Problem:
Do you like having natural light in the room during the day, but often forget to close the curtains at night and so get woken up earlier than you wanted in the morning? Do you ever just want to wake up with natural light in the room, but not get woken up too early by it? After not getting enough sleep, do you find yourself having trouble getting up to your alarm or being blinded when you turn on the light or open the curtains because your eyes aren’t used to it? If you’re like me and have answered yes to these questions, this worry free curtain and alarm clock supplement could be the solution!
Proposed Solution:
Enter the time times you want to wake up each day of the week, the current date and time, upload the program, and that’s it your curtains will open according to the time you want to wake up and when you went to bed (set by pressing button) and close when it’s evening and dark. If you didn’t get nearly enough sleep, a fan will also blow in your face when your alarm goes off to help ensure you wake up. The fan could be an overhead one, but one on a nightstand or a taller one on the floor next to the bed would be best. I used the left and right threaded rods coupled together that I had from a previous project to be able to control the open and closing of the curtains movement. The logic I used for when the curtains should open compared to when you want to wake up and when you went to sleep were set by my preferences wherein the less sleep I got, the earlier the curtains would open based on time frames of amount of sleep. For me if I’ve gotten less sleep, I wake up to slight changes less than if I got enough sleep. Also, the fan is set to blow at a low speed for a minute when you’ve had under 5 hours sleep and high speed for 30 seconds if you’ve had between 5 and 6 hours. This is because higher speed will be more annoying and so needs to be on less; the lower speed is for the least sleep case because you are more likely to get sick on less sleep. I used a stepper motor to control the opening and closing of the curtains as that is what I had available and I can easily control the distance moved forward and reverse precisely. I would’ve used a faster motor if available and ideally a DC motor and an encoder instead as I’d be able to control the amounts forward and reverse easily and it’s more efficient in this scenario. Additionally, if I had a pressure sensor, the time you went to bed would be detected by the time you went on the bed and didn’t get up again before the alarm. This way you wouldn’t have to remember to click a button. To add to this, I would add a speaker so that this could be used for an alarm sound itself too. Finally, as someone mentioned during the crit, an app would be really useful for inputting the parameters and quickly making any changes. With the materials I was able to use and the time given, I’m happy with this prototype and in the future may make this for myself on an actual scale.
For reference, this is what the curtains in my room look like:
Ideal fans:
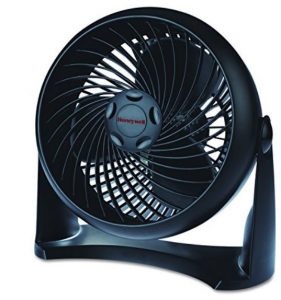
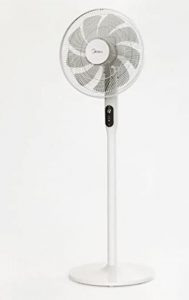
Proof of Concept:
Closing the curtains after 6:00PM and dark
Setting the time went to bed, opening curtains, and blowing the fan.
You might notice that in this video, the printing format changed from day, month, year to month, day, year. This was an intentional change after the first video because it’s the format we are accustomed to.
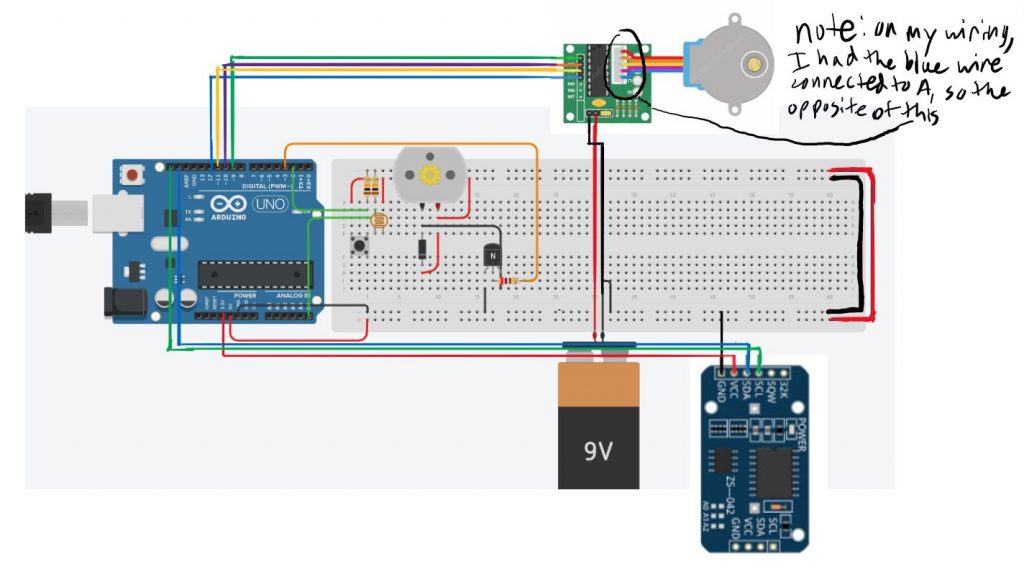
