// Jiyeon Chun
// Section C
// jiyeonch@andrew.cmu.edu
// Project-06-A
var glowsize = 200; //sec
var waxDrip; //min
var candleHeight = 180; //hour
function setup() {
createCanvas(300, 480);
}
function draw() {
background(136, 39, 39);
//get current time
var H = hour();
var M = minute();
var S = second();
//second to glow toggle
if (S%2 == 0) {
glowSize = 180;
} else {
glowSize = 200;
}
//hour to candle height
candleHeight = 180+(H*5);
//minute to wax drip
waxDrip = candleHeight+30+M;
//glow
noStroke();
fill(255, 176, 0,50);
ellipse(150,candleHeight-40,glowSize);
//candle
noStroke();
fill(198, 187, 141);
rect(120,candleHeight,60,220);
//background
noStroke();
fill(136, 39, 39);
rect(0,400,300,220);
//dish
noStroke();
fill(151, 127, 33);
quad(80,390,100,430,200,430,220,390);
//dish handle
strokeWeight(8);
stroke(151, 127, 33);
fill(136, 39, 39);
ellipse(232,400,40);
//flame
noStroke();
fill(255, 176, 0);
ellipse(150,candleHeight-40,40,50);
triangle(131,candleHeight-48,150,candleHeight-95,169,candleHeight-48);
//wax
strokeWeight(8);
stroke(237, 229, 195);
line(120,candleHeight,180,candleHeight);
line(158,candleHeight,158,waxDrip); //longone
line(170,candleHeight,170,waxDrip-20); //shortone
//bible verse
fill(241, 240, 217);
noStroke();
textSize(12);
textAlign(CENTER);
text("MATTHEW 25:13",246,470);
}
Category: Project-06-Abstract-Clock
Project 6: Abstract Clock
Going into this project, I first started thinking about my favorite ways to physically tell time. How does my body determine what time it is? I eventually settled on the changing daylight. From there, I decided that I both wanted to create a landscape and didn’t want to directly show the sun or the moon (that feels overdone). In the end, I like the effect that I got with all the different elements in my desert landscape. I think the bird could be a little more accurate to a buzzard and I think it would have been cool if the tumbleweed could have rotated, but I just didn’t have time to complete either of those. Perhaps I’ll go back into my code later and fix them to be the best version I can get them.
//Elise Chapman
//ejchapma
//ejchapma@andrew.cmu.edu
//Section D
function setup() {
createCanvas(480,300);
rectMode(CENTER);
}
function draw() {
background(200); //background is white
//draws a buzzard that flies across the screen every minute
noStroke();
var dx=430/60;
var xPos=dx*second(); //x position of the buzzard
var yPos=50; //y position of the buzzard
fill(75);
ellipse(xPos,yPos,25,15);
ellipse(xPos+15,yPos,15);
triangle(xPos+20,yPos-5,xPos+20,yPos+5,xPos+30,yPos);
//the buzzard will move its wings every second
if (second()%2==0) {
triangle(xPos-10,yPos-3,xPos+10,yPos-3,xPos,yPos-20);
} else {
triangle(xPos-10,yPos+3,xPos+10,yPos+3,xPos,yPos+20);
}
landscape(0,0);
tumbleweed(0,0);
sky(0,0);
}
//draws the tumbleweed, that will eventually move
function weed(x,y) {
push();
translate(x,y);
stroke(241,208,160); //beige
strokeWeight(5);
line(-15,-20,25,10);
line(-15,20,20,-15);
line(-25,0,25,-10);
line(0,-25,0,25);
line(-20,10,20,15);
line(10,20,15,-20);
line(-10,20,-15,-20);
line(-20,-10,5,-15);
pop();
}
//makes the weed tumble, moving every minute
function tumbleweed(x,y) {
