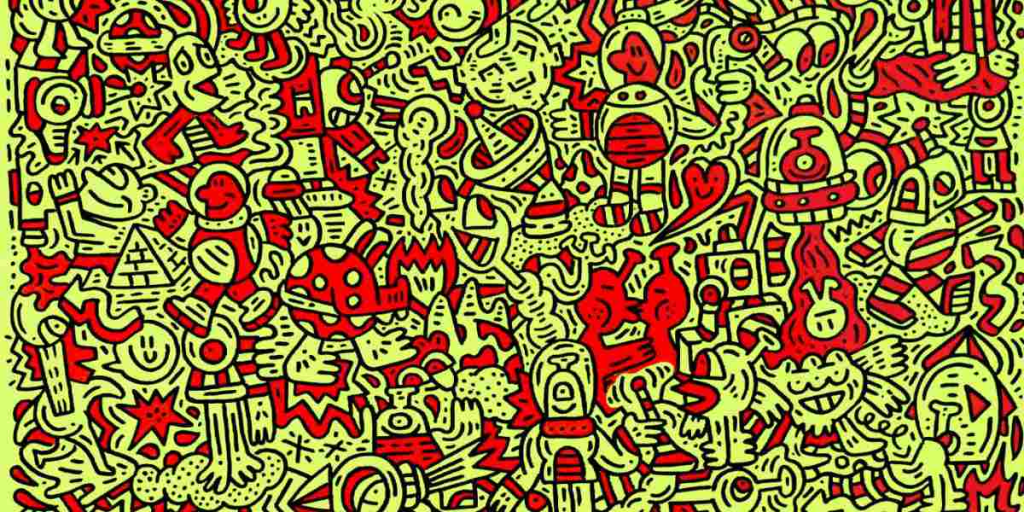
Project 05- Pacman Wallpaper
Initially, I wanted to make a wallpaper that resembled Pacman. I approached the assignment by using for loops to create 2 sets of grids, the first was the dots that Pacman eats, and the second is the grid for the blue squares.
// Your name: Ashley Su
// Your andrew ID: ashleysu
// Your section (C):
function setup() {
createCanvas(450,590);
background('Black');
}
function dotGrid() {
var PADDING = 20;
var ROWS =19;
var COLUMNS =13;
var CELL_COLOR = 'Blue'
for (var col=0;col<COLUMNS;col++) {
for (var row=0;row<ROWS;row++) {
var left = PADDING+(col*34);
var top = PADDING+(row*34.3);
fill(random(255),random(255),random(255))
circle(left,top,10);
}
}
}
function blueBaracades() {
var PADDING = 40;
var ROWS = 4;
var COLUMNS = 3;
var CELL_SIZE = 140;
var CELL_COLOR = 'Blue'
fill(0);
stroke('Blue')
strokeWeight(3);
for (var col=0;col<COLUMNS;col++) {
for (var row=0;row<ROWS;row++) {
var left = PADDING+(col*CELL_SIZE);
var top = PADDING+(row*CELL_SIZE);
var size = CELL_SIZE - 50;
rect(left,top,size,size);
}
}
}
function pacmans(){
fill('yellow');
noStroke();
push()
translate(width/5,height/4+7);
rotate(radians(310));
arc(0,0,35,35,radians(80),radians(35)/2);
pop();
push();
translate(width/3*2,height/2);
rotate(radians(140));
arc(0,0,35,35,radians(80),radians(20)/2);
pop();
push();
translate(width/3+4,height-height/6);
rotate(radians(230));
arc(0,0,35,35,radians(80),radians(20)/2);
pop();
push();
translate(width-width/7,height-20);
rotate(radians(140));
arc(0,0,35,35,radians(80),radians(20)/2);
pop();
push();
translate(width-20,height/26);
rotate(radians(45));
arc(0,0,35,35,radians(80),radians(20)/2);
pop();
}
function draw(){
dotGrid();
blueBaracades();
pacmans();
noLoop();
}
Looking Outwards 05
The artwork I came across while looking into 3D computer graphics was a series of pieces created by the studio Nervous System. The studio takes research from the body and cells. The creators based there computational systems on the ways that cells divide, and subdivide into parts that produce a wrinkle-like blob. Through this, the artists at Nervous System takes the generations from the rules following theses biological systems, they 3D print these very elegant objects at all types of scales. The inwards and outwards folds though may seem random, are a result of a fairly simple set of rules. Additionally, I found it quite interesting to see how such a overlooked concept of the human body, is scaled both up and down into a piece of art that can be held and experienced in different ways. It many ways, it gives a new perspective on the connotations or understandings we already have of existing forms in nature and in our everyday lives.
