//Michelle Dang
//mtdang
//Section D
var cloud = [];
var water = [];
var offset = 0;
mountain = [];
var inc = 0.01;
var xoff = 0;
function newWater(px, py, pw) {
var p = {x: px, y: py, w: pw,
right: watRight};
return p;
}
// compute the location of the right end of a platform
function watRight() {
return this.x + this.w;
}
function setup() {
createCanvas(480, 300);
// create an initial collection of cloud
for (var i = 0; i < 10; i++){
var rx = random(width);
cloud[i] = makeCloud(rx);
}
for (var i=0; i<10; i++) {
var rx = random(width);
}
var pl = newWater(0, 210, 60); // first platform
water.push(pl);
for (var i=0; i<width/5+1; i++) {
var n = noise(xoff)
var value = map(n, 0, 1, 0, height);
xoff+=inc;
mountain.push(value);
}
frameRate(10);
}
function draw() {
background(220)
//background
noStroke()
displayHorizon();
updateAndDisplaycloud();
removecloudThatHaveSlippedOutOfView();
addNewcloudWithSomeRandomProbability();
//mountainss
beginShape()
fill("blue")
vertex(0, height); //bottom left corner of mountain
for (var i=0; i<width/5; i++) {
vertex(i*6, mountain[i]) //mountain surface
}
vertex(width, height) //bottom right corner of mountain
endShape();
mountain.shift();
mountain.push(map(noise(xoff), 0, 1, 0, 300));
xoff+= inc;
//water and sand
fill(235, 226, 160)
rect(0, 250, width, 200);
fill(98, 147, 204)
rect(0, 200, width, 50);
fill("blue");
noStroke(0);
for (var i = 0; i < water.length; i++) {
var p = water[i];
rect(p.x - offset, p.y, p.w, 10, 10);
}
// if first platform is offscreen to left, remove it
if (water.length > 0 & water[0].right() < offset) {
water.shift();
}
// if last platform is totally within canvas, make a new one
var lastPlat = water[water.length-1];
if (lastPlat.right() - offset < width) {
var p = newWater(10+lastPlat.right(), // start location
random(210, 235), // height of new platform
60); // all water have width 200 for now
water.push(p); // add to our array of water
}
offset += 1;
}
function updateAndDisplaycloud(){
// Update the building's positions, and display them.
for (var i = 0; i < cloud.length; i++){
cloud[i].move();
cloud[i].display();
}
}
function removecloudThatHaveSlippedOutOfView(){
// If a building has dropped off the left edge,
// remove it from the array. This is quite tricky, but
// we've seen something like this before with particles.
// The easy part is scanning the array to find cloud
// to remove. The tricky part is if we remove them
// immediately, we'll alter the array, and our plan to
// step through each item in the array might not work.
// Our solution is to just copy all the cloud
// we want to keep into a new array.
var cloudToKeep = [];
for (var i = 0; i < cloud.length; i++){
if (cloud[i].x + cloud[i].breadth > 0) {
cloudToKeep.push(cloud[i]);
}
}
cloud = cloudToKeep; // remember the surviving cloud
}
function addNewcloudWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newCloudLikelihood = 0.007;
if (random(0,1) < newCloudLikelihood) {
cloud.push(makeCloud(width));
}
}
// method to update position of building every frame
function cloudMove() {
this.x += this.speed;
}
// draw the building and some windows
function cloudDisplay() {
var floorHeight = 5;
var bHeight = 30;
var cHeight = 40;
fill(255);
noStroke()
push();
translate(this.x, height - 40);
rect(0, -bHeight-100, this.breadth, bHeight, 20);
rect(20, -bHeight-100, this.breadth, bHeight+10, 20);
rect(20, -bHeight-200, this.breadth, cHeight, 20);
stroke(200);
pop();
}
function makeCloud(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 60,
speed: -1.0,
nFloors: round(random(2, 7)),
move: cloudMove,
display: cloudDisplay}
return bldg;
}
function displayHorizon(){
stroke(0);
line (0,height-50, width, height-50);
}