var xPos=0; //x position of tumbleweed
var yPos=(height/3)+140; //y position of tumbleweed
var dx=width/60; //how much the tumbleweed moves by
weed(xPos+(dx * minute()),yPos);
}
//draws the main background
function landscape(x,y) {
push();
translate(width/2,height/2);
noStroke();
//background plateau
fill(249,160,63); //orange sand color
rect(width/4,height/4,100,height,30,30);
rect(width/4+50,height/3,50,height,30,30);
rect(width/4+100,height/2.25,100,height);
rect(width/4-25,height/2.5,100,height,30,30);
rect(width/4-75,height/1.75,100,height,30,30);
//even further background plateu
fill(255,193,100); //lightened orange sand color
rect(-width/4-50,height/3,75,height,30,30);
rect(-width/4-10,height/2.25,75,height,30,30);
rect(-width/4-100,height/1.75,50,height,30,30);
//foreground
fill(212,122,19); //red sand color
rect(0,height/3,width,height/3);
//cactus
fill(122,132,80); //green
rect(-width/5+10,0,30,200,30,30,30,30);
rect(-width/5+40,0,80,30,30,30,30,30);
rect(-width/5+65,-20,30,60,30,30,30,30);
rect(-width/5-20,-40,50,30,30,30,30,30);
rect(-width/5-35,-70,30,85,30,30,30,30);
pop();
}
//changes the color of the sky (and ambiance) every hour
function sky(x,y) {
noStroke();
if (hour()==0) {
fill(18,27,103,150);
} else if (hour()==1) {
fill(67, 49, 82,150);
} else if (hour()==2) {
fill(107, 66, 65,150);
} else if (hour()==3) {
fill(146, 84, 48,150);
} else if (hour()==4) {
fill(176, 97, 35,150);
} else if (hour()==5) {
fill(216, 115, 18,120);
} else if (hour()==6) {
fill(255, 132, 1,120);
} else if (hour()==7) {
fill(208, 147, 48,90);
} else if (hour()==8) {
fill(170, 159, 86,90);
} else if (hour()==9) {
fill(113, 177, 142,60);
} else if (hour()==10) {
fill(57, 195, 199,60);
} else if (hour()==11) {
fill(0, 212, 255,60);
} else if (hour()==12) {
fill(23, 186, 235,60);
} else if (hour()==13) {
fill(46, 159, 215,60);
} else if (hour()==14) {
fill(92, 106, 175,60);
} else if (hour()==15) {
fill(115, 80, 155,60);
} else if (hour()==16) {
fill(138, 53, 135,60);
} else if (hour()==17) {
fill(161, 27, 115,90);
} else if (hour()==18) {
fill(183, 0, 95,120);
} else if (hour()==19) {
fill(136, 8, 97,120);
} else if (hour()==20) {
fill(113, 12, 98,120);
} else if (hour()==21) {
fill(89, 16, 99,150);
} else if (hour()==22) {
fill(50, 22, 101,150);
} else if (hour()==23) {
fill(8, 17, 94,150);
}
rect(width/2,height/2,width,height);
}
Project-06: Abstract Clock
//Catherine Liu
//Section D
//jianingl@andrew.cmu.edu
//Assignment-06-Project
//an abstract clock where the objects move according to seconds, minutes, and hours
var xPos = []; //array for x position of clouds
var yPos = []; //array for y position of clouds
function setup() {
createCanvas(480, 400);
//creates random positions for 60 clouds
for (i = 0; i < 60; i++) {
xPos[i] = random(0,480);
yPos[i] = random(0,150)
}
}
function draw() {
background(155,212,255);
fill(0,0,102);
rect(0,height/2, width, height/2);
//draws a number of clouds according to the current second
for (i = 0; i <= second(); i++) {
clouds(xPos[i], yPos[i]);
}
push();
sunMoon(-150, 0); //calls function that draws the rotating sun and moon
pop();
//aliens move closer to each other according to minutes
print(minute().toString())
