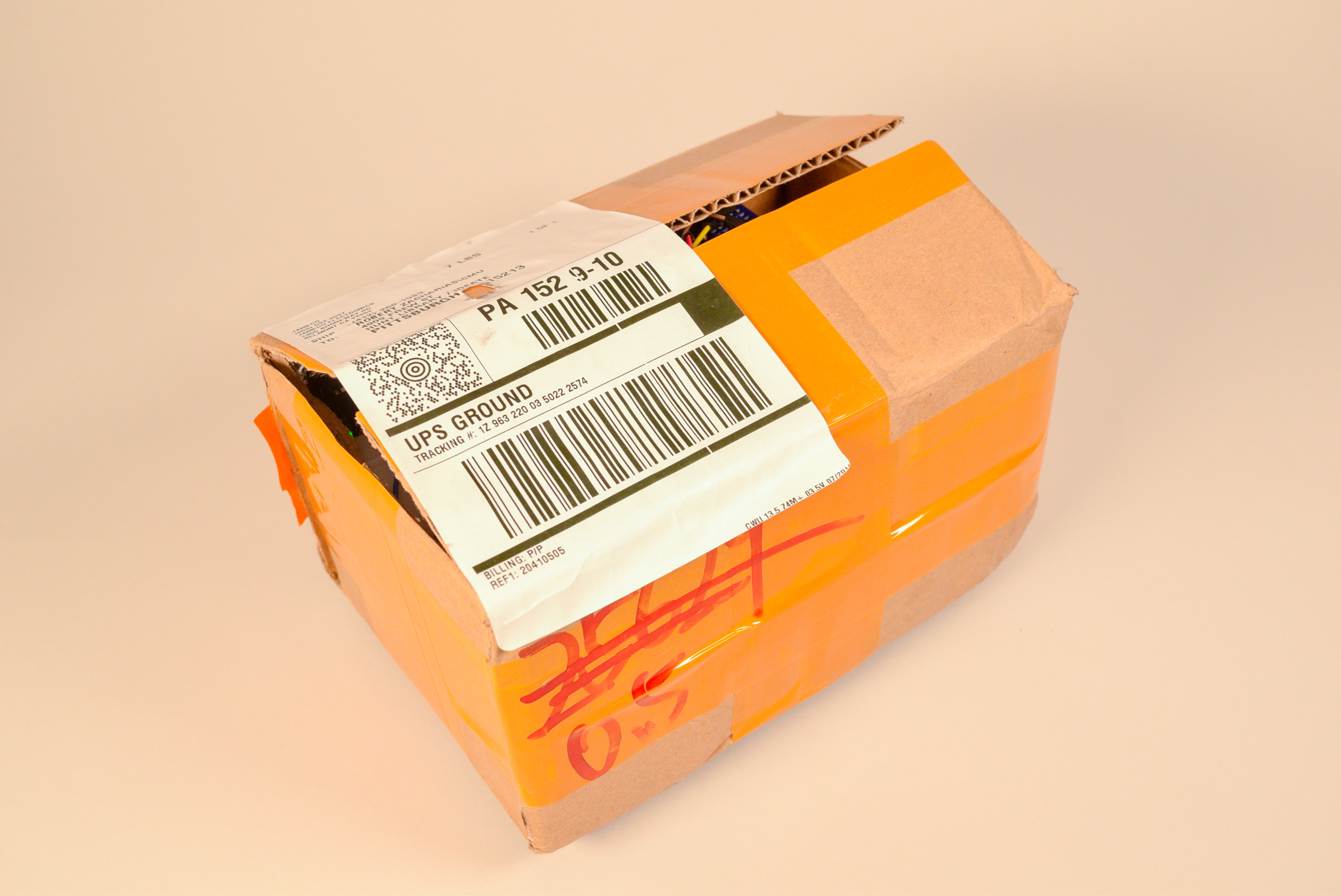
The appearance of moving courier box.
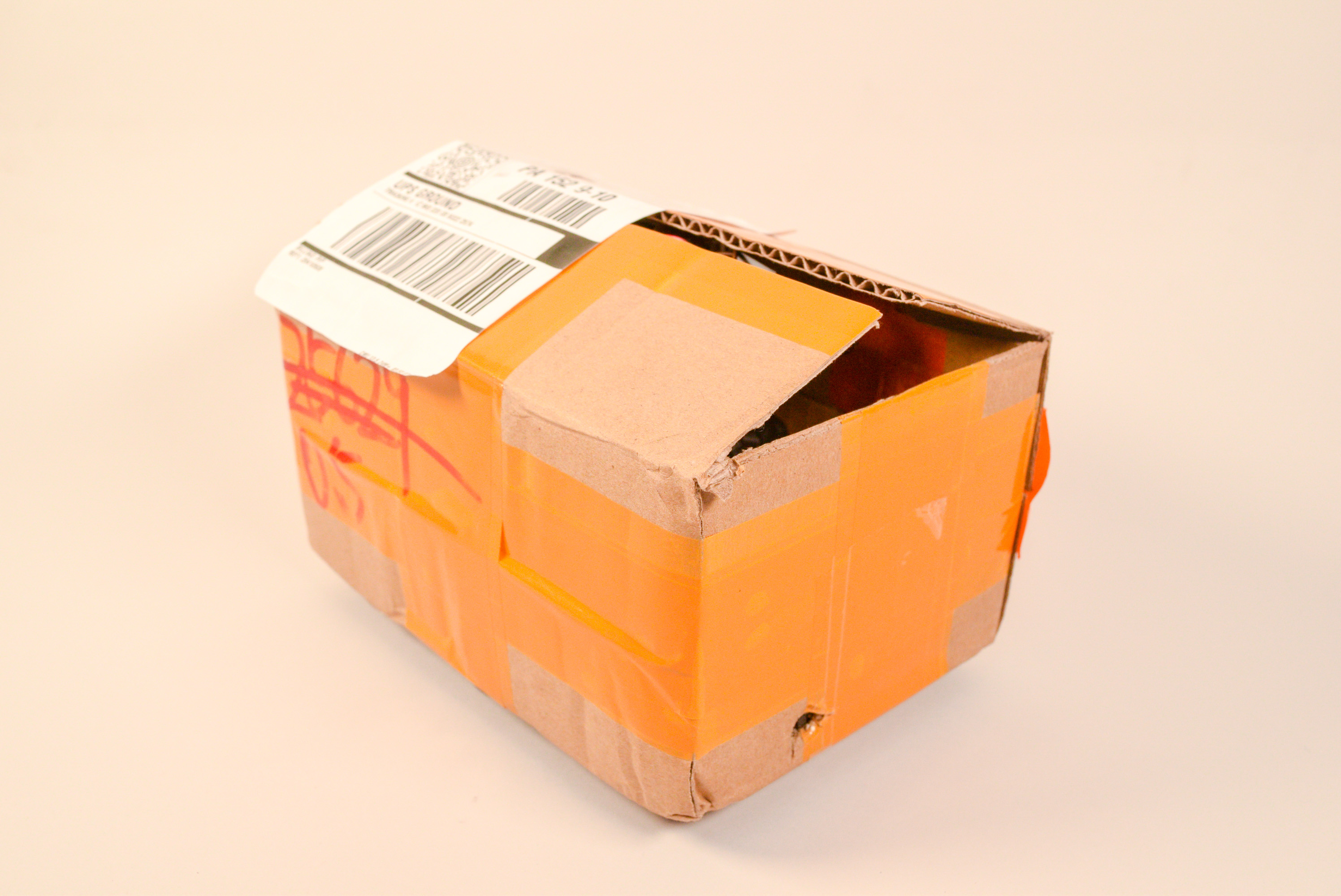
The appearance of moving courier box.