Category: Project-11-Landscape
Project 11: Generative Landscape
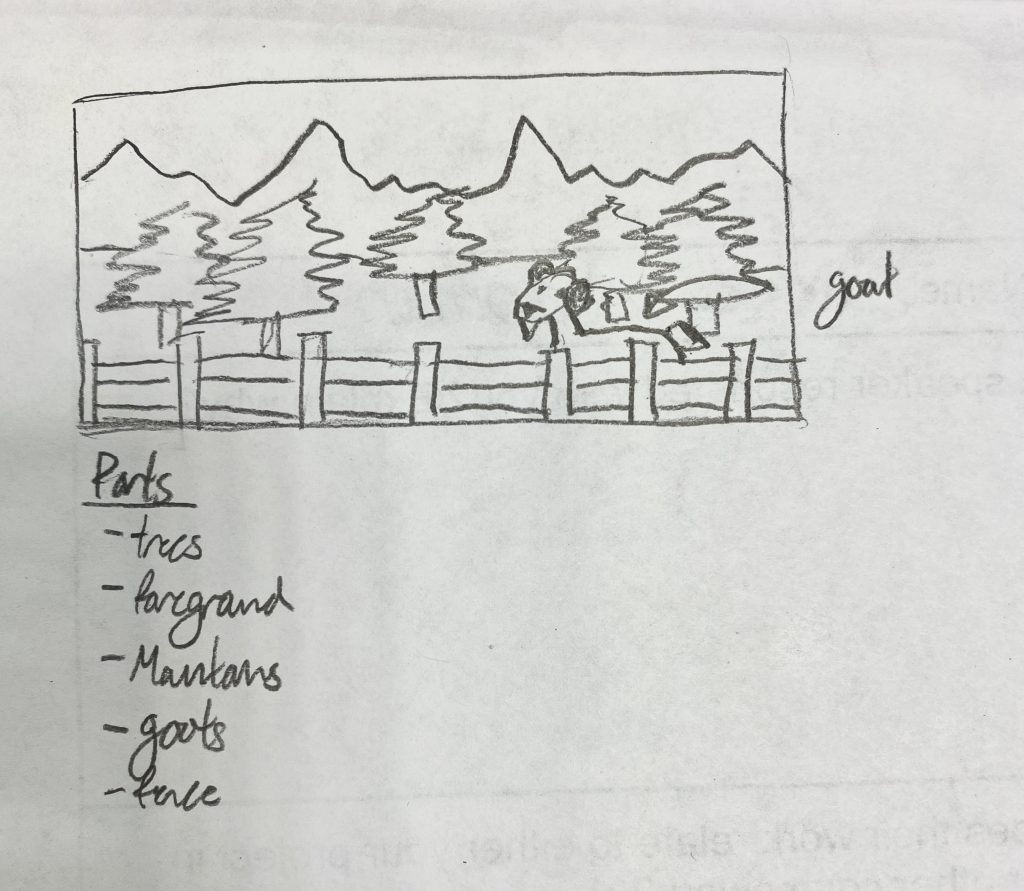
I’ve been missing my grandparents recently, so I knew I wanted to do something that reminds me of them. That means mountains. My grandfather is Austrian and he and my grandmother live in Colorado currently, so I wanted to make the scene feel like I was riding in the car in some mountainous area, complete with rolling hills, pine trees, a nice wooden fence, and a couple goats. I used a noise function for the mountains and hills at different amplitudes. The hill noise function gave me a little trouble when I started implementing my trees, but by lowering the amplitude more and limiting the range for the random tree generation, I was able to mitigate most of the floating trees. Originally, I was thinking about making the goat change color as well, but I couldn’t successfully implement it, so I left it out. Overall, I’m happy with how my landscape came out. I think that if I had more time, there are certainly more tweaks I would make, but with the time that was available to me this week I’m happy with what I made.
//Elise Chapman
//ejchapma
//ejchapma@andrew.cmu.edu
//Section D
var goats = [];
var hill = [];
var mountain = [];
var fence = [];
var trees = [];
var noiseParam = 1; //for the mountains
var noiseStep = 0.05; //for the mountains
var noiseParam2 = 5; //for the hills
var noiseStep2 = 0.005; //for the hills
function preload() {
treeImg = loadImage("https://i.imgur.com/eI7dRxU.png");
fenceImg = loadImage("https://i.imgur.com/8f0PfZu.png");
goatImg = loadImage("https://i.imgur.com/TX6vImS.png");
}
//the mountains
function mountains() {
//sets up the mountains
strokeWeight(3);
stroke(100); //dark gray
fill(100); //dark gray
//creates the mountains
translate(0,0.5);
beginShape();
vertex(0,height);
for (var i=0; i<(width/5)+1; i+=1) {
vertex(i*5,mountain[i]);
}
vertex(width,height);
endShape();
mountain.shift();
var n=noise(noiseParam);
var value=map(n,0,1,0,height);
mountain.push(value);
noiseParam+=noiseStep;
}
//the hills
function hills() {
//sets up the hills
strokeWeight(3);
stroke(27,81,45); //dark green
fill(27,81,45); //dark green
//creates the hills
translate(0,10);
beginShape();
vertex(0,height);
for (var i=0; i<(width/5)+1; i+=1) {
vertex(i*5,hill[i]);
}
vertex(width,height);
endShape();
hill.shift();
var n=noise(noiseParam2);
var value=map(n,0,1,0,height);
hill.push(value);
noiseParam2+=noiseStep2;
}
//the goats
function makeGoat(gx) {
var g = {x: gx,
goatSpeed: -3.0,
goatSizeMod: random(0,51),
move: goatMove,
display: goatDisplay}
return g;
}
function goatDisplay() {
image(goatImg, this.x+200,90-this.goatSizeMod, 100+this.goatSizeMod,100+this.goatSizeMod);
}
function goatMove() {
this.x += this.goatSpeed;
}
function updateGoats() {
//update the goat positions and display them
for (var i=0; i<goats.length; i+=1) {
goats[i].move();
goats[i].display();
}
}
function removeGoats() {
//remove goat when no longer on screen
var goatOnScreen = [];
for (var i=0; i<goats.length; i+=1) {
if (goats[i].x + 400 > 0) {
goatOnScreen.push(goats[i]);
}
}
goats = goatOnScreen;
}
function addNewGoats() {
var newGoatChance = 0.004;
if (random(0,1) < newGoatChance) {
goats.push(makeGoat(width));
}
}
//the trees
function makeTree(tx) {
var t = {x: tx,
y: 90-random(0,50),
treeSpeed: -5.0,
treeSizeMod: random(0,51),
move: treeMove,
display: treeDisplay}
return t;
}
function treeDisplay() {
image(treeImg, this.x+200, this.y, 50+this.treeSizeMod,75+this.treeSizeMod);
}
function treeMove() {
this.x += this.treeSpeed;
}
function updateTrees() {
//update the tree positions and display them
for (var i=0; i<trees.length; i+=1) {
trees[i].move();
trees[i].display();
}
}