rightAlien(480 - 8 * minute(), height/2); //calls function that draws right alien
leftAlien(0 + 8 * minute(), height/2); //calls function that draws left alien
}
function rightAlien (x, y) { //draws right alien
stroke(0,102,51);
strokeWeight(5);
//arm waves up and down according to seconds using mod
if (second() % 2 == 0) {
line(x-40, y-60, x-13,y-30); //left arm
} else if (second() % 2 != 0) {
line(x-45, y-50, x-13,y-30); //left arm
}
line(x+13, y-30, x+30, y-15); //right arm
line(x, y-50, x, y-30); //neck
stroke(204, 255, 153);
line(x-10, y, x-10, height); //left leg
line(x+10, y, x+10, height); // right leg
stroke(0,102,51);
line(x-17, y-70, x-7, y-50); //antennae
line(x+17, y-70, x+7, y-50); //antennae
noStroke();
fill(204, 255, 153);
ellipse(x, y-50, 30,20); //head
rect(x-15, y-35, 30,120,10); //body
fill(0,102,51)
//eyes look back when the aliens pass each other halfway
if (minute() < 31) {
circle(x-10, y-51, 5); // left eye
circle(x-3, y-51, 5); // right eye
} else if (minute() >= 31) {
circle(x+10, y-51, 5); // left eye
circle(x+3, y-51, 5); // right eye
}
}
function leftAlien(x, y) { //draws left alien
stroke(0,102,51);
strokeWeight(5);
//arm waves up and down according to seconds using mod
if (second() % 2 != 0) {
line(x+40, y-60, x+13,y-30); //left arm
} else if (second() % 2 == 0) {
line(x+45, y-50, x+13,y-30); //left arm
}
line(x-13, y-30, x-30, y-15); //left arm
line(x, y-50, x, y-30); //neck
stroke(204, 255, 153);
line(x-10, y, x-10, height); //left leg
line(x+10, y, x+10, height); //right leg
stroke(0,102,51);
line(x-17, y-70, x-7, y-50); //left antennae
line(x+17, y-70, x+7, y-50); //right antennae
noStroke();
fill(204, 255, 153);
ellipse(x, y-50, 30,20); //left man's head
rect(x-15, y-35, 30,120,10); //left man's body
fill(0,102,51)
//eyes look back when the aliens pass each other halfway
if (minute() < 31) {
circle(x+10, y-51, 5); // left eye
circle(x+3, y-51, 5); // right eye
} else if (minute() >= 31) {
circle(x-10, y-51, 5); // left eye
circle(x-3, y-51, 5); // right eye
}
}
function sunMoon (x, y) { //sun and moon rotates according to hour, from 0-12 it is sun, from 12-23 it is moon
translate(width/2, height/2);
var dx = 30 * hour();
rotate (radians(dx));
if (hour() > 12 & hour() <=23) {
fill(255, 243, 176);
arc(x, y, 100, 100, 0, PI + QUARTER_PI, PIE); //moon
ellipse(x + 30, y - 30, 5, 10);
ellipse(x - 30, y + 70, 5, 10);
} else if (hour() >= 0 & hour() <= 12) {
fill(255, 128, 0);
ellipse(x, y, 100, 100); //sun
}
}
function clouds(x, y) { //draws a cloud at position x, y
fill(233, 254, 255);
rect(x, y, 20, 10,30); //cloud
}
I started off this project by sketching a rough idea of what I wanted to make, which was two people playing badminton, with the shuttlecock moving from one end to the next in minutes. The sun and moon would then rotate according to the hour.
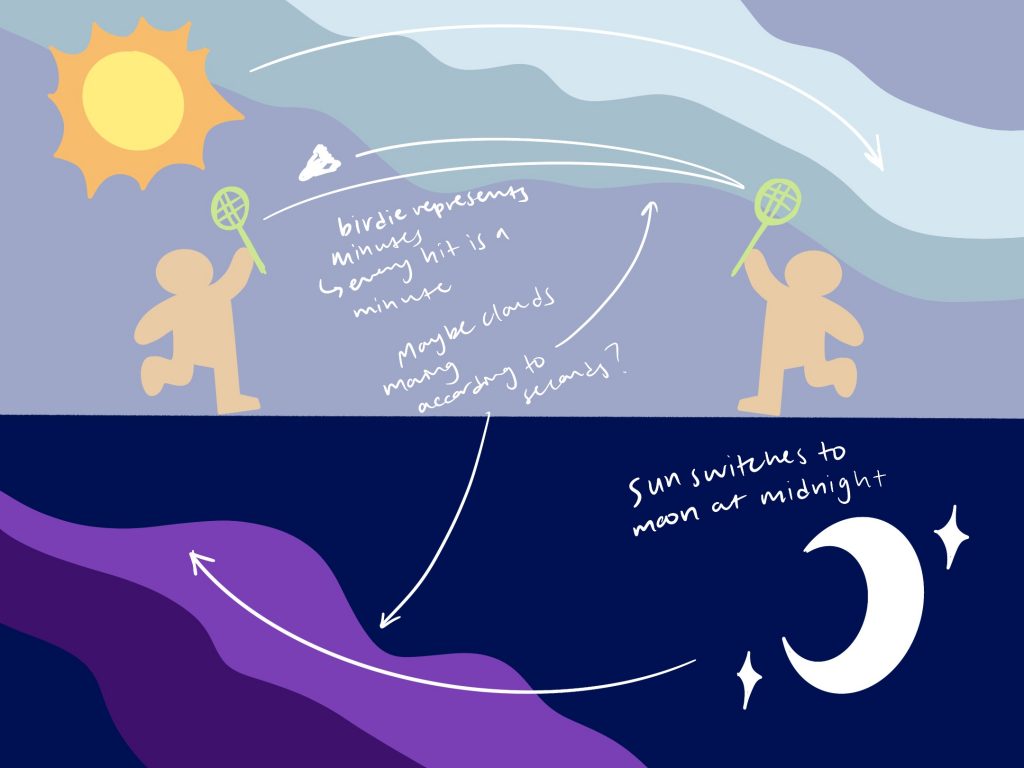
However, I came across some coding problems so I changed my idea slightly. Now, they are aliens that can only move with every minute. As they move closer to each other, they are waving and their eyes follow each other. While I kept the sun and moon concept the same, I changed the background clouds to increase numbers according to seconds.
