/*
* Andrew J Wang
* ajw2@andrew.cmu.edu
* Section A
*
* This program draws line arts
*/
var maxNum = 30;
function setup() {
createCanvas(400, 300);
background(0);
}
function draw() {
background(0);
//using the 3 functions for 3 different shapes
backgroundStrings (50);
stroke(255);
circleStrings(200,150,200,500);
stroke(255,0,0,255);
circleStrings(200,150,100,58);
push();
fill(255);
ellipse(200,150,100);
pop();
stroke(255,0,0,255);
hexagonStrings(200,150,100,5,10);
}
function circleStrings(Cx,Cy,Cd,Cl) {
for (var k = 0; k < maxNum; k++)
{
//grabing points on the circle
y=sin((k*(360/maxNum))*Math.PI/180)*(Cd/2)+Cy;
x=cos((k*(360/maxNum))*Math.PI/180)*(Cd/2)+Cx;
//making lines that is perpandicular to the radius with adjustable lengths
p1X = x + cos(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p1Y = y + sin(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p2X = x - cos(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p2Y = y - sin(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
line (p2X,p2Y,p1X,p1Y);
}
}
function hexagonStrings(Hx,Hy,Hd,num,seg) {
//make arrays
const array = [];
var angle = 360/num;
for (var k = 0; k < num; k++)
{
array[k] = new Array();
y=sin(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)+Hy;
x=cos(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)+Hx;
line (Hx,Hy,x,y);
for (var s = 0; s<seg; s++)
{
//3D array grabing every points on the line of the star
array[k][s] = new Array();
var y1=sin(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)*s/seg+Hy;
var x1=cos(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)*s/seg+Hx;
array[k][s][0] = x1;
array[k][s][1] = y1;
}
}
//cross connecting those lines using for loop
for (var k = 0; k < num-1; k++)
{
for (var s = 0; s<seg; s++)
{
line(array[k][s][0],array[k][s][1],array[k+1][seg-1-s][0],array[k+1][seg-1-s][1]);
}
}
for (var s = 0; s<seg; s++)
{
line(array[num-1][s][0],array[num-1][s][1],array[0][seg-1-s][0],array[0][seg-1-s][1]);
}
}
function backgroundStrings (num) {
for (var k=0; k<num; k++)
{
//cross connecting with for loop
stroke(255/num*k,0,0,255);
line (k*width/num,0,width,k*height/num);
line (width-k*width/num,height,0,height-k*height/num);
line (k*width/num,height,width,height-k*height/num);
line (width-k*width/num,0,0,k*height/num);
}
}
Tag: Section A
LO 4 – Sound Art
I really like the albums that the Fat Rat, a music producer, made because I really like his style and his unique use of those special sound effects. (I’ll put a link for one of the songs he made (Unity) here) Most digital music producers always use a DAW (digital audio workstation) to make their music, and I would briefly explain how those producers use a DAW to make their music. First, they put down chords on some specific track lists (that look like a timeline) using pre-recorded chords of different instruments (which the Fat Rat mostly uses pre-recorded game sound effects, usually electronic music using programmable sound generator sound chips) in order like building blocks and then, they arrange those musical sentences (chords) so it all fits together to form a rhythm. After arranging those notes, producers have to adjust the audio levels of each track list (usually different instruments) to make them sound right, and this process is called the mix stage. In between the stages, the producer can easily add effects or change chords (like laying on top of the base note), making the music original and flexible to changes.
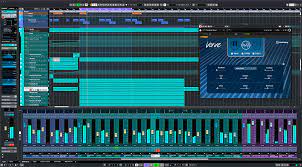
Looking Outwards-03, Section A
I thought the piece by David Bizer was particularly inspirational. He used the timeline of an audiofile and then 3D printed it to create a jewelry piece for someone saying, “I love you.” I thought this piece was really cool because of how Bizer captures something that is typically thought to not be tangible. I just think it is a neat way to convey a message.
The algorithms must be able to pick up sound and then return different lengths of lines based on the sound.
The creator’s artisitic vision is shown in this piece because of the materials he chooses to make. Even though the shape of the piece is determined by the sound file, he chooses the material used.
Here is the link to the piece by David Bizer (2015).
blog 3
Paul Li Section A Arabesque Wall (2014/2015) This project is very similar to my selection from last week, the Subdivide Column by Michael Hansmeyer. In fact, it was a project by Benjamin Dillenburger in collaboration with Hansmeyer. I just found their work so limitless and inspiring. I think the mixture of the ornamentation that is reminiscent of historical decoration and the sci-fi aspect of the forms and curves really pushes ideas of architectural concepts and forms I’ve been taught. The algorithm behind the creation was based on actual arabesque ornament examples, which are very mathematical and geometric in nature. By dividing and repositioning surfaces, tiles are altered into microscopic to large pieces that are folded and in the end composes a very complex geometry. I also really admire the amount of details of these structures, while being grand in size as well. The artistic aspect of these projects is that there is unlimited potential and every one of them can be adjusted with generative controls that ensures the uniqueness of each piece while being able to reach a certain desirable form. https://benjamin-dillenburger.com/arabesque-wall/
LO 3 – Computational Fabrication
The reason why I so admire the Voronoi architecture statue made by June Lee is that I am really impressed by the organic form in architecture. The Voronoi diagram is a type of partition created by segmenting midpoints of distances of different points. To make this random geometric two-dimensional diagram look smooth and organic, the creator smoothened the shape of each Voronoi cell in grasshopper, then using the negative space created by smaller smoothened cells and the square border of the cube, the creator made a wall with organic Voronoi shaped holes. One great thing about using the grasshopper algorithm to generate shapes is that it allows the creator to easily tweak and manipulate every aspect of the geometry. For example, in this project, June Lee is able to change the size of the cube, how big the holes are, and even how smooth those holes are, without remaking the whole shape.
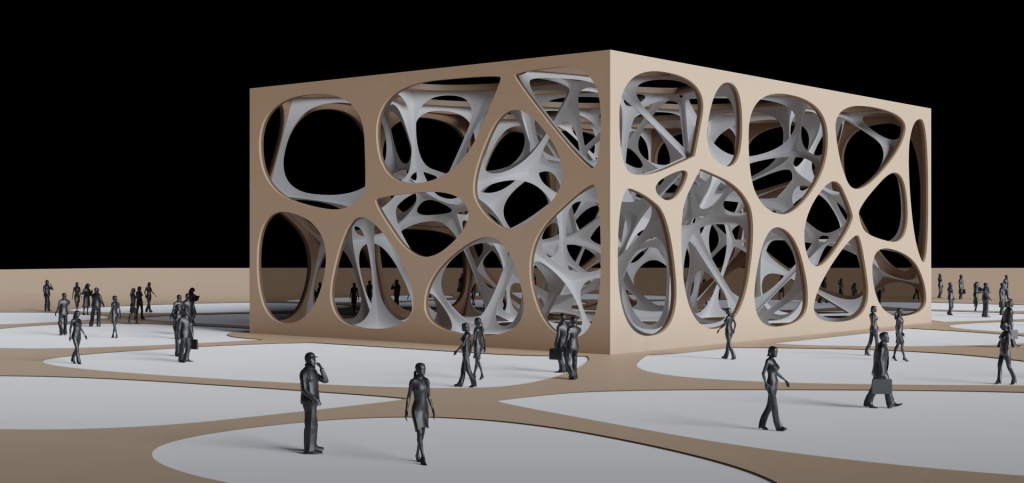
Project 03: Dynamic Drawing
- Size of large circle changes
- Shade of large circle changes
- Rotation of smaller circle changes
- Stroke of smaller circles changes
var uniX = 0;
var uniY = 0;
function setup() {
createCanvas(600, 450);
background(0);
}
function draw() {
uniX = mouseX;
uniY = mouseY;
background(0);
push();
fill(255);
ellipse (uniX,uniY,200);
pop();
for (let i = 25; i <= 575; i+=50)
{
for (let j = 25; j <= 425; j+=50)
{
circleChange(i,j);
}
}
}
function circleChange(Cx,Cy) {
distanceO = Math.sqrt((uniX-Cx)*(uniX-Cx) + (uniY-Cy)*(uniY-Cy));
distance = constrain(distanceO, 10, 200);
var diameter = distance/4;
var angle = Math.atan2((uniY-Cy),(uniX-Cx));
push();
strokeWeight(1);
stroke(0,0,255,255);
noFill();
arc(Cx, Cy, diameter, diameter, -0.25*PI, 0.75*PI);
pop();
push();
strokeWeight(1);
stroke(255,0,0,255);
arc(Cx, Cy, diameter, diameter, 0.75*PI, 1.75*PI);
pop();
push();
strokeWeight(0);
fill(distance/200*255);
ellipse (Cx,Cy,diameter-2);
pop();
fill(255);
strokeWeight(distanceO/60);
ellipse((Cx+diameter*Math.cos(angle)/3),(Cy+diameter*Math.sin(angle)/3),diameter/3);
}
Project 02 Variable Face
Variables:
- The face changes color as u move your mouse.
- Eyes and arms follows the mouse.
- When mouse is pressed, the hands will grow fingers.
var mouse = false;
function setup() {
createCanvas(480, 640);
background(220);
}
function draw() {
background(220);
drawFace();
noFill();
drawMouth();
drawTeeth();
drawLip();
eyeBallOne();
eyeBallTwo();
handOne();
handTwo();
}
function drawMouth() {
strokeWeight(4);
fill(255,0,0,255);
curve(145, 250, 145, 200, 335, 200, 335, 250);
curve(145, -400, 145, 200, 335, 200, 335, -400);
}
function drawLip() {
strokeWeight(4);
stroke(0);
noFill();
curve(145, 250, 145, 200, 335, 200, 335, 250);
curve(145, -400, 145, 200, 335, 200, 335, -400);
}
function drawTeeth() {
strokeWeight(3);
stroke(255);
line(145, 200, 175, 248);
line(335, 200, 305, 248);
line(175, 248, 205, 195);
line(305, 248, 275, 195);
line (205, 195, 240, 275);
line (275, 195, 240, 275);
}
function drawFace() {
strokeWeight(5);
let xValue = mouseX/width*255;
let yValue = mouseY/width*255;
let zValue = (xValue+yValue)/4;
fill(xValue,yValue,zValue+120);
rect (100,100,280,320);
}
function eyeBallOne() {
strokeWeight(4);
fill(255,255,255);
ellipse (150,150,50);
let angle = atan2(mouseY - 150, mouseX - 150); // calculate arctangent //
let x = 150 + 20*0.75*cos(angle);
let y = 150 + 20*0.75*sin(angle);
fill(255,0,255);
circle (x,y,15);
}
function eyeBallTwo() {
strokeWeight(4);
fill(255,255,255);
ellipse (330,150,50);
let angle = atan2(mouseY - 150, mouseX - 250); // calculate arctangent //
let x = 330 + 20*0.75*cos(angle);
let y = 150 + 20*0.75*sin(angle);
fill(150,150,0);
circle (x,y,15);
}
function handOne() {
var x = 100;
var y = 420;
var tan = atan2(mouseX-x,mouseY-y);
if (dist (mouseX,mouseY,x,y) < 200)
//if arm mouse is within arm distance//
{ var dis = dist (mouseX,mouseY,x,y) /2;
// mid point of distance between mouse and arm//
var num = (10000)-(dis*dis);
var sid = sqrt(num);
//pathegoriam therom//
stroke (10);
line (x,y, (mouseX-x)/2+x+cos(tan)*sid, (mouseY-y)/2+y-sin(tan)*sid);
line ((mouseX-x)/2+x+cos(tan)*sid, (mouseY-y)/2+y-sin(tan)*sid, mouseX, mouseY);
fill(255,255,255);
ellipse ((mouseX-x)/2+x+cos(tan)*sid, (mouseY-y)/2+y-sin(tan)*sid, 25,25);
if (mouse)
{
strokeWeight(5);
line (mouseX, mouseY, mouseX+30, mouseY-30);
line (mouseX, mouseY, mouseX-30, mouseY-30);
line (mouseX, mouseY, mouseX, mouseY-60);
ellipse (mouseX,mouseY,50,50);
}
else {
ellipse (mouseX,mouseY,50,50);
}
}
else
{
stroke(10);
line (x,y, x+sin(tan)*200, y+cos(tan)*200);
fill(255,255,255);
ellipse (x+sin(tan)*100, y+cos(tan)*100, 25,25);
if (mouse)
{
//hand//
strokeWeight(5);
line (x+sin(tan)*200, y+cos(tan)*200, x+sin(tan)*200+30, y+cos(tan)*200-30);
line (x+sin(tan)*200, y+cos(tan)*200, x+sin(tan)*200-30, y+cos(tan)*200-30);
line (x+sin(tan)*200, y+cos(tan)*200, x+sin(tan)*200, y+cos(tan)*200-60);
//fingers//
ellipse (x+sin(tan)*200, y+cos(tan)*200,50,50);
}
else {
ellipse (x+sin(tan)*200, y+cos(tan)*200,50,50);
}
}
}
function handTwo() {
var x = 380;
var y = 420;
var tan = atan2(mouseX-x,mouseY-y);
if (dist (mouseX,mouseY,x,y) < 200)
//if arm mouse is within arm distance//
{ var dis = dist (mouseX,mouseY,x,y) /2;
// mid point of distance between mouse and arm//
var num = (10000)-(dis*dis);
var sid = sqrt(num);
//pathegoriam therom//
stroke (10);
line (x,y, (mouseX-x)/2+x-cos(tan)*sid, (mouseY-y)/2+y+sin(tan)*sid);
//origin point to the medien of the triangle (isosceles)//
line ((mouseX-x)/2+x-cos(tan)*sid, (mouseY-y)/2+y+sin(tan)*sid, mouseX, mouseY);
fill(255,255,255);
ellipse ((mouseX-x)/2+x-cos(tan)*sid, (mouseY-y)/2+y+sin(tan)*sid, 25,25);
if (mouse)
{
strokeWeight(5);
line (mouseX, mouseY, mouseX+30, mouseY-30);
line (mouseX, mouseY, mouseX-30, mouseY-30);
line (mouseX, mouseY, mouseX, mouseY-60);
ellipse (mouseX,mouseY,50,50);
}
else {
ellipse (mouseX,mouseY,50,50);
}
}
else
{
stroke(10);
line (x,y, x+sin(tan)*200, y+cos(tan)*200);
fill(255,255,255);
ellipse (x+sin(tan)*100, y+cos(tan)*100, 25,25);
if (mouse)
{
strokeWeight(5);
line (x+sin(tan)*200, y+cos(tan)*200, x+sin(tan)*200+30, y+cos(tan)*200-30);
line (x+sin(tan)*200, y+cos(tan)*200, x+sin(tan)*200-30, y+cos(tan)*200-30);
line (x+sin(tan)*200, y+cos(tan)*200, x+sin(tan)*200, y+cos(tan)*200-60);
ellipse (x+sin(tan)*200, y+cos(tan)*200,50,50);
}
else {
ellipse (x+sin(tan)*200, y+cos(tan)*200,50,50);
}
}
}
function mousePressed() {
mouse =!mouse;
// when pressed show fingers//
}
LO 2 – Generative Art
I really admire the 3D printed items made by the Nervous system for their choice of forms and their shapes, minimizing the use of materials and still managing to serve the function of the item while creating fancy patterns out of those hollowed materials.
Additionally, I also made several 3D models similar to the ones that the Nervous System made through using the grasshopper and rhinoceros program. I am pretty sure that most of the models of the ones shown on the Nervous System websites are made through making one or more Voronoi diagrams first then thicken and smoothen the lines to make it into a solid shape. One good aspect of using Grasshopper is that most variables of the 3D models are configurable, making each design can have an infinite amount of iterations.
I think the creator wanted to create something in between visibility and invisibility through the hollowed shapes using the mathematical methods to minimize visible parts of each item it creates while still working as an object without those holes.
Reference: https://n-e-r-v-o-u-s.com/shop/search_tags.php?search=3dprin
https://n-e-r-v-o-u-s.com/shop/search_tags.php?search=3dprint
Project 1: My Self Portrait
function setup() {
createCanvas(400, 400);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
ears ();
drawFace();
noFill();
drawMouth();
drawTeeth();
drawLip();
eyeBallOne();
eyeBallTwo();
}
function drawMouth() {
strokeWeight(4);
fill(255,0,0,255);
curve(105, 250, 105, 200, 295, 200, 295, 250);
curve(105, -400, 105, 200, 295, 200, 295, -400);
}
function drawLip() {
strokeWeight(4);
stroke(0);
noFill();
curve(105, 250, 105, 200, 295, 200, 295, 250);
curve(105, -400, 105, 200, 295, 200, 295, -400);
}
function drawTeeth() {
strokeWeight(3);
stroke(255);
line(105, 200, 135, 248);
line(295, 200, 265, 248);
line(135, 248, 165, 195);
line(265, 248, 235, 195);
line (165, 195, 200, 275);
line (235, 195, 200, 275);
}
function drawFace() {
strokeWeight(5);
let xValue = mouseX/width*255;
let yValue = mouseY/width*255;
let zValue = (xValue+yValue)/4;
fill(xValue,yValue,zValue+120);
bezier(50, 225, 50, 400, 350, 400, 350, 225);
bezier(50, 225, 50, -50, 350, -50, 350, 225);
}
function eyeBallOne() {
strokeWeight(4);
fill(255,255,255);
ellipse (150,150,50);
let angle = atan2(mouseY - 150, mouseX - 150); // calculate arctangent //
let x = 150 + 20*0.75*cos(angle);
let y = 150 + 20*0.75*sin(angle);
fill(255,0,255);
circle (x,y,15);
}
function eyeBallTwo() {
strokeWeight(4);
fill(255,255,255);
ellipse (250,150,50);
let angle = atan2(mouseY - 150, mouseX - 250); // calculate arctangent //
let x = 250 + 20*0.75*cos(angle);
let y = 150 + 20*0.75*sin(angle);
fill(150,150,0);
circle (x,y,15);
}
function ears() {
strokeWeight(5);
let xValue = mouseX/width*255;
let yValue = mouseY/width*255;
let zValue = (xValue+yValue)/4;
fill(xValue,yValue,zValue+120,100);
ellipse (350,150,50.100);
ellipse (50,150,50.100);
}