Studio Website: https://n-e-r-v-o-u-s.com/
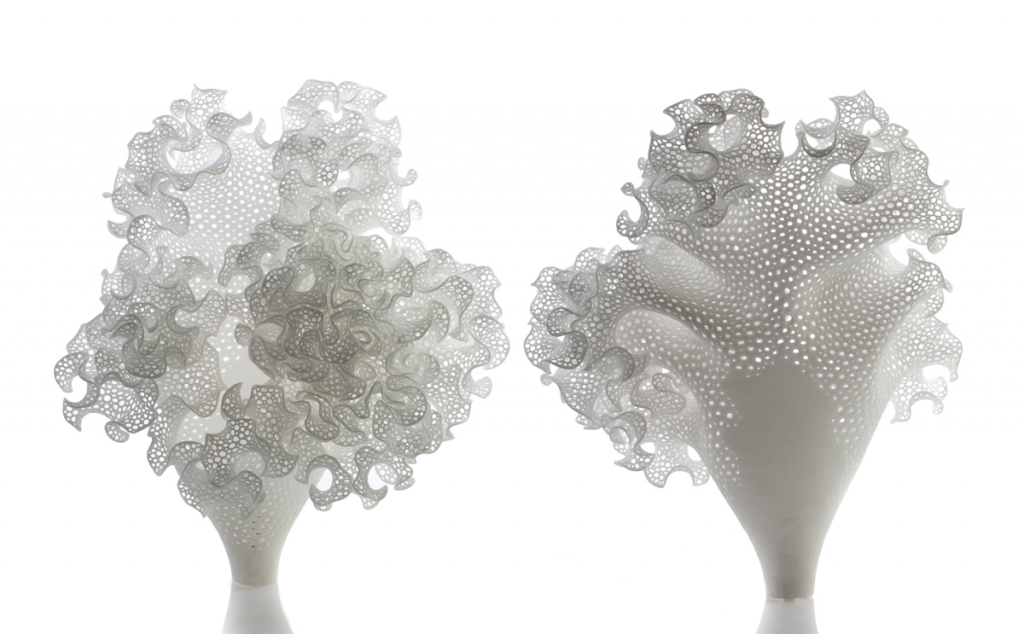
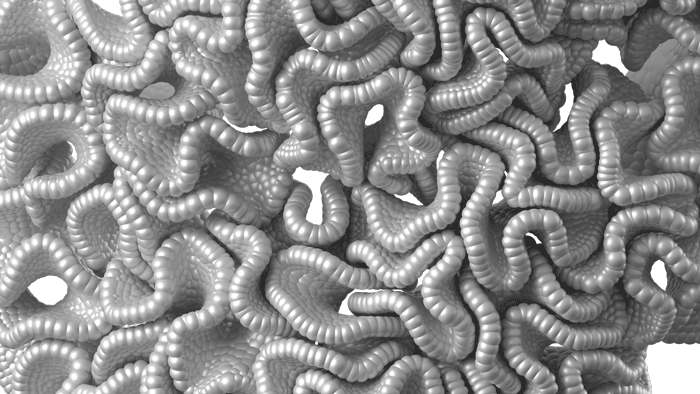
Looking Outwards 04
While looking into sound and computational artwork, I stumbled upon the sonic exhibition ‘Rupture’ by Camille Norman. The work was a performance pieces in which a series of panel glass frames lay broken or shattered in a room with microphones hanging above that emit a vibration. I think this work was makes an interesting comment on the way sound waves and vibrations produce types of factures within the air. In some sense, these can seem random, but in others these waves can be manipulated to produce a certain outcome of experience. The idea of path in this sense is taken to understanding from the microphone to the experiencer’s ears. I think this in a sense relates to the paths of the computational design. Weaving and interweaving within each other to finally reach the eye at one given instant. Amongst the chaos and the parameters of these waves and progressions, there results a single moment in time of experience.