Project 06 – Abstract Clock
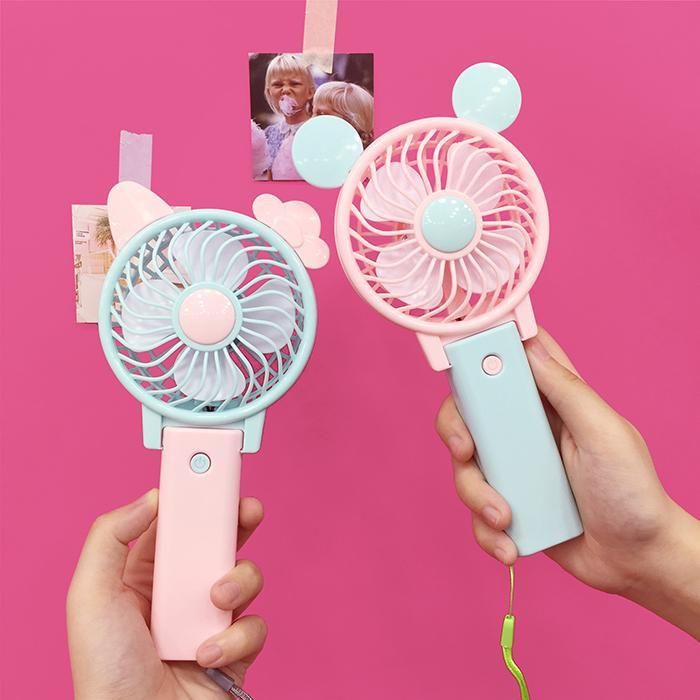
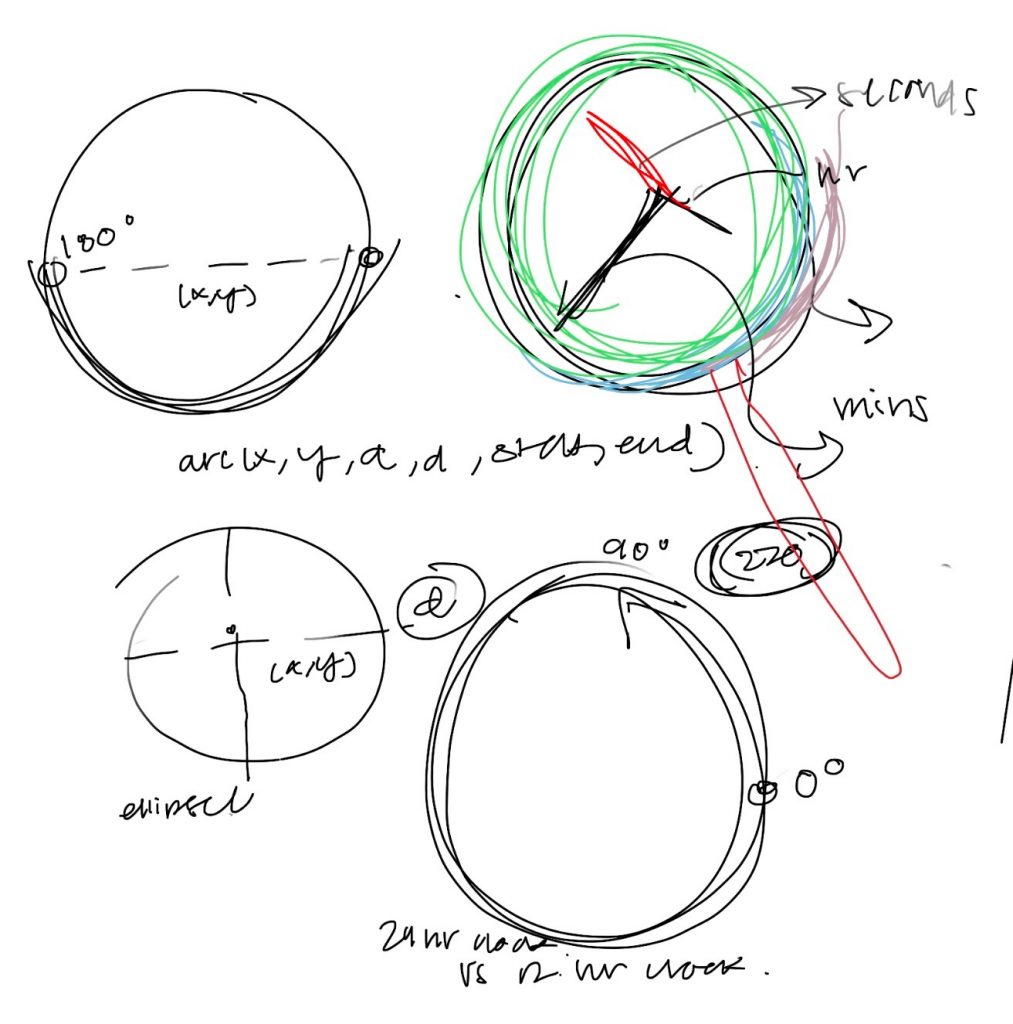
function setup() {
createCanvas(400, 400);
angleMode(DEGREES);
}
function draw() {
background(0); //black
translate(200,200);
let hr = hour();
let min = minute();
let sec = second();
strokeWeight(10);
stroke(244,195,195); // pink outline
noFill();
let end1 = map(sec, 0, 60, 0, 360); //seconds
arc(0,0,300,300,0,end1);
push();
rotate(end1); //rotate following the seconds
stroke(255,0,0);
line(0,0,100,0); //big clock line RED
pop();
stroke(137,207,240); // blue outline
let end2 = map(min, 0, 60, 0, 360); //minutes
arc(0,0,280,280,0,end2);
push();
rotate(end2); //rotate following the minutes
stroke(255);
line(0,0,100,0); //big clock line WHITE
pop();
stroke(157,193,131); //green outline
let end3 = map(hr,0,24,0,360); //hour
arc(0,0,260,260,0,end3);
push();
rotate(end3); //rotate following the hour
stroke(255);
line(0,0,50,0); //small clock line WHITE
pop();
//let end = map(mouseX, 0, width, 0, 360);
//arc(200,200,300,300,0,360);
//strokeWeight(6);
//stroke(255); // white
//noFill();
//ellipse(200,200,300,300);
//fill(255);
// noStroke();
// text
}
Project 6: Abstract Clock
// Yash Mittal
// Section D
var r1; // road 1
var r2; // road 2
var r3; // road 3
var secondscarwidth;
var minutecarwidth;
var hourcarwidth;
function setup() {
createCanvas(480, 480);
background (200);
}
function road1 (r1) {
noStroke();
fill (50);
rect (0, (height / 2 - 30), width, (height / 2 - 160)); // road 1
fill (250);
rect (40, (height / 2 + 3), 50, 10); // white stripe 1
rect (150, (height / 2 + 3), 50, 10); // white stripe 2
rect (260, (height / 2 + 3), 50, 10); // white stripe 3
rect (370, (height / 2 + 3), 50, 10); // white stripe 4
fill ("yellow");
rect (0, (height / 2 - 30), width, 5); // yellow stripe 1
rect (0, (height / 2 + 45), width, 5); // yellow stripe 2
}
function road2 (r2) {