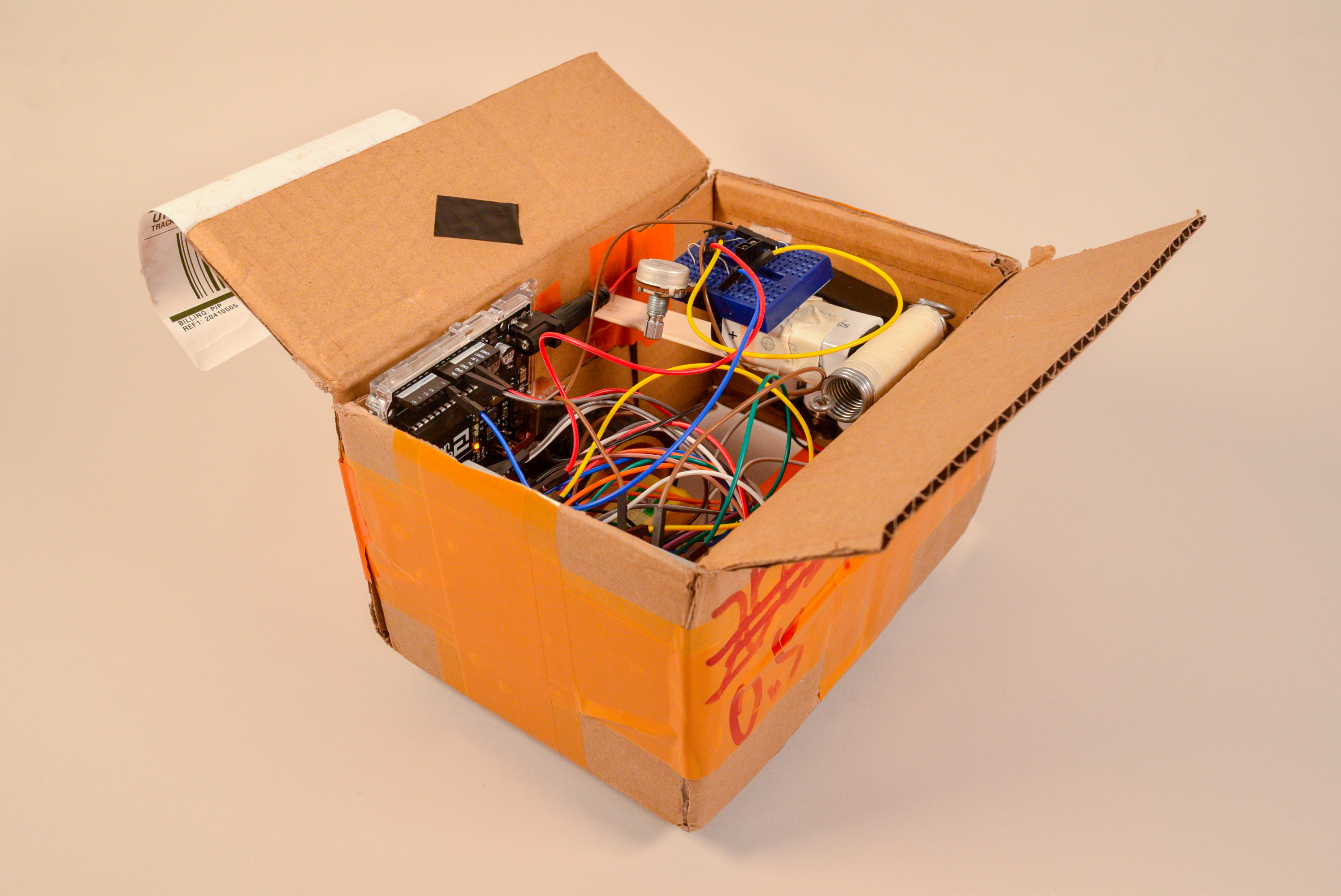
The internal structure of moving courier box.