function removeTrees() {
//remove tree when no longer on screen
var treesOnScreen = [];
for (var i=0; i<trees.length; i+=1) {
if (trees[i].x + 400 > 0) {
treesOnScreen.push(trees[i]);
}
}
trees = treesOnScreen;
}
function addNewTrees() {
var newTreeChance = 0.1;
if (random(0,1) < newTreeChance) {
trees.push(makeTree(width));
}
}
function setup() {
createCanvas(480,200);
frameRate(25);
//for the mountains
for (var i=0; i<(width/5)+1; i+=1) {
var n=noise(noiseParam);
var value=map(n,0,1,0,height);
mountain[i]=value;
noiseParam+=noiseStep;
}
//for the mountains
for (var i=0; i<(width/5)+1; i+=1) {
var n=noise(noiseParam2);
var value=map(n,0,1,0,height);
hill[i]=value;
noiseParam2+=noiseStep2;
}
//inital collection of trees
for (var i=0; i<10; i+=1) {
var rx = random(width);
trees[i]=makeTree(rx);
}
}
function draw() {
background(123, 196, 227); //sky blue
//sun
noStroke();
fill(255, 255, 227); //very light yellow
ellipse(40,30,25);
mountains();
hills();
//trees
updateTrees();
removeTrees();
addNewTrees();
//goats
updateGoats();
removeGoats();
addNewGoats();
//fence
var fenceX=0;
for (var i=0; i<5; i+=1) {
image(fenceImg, fenceX+(i*200),115, 200,100);
}
}
Project 11 – Moving Landscape
Trees and birds and trees and birds
var trees = [];
var birds = [];
var dx = -1;
function setup() {
createCanvas(400, 200);
for (var i = 0; i < 10; i++){ //populate trees
var rx = random(width);
trees[i] = makeTree(rx);
}for (var i = 0; i < 3; i++){ //populate birds
var bx = random(width);
birds[i] = makeTree(bx);
}
frameRate(10);
}
function draw() {
background('lightblue');
//dirt
noStroke();
fill('darkgreen');
rect(-10, 100, width+20, height);
updateAndDisplayTrees();
removeTree();
newTree();
updateAndDisplayBird();
removeBird();
newBird();
drawRoad();
}
function drawRoad() {
fill('grey');
rect(-10, 130, width+20, 30);
stroke('black');
var dividerLines = [];
for (var i = 0; i < 30; i++) { //make vals
var a = i*width/30;
dividerLines.push(a);
} for (var i = 0; i < 30; i++) { //draw lines
line(dividerLines[i], 145, dividerLines[i]+width/60, 145);
} for (var i = 0; i < 30; i++) { //move lines
dividerLines[i] += dx;
if (dividerLines[i] <= -width/60) {
dividerLines[i] = width;
}
}
}
function updateAndDisplayTrees(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
function updateAndDisplayBird(){
for (var i = 0; i < birds.length; i++){
birds[i].move();
birds[i].display();
}
}
function removeTree(){
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x > -50) {
treesToKeep.push(trees[i]);
}
}
trees = treesToKeep;
}
function removeBird(){
var birdsToKeep = [];
for (var i = 0; i < birds.length; i++){
if (birds[i].x > -50) {
birdsToKeep.push(birds[i]);
}
}
birds = birdsToKeep;
}
function newTree() {
var newTreeLikelihood = 0.02;
if (random(0,1) < newTreeLikelihood) {
trees.push(makeTree(width+50));
}
}
function newBird() {
var newBirdLikelihood = 0.05;
if (random(0,1) < newBirdLikelihood) {
birds.push(makeBird(width));
}
}
// method to update position of Tree every frame
function treeMove() {
this.x += this.speed * this.scal/3;
}
function birdMove() {
this.x += this.speed;
}
function treeDisplay() {
push();
translate(this.x, 100);
scale(this.scal)
stroke('brown');
strokeWeight(5);
line(0, 0, 0, 20);
stroke('green');
fill('green');
for (var i = 0; i < 5; i++) {
triangle(-20+2*i, -7*i, -2, -7*i, -2, -20-7*i);
} for (var i = 0; i < 5; i++) {
triangle(20-2*i, -7*i, 2, -7*i, 2, -20-7*i);
}
pop();
}
function birdDisplay() {
push();
translate(this.x, this.y);
stroke('black');
noFill();
strokeWeight(2);
arc(0, 0, 20, 20, PI+PI/3, 2*PI-PI/3);
arc(10, 0, 20, 20, PI+PI/3, 2*PI-PI/3);
pop();
}
function makeTree(birthLocationX) {
var tree = {x: birthLocationX,
scal: random(0.5, 2),