fill (50);
rect (0, (height / 2 + 140), width, (height / 2 - 160)); // road 1
fill (250);
rect (40, (height / 2 + 173), 50, 10); // white stripe 1
rect (150, (height / 2 + 173), 50, 10); // white stripe 2
rect (260, (height / 2 + 173), 50, 10); // white stripe 3
rect (370, (height / 2 + 173), 50, 10); // white stripe 4
fill ("yellow");
rect (0, (height / 2 + 140), width, 5); // yellow stripe 1
rect (0, (height / 2 + 215), width, 5); // yellow stripe 2
}
function road3 (r3) {
fill (50);
rect (0, (height / 2 - 200), width, (height / 2 - 160)); // road 1
fill (250);
rect (40, (height / 2 - 167), 50, 10); // white stripe 1
rect (150, (height / 2 - 167), 50, 10); // white stripe 2
rect (260, (height / 2 - 167), 50, 10); // white stripe 3
rect (370, (height / 2 - 167), 50, 10); // white stripe 4
fill ("yellow");
rect (0, (height / 2 - 200), width, 5); // yellow stripe 1
rect (0, (height / 2 - 125), width, 5); // yellow stripe 2
}
function draw() {
background (10);
var sc = second(); // seconds
var mt = minute(); // minutes
var hr = hour(); // hours
road1 (r1);
road2 (r2);
road3 (r3);
var secondscarwidth = map (sc, 0, 59, -5, width - 3); // variable for seconds
var minutecarwidth = map (mt, 0, 59, -5, width - 3); // variable for minutes
var hourcarwidth = map (hr, 0, 23, -5, width - 3); // variable for hours
// car 1 - seconds car
fill (150, 0, 0);
rect (secondscarwidth, 60, 70, 40); // base of car
fill (100, 0, 0);
triangle (secondscarwidth + 35, 80, secondscarwidth + 70, 60, secondscarwidth + 70, 100); // window highlight
fill (250, 0, 0);
rect (secondscarwidth + 18, 70, 35, 20); // roof of car
fill (255, 255, 0);
rect (secondscarwidth + 70, 65, 2, 10); // headlight 1
rect (secondscarwidth + 70, 85, 2, 10); // headlight 2
fill (200, 0, 0);
rect (secondscarwidth + 50, 57, 10, 3); // side mirror 1
rect (secondscarwidth + 50, 100, 10, 3); // side mirror 2