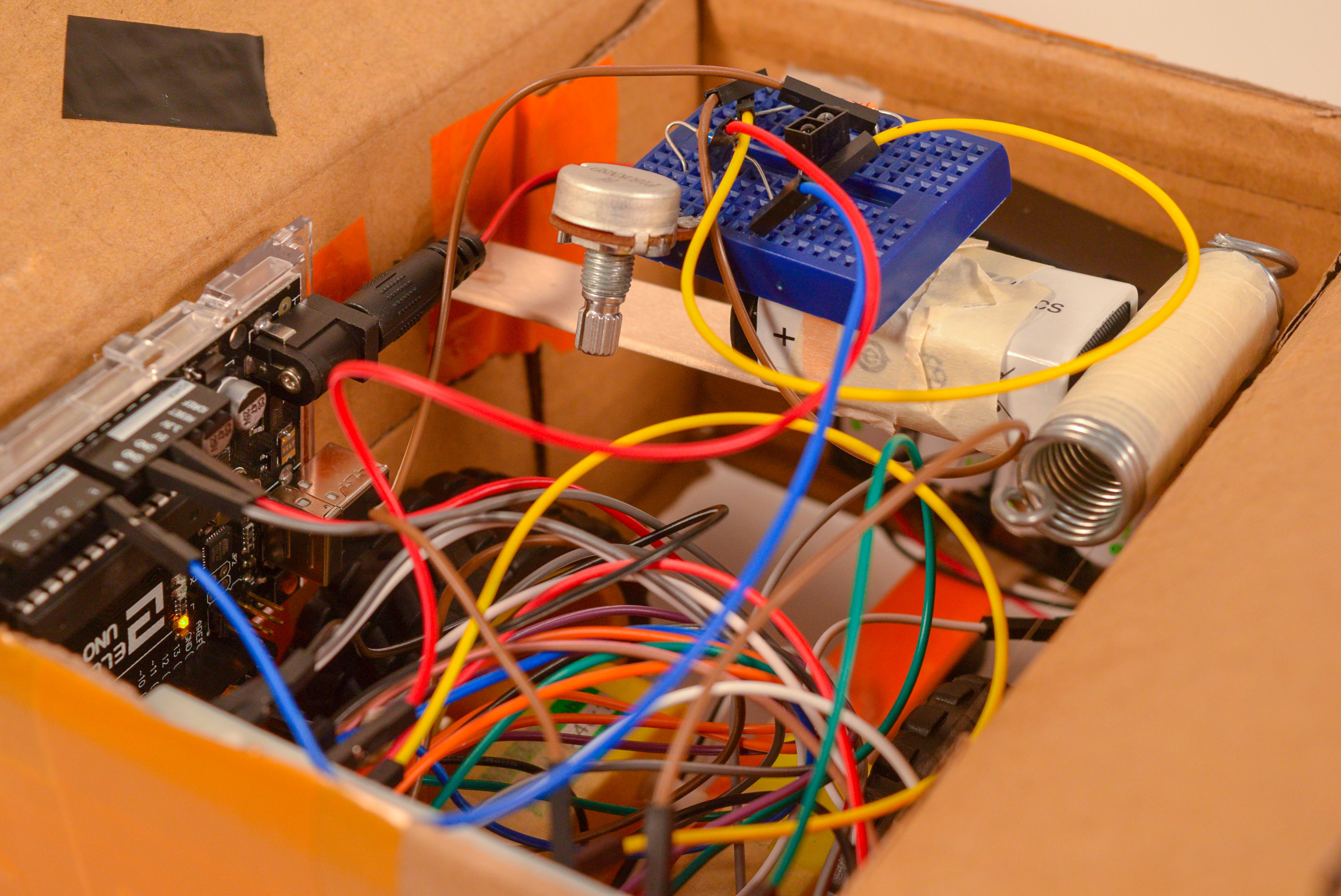
The internal structure of moving courier box.
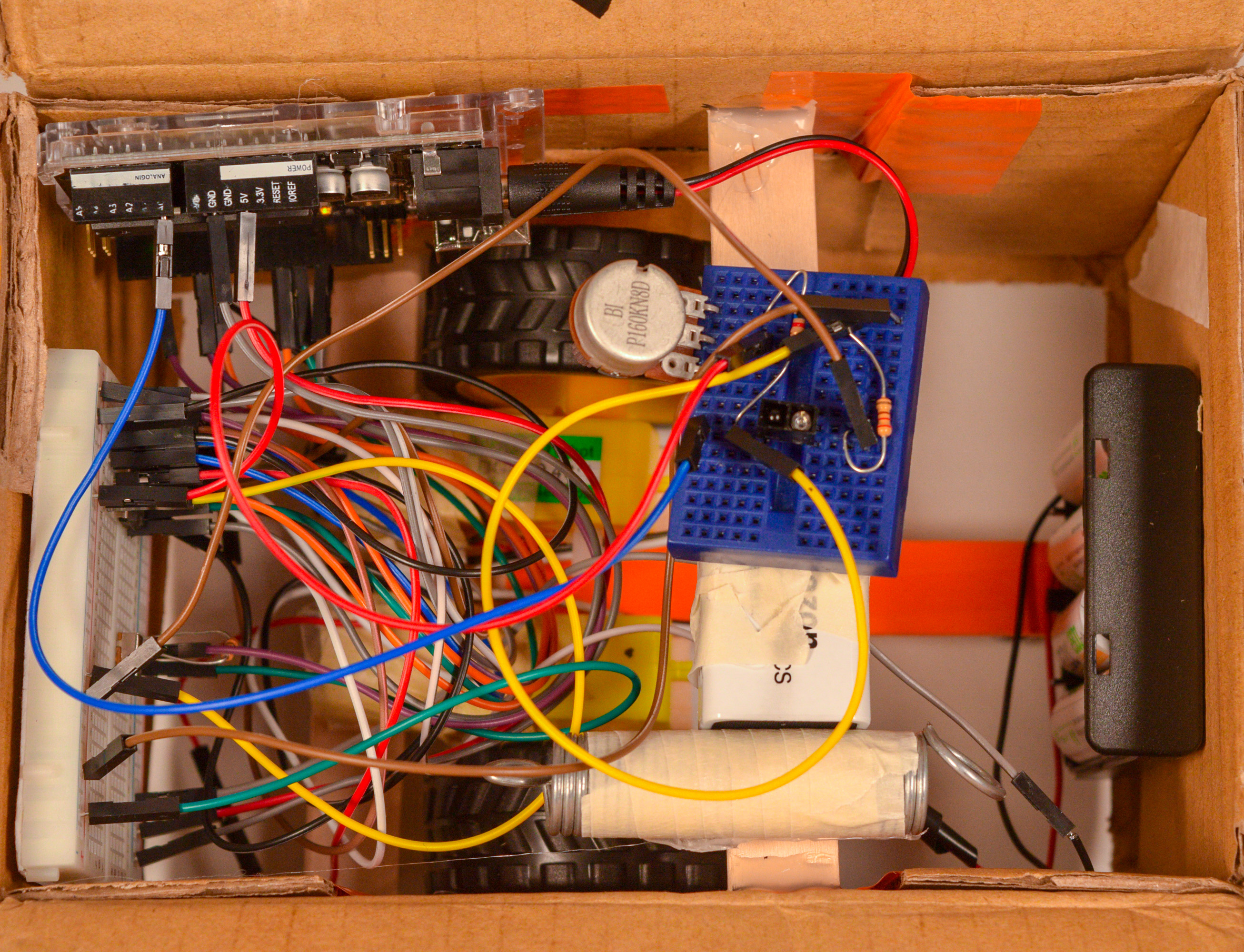
The top view of moving courier box.