#include <AccelStepper.h> // stepper Motor pin definitions: #define motorPin1 9 // IN1 on the ULN2003 driver #define motorPin2 10 // IN2 on the ULN2003 driver #define motorPin3 11 // IN3 on the ULN2003 driver #define motorPin4 12 // IN4 on the ULN2003 driver // Define the AccelStepper interface type; 4 wire motor in half step mode: #define MotorInterfaceType 8 // Initialize with pin sequence IN1-IN3-IN2-IN4 for using the AccelStepper library with 28BYJ-48 stepper motor: AccelStepper stepper = AccelStepper(MotorInterfaceType, motorPin1, motorPin3, motorPin2, motorPin4); //above from https://www.makerguides.com to learn how to use this specific stepper motor and driver #include <Wire.h> #include <RTC.h> const int photoPin = A5; static DS3231 RTC; //input times for each day with hr then min; 24 hr time but as it'll be morning, it shouldn't make too much of a difference int wakeUpTimes[7][2] ={{12, 12}, //sun {9, 0}, //mon {9, 35}, //tues {8, 55}, //wed {9, 30}, //thurs {10, 10}, //fri {11, 11}};//sat const int fanMotorPin = 3; int low = 50; int high = 150; int fanSpeed; int turnOnFan; int fanDuration; int timeSlept[3]; int openCurtainsXbeforeWakeUpTime; int sleep = 0; const int buttonPin = 2; int timeWentToBed[3]; void calcTimeSlept() { //seconds int secs = 60 - timeWentToBed[0]; if (secs == 60) { timeSlept[2] = 0; } else { timeSlept[2] = secs; } //mins if (wakeUpTimes[RTC.getWeek() - 1][1] >= timeWentToBed[1]) { if (timeSlept[2] == 0) { timeSlept[1] = wakeUpTimes[RTC.getWeek() - 1][1] - timeWentToBed[1]; } else { timeSlept[1] = wakeUpTimes[RTC.getWeek() - 1][1] - timeWentToBed[1] - 1; } } else { if (timeSlept[2] == 0) { timeSlept[1] = wakeUpTimes[RTC.getWeek() - 1][1] + 60 - timeWentToBed[1]; } else { timeSlept[1] = wakeUpTimes[RTC.getWeek() - 1][1] + 60 - timeWentToBed[1] - 1; } } //hrs if (timeWentToBed[0] < 12) { if (wakeUpTimes[RTC.getWeek() - 1][1] >= timeWentToBed[1]) { timeSlept[0] = wakeUpTimes[RTC.getWeek() - 1][0] - timeWentToBed[0]; } else { timeSlept[0] = wakeUpTimes[RTC.getWeek() - 1][0] - timeWentToBed[0] - 1; } } else { //if went to bed before midnight, need to add hours bc subtraction not right otherwise int hrsBeforeMidnight = 24 - timeWentToBed[0]; if (wakeUpTimes[RTC.getWeek() - 1][1] >= timeWentToBed[1]) { timeSlept[0] = wakeUpTimes[RTC.getWeek() - 1][0] + hrsBeforeMidnight; } else { timeSlept[0] = wakeUpTimes[RTC.getWeek() - 1][0] + hrsBeforeMidnight - 1; } } } void goinToSleep() { if (digitalRead(buttonPin) == HIGH) { sleep = 1; } } void openCurtains() { while (stepper.currentPosition() != 4096 * 7) { //choosing this bc it was enough rev to at least show some opening stepper.setSpeed(1000); stepper.runSpeed(); } } void closeCurtains() { while (stepper.currentPosition() != 0) { stepper.setSpeed(-1000); stepper.runSpeed(); } } void setup() { // put your setup code here, to run once: stepper.setMaxSpeed(1000); Serial.begin(9600); RTC.begin(); RTC.setDay(16); RTC.setMonth(10); RTC.setYear(2020); RTC.setWeek(6); //to show setting time went to bed from button for Fri RTC.setHours(10); RTC.setMinutes(8); RTC.setSeconds(0); //to show fan turning on when not enough sleep for Mon // RTC.setHours(8); // RTC.setMinutes(59); // RTC.setSeconds(30); ////to show closing: // stepper.setCurrentPosition(4096 * 7); //to show curtains closing past 5 and dark // RTC.setHours(17); // RTC.setMinutes(59); // RTC.setSeconds(45); //to show opening: stepper.setCurrentPosition(0); //to show curtain opening: // RTC.setHours(8); // RTC.setMinutes(49); // RTC.setSeconds(58); RTC.setHourMode(CLOCK_H24); pinMode(fanMotorPin, OUTPUT); attachInterrupt (digitalPinToInterrupt (buttonPin), goinToSleep, HIGH); /*set time went to bed to hr before wakeup as default after woken up will change if/when press button before going to sleep this way if you forget still get this, and assuming if didn't press, you went to bed really late */ //commenting out for demo of button to set time went to bed timeWentToBed[0] = wakeUpTimes[RTC.getWeek() - 1][0] - 1; timeWentToBed[1] = wakeUpTimes[RTC.getWeek() - 1][1]; timeWentToBed[2] = 0; } void loop() { int photoVal = analogRead(photoPin); //Serial.println(photoVal); if (RTC.getHours() >= 18) {//6:00PM if (photoVal < 600) { //if it's past 6PM and dark(here just chose 600, but would be lower in real thing), close curtains closeCurtains(); } } if (sleep == 1) { Serial.println("clicked"); timeWentToBed[0] = RTC.getHours(); timeWentToBed[1] = RTC.getMinutes(); timeWentToBed[2] = RTC.getSeconds(); calcTimeSlept(); sleep = 0; } if (timeSlept[0] >= 8) { openCurtainsXbeforeWakeUpTime = 5; //mins } else if (6 <= timeSlept[0] && timeSlept[0] < 8) { openCurtainsXbeforeWakeUpTime = 30; //mins } else if (5 <= timeSlept[0] && timeSlept[0] < 6) { openCurtainsXbeforeWakeUpTime = 45; //mins turnOnFan = 1; fanSpeed = high; fanDuration = 30; } else {//under 5 hrs //for demo //openCurtainsXbeforeWakeUpTime = 1; //mins openCurtainsXbeforeWakeUpTime = 55; //mins turnOnFan = 1; fanSpeed = low; fanDuration = 60; } if (wakeUpTimes[RTC.getWeek() - 1][1] >= openCurtainsXbeforeWakeUpTime) { if (RTC.getHours() == wakeUpTimes[RTC.getWeek() - 1][0]) { //Serial.println(openCurtainsXbeforeWakeUpTime); //Serial.println((wakeUpTimes[RTC.getWeek() - 1][1] - openCurtainsXbeforeWakeUpTime)); if (RTC.getMinutes() == (wakeUpTimes[RTC.getWeek() - 1][1] - openCurtainsXbeforeWakeUpTime)) { openCurtains(); timeWentToBed[0] = wakeUpTimes[RTC.getWeek() - 1][0] - 1; timeWentToBed[1] = wakeUpTimes[RTC.getWeek() - 1][1]; timeWentToBed[2] = 0; } } } else {//minutes of wakeUpTime<time to subtract to wake up if (RTC.getHours() == (wakeUpTimes[RTC.getWeek() - 1][0] - 1)) { //took hr off here //right side of eq subtracted amount of time left after changing hr if (RTC.getMinutes() == (60 - openCurtainsXbeforeWakeUpTime + wakeUpTimes[RTC.getWeek() - 1][1])) { openCurtains(); timeWentToBed[0] = wakeUpTimes[RTC.getWeek() - 1][0] - 1; timeWentToBed[1] = wakeUpTimes[RTC.getWeek() - 1][1]; timeWentToBed[2] = 0; } } } if (RTC.getHours() == wakeUpTimes[RTC.getWeek() - 1][0]) { if (RTC.getMinutes() == wakeUpTimes[RTC.getWeek() - 1][1]) { //Serial.println(RTC.getSeconds()); if (turnOnFan) { if (RTC.getSeconds() <= fanDuration) { //Serial.println(RTC.getSeconds()); analogWrite(fanMotorPin, fanSpeed); } else { analogWrite(fanMotorPin, 0); turnOnFan = 0; } } } } static int startPrint=millis(); if ((millis()-startPrint)>=1000){//putting this here so don't use delay while also showing the times switch (RTC.getWeek()) { case 1: Serial.print("SUN"); break; case 2: Serial.print("MON"); break; case 3: Serial.print("TUE"); break; case 4: Serial.print("WED"); break; case 5: Serial.print("THU"); break; case 6: Serial.print("FRI"); break; case 7: Serial.print("SAT"); break; } Serial.print(" "); Serial.print(RTC.getMonth()); Serial.print("-"); Serial.print(RTC.getDay()); Serial.print("-"); Serial.print(RTC.getYear()); Serial.print(" "); Serial.print(RTC.getHours()); Serial.print(":"); Serial.print(RTC.getMinutes()); Serial.print(":"); Serial.println(RTC.getSeconds()); startPrint=millis(); } // delay(1000); }