// car 2 - minutes car
fill (173, 216, 230);
rect (minutecarwidth, 230, 70, 40); // base of car
fill (30, 144, 255);
triangle (minutecarwidth + 35, 250, minutecarwidth + 70, 230, minutecarwidth + 70, 270); // window highlight
fill (70, 130, 180);
rect (minutecarwidth + 18, 240, 35, 20); // roof of car
fill (255, 255, 0);
rect (minutecarwidth + 70, 235, 2, 10); // headlight 1
rect (minutecarwidth + 70, 255, 2, 10); // headlight 2
fill (135, 206, 255);
rect (minutecarwidth + 50, 227, 10, 3); // side mirror 1
rect (minutecarwidth + 50, 270, 10, 3); // side mirror 2
// car 3 - hours car
fill (0, 255, 127);
rect (hourcarwidth, 400, 70, 40); // base of car
fill (150, 205, 50);
triangle (hourcarwidth + 35, 420, hourcarwidth + 70, 400, hourcarwidth + 70, 440); // window highlight
fill (0, 165, 0);
rect (hourcarwidth + 18, 410, 35, 20); // roof of car
fill (255, 255, 0);
rect (hourcarwidth + 70, 405, 2, 10); // headlight 1
rect (hourcarwidth + 70, 425, 2, 10); // headlight 2
fill (152, 251, 152);
rect (hourcarwidth + 50, 397, 10, 3); // side mirror 1
rect (hourcarwidth + 50, 440, 10, 3); // side mirror 2
}
I wanted to keep my idea simple yet creative so I decided to make three roads with each car assigned to seconds, minutes, and hours respectively. The concept is very basic but I am happy with how the execution turned out because I was afraid that the car wouldn’t actually represent a car but I think it turned out well.
Abstract Clock
var time = 0;
var pupil = 400;
function setup() {
createCanvas(600, 600);
background(182, 217, 214);
}
function draw() {
fill(200, 200, 0);
ellipse(300, 300, 600, 400);
fill(0, 0, 0);
if(time <= 720) pupil -= 0.4;
else pupil += 0.4;
ellipse(300, 300, pupil, 400);
time++;
time%=1440;
}
This clock is inspired by this image, which claims that a cat’s eyes will change during the day. Although not all that practical, I think it’s a super cool idea.