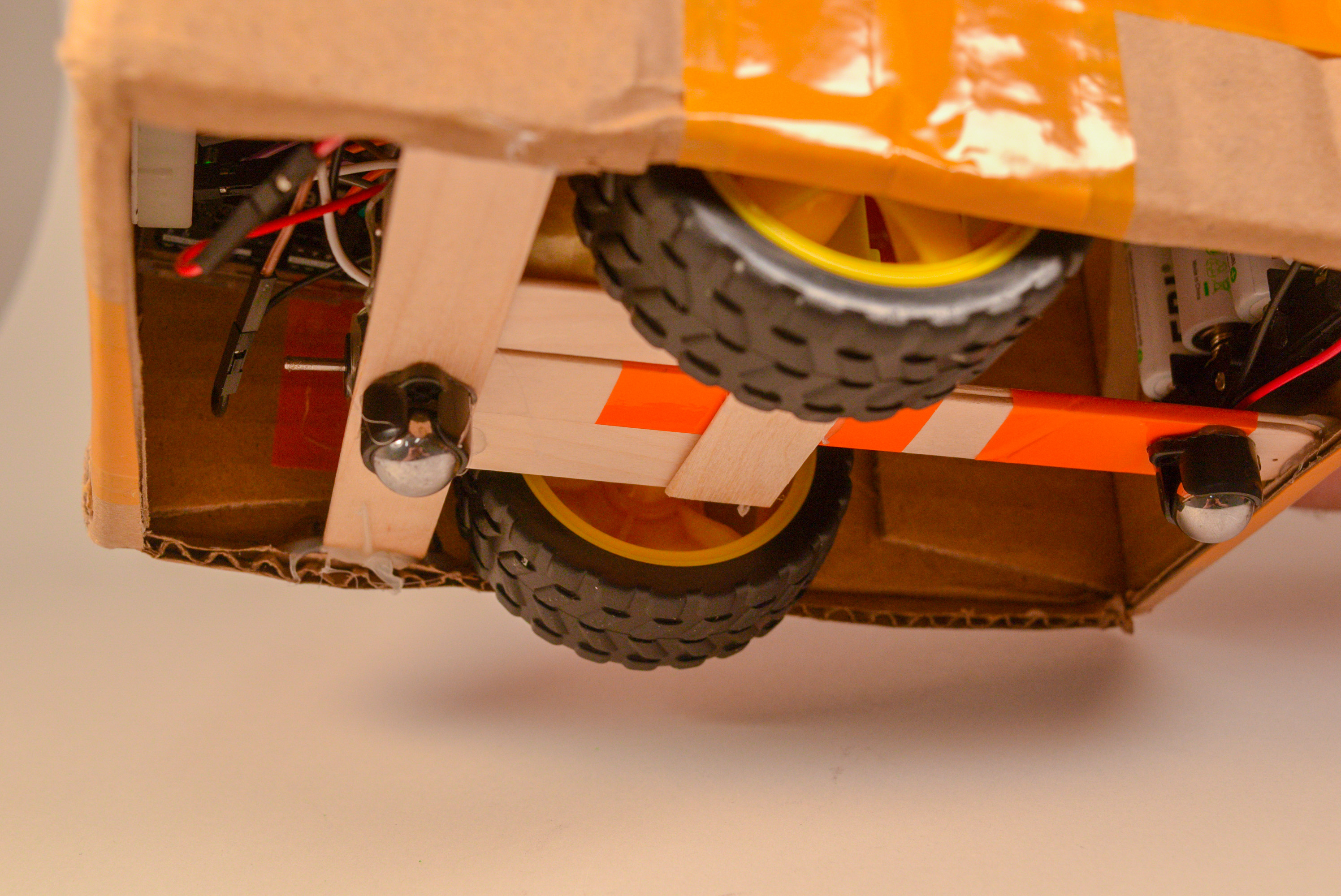
Bottom of moving courier box.
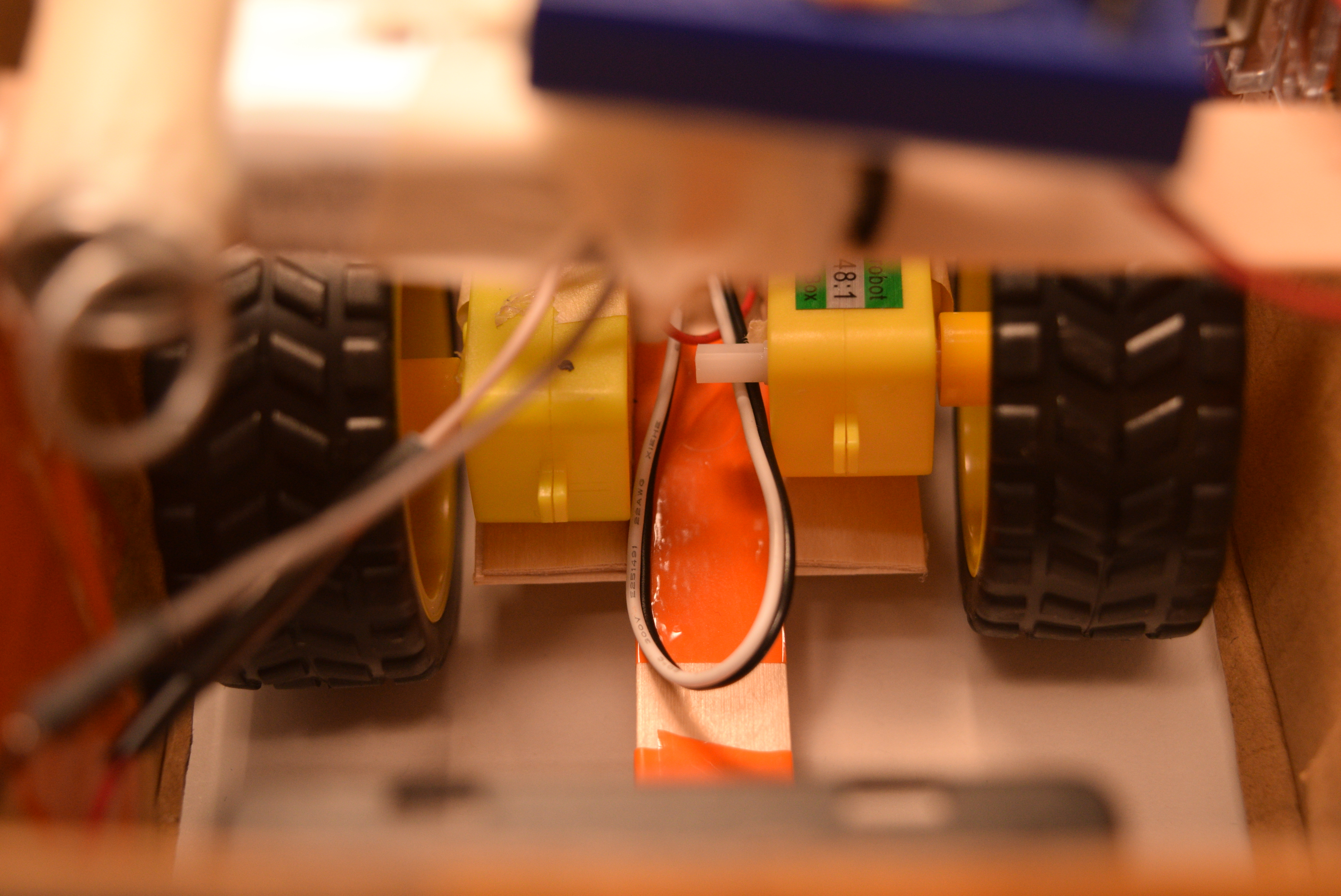
The driving motors and wheels of the moving courier box.
