//Evette LaComb
//Section D
var sect = 100; // section size
function setup() {
createCanvas(600, 600);
}
function draw() {
background(225, 200, 165);
for (var y = -50; y < height +60; y += 100){
for (var x = 0; x < width +60; x += 100){
flower(x, y);
}
}
noLoop();
}
function flower(x, y){
push();
translate(x, y);
// boarder details
fill(110, 40, 10); //brown
noStroke();
rect(0, 0, 7, sect);
rect(sect -7, 0, 7, sect);
noStroke();
arc(100, 25, 25, 30, radians(90), radians(270), OPEN);
strokeWeight(2);
stroke(190, 80, 10); // orange
line (15, 0, 15, sect);
strokeWeight(3);
line (10, 0, 10, sect);
stroke(110, 40, 10); //brown
line(50, 90, 50, 150);
//vines
noStroke();
noFill();
strokeWeight(5);
stroke(30, 70, 35); // dark green
arc(50, 0, 50, 50, radians(90), radians(180), OPEN);
arc(50, 50, 50, 50, radians(270), radians(90), OPEN);
arc(50, 100, 50, 50, radians(180), radians(360), OPEN);
strokeWeight(4);
arc(50, 100, 50, 25, radians(180), radians(25), OPEN);
strokeWeight(2);
arc(50, 100, 47, 37, radians(180), radians(360), OPEN);
//center dimond detail
push();
fill(30, 70, 35); // dark green
translate(50, 50);
rotate(radians(45));
rectMode(CENTER);
rect(0, 0, 27, 27);
pop();
fill(190, 80, 10); // orange
ellipse(50, 50, 27, 27);
fill(225, 200, 165);
ellipse(50, 50, 19, 19);
pop();
}
Month: October 2022
Project 05
Wallpaper Art – Floral pattern
// Theresa Ye
// Section E
// thye@andrew.cmu.edu
// Project-05
function setup() {
createCanvas(400, 600);
background(215,200,204);
}
function draw() {
for (var x = 0; x < 450; x += 25) {
for (var y = 0; y < 650; y += 30) {
drawLeavesSmall(x,y);
}
}
for (x = 25; x < 390; x += 50) {
for (y = 25; y < 600; y += 60) {
stroke(215,200,204)
fill(176,142,149);
ellipse(x,y,45)
}
}
for (x = 25; x < 390; x += 50) {
for (y = 25; y < 600; y += 60) {
drawLeaves(x,y);
drawFlower(x,y);
}
}
}
function drawFlower (x,y) {
push();
translate(x,y);
scale(0.5)
fill(188,164,170);
stroke(215,200,204);
strokeWeight(0.5);
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-10,-16);
curveVertex(-15,-20);
curveVertex(0,-30);
curveVertex(25,-24);
curveVertex(30,-20);
curveVertex(28,-10);
curveVertex(0,0);
curveVertex(0,0);
endShape();
fill(161,127,136);
ellipse(4,-10,18,15);
fill(188,164,170);
rotate(radians(120));
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-10,-16);
curveVertex(-15,-20);
curveVertex(0,-30);
curveVertex(25,-24);
curveVertex(30,-20);
curveVertex(28,-10);
curveVertex(0,0);
curveVertex(0,0);
endShape();
fill(161,127,136);
ellipse(4,-10,18,15);
fill(188,164,170);
rotate(radians(120));
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-10,-16);
curveVertex(-15,-20);
curveVertex(0,-30);
curveVertex(25,-24);
curveVertex(30,-20);
curveVertex(28,-10);
curveVertex(0,0);
curveVertex(0,0);
endShape();
fill(161,127,136);
ellipse(4,-10,18,15);
fill(255);
stroke(255);
ellipse(0,0,10);
pop();
}
function drawLeaves(x,y) {
push();
translate(x,y);
scale(0.5);
stroke(215,200,204)
fill(176,142,149);
ellipse(0,0,45)
fill(141,99,110);
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-2,-35);
curveVertex(-16,-50);
curveVertex(-30, -55);
curveVertex(-50,-60);
curveVertex(-50,-60);
curveVertex(-40,-28);
curveVertex(-20,-8);
curveVertex(0,0);
curveVertex(0,0);
endShape();
rotate(radians(90));
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-2,-35);
curveVertex(-16,-50);