speed: dx,
move: treeMove,
display: treeDisplay}
return tree;
}
function makeBird(birthLocationX) {
var bird = {x: birthLocationX,
y: random(0, 70),
speed: random(-5,-2),
move: birdMove,
display: birdDisplay}
return bird;
}
Project 11: Generative Landscape
//global variables
var cloudsX = [];
var cloudsY = [];
var brickX = [];
var brickY = [];
var mushroomX = [];
var mushroomY = [];
var cloudImg;
var brickImg;
var floorImg;
var noiseParam = 0;
var noiseStep = 0.01;
var numClouds = 5;
var numBricks = 3;
var numMushrooms = 3;
var mh = []; //height of the mountains
function preload() {
//preload images
cloudImg = loadImage("https://i.imgur.com/i3pLDv0.png");
brickImg = loadImage("https://i.imgur.com/yNNs74U.png");
floorImg = loadImage("https://i.imgur.com/LZwSMdA.png");
mushroomImg = loadImage("https://i.imgur.com/tflvlXK.png");
}
function setup() {
createCanvas(480, 400);
//mountain
for (var i = 0; i < width/2 + 1; i ++) {
var n = noise(noiseParam);
var v = map(n, 0, 1, height/2, height);
mh.push(v);
noiseParam += noiseStep;
}
//cloud
for (var i = 0; i < numClouds; i ++) {
var cx = random(width/numClouds*i, width/numClouds*(i+1));
var cy = random(height/4);
cloudsX.push(cx);
cloudsY.push(cy);
}
//brick
for (var i = 0; i < numBricks; i ++) {
var bx = random(width/numBricks*i, width/numBricks*(i+1));
var by = random(height/3, height/2);
brickX.push(bx);
brickY.push(by);
}
//mushroom
for (var i = 0; i < numMushrooms; i ++) {
var mx = random(width/numMushrooms*i, width/numMushrooms*(i+1));
var my = height-90;
mushroomX.push(mx);
mushroomY.push(my);
}
frameRate(10);
}
function draw() {
background(102, 154, 255); //blue
drawMountain();
drawCloud();
drawBrick();
drawMushroom();
//floor
image(floorImg, 0, 340, 130, 30);
image(floorImg, 129, 340, 130, 30);
image(floorImg, 258, 340, 130, 30);
image(floorImg, 387, 340, 130, 30);
image(floorImg, 0, 370, 130, 30);
image(floorImg, 129, 370, 130, 30);
image(floorImg, 258, 370, 130, 30);
image(floorImg, 387, 370, 130, 30);
}
//mountains
function drawMountain() {
fill(52, 151, 9); //green
beginShape();
vertex(0, height);
for (var i = 0; i < mh.length; i ++) {
x = 2*i;
y = mh[i];
vertex(x,y);
}
vertex(width, height);
endShape();
mh.shift();
n = noise(noiseParam);
v = map(n, 0, 1, height/2, height);
mh.push(v);
noiseParam += noiseStep;
}
//cloud
function drawCloud() {
for (var i = 0; i < numClouds; i ++) {
image(cloudImg, cloudsX[i], cloudsY[i], 100, 33);
}
for (var i = 0; i < numClouds; i ++) {
cloudsX[i] -= 3; //update cloud position
if (cloudsX[i] < -100) { //remove cloud
cloudsX[i] = width;
cloudsY[i] = random(height/4);
}
}
}
//brick
function drawBrick() {
for (var i = 0; i < numBricks; i ++) {
image(brickImg, brickX[i], brickY[i], 100, 30);
}
for (var i = 0; i < numBricks; i ++) {
brickX[i] -= 3; //update brick position
if (brickX[i] < -100) { //remove cloud
brickX[i] = width;
brickY[i] = random(height/3, height/2);
}
}
}
//mushroom
function drawMushroom() {
for (var i = 0; i < numMushrooms; i ++) {
image(mushroomImg, mushroomX[i], mushroomY[i], 40, 30);
}
for (var i = 0; i < numMushrooms; i ++) {
mushroomX[i] -= 7; //update brick position
if (mushroomX[i] < -100) { //remove cloud
mushroomX[i] = width;
mushroomY[i] = height-90;
}
}
}
For this project, I decided to replicate one of my favorite childhood game, Super Mario Bros Game we used to play with Nintendo. For moving variables, I added clouds, bricks, mushrooms and the mountain the background, and included floor tiles using png files. It was challenging at first to make them move and disappear, but it was really fun to create this game-like scenery. If I had more time, I would like to add a jumping Mario so the character interacts with the game scene.