Narrative Description
The project was inspired by an Arduino project found online where a prank was done to people who were trying to get tissue from a tissue box. The tissue box would sense a hand is getting close and the box would move away from the approaching hand, not letting the person get the tissue. The aim of this project was similar, which was producing an object that would sense a human approaching and run away.
The object was intentionally chosen as an object that would be an everyday-item that people would expect to see at any place instead of an item specially made for this project. This induces the surprise of the project by making an object that people would not expect to move. Original ideas included chairs or tables in the lab, but because of physical constraints, the object was chosen to be a courier box.
progress images
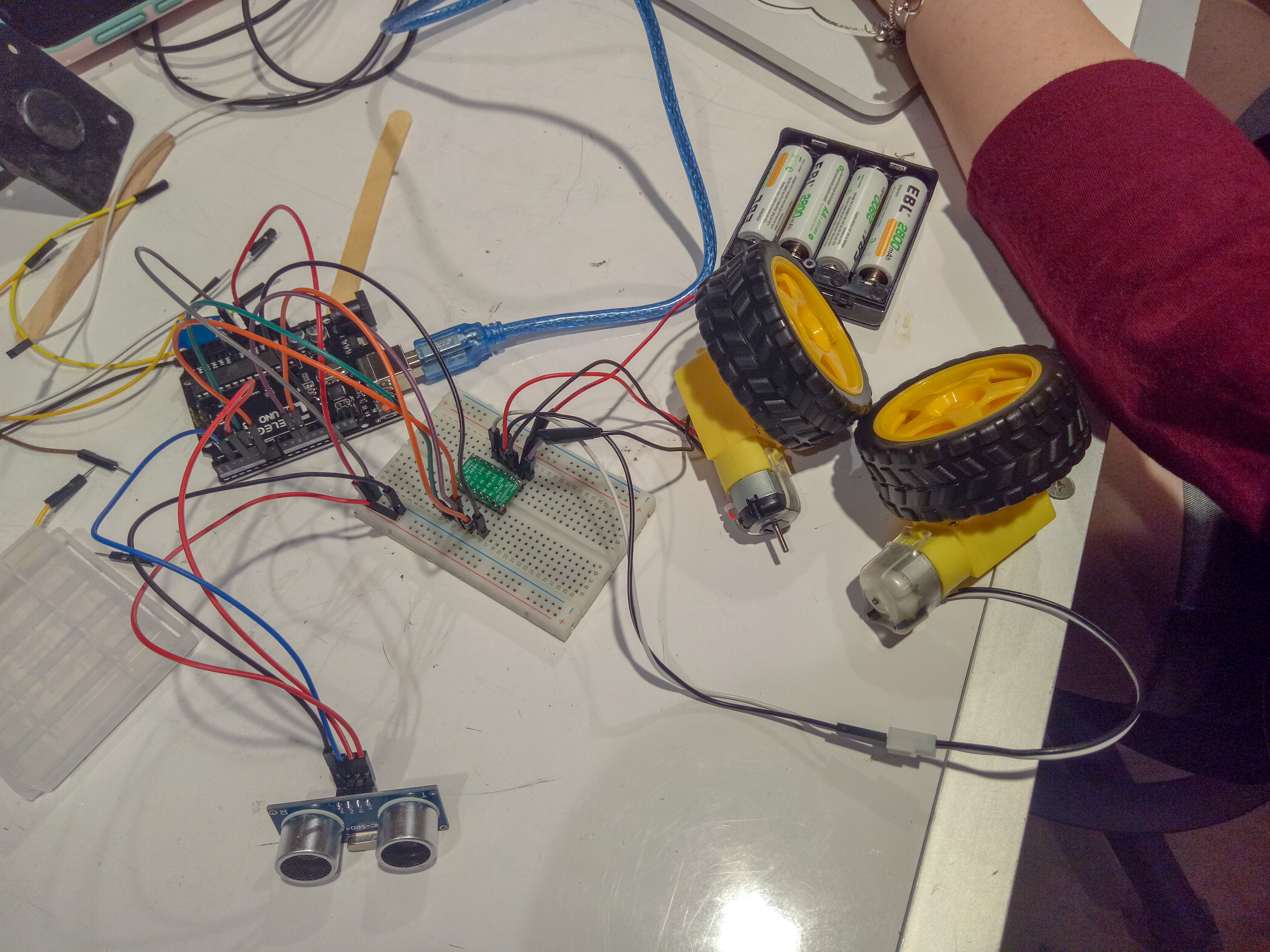
An ultrasonic ranger was used in our first attempt. However, it didn’t work well.
