// This program deconstructs an image and then reconstructs it in a new way.
// The image is sliced into pieces and then drawn together in a new order
// Horizontally and vertically
var img;
var lineHeight = [];
var lineWidth = [];
var numSlices = 6; //must be even
// slices are ordered and grouped into 4 arrays
var imgSlices1 = [];
var imgSlices2 = [];
var imgSlices3 = [];
var imgSlices4 = [];
function preload() {
img = loadImage('https://i.imgur.com/c6ZRijQ.jpg');
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
noStroke();
img.loadPixels();
// get x and y values for the slices based on canvas size and number of slices
for (var i=0; i<numSlices; i++) {
let h = height/(numSlices+1);
let h1 = height/numSlices;
let w = width/(numSlices+1);
let w1 = width/numSlices;
lineHeight[i] = h1*i + h1/2;
lineWidth[i] = w1*i + w1/2;
// rearrange image pieces based on odd or even and fill arrays
if (i%2==0) {
imgSlices2.push(img.get(0, lineHeight[i], width/2, h));
imgSlices4.push(img.get(lineWidth[i], 0, w, height));
}
else {
imgSlices1.push(img.get(0, lineHeight[i], width/2, h));
imgSlices3.push(img.get(lineWidth[i], 0, w, height));
}
}
}
function draw() {
//there is some background that shows ecpecially with small numSlices values
// this sets background to match original image
var bgrnd = img.get(random(width), random(height));
background(bgrnd);
//draw image in new order
for (var i=0; i<numSlices/2; i++) {
image(imgSlices1[i], width/4, lineHeight[i]*2);
image(imgSlices2[i], 3*width/4, lineHeight[i]*2);
image(imgSlices3[i], lineWidth[i], height/2);
image(imgSlices4[i], lineWidth[i]+width/2, height/2);
}
noLoop();
}
Based on what I’ve seen classmates post, I am not sure I did this project correctly. I am really happy with my outcome, though, and it is close to what I visualized before I started writing any code. When I read the prompt for this assignment, I was inspired by I video I had seen of an artist cutting up pictures and then putting them back together in strange ways that added new perspective and dimension. With this in mind, I decided to cut up the image and then layer it on top of itself in new ways. Technically, the image is displayed twice, but not the same way either time. With a quick change of code you can see how the portrait is drawn with more or fewer ‘slices’.
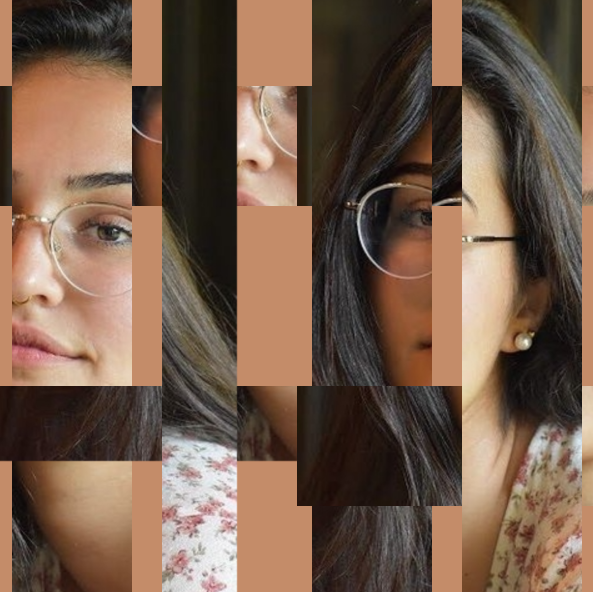
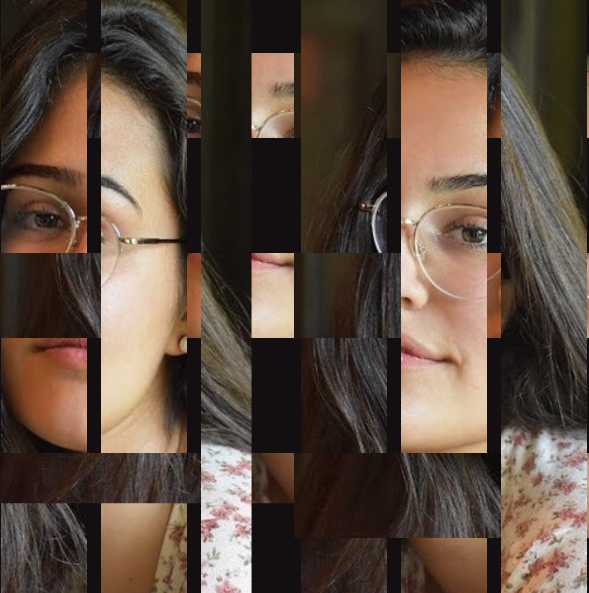
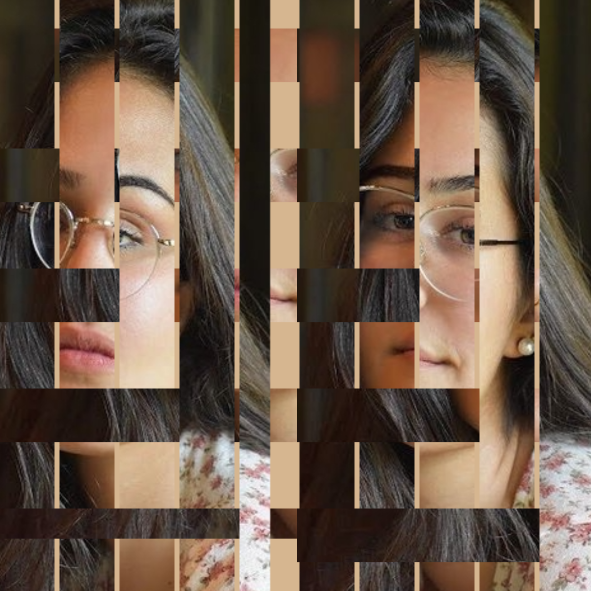
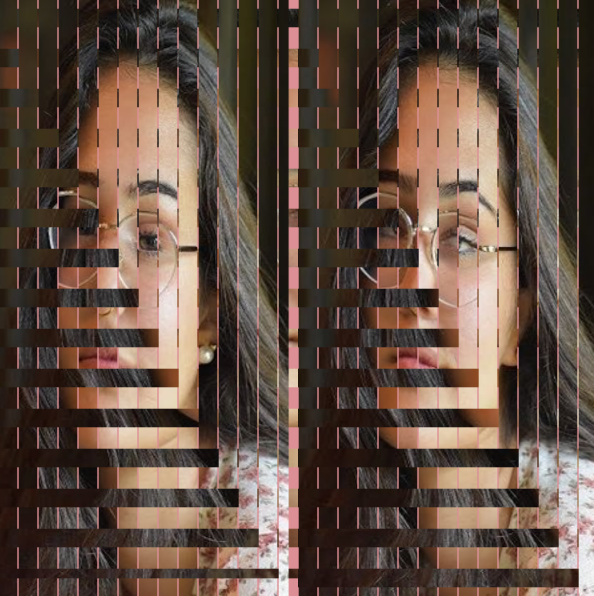