curveVertex(-30, -55);
curveVertex(-50,-60);
curveVertex(-50,-60);
curveVertex(-40,-28);
curveVertex(-20,-8);
curveVertex(0,0);
curveVertex(0,0);
endShape();
rotate(radians(145));
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-2,-35);
curveVertex(-16,-50);
curveVertex(-30, -55);
curveVertex(-50,-60);
curveVertex(-50,-60);
curveVertex(-40,-28);
curveVertex(-20,-8);
curveVertex(0,0);
curveVertex(0,0);
endShape();
pop();
}
function drawLeavesSmall(x,y) {
push();
translate(x,y);
scale(0.25);
stroke(215,200,204)
fill(239,233,235);
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-2,-35);
curveVertex(-16,-50);
curveVertex(-30, -55);
curveVertex(-50,-60);
curveVertex(-50,-60);
curveVertex(-40,-28);
curveVertex(-20,-8);
curveVertex(0,0);
curveVertex(0,0);
endShape();
rotate(radians(90));
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-2,-35);
curveVertex(-16,-50);
curveVertex(-30, -55);
curveVertex(-50,-60);
curveVertex(-50,-60);
curveVertex(-40,-28);
curveVertex(-20,-8);
curveVertex(0,0);
curveVertex(0,0);
endShape();
rotate(radians(145));
beginShape();
curveVertex(0,0);
curveVertex(0,0);
curveVertex(-2,-35);
curveVertex(-16,-50);
curveVertex(-30, -55);
curveVertex(-50,-60);
curveVertex(-50,-60);
curveVertex(-40,-28);
curveVertex(-20,-8);
curveVertex(0,0);
curveVertex(0,0);
endShape();
pop();
}
Project 5: Wallpaper
In this project I tried to create a set of repetitive diamond geometry and also a glowing effect that makes it look like it’s in 3D.
var r=50
function setup() {
createCanvas(800,600);
background(220);
}
function draw() {
drawingContext.shadowBlur=20;
drawingContext.shadowColor=color(20); //glowing effect and black shadow
noStroke();
//first set of geometries
for(var col=0;col<4;col++){
for(var row=0;row<20;row++){
push();
translate(col*r,(row-2)*r);
diamondColor(row);
diamondOne();
pop();
}
}
//second set of geometries
for(var col=4;col<8;col++){
for(var row=0;row<20;row++){
push();
translate(col*r,(row-2)*r);
diamondColor(row);
diamondTwo();
pop();
}
}
//third set of geometries
for(var col=8;col<12;col++){
for(var row=0;row<20;row++){
push();
translate(col*r,(row-2)*r);
diamondColor(row);
diamondOne();
pop();
}
}
//fourth set of geometries
for(var col=12;col<16;col++){
for(var row=0;row<20;row++){
push();
translate(col*r,(row-2)*r);
diamondColor(row);
diamondTwo();
pop();
}
}
}
//first type of diamond
function diamondOne(){
quad(0,0,r,r,r,2*r,0,r);
}
//second set of diamond
function diamondTwo(){
quad(0,r,r,0,r,r,0,2*r);
}
//coloring the diamonds
function diamondColor(row){
if(row%8==0){
fill(22,76,122);
}else if(row%8==1){
fill(254,252,204);
}else if(row%8==2){
fill(255,190,14);
}else if(row%8==3){
fill(226,92,3);
}else if(row%8==4){
fill(119,147,172);
}else if(row%8==5){
fill(1,24,66);
}else if(row%8==6){
fill(224,28,1);
}else if(row%8==7){
fill(255,141,6);
}
}
LO5: 3D Computer Graphics
In this week’s looking outward practice, I’m very interested in a 3D graphic art, Forest Diorama, designed by Roman Klčo. This piece of art portraits a forest scene with possibly 3D rendering methods. In general, the art creates a axonometric view of a certain part of land, putting trees, rocks and mountain slopes onto it, also simplifying it in more cartoonish shapes. These kind of art could be done with renderers like Blender, which is very interesting in making small scenes that tell stories.