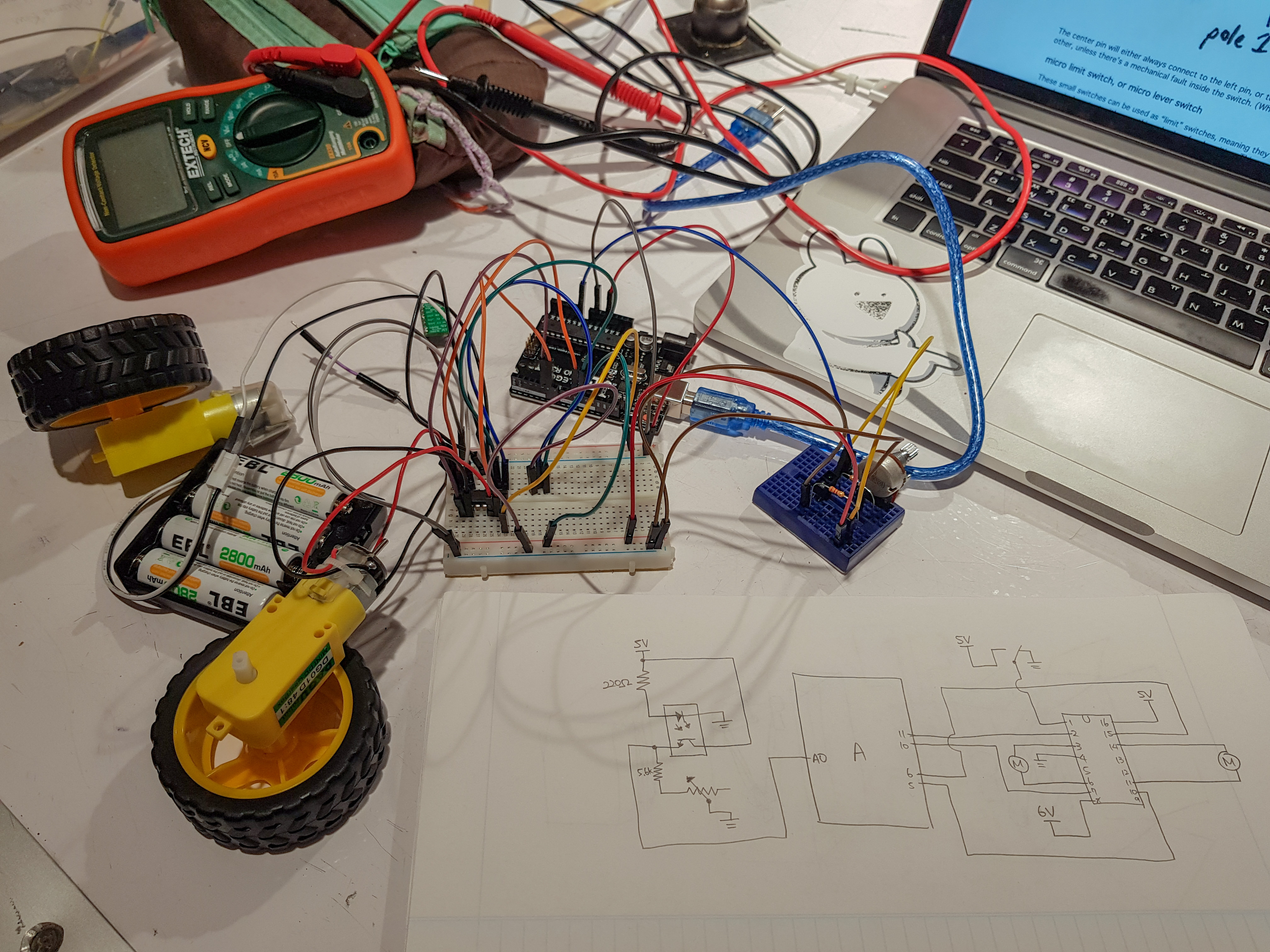
After several attempts, the IR sensor was selected since its good performance. The code and circuit parts were basically completed.
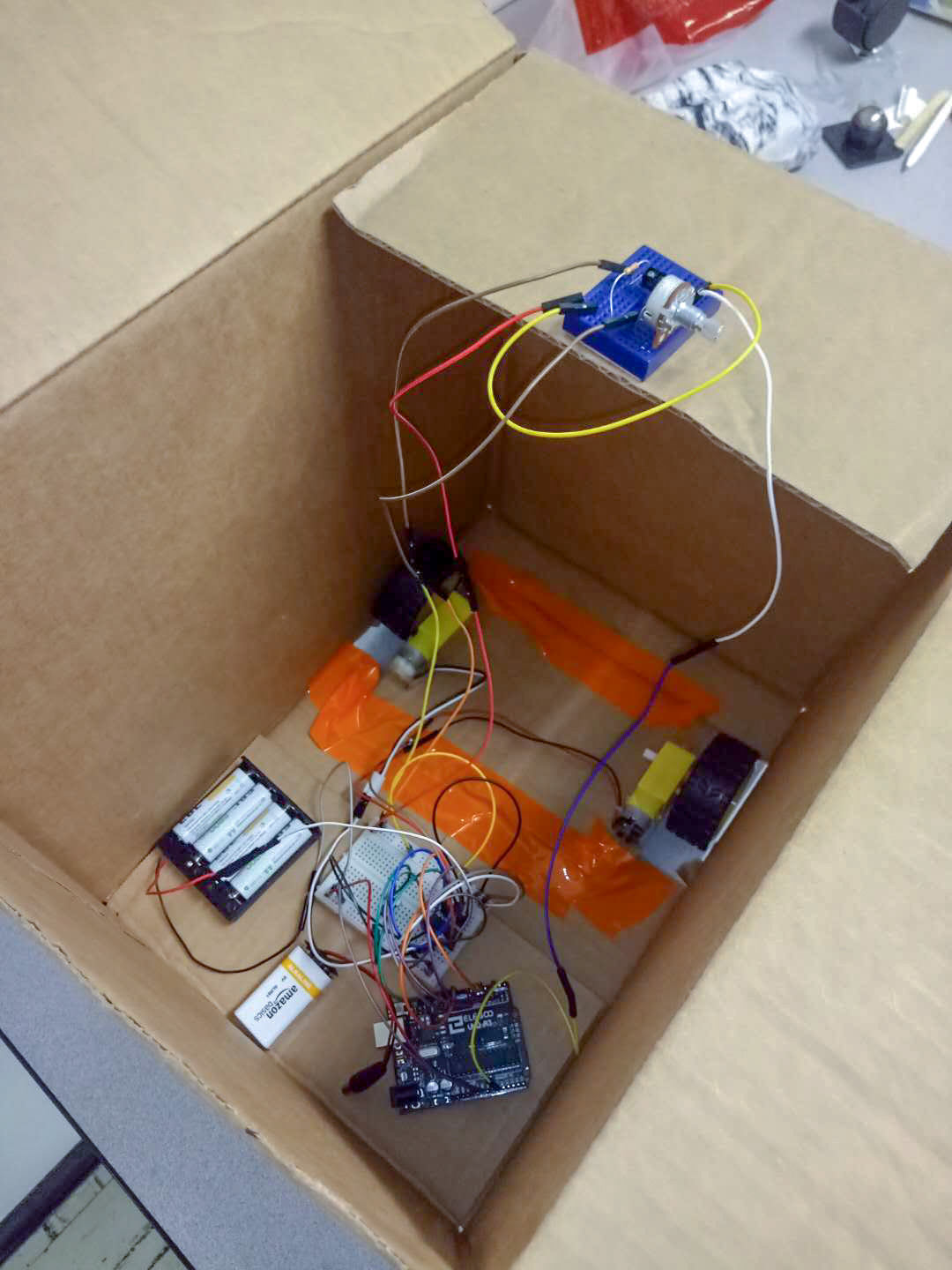
The power output of the motors was not enough to support the movement of the large box. Also, the bottom cardboard was bent due to the weight of the battery and other stuff. Therefore, we decided to change the size of the box.
