Problem
Plotted plants are great choice for indoor decoration offering a sense of liveliness. However, the humidity in people’s rooms may be too low or too high. For example, some tropical plants typically require relatively higher humidity. In addition, it may get very dry during winter, making it difficult for the plotted plants to thrive.
Solution
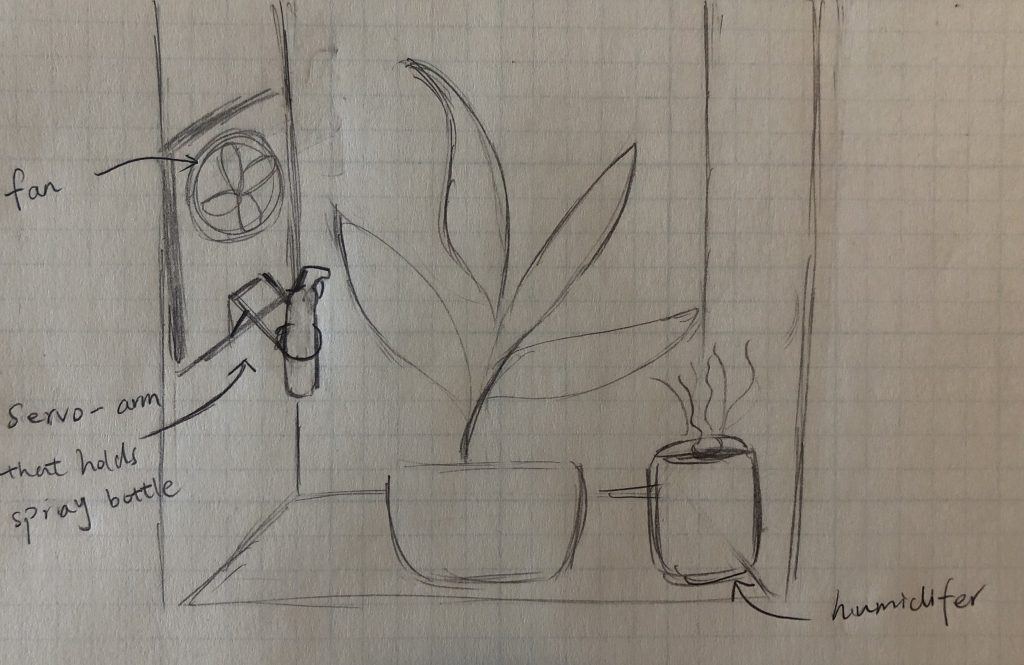
The solution I came up with is a system that monitors the humidity at the proximity of the plant. The system is composed of two separate device. The device on the left is hung on the wall with a fan and a servo arm that holds a spray bottle. The device on the right is a humidifier with a humidity sensor on it.
To demonstrate how the system work, suppose we have a delicate plant that requires misting if the average humidity is below 60% for the past 4 hours. The humidity cannot be over 90% because the plant can be suffocated or harmed by pests. Therefore, every 4 hours the system will check the average humidity and if it is below 60%, the servo-arm will swing up and down to remind the person in the room to mist the plant. If the humidity level does not change in 5 minutes, the servo-arm will stop and the humidifier will be turned on to increase the humidity and sustain it for a specific period. If the humidity level is over 90%, the fan will work until the humidity is below 90%.
When higher humidity is required, the servo-arm comes in first because manual misting is preferred, which gives the person more awareness of the condition of the plant. A night mode can be selected and the humidity is regulated autonomously by the system. Data and commands are sent between the two devices through IR transmitter and receiver.