https://dribbble.com/shots/5455348-Forest-Diorama
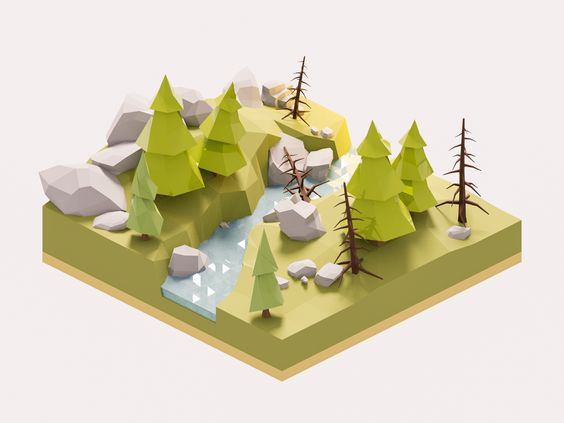
Looking Outwards -05
3d Computer Graphics
An artist in this field that I admire a lot is Omar Aqil. I first got to know him from his series of 3D recreations of famous Picasso paintings. Omar uses computer-generated imagery to portray three-dimensional aspects of two-dimensional drawings and represent them as renderings or animation. His works are very representative of the original paintings but with an almost cute twist because of the roundness of the three dimensional shapes he uses. I like these projects so much because they just add an extra depth to these familiar paintings and make them fun and interesting. Other than his mimicry series, Omar also has other series of portraits, products, and other sculptures that are dynamic in colour and depth. His renderings often incorporate organic aesthetic elements that contrast the “tech” feel of the piece, making the image look unreal and tangible at the same time.
Omar Aqil
Project -05: Wallpaper
I wanted my wallpaper to look 3D and look like if the light was shining from the top left side!
function setup() {
createCanvas(400, 390);
background(0);
}
function draw() {
rotateGrid(-50,0); //rotate's final grid
}
function drawTriangleOne(x,y) { //draws Triangle 1
strokeWeight(0.1);
fill(150); //Triangle 1
triangle(x, y, x + 50, y, x + 25, y + (sqrt(1875))/2);
fill(80)
triangle(x + 50, y, x + 25, y + sqrt(1875), x + 25, y + (sqrt(1875))/2);
fill(230)
triangle(0, 0, x + 25, y + (sqrt(1875))/2, x + 25, y + sqrt(1875));
}
function drawTriangleTwo(x,y) { //draws Triangle 2
fill(200); //Triangle 2
triangle(x + 50, y, x + 25, y + (sqrt(1875)), x + 50, y + (sqrt(1875))/2);
fill(150);
triangle(x + 50, y, x + 50, y + (sqrt(1875))/2, x + 75, y + sqrt(1875));
fill(80);
triangle(x + 25, y + (sqrt(1875)), x + 50, y + (sqrt(1875))/2, x + 75, y + sqrt(1875));
}
function drawFlipTriangleOne(x,y) { //Draws Triangle 3
push();
translate(-25, sqrt(1875));
drawTriangleTwo(x,y);
pop();
}
function drawFlipTriangleTwo(x,y) { //Draws Triangle 4
push();
translate(25, sqrt(1875));
drawTriangleOne(x,y);
pop();
}
function drawSingleRowTriangles(x,y) { //Draws First Row of Triangles
for (var i = 0; i < 8; i++) {
drawTriangleOne(x,y);
drawTriangleTwo(x,y);
translate(50,0);
}
}
function drawFourStartingTriangles(x,y) { //Groups First Four Starting Triangles
for (var i = 0; i < 9; i++) {
drawTriangleOne(x,y);
drawTriangleTwo(x,y);
drawFlipTriangleOne(x,y);
drawFlipTriangleTwo(x,y);
}
}
function drawTwoRowTriangles(x,y) { //Draws First 2 Rows of Triangles
push();
for (var i = 0; i < 11; i++) {
drawFourStartingTriangles(x,y);
translate(50,0);
}
drawTriangleOne(x,y);
drawFlipTriangleOne(x,y);
pop();
}
function drawTriangleGrid(x,y) { //Uses for loops to create entire Triangle Grid