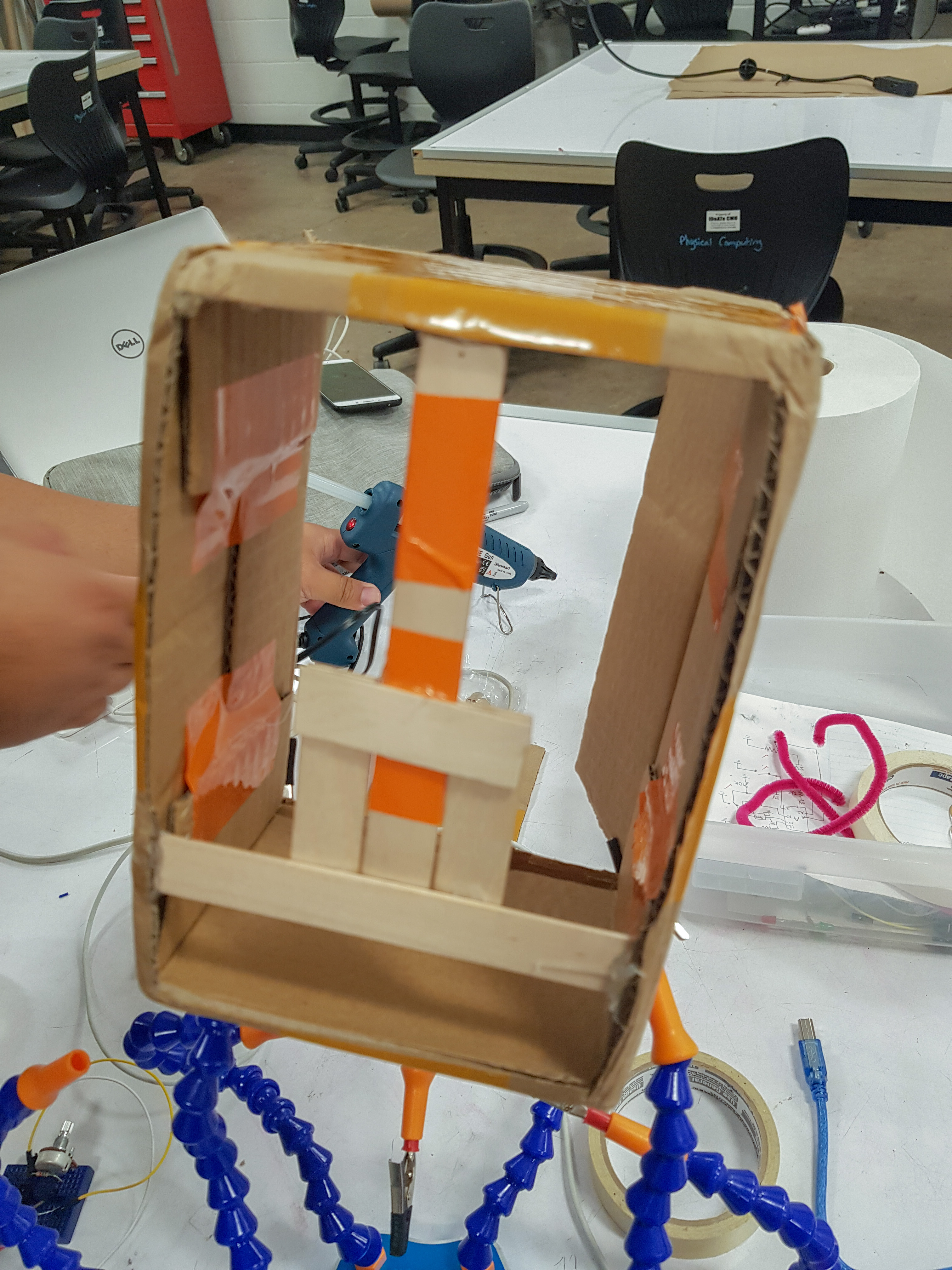
Having learned the lesson for the first time, we made the underside support structure out of wood to make sure the motors and wheels could work properly.
Discussion
Our initial concept was that once a hand approaches above the box in an attempt to open it, the box would sense the hand and run away from it in a random direction with random speed, using the random numbers that were generated by the Arduino.
Instead of the ultrasonic ranger that was introduced in class, an IR sensor has been used due to the instability of the data that the ultrasonic ranger gave and the smaller size of the IR sensor.
After the completion of the code and circuit parts, we tried to put it in a large cardboard box, but the power output of the motor was not enough to support the movement of the box. What’s more, the bottom cardboard was bent due to the weight of the battery and other stuff. Therefore, we had to choose a smaller courier box to achieve our initial goal.
The IR sensor’s unstable data and the physical installation of the electronics into the box resulted in a wobbly irregular movement of the box. It was not behaving perfectly as originally planned, but the mere appearance of a package box with a label moving around on a table still had a surprising element of an ordinary item moving as intended.