Project 11
// gnmarino
// gia marino
// section D
// sushi conveyor belt animation
var sushis = [];
var nigiris = [];
function setup() {
createCanvas(480, 320);
}
function draw() {
background(220);
// moves sushis and nigiris
moveDisplayOfSushiAndNigiri();
// removes them if they go off the screen
removeOldSushiAndNigiri();
// adds new sushis and nigiris based off a low probability
addSushi_or_Nigiri();
// makes conveyour belt for sushi and nigiri to move on
conveyorBelt();
}
function removeOldSushiAndNigiri() {
var freshSushi = [];
var freshNigiri = [];
// if sushis are on the screen then put them in fresh sushi array
// if they are not on the screen then they will disappear because
// they won't be added to the new array
for (var i = 0; i < sushis.length; i++){
if (sushis[i].x + sushis[i].sushiWidth > 0) {
freshSushi.push(sushis[i]);
}
}
// same with nigiris
for (var i = 0; i < nigiris.length; i++){
if (nigiris[i].x + nigiris[i].nigiriWidth > 0) {
freshNigiri.push(nigiris[i]);
}
}
sushis = freshSushi;
nigiris = freshNigiri;
}
function moveDisplayOfSushiAndNigiri(){
for(var i = 0; i < sushis.length; i ++){
sushis[i].move();
sushis[i].display();
}
for(var i = 0; i < nigiris.length; i ++) {
nigiris[i].move();
nigiris[i].display();
}
}
function addSushi_or_Nigiri() {
// this is the probability of being added everytime code loops
var addSushiLikelihood = .005;
var addNigiriLikelihood = .004;
if(random(0, 1) < addSushiLikelihood) {
sushis.push(makeSushi(width));
}
if(random(0, 1) < addNigiriLikelihood) {
nigiris.push(makeNigiri(width +10));
}
}
function displayNigiri() {
push();
strokeWeight(2);
// these variables are used to make it easier to put the shapes together
var nigiriTop = 180-this.nigiriHeight // conveyor belt is at 180
var nigiriMiddle = this.nigiriWidth/2 + this.x
fill(247, 246, 241); //off-white
rect(this.x, nigiriTop, this.nigiriWidth, this.nigiriHeight);
fill(this.sashimiColor);
ellipse(nigiriMiddle, nigiriTop, this.nigiriWidth + 15, 40);
// this is to cover last ellipse so it looks more like shashimi
fill(247, 246, 241); //off-white
ellipse(nigiriMiddle, nigiriTop + 10, this.nigiriWidth, 25);
noStroke();
rect(this.x + 1, nigiriTop + 10, this.nigiriWidth-2, 15);
pop();
}
function displaySushi() {
// these variables are used to make it easier to put the shapes together
var sushiMiddle = this.sushiWidth/2 + this.x
var sushiTop = 180-this.sushiHeight // conveyor belt is at 180
push();
strokeWeight(2);
fill(this.wrapColor);
rect(this.x, sushiTop, this.sushiWidth, this.sushiHeight);
fill(247, 246, 241); //off-white
ellipse(sushiMiddle, sushiTop, this.sushiWidth, 25);
fill(this.fishColor);
ellipse(sushiMiddle, sushiTop, this.sushiWidth-20, 15);
pop();
}
function move_sushi_nigiri() {
this.x += this.speed;
}
// sushi object
function makeSushi(originX) {
var sushi = {x: originX,
sushiWidth: random(55, 100),
sushiHeight: random(35, 70),
wrapColor: color(0, random(100) , 0),
fishColor:
color(random(230, 260), random(145, 225), random(70, 160)),
speed: -3,
move: move_sushi_nigiri,
display: displaySushi}
return sushi;
}
// nigiri object
function makeNigiri(originX) {
var nigiri = {x: originX, // don't know if i need to change that
nigiriWidth: random( 70, 110),
nigiriHeight: random( 15, 40),
sashimiColor:
color(random(230, 260), random(145, 225), random(70, 160)),
speed: -3,
move: move_sushi_nigiri,
display: displayNigiri}
return nigiri;
}
function conveyorBelt() {
push();
fill(70);
rect(40, 185, 440, 150)
fill(180);
strokeWeight(5);
ellipse(30, 260, 150);
ellipse(450, 260, 150);
strokeWeight(12);
line(30, 335, 450, 335);
line(30, 185, 450, 185);
arc(30, 260, 150, 150, radians(90), radians(270), OPEN);
arc(450, 260, 150, 150, radians(-90), radians(90), OPEN);
pop();
}
Project 11: Generative Landscape
var sushi=["https://i.imgur.com/77xfiZT.png", "https://i.imgur.com/LEndRAV.png", "https://i.imgur.com/d1fWjnJ.png", "https://i.imgur.com/UyTqQin.png"];
let belt;
var sushi1;
var sushi2;
var sushi3;
var sushi4;
//var x = 80;
var speed= 7;
var plate=[];
var inChopstick=false;
var nSushi=200;
var x=0;
var speed=7;
function preload() {
belt = loadImage("https://i.imgur.com/zBwKyLa.png")
sushi1=loadImage(sushi[0]);
sushi2=loadImage(sushi[1]);
sushi3=loadImage(sushi[2]);
sushi4=loadImage(sushi[3]);
}
function setup() {
createCanvas(500, 400);
var n = 0;
for (var i = 0; i < 1000; i++){ //continually creates (1000) plates of sushi
plate[i] = makePlate(n);
n -= 200;
}
frameRate(30);
}
function draw() {
background(0);
image(belt,0,0);
plateShow();
}
function plateGo(){
this.x+=this.dx;
}
function plateShow(){
for(var i = 0; i < plate.length; i++){
plate[i].go();
plate[i].show();
// plate[i].mP();
}
}
function makePlate(px){
var plate={x: px, y:180, w:150, h:100,
dx: 7, go:plateGo, show:drawPlate,
sushi: random([sushi1, sushi2, sushi3, sushi4]),
}
return plate;
}
function drawPlate(){
push();
fill(215);
noStroke();
ellipse(this.x, this.y, this.w, this.h);
stroke(185);
noFill();
ellipse(this.x, this.y, 0.75*this.w, 0.75*this.h);
if (this.sushi==sushi1){
image(sushi1, this.x-50, 0);
}
if (this.sushi==sushi2){
image(sushi2, this.x-50, 0);
}
if (this.sushi==sushi3){
image(sushi3, this.x-50, 0);
}
if (this.sushi==sushi4){
image(sushi4, this.x-50, 0);
}
}
Project Landscape
//Yanina Shavialenka
//Section B
/*
The following project was written with an intention to represent world
peace, love, diversity, and care for the planet. I used world map to
represent the world, people in different national outfits to represent
people from around the world, love symbold to represent love, rainbow
peace sign to represent peace in the world and inclusion, recycling
symbol to represent the fact that we need to recycle and save our planet.