push();
for (var i = 0; i < 6; i++) {
drawTwoRowTriangles(x,y);
translate(0, 2*sqrt(1875));
}
drawSingleRowTriangles(x,y);
drawTriangleOne(x,y);
drawTriangleTwo(x,y);
pop();
}
function rotateGrid(x,y) {
angleMode(DEGREES);
rotate(30);
translate(0,-200);
drawTriangleGrid(x,y);
}
Looking Outwards – 05: 3D Computer Graphics
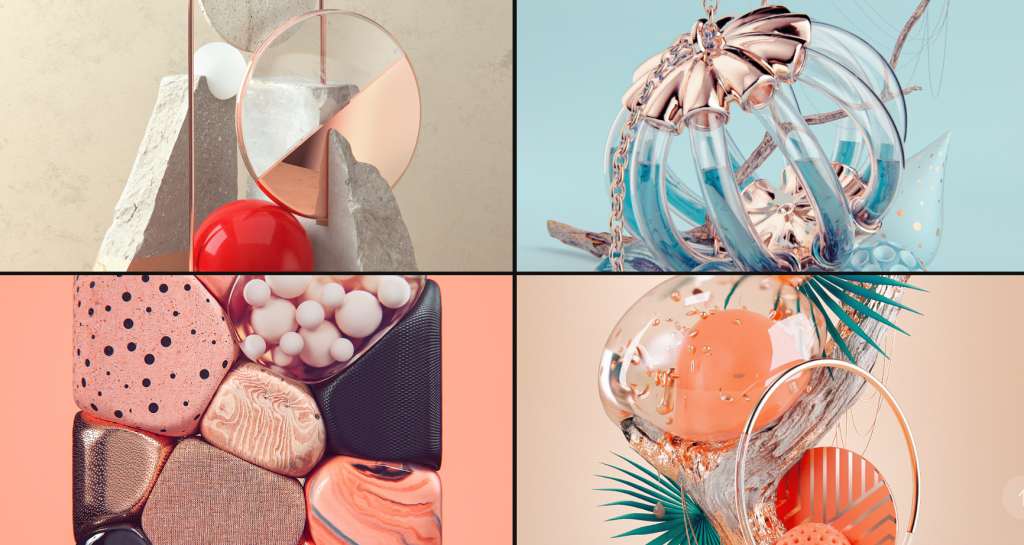
Roman Bratschi is the owner and creative director of his 3D designs made in Switzerland. He is also an experienced animation director, and has experienced his career as a graphic designer. His work is inspired by natural shapes, forms, and patterns. His techniques are expert in exposing their natural simplicity in the final piece. One thing I enjoy about his work is how all of his work reminds me of MacBook Wallpaper. Also, I love how organic some of his work is. Some of them resemble fruits and other vegetables, but they all have the same theme.
Looking Outwards 05: 3D Computer Graphics
Work Title: “WAVE” with Anamorphic illusion
Artist: D’strict, South Korea
The LED display located in COEX K-pop square simulates the motion of a wave through virtual reality. The design took about three months to develop; the display screen size is superb and can fit four basketball courts. This interaction of waves splashing down is made using an anamorphic illusion through the curved screen. When we begin to design 3-dimensionally, we also need to consider all x, y, and z planes and how the shapes/forms of design construct through those planes. In this work, the plane is transformed into a curved surface, allowing visual illusion as well as depth. Because of its color and lively motion, the plaza is filled with energy and enthusiasm for people to learn more about the artwork. Lee Sung-ho, CEO of d’strict explained: “We plan to get motives from nature moving forward so people can also experience a healing within the city.”
LookingOutwards-05
In her artwork, Nimbes, Joanie Lemeecier and James Ginzburg attempt to capture the paradox of observation: how as we observe and understand more rules and truths in our universe we understand that we know less and even that through a different lens these truths no longer apply. These digital artists use 3D rendering to compute a series of images that consist of small individual pieces that occasionally relate to each other to create a recognizable image of a church or tree canopy for example before exploding. This disintegration of the recognizable, the observable, represents the limits of our understanding and how the truths we may understand as the image of a church are in fact irrelevant. I find their work particularly interesting because it serves as a reminder of the chaos that exists outside our collective and individual perception of the universe.