Schematic
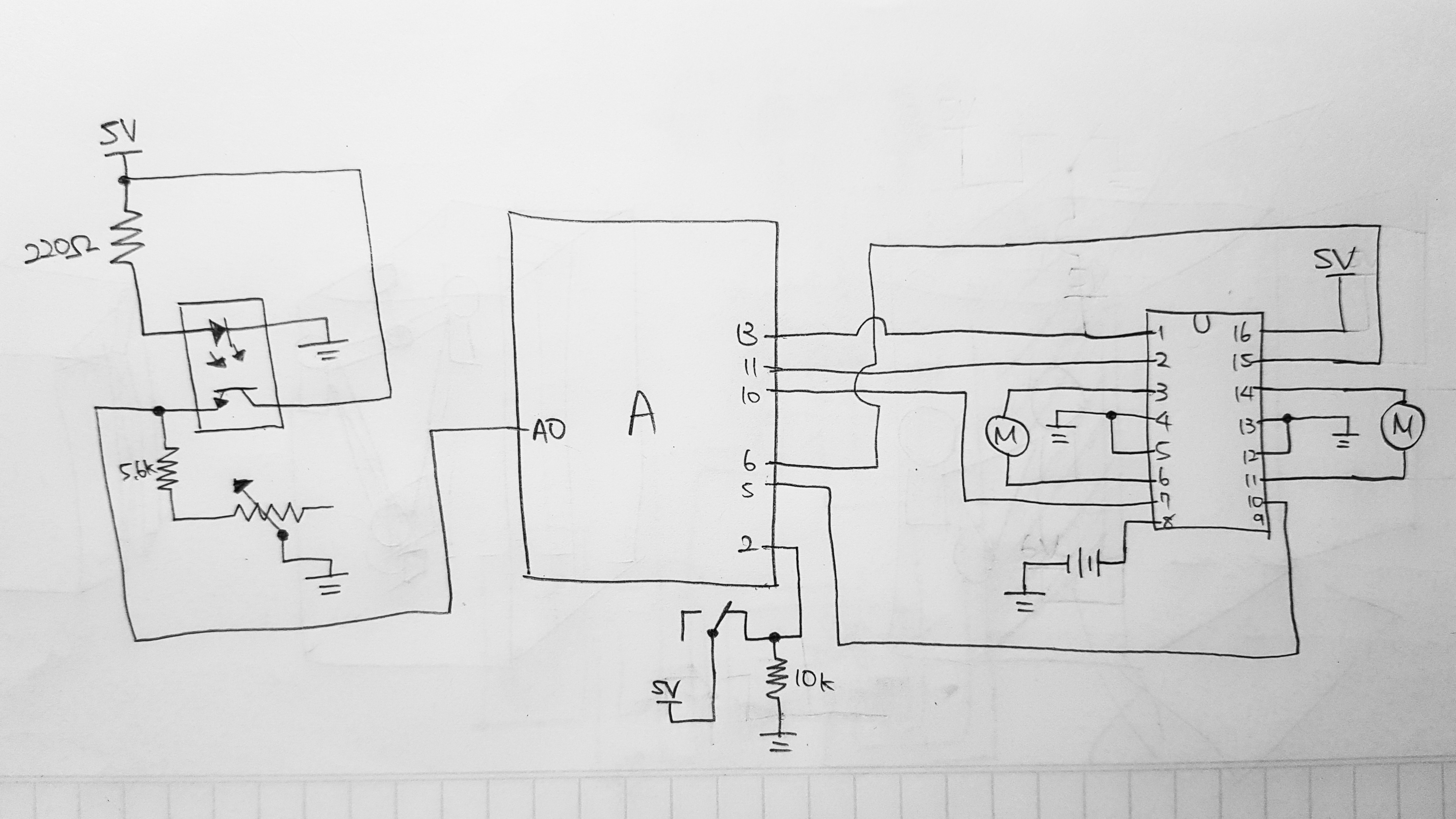
Schematic of the moving courier box
Code
//pin numbers const int MOTORR1 = 6; //first pin for motor on the right const int MOTORR2 = 5; //second pin for motor on the right const int MOTORL1 = 10; //first pin for motor on the left const int MOTORL2 = 11; //second pin for motor on the left const int IRSENSOR = A0; const int SWITCHPIN = 2; const int DRIVERPIN = 13; int direc; int speedR; int speedL; void setup() { //Initialize pins pinMode(MOTORR1, OUTPUT); pinMode(MOTORR2, OUTPUT); pinMode(MOTORL1, OUTPUT); pinMode(MOTORL2, OUTPUT); pinMode(IRSENSOR, INPUT); //sensing distance pinMode(SWITCHPIN, INPUT); //decides whether to turn the driver on or off pinMode(DRIVERPIN, OUTPUT); //on/off will be delivered to driver //box will start not moving digitalWrite(MOTORR1, LOW); digitalWrite(MOTORR2, LOW); digitalWrite(MOTORL1, LOW); digitalWrite(MOTORL2, LOW); randomSeed(analogRead(0)); //don't plug anything into 0 //Serial.begin(9600); } void loop() { //box will check if anything is too close int distance = analogRead(IRSENSOR); //Serial.println(distance); direc = random(2); speedR = random(255); speedL = random(255); int switchVal = digitalRead(SWITCHPIN); //driver turned on if (switchVal == HIGH) { digitalWrite(DRIVERPIN, HIGH); //maximum distance below is 50 for now, but it can be adjusted by wiggling the potentiometer //IR sensor will give low number when nothing is nearby and high number when something is close if (distance > 50 ) { //people are too close, so it wants to run away 🙁 if (direc == 0) { //box moves forward //speed for motors will be different from each other //speed for each motor will be random so that the box will move at a random direction analogWrite(MOTORR1, speedR); analogWrite(MOTORR2, 0); analogWrite(MOTORL1, speedL); analogWrite(MOTORL2, 0); //Serial.println("forward,"+ String(speedR)); delay(3000); } if (direc == 1) { //box moves backward analogWrite(MOTORR1, 0); analogWrite(MOTORR2, speedR); analogWrite(MOTORL1, 0); analogWrite(MOTORL2, speedL); //Serial.println("backward"); delay(3000); } } else { digitalWrite(MOTORR1, LOW); digitalWrite(MOTORR2, LOW); digitalWrite(MOTORL1, LOW); digitalWrite(MOTORL2, LOW); } } else { digitalWrite(DRIVERPIN, LOW); //Serial.println("driver off"); } }
Leave a Reply
You must be logged in to post a comment.