*/
//Initial arrays for images and objects
var people = [];
var world = [];
var symbol = [];
var loadPeople = [];
var loadSymbol = [];
var worldmap;
//Value of change of movement
var dx1 = 1.5;
//Preloads images
function preload() {
var file = [];
file[0] = "https://i.imgur.com/PAWmbXw.png";
file[1] = "https://i.imgur.com/t0fgBq2.png";
file[2] = "https://i.imgur.com/SkGPugf.png";
file[3] = "https://i.imgur.com/dbMZm7H.png";
file[4] = "https://i.imgur.com/L1ODD20.png";
file[5] = "https://i.imgur.com/b5hpqxP.png";
for(var i = 0 ; i < file.length; i++) {
people[i] = loadImage(file[i]);
}
var file1 = [];
file1[0] = "https://i.imgur.com/IRxGbDw.png";
file1[1] = "https://i.imgur.com/Xkq7Q0x.png";
file1[2] = "https://i.imgur.com/w9crIqx.png";
for(var i = 0 ; i < file1.length; i++) {
symbol[i] = loadImage(file1[i]);
}
worldmap = loadImage("https://i.imgur.com/LhjqpdM.png");
world.push(makeBackground(0,0,dx1));
}
function setup() {
createCanvas(480, 480);
}
//Object functions that create object
//Below function has an object for background
function makeBackground(cx,cy,cdx) {
var c = { x: cx, y: cy, dx: cdx,
stepFunction: stepBackground,
drawFunction: drawBackground
}
return c;
}
//Below function has an object for characters
function makeCharacter(cx, cdx, num) {
var r = random(320);
var c = { x: cx, y: r, dx: cdx,
imageNum: num,
stepFun: stepCharacter,
drawFun: drawCharacter
}
return c;
}
//Below function has an object for symbols
function makeSymbol(cx, cdx, num) {
var r = random(320);
var c = { x: cx, y: r, dx: cdx,
imageNum: num,
step: stepSymbol,
draw: drawSymbol
}
return c;
}
//Object functions that move objects
function stepBackground() {
this.x -= this.dx;
}
function stepCharacter() {
this.x -= this.dx;
}
function stepSymbol() {
this.x -= this.dx;
}
//Object functions that draw objects
function drawBackground() {
image(worldmap,this.x,this.y);
}
function drawCharacter() {
image(people[this.imageNum],this.x,this.y);
}
function drawSymbol() {
image(symbol[this.imageNum],this.x,this.y);
}
function draw() {
background(220);
//random values
var num = random(people.length - 2);
num = int(num);
var num1 = random(symbol.length);
num1 = int(num1);
var peopleCount = random(100,200);
peopleCount = int(peopleCount);
var symbolCount = random(300);
symbolCount = int(symbolCount);
//moves and draws map
for(var i = 0 ; i < world.length ; i++) {
world[i].stepFunction();
world[i].drawFunction();
}
//when the latest world x postion is 0, a new map is drawn at x coordinate of the world map width
if(world[world.length-1].x <= 0) {
world.push(makeBackground(802,0,dx1));
}
//new position for people and symbols
if(frameCount % peopleCount == 0) {
loadPeople.push(makeCharacter(480,dx1,num));
}
if(frameCount % symbolCount == 0) {
loadSymbol.push(makeSymbol(480,dx1,num1));
}
//moves and draws people and symbols
for(var i = 0 ; i < loadPeople.length ; i++) {
loadPeople[i].stepFun();
loadPeople[i].drawFun();
}
for(var i = 0 ; i < loadSymbol.length ; i++){
loadSymbol[i].step();
loadSymbol[i].draw();
}
}
For this week’s project, I decided to portray the idea of utopia where all people around the world live in peace, love, and inclusion. I used the background of a world map to represent the world; people in different national outfits to represent the people around the world; love symbol to represent love; rainbow peace sign to represent peace and inclusion; recycling symbol to represent the idea that we need to recycle to save the earth and have that ideal utopia.
In my project, I encountered a problem with a background since I used an image instead of drawing it out myself: when the image would end, it would not reappear so I came with an idea to use a double image “glued” together which eventually did the trick. Overall I really enjoyed this assignment and found it really fun to work on!