Project 6 – Clock
This work is inspired by “A Million Times”, though it tells time in a very different way. I created an array of small, one-arm clocks to tell seconds, minutes, and hours, based on their columns and rows. This effectively creates a vector field that tells time with its coefficients. While each time in the day looks distinct and often disjointed, the array becomes spontaneously synchronized every now and again and blurs the viewer’s ability to tell the time. I understand if you don’t want to put this on your wrist but I hope you enjoy it on your computer screen for a little bit 🙂
var d = 18;
function setup() {
createCanvas(480, 240);
background(240);
}
function drawClock(x,y,theta) {
push();
translate(x,y);
stroke(220);
strokeWeight(1);
circle(0, 0, 40);
stroke(0);
strokeWeight(2);
line(0, 0, d*cos(radians(theta)), d*sin(radians(theta)));
pop();
}
function draw() {
for (var j = 0; j < 6; j++) {
for (var i = 0; i < 4; i++) {
sMap = map(second(), 0, 59, 0, 360);
drawClock(40*i+20, 40*j+20, sMap+10*i-10*j);
}
for (var i = 0; i < 4; i++) {
push();
translate(160,0);
mMap = map(minute(), 0, 59, 0, 360);
drawClock(40*i+20, 40*j+20, mMap+10*i-10*j);
pop();
}
for (var i = 0; i < 4; i++) {
push();
translate(320,0);
hMap = map(hour(), 0, 59, 0, 360);
drawClock(40*i+20, 40*j+20, hMap+10*i-10*j);
pop();
}
}
}
Project 06: Abstract Clock
I found this project a little challenging, but I do like what I was able to make in the end! There were elements that I was really hoping to add, like an inverted color for the background and lines between the morning and afternoon, but my hours function was not working so I wasn’t able to include that aspect. But I mainly wanted to create a clock that created a full picture with the end of a day. The circles on the right count the minutes and sections and the lines on the right create a grid that has lines added each hour.
//Jacky Lococo
//jlococo
//Section C
function setup() {
createCanvas(370, 600);
}
function draw() {
background(0);
var sec = second() // variable ofr the seconds
var min = minute() // variable for minutes
var h = hour()
var strokeLine = 255 // varible for stroke (ended up not working)
//white lines that divide seconds, minutes, hours
strokeWeight(1)
stroke(strokeLine)
line(330, 0, 330, 600)
strokeWeight(1)
stroke(strokeLine)
line(290, 0, 290, 600)
//y value for the seconds cirlces
for (var y = 10; y <= 10*(sec); y += 10) {
strokeWeight(0)
fill(255, 204, 204)
ellipse(350, y, 10, 10);
}
//y value for the minutes since x is the same for each added cirlce
for(var ym = 10; ym <= 10*(min); ym +=10){
strokeWeight(0)
fill(255, 102, 102)
ellipse(310, ym, 10, 10)
}
//hour-horizontal and verticle lines will be drawn with each hour to create a grid
//y value change for the hours - horizontal visualization
for(var yh = 25; yh <= 25*(h); yh += 25){
strokeWeight(1)
stroke(strokeLine)
line(0, yh, 290, yh)
}
//x valye for the change in hour - verticle depiction
for(var xh = 290/24; xh <= (290/24)*(h); xh += 290/24){
strokeWeight(1)
stroke(strokeLine)
line(xh, 0, xh, 600)
