//Name: Hari Vardhan Sampath
//Section: E
//eMail address: harivars@andrew.cmu.edu
//Project-11
var snowForeground = []; // array of snow particles in the foreground
var snowBackground = []; // array of snow particles in the background
var snowAmountForeground = 500;
var snowAmountBackground = 750;
var snowSize = 3;
var snowLayers = 3;
function setup() {
createCanvas(500, 300);
for (i = 0; i < snowAmountForeground; i++) {
snowForeground[i] = new Object();
snowForeground[i].x = random(width);
snowForeground[i].y = random(height);
snowForeground[i].dy = random(0.25, 1);
snowForeground[i].c = color(random(200, 255));
}
for (i = 0; i < snowAmountBackground; i++) {
snowBackground[i] = new Object();
snowBackground[i].x = random(width);
snowBackground[i].y = random(height);
snowBackground[i].dy = random(0.25, 1);
snowBackground[i].c = color(random(200, 255));
}
}
// update position and draw each of 100 rectangles
function draw() {
// background sky gradient
for (var i = 0; i < height; i++) {
//
var c1 = color(0, 20, 52);
var c2 = color(0, 71, 126);
//
var gradient = map(i, 0, height, 0, 1);
var c = lerpColor(c1, c2, gradient);
stroke(c);
line(0, i, width, i);
}
// snow falling in the background
noStroke();
for (i = 0; i < snowAmountBackground; i++) {
drawSnowBackground(snowBackground[i]);
updateSnowBackground(snowBackground[i]);
}
// layers of winter forest
forest();
// snow falling in the foreground
noStroke();
for (i = 0; i < snowAmountForeground; i++) {
drawSnowForeground(snowForeground[i]);
updateSnowForeground(snowForeground[i]);
}
}
function forest() {
foliage0 = 0.06;
foliage1 = 0.04;
foliage2 = 0.02;
foliage3 = 0.01;
foliageSpeed0 = 0.001;
foliageSpeed1 = 0.002;
foliageSpeed2 = 0.003;
foliageSpeed3 = 0.004;
stroke(163, 196, 217);
beginShape();
for (var i = 0; i < width; i++) {
var t0 = (i * foliage0) + (millis() * foliageSpeed0);
var y0 = map(noise(t0), 0, 1, 200, 5);
line(i, y0, i, height);
}
endShape();
stroke(117, 163, 191);
beginShape();
for (var i = 0; i < width; i++) {
var t1 = (i * foliage1) + (millis() * foliageSpeed1);
var y1 = map(noise(t1), 0, 1, 250, 6);
line(i, y1, i, height);
}
endShape();
stroke(70, 113, 140);
beginShape();
for (var i = 0; i < width; i++) {
var t2 = (i * foliage2) + (millis() * foliageSpeed2);
var y2 = map(noise(t2), 0, 1, 275, 7);
line(i, y2, i, height);
}
endShape();
stroke(30, 51, 64);
beginShape();
for (var i = 0; i < width; i++) {
var t3 = (i * foliage3) + (millis() * foliageSpeed2);
var y3 = map(noise(t3), 0, 1, 300, 8);
line(i, y3, i, height);
}
endShape();
}
function drawSnowForeground(s) {
fill(s.c);
circle(s.x, s.y, 2);
}
function updateSnowForeground(s) {
s.y += s.dy;
if (s.y > height) {
s.y = 0;
}
else if (s.y < 0) {
s.y = height;
}
}
function drawSnowBackground(s) {
fill(s.c);
circle(s.x, s.y, random(0.5,1.5));
}
function updateSnowBackground(s) {
s.y += s.dy;
if (s.y > height) {
s.y = 0;
}
else if (s.y < 0) {
s.y = height;
}
}
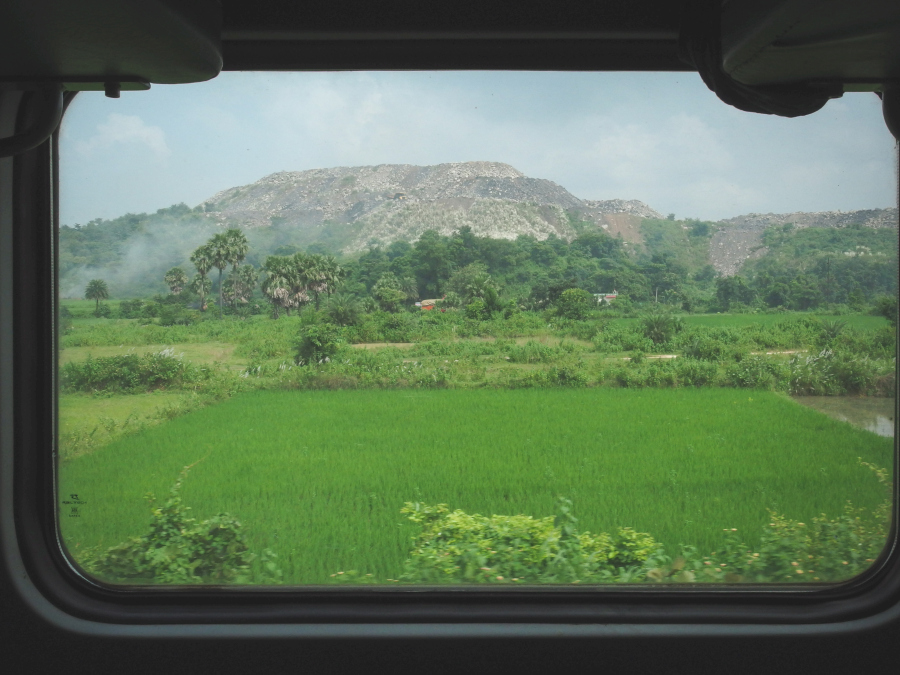
Credits: https://www.journeyb.com/2019/10/journeybasket-short-history-indian-railways-book-photos.html