https://www.norment.net/work/objects-installations-ind/rapture/
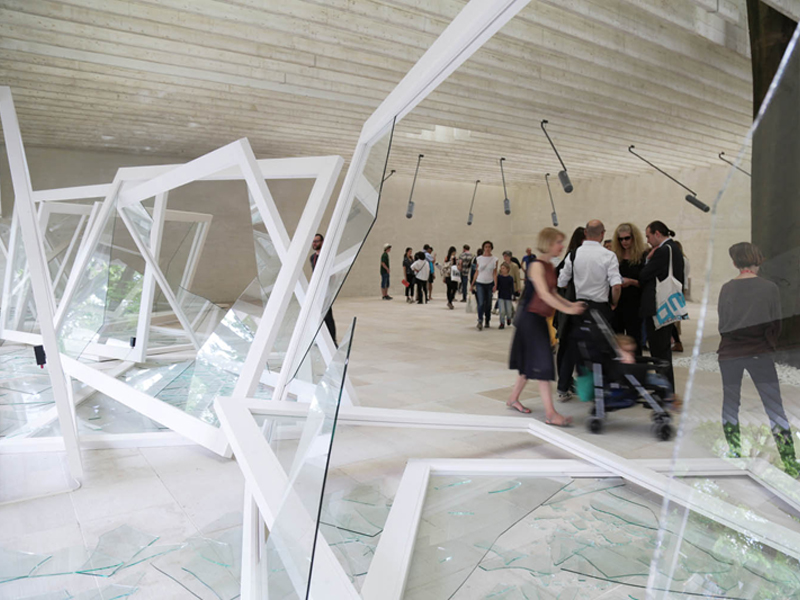
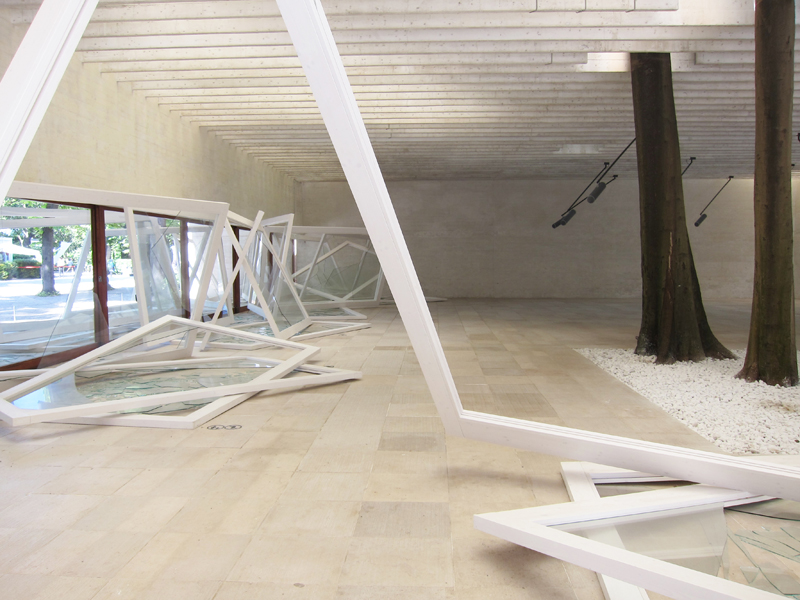