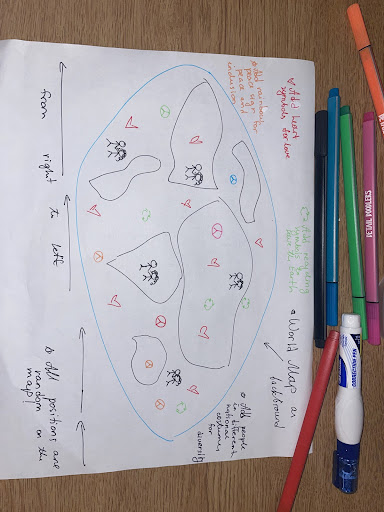
Project 11: Generative Landscape
//Julianna Bolivar
//jbolivar@andrew.cmu.edu
//Section D
//Generative Landscape
var hills = []; //hills array
var trees = [];
var treesShowing = [];
var clouds = [];
var cloudsShowing = [];
var counter = 0;
var cloudCounter = 0;
var noiseParam = 0;
var noiseStep = 0.002;
function setup() {
createCanvas(480, 300);
//trees
for (var i = 0; i < 20;i++){
var trs = random(width);
trees[i] = makeTrees(trs);
}
//hills
for (var i=0; i<width/2+1; i++){
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height);
noiseParam += noiseStep;
hills.push(value);
}
for (var i = 0; i < 5; i ++) {
var cloudX = random(width);
var cloudY = random(0, 40);
cloudsShowing[i] = makeClouds(cloudX, cloudY);
}
frameRate(20);
}
function draw() {
background(165,182,182);
noStroke();
//sun
fill(204,102,0);
circle(40,50,30);
//hills fill shape
beginShape();
fill(56,87,33);
vertex(0, height);
for (var i=0; i<width/2; i+=1){
vertex(i*5, hills[i]);
}
vertex(width, height);
endShape();
hills.shift();
hills.push(map(noise(noiseParam), 0, 1, 0, height));
noiseParam += noiseStep; //move sideways
updateAndDrawClouds();
removeCloudsOffScreen();
addNewClouds();
updateandDrawTrees();
removeTreesOffScreen();
addNewTrees();
}
function updateandDrawTrees(){
for(var i=0; i < treesShowing.length; i++){
treesShowing[i].move();
treesShowing[i].draw();
}
}
function removeTreesOffScreen(){
var treesToKeep = [];
for (var i = 0; i < treesShowing.length; i++){
if (treesShowing[i].x+20 > 0) {
treesToKeep.push(treesShowing[i]);
}
}
treesShowing = treesToKeep; // remember showing trees
}
function addNewTrees(){
counter+=1;
if (counter % 25 == 0){
treesShowing.push(makeTrees(width, random(150,300)));
}
}
function makeTrees(tx, ty){
var trees = {x:tx, y:ty,
width:random(10, 20),
height:random(50, 90),
r:random(75,200), g:random(75,100), b: 0,
speed: -1.0,
move: treesMove,
draw: drawTrees }
return trees;
}
//draw trees
function drawTrees(){
fill(160,82,45);
rect(this.x, this.y, this.width, this.height);
fill(this.r, this.g, this.b);
circle(this.x-5, this.y-10, 50);
circle(this.x+20, this.y-10, 50);
circle(this.x+5, this.y-20, 50);
}
function treesMove(){
this.x += this.speed;
}
function updateAndDrawClouds(){
for (var i = 0; i < cloudsShowing.length; i++) {
cloudsShowing[i].move();
cloudsShowing[i].draw();
}
}
function removeCloudsOffScreen(){
var cloudsToKeep = [];
for (var i = 0; i < cloudsShowing.length; i++){
if (cloudsShowing[i].cloudX + 10 > 0) {
cloudsToKeep.push(cloudsShowing[i]);
}
}
cloudsShowing = cloudsToKeep;
}
function addNewClouds(){
cloudCounter+=1;
if (cloudCounter % 100 == 0){
cloudsShowing.push(makeClouds(width, random(0,60)));
}
}
function makeClouds(cx, cy){
var c = {cloudX: cx,
cloudY: cy,
cloudSpeed: -1,
move: cloudMove,
draw: cloudDraw}
return c;
}
function cloudMove(){
this.cloudX += this.cloudSpeed;
}
function cloudDraw(){
fill(165);
noStroke();
ellipse(this.cloudX, this.cloudY - 5, 60, 50);
ellipse(this.cloudX - 20, this.cloudY + 10, 60, 50);
ellipse(this.cloudX + 15, this.cloudY - 5, 70, 50);
ellipse(this.cloudX + 5, this.cloudY + 20, 60, 50);
ellipse(this.cloudX + 30, this.cloudY + 10, 80, 50);
}
My landscape is inspired by the autumn. The view from the design studio is very scenic. I think I am most proud of this deliverable. I’ve struggled to make beautiful projects, but I’m pretty satisfied with this one.
project-11