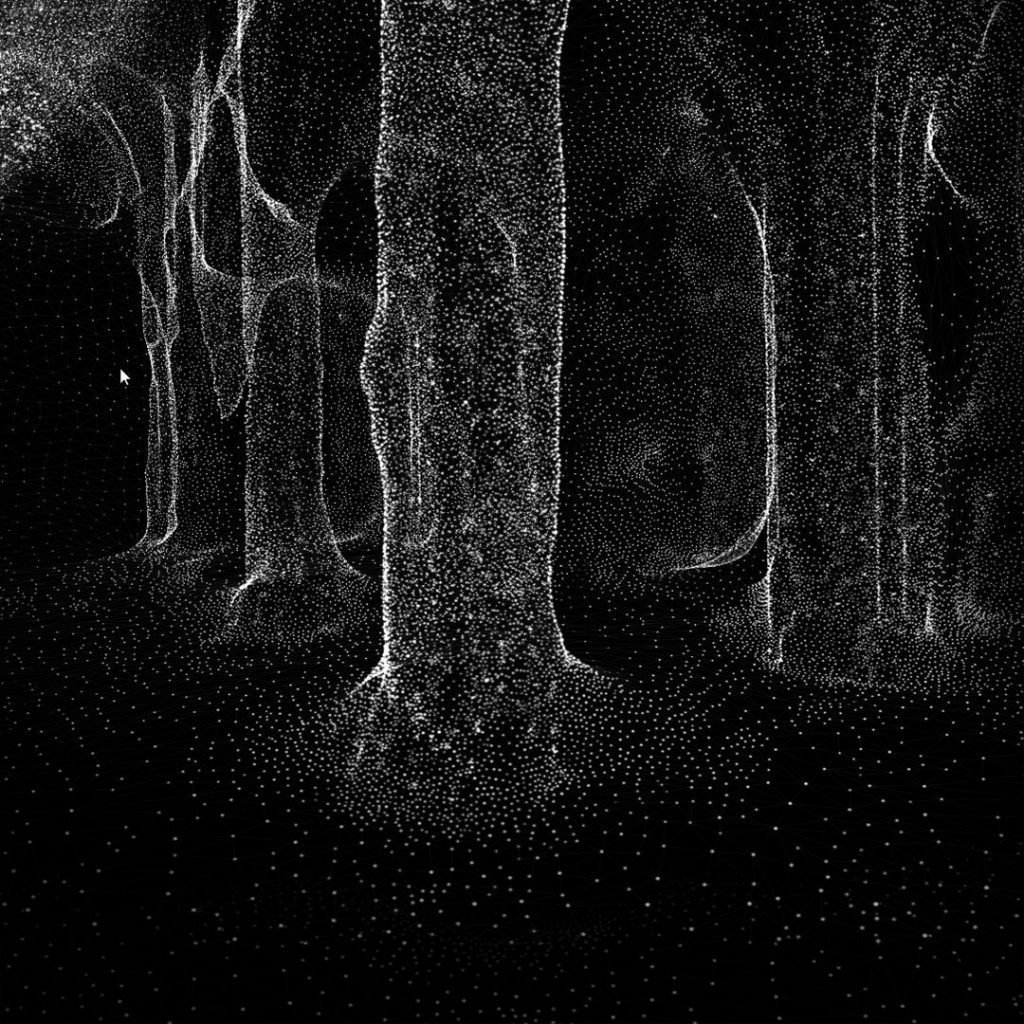
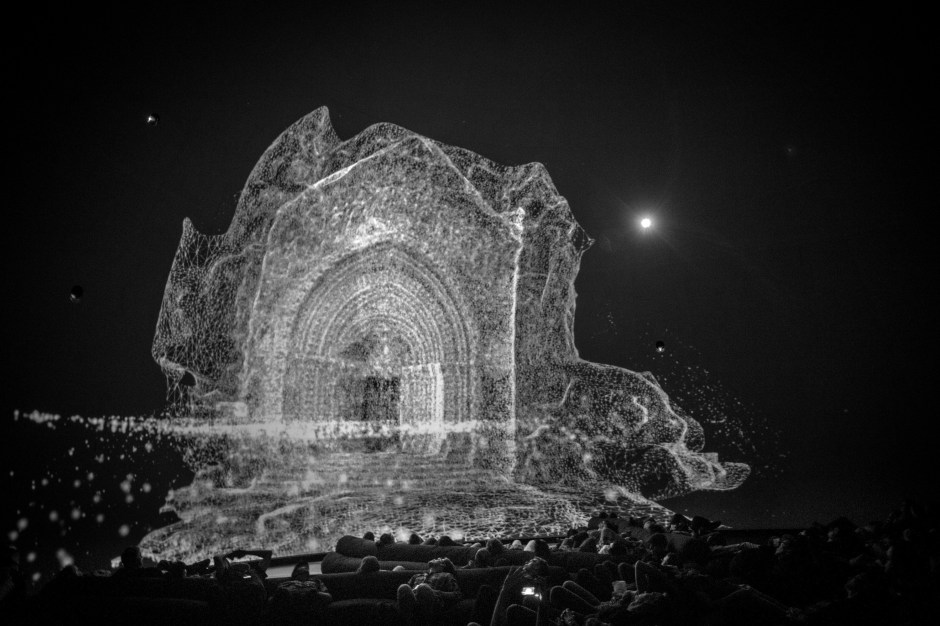