Proof of Concept
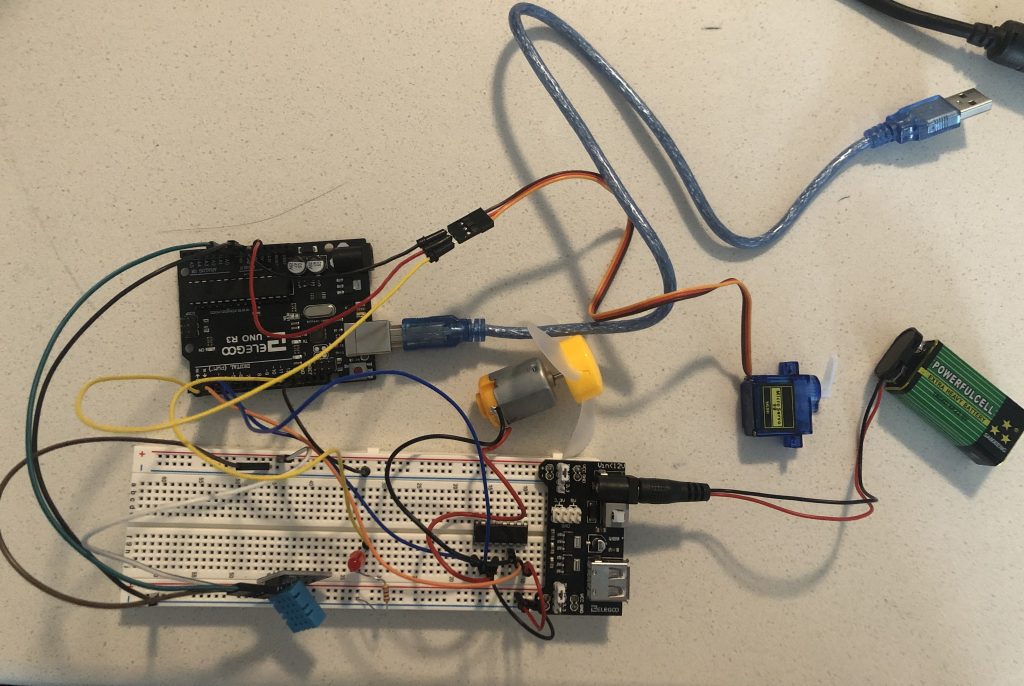
Code:
#include <dht.h> #include <Servo.h> // Analog Pin sensor is connected to #define dht_apin A0 #define SERVO_PIN 2 #define LED_PIN 13 #define ENABLE 5 #define DIRA 3 #define DIRB 4 dht DHT; Servo servo; //The vairables that define time-flow const int hour = 8000; //In this time-scale 4 sec represent 1 hour const int ideal_humid = 60; //The array that store the humidities int humidity_Data[48]; int record_digit; //The digit for inputing data into the array int check_digit; // The digit for checkingg every 2-hour int check_points = 8; // How many datapoints to check for the checking period String plant_status; //Clocks unsigned long clock_record = 0; // The clock for recording humidity const int INTERVAL1 = 2000; // milliseconds between each recroding //Funtions //Universal clock bool Universal_clock(String type) { if (type == "record") { if (millis() >= clock_record) { clock_record = millis() + INTERVAL1; return true; } return false; } } //Check if the plant need to be mist void need_mist() { int sum = 0; int average_humidity; //Serial.println(sum); for (int i = 0; i < check_points; i ++ ) { int d = check_digit + i; int humid = humidity_Data[d]; sum = sum + humid; Serial.println(i); Serial.println(humidity_Data[d]); Serial.println(sum); //Serial.println("hey"); } check_digit += check_points; //Serial.println("humidity checked"); average_humidity = sum / check_points; if (average_humidity < ideal_humid) { servo.write(0); delay(200); servo.write(180); plant_status = "dry"; //Serial.println(average_humidity); Serial.println("dry!"); } } void setup() { pinMode(LED_PIN, OUTPUT); pinMode(ENABLE, OUTPUT); pinMode(DIRA, OUTPUT); pinMode(DIRB, OUTPUT); servo.attach(SERVO_PIN); Serial.begin(9600); Serial.println("DHT11 Humidity & temperature Sensor\n\n"); delay(200);//Wait before accessing Sensor } void loop() { //Record the humidity if (Universal_clock("record") == true) { DHT.read11(dht_apin); humidity_Data[record_digit] = DHT.humidity ; Serial.println(humidity_Data[record_digit]); if (DHT.humidity >= 90) { plant_status = "wet"; digitalWrite(ENABLE, HIGH); digitalWrite(DIRA, HIGH); //one way digitalWrite(DIRB, LOW); } if (plant_status == "wet") { //check if it is still wet if (DHT.humidity < 90) { plant_status = "fine"; digitalWrite(ENABLE, LOW); check_digit = record_digit; } } if (plant_status == "dry") { //check if it is still dry if (DHT.humidity >= ideal_humid) { plant_status = "fine"; digitalWrite(LED_PIN, LOW); check_digit = record_digit; } else { digitalWrite(LED_PIN, HIGH); } } if (record_digit - check_digit == 7) { need_mist(); } record_digit += 1; } //Check if the plant is too dry in the past 2 hours //Serial.print("Current humidity = "); //Serial.print(DHT.humidity); //Serial.print("% "); //Serial.print("temperature = "); //Serial.print(DHT.temperature); //Serial.println("C "); //delay(5000);//Wait 5 seconds before accessing sensor again. //Fastest should be once every two seconds. }