this project was pretty interesting. there are birds, clouds, and a landscape going across the screen.
var cloud = []
var bird = []
function setup() {
createCanvas(480, 240)
frameRate(10)
for (i = 0; i < 30; i++) {
birdX = random(width)
birdY = random(height)
bird[i] = makeBird(birdX, birdY)
}
for (i = 0; i < 15; i++) {
cloudX = random(width)
cloudY = random(height/1.5)
cloud[i] = makeCloud(cloudX, cloudY)
}
}
function draw() {
background(140, 200, 255)
strokeWeight(2)
landscape()
showCloud()
showBird()
}
function landscape() {
stroke(86, 125, 70)
beginShape()
for (var i = 0; i < width; i++) {
var x = (i * .01) + (millis() * .0004)
var s = map(noise(x), 0, 1, 150, 200)
line(i, s, i, height)
}
endShape()
}
function makeBird(birdX, birdY) {
var bird = {
fx: birdX,
fy: birdY,
birdspeed: random(-3, -8),
birdmove: moveBird,
birdcolor: color(random(100, 200), random(100, 200), random(100, 200)),
birddraw: drawBird
}
return bird
}
function moveBird() {
this.fx += this.birdspeed
if (this.fx <= -10) {
this.fx += width
}
}
function drawBird() {
stroke(0)
fill(this.birdcolor);
ellipse(this.fx, this.fy, 10, 10)
line(this.fx - 5, this.fy, this.fx - 10, this.fy - 2)
line(this.fx + 5, this.fy, this.fx + 10, this.fy - 2)
}
function showBird() {
for (i = 0; i < bird.length; i++) {
bird[i].birdmove()
bird[i].birddraw()
}
}
function makeCloud(cloudX, cloudY) {
var cloud = {
fx: cloudX,
fy: cloudY,
cloudspeed: random(-1, -2),
cloudmove: moveCloud,
clouddraw: drawCloud
}
return cloud
}
function moveCloud() {
this.fx += this.cloudspeed
if (this.fx <= -10) {
this.fx += width
}
}
function drawCloud() {
noStroke()
fill(255);
ellipse(this.fx, this.fy, 10, 10)
ellipse(this.fx-4, this.fy-1, 10, 10)
}
function showCloud() {
for (i = 0; i < cloud.length; i++) {
cloud[i].cloudmove()
cloud[i].clouddraw()
}
}
Project: Generative Landscape
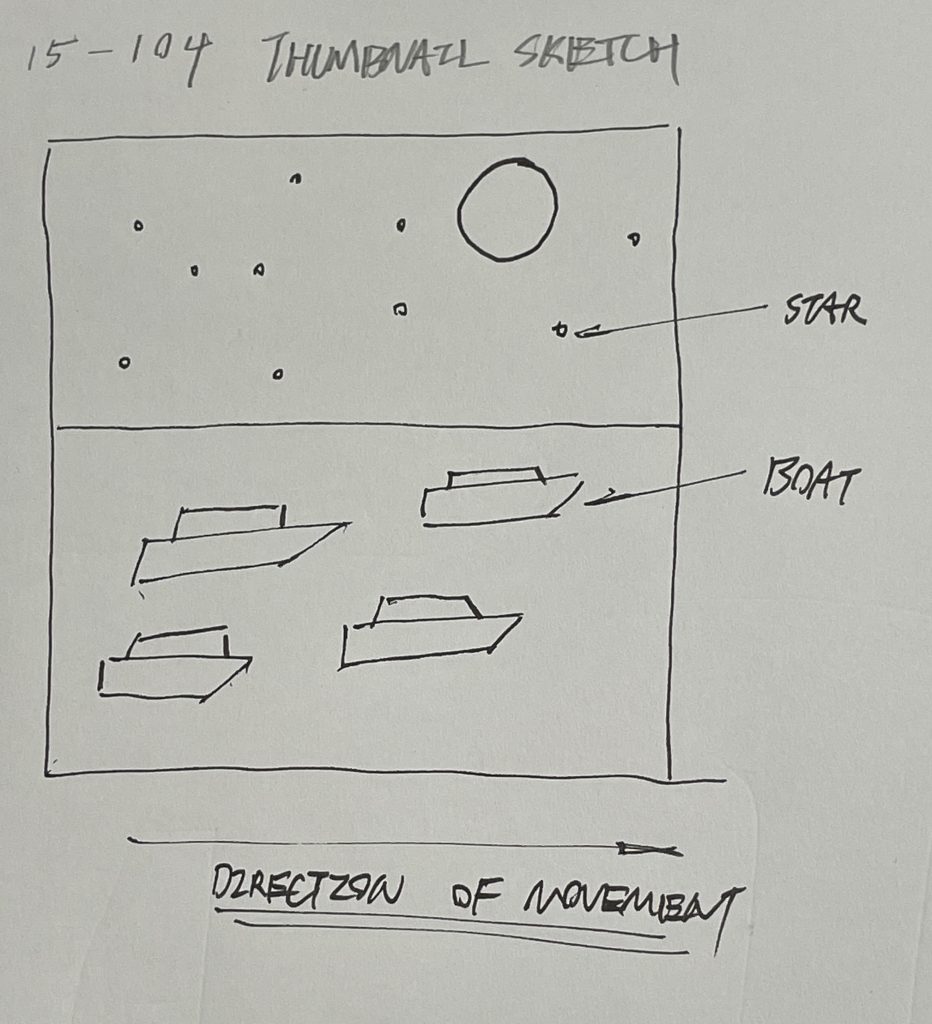
//lucacao
//sectionD
//Project11
var boat = [];//array to store boat number
var speed = 1;
function setup() {
createCanvas(480, 480);
noStroke();
frameRate(5);
for (var i = 0; i < 10;i++){
bx = random(width);//boat x position
}
}
function draw() {
background(220);
sea();
sky();
moon();
for(var i = 0; i < 5; i++){
drawBoat();
boat++;
print(boat);
}
}
function sea() {
fill(0, 64, 108);
rect(0,240,480,240);
}
function sky(){
fill(0, 37, 62);
rect(0,0,480,240);
for (var i = 0; i < 100;i++){
var starx = random(0,480);
var stary = random(0,240);
fill(255,255,255);
ellipse(starx,stary,3,3);
starx += speed;
}
// noLoop();
}
function moon(){
fill(252, 202, 70);
ellipse(350,80,100,100);
}
function drawBoat(){
var blength = random(50,100);
var bheight = random(20,30);
var bx = random(0,480);
var by = random(240,480);
this.bx += this.speed;
fill(0);
rect(bx,by,blength,bheight);
rect(bx+20,by-10,blength/2,bheight/2);
triangle(bx+blength,by+bheight,bx+blength,by,
bx+blength+30,by-10);
}
For my landscape, I wanted to depict a scene with boats and stars moving at night. In my thumbnail sketch, I divided the canvas into halves, with a sky which is the top half, and the sea at the bottom half. I thought it would be more visually interesting with objects moving on all areas of the canvas. During this project, I faced difficulty trying to animate my objects, but I solved the problem by creating multiple functions which helped me clarify my code structure and tackle specific problems. The randomness in my code exists in the size and position of the boat.