}
//red cirlces that follow the hour lines
strokeWeight(0)
fill(255, 51, 51)
ellipse(275, yh - 25, 10, 10)
strokeWeight(0)
fill(255, 51, 51)
ellipse(xh-290/24, 580, 10, 10)
}
Project 6 – Abstract Clock
Emilio Bustamante
Section D
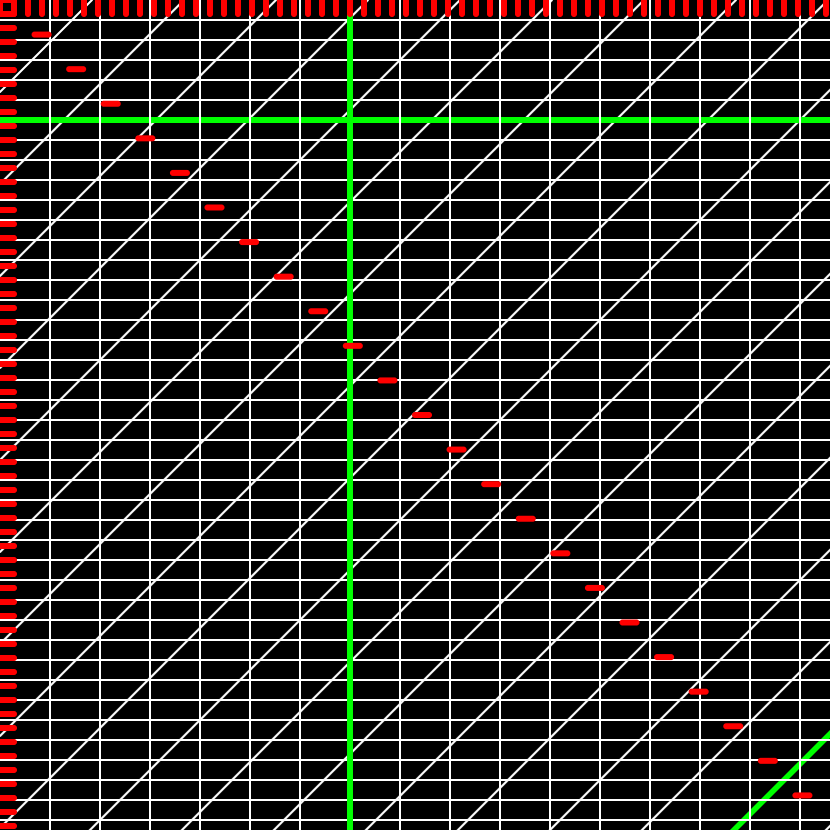
//Emilio Bustamante
//ebustama@andrew.cmu.edu
//Section D
//Project-06-Abstract clock
function setup() {
createCanvas(415, 415);
}
function draw() {
//variables for the time
var seconds = second();
var minutes = minute();
var hours= hour();
background(0)
push();
stroke(255);
strokeWeight(1);
// hours
line(hours*2,0,0,hours*2);
line(hours*4,0,0,hours*4);
line(hours*6,0,0,hours*6);
line(hours*8,0,0,hours*8);
line(hours*10,0,0,hours*10);
line(hours*12,0,0,hours*12);
line(hours*14,0,0,hours*14);
line(hours*16,0,0,hours*16);
line(hours*18,0,0,hours*18);
line(hours*20,0,0,hours*20);
line(hours*22,0,0,hours*22);
line(hours*24,0,0,hours*24);
line(hours*26,0,0,hours*26);
line(hours*28,0,0,hours*28);
line(hours*30,0,0,hours*30);
line(hours*32,0,0,hours*32);
// hours real time line
stroke(0,255,0);
strokeWeight(3);
line(hours*34,0,0,hours*34);
stroke(255);
strokeWeight(1);
line(hours*36,0,0,hours*36);
line(hours*38,0,0,hours*38);
line(hours*40,0,0,hours*40);
line(hours*42,0,0,hours*42);
line(hours*44,0,0,hours*44);
line(hours*46,0,0,hours*46);
line(hours*48,0,0,hours*48);
line(hours*50,0,0,hours*50);
// minutes
line(0,minutes,415,minutes);
line(0,minutes*2,415,minutes*2);
line(0,minutes*3,415,minutes*3);
line(0,minutes*4,415,minutes*4);
line(0,minutes*5,415,minutes*5);
// minutes real time line
stroke(0,255,0);
strokeWeight(3);
line(0,minutes*6,415,minutes*6);
stroke(255);
strokeWeight(1);
line(0,minutes*7,415,minutes*7);
line(0,minutes*8,415,minutes*8);
line(0,minutes*9,415,minutes*9);
line(0,minutes*10,415,minutes*10);
line(0,minutes*11,415,minutes*11);
line(0,minutes*12,415,minutes*12);
line(0,minutes*13,415,minutes*13);
line(0,minutes*14,415,minutes*14);
line(0,minutes*15,415,minutes*15);
line(0,minutes*16,415,minutes*16);
line(0,minutes*17,415,minutes*17);
line(0,minutes*18,415,minutes*18);
line(0,minutes*19,415,minutes*19);
line(0,minutes*20,415,minutes*20);
line(0,minutes*21,415,minutes*21);
line(0,minutes*22,415,minutes*22);
line(0,minutes*23,415,minutes*23);
line(0,minutes*24,415,minutes*24);
line(0,minutes*25,415,minutes*25);
line(0,minutes*26,415,minutes*26);
line(0,minutes*27,415,minutes*27);
line(0,minutes*28,415,minutes*28);
line(0,minutes*29,415,minutes*29);
line(0,minutes*30,415,minutes*30);
line(0,minutes*31,415,minutes*31);
line(0,minutes*32,415,minutes*32);
line(0,minutes*33,415,minutes*33);
line(0,minutes*34,415,minutes*34);
line(0,minutes*35,415,minutes*35);
line(0,minutes*36,415,minutes*36);
line(0,minutes*37,415,minutes*37);
line(0,minutes*38,415,minutes*38);
line(0,minutes*39,415,minutes*39);
line(0,minutes*40,415,minutes*40);
line(0,minutes*41,415,minutes*41);
line(0,minutes*42,415,minutes*42);
line(0,minutes*43,415,minutes*43);
line(0,minutes*44,415,minutes*44);
line(0,minutes*45,415,minutes*45);
line(0,minutes*46,415,minutