
Problem: I cook almost exclusively on cast iron. It takes a while to heat up, but retains that heat super well. There are often times where I have to step away from the skillet for a while to let it cool down. I also moved in with new roommates recently who promptly touched the handle when it was still scalding hot. It is difficult to tell how hot the pan is without holding the back of your hand up to it; there is a small visual indicator of heat coming off it, but it is minute. The primary feedback is physical. A further issue is our individual heat tolerance–I can take and wash the pan / reseason it in the sink when its hotter than when my roomates can or want to. It is impossible to tell just how hot it is without actually touching it, thermometer excluded.
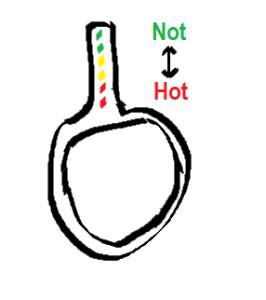
Solution: Basically, heat indicators built into the pan. This would allow a user to know at a glance if the pan were hot or not, instead of using their hand. This would be accomplished by a thermometer testing the surface of the pan. Because cast iron pans heat fairly evenly, it has a fairly large margin of error in terms of where its sampling from. No idea on how thermometers actually work so that may be wrong.
The embedded LEDs range from green to red, fairly common stop and go indicators. Red fairly clearly means hot when used on cookware as well. I see the lights lighting up linearly from Not to Hot based on temperature, so only 1 LED would be in use if it were cold, and all in use when Hot.
I think this would at least ease the use of ambiguously hot pans, in a similar way to the audio feedback project by another student from Assignment #2.
Proof of Concept:
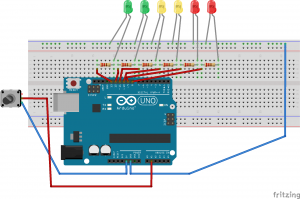
Chance Assignment 4
Fairly straightforward code that lights up LEDs in a row based on how large a value is read from a pot, acting as a thermostat stand in. I am pretty sure I fried one of my pots but this does work fine, so hope for that demo in class!
// Chance, Assignment 4. Not really digital states, but approximations of analog ones
#define Serial SerialUSB
const int buttonPin = 2; // momentary button
const int greenLED = 13; // green LED
const int green2LED = 12; // green LED
const int yelLED = 11; // yellow LED
const int yel2LED = 10; // yellow LED
const int redLED = 9; // red LED
const int red2LED = 8; // red2 LED
const int potPin = 1; // analog 1
float LEDcount = 6.0f; // should just cast this later but fine to be declared as this
int startPin = 8;
void setup() {
// initialize the LED pins as an outputs:
for (int i = red2LED; i <= greenLED; i++) {
pinMode(i, OUTPUT);
}
// initialize the pot pin as an analog input:
pinMode(potPin, INPUT);
}
void loop() {
// read in the pot / thermo stand-in
int val = analogRead(potPin);
Serial.println(val);
for(int i = LEDcount - 1; i >= 0; i--) {
if(val >= (((float)i / LEDcount) * 1023)) digitalWrite(i + startPin, HIGH);
else
digitalWrite(i + startPin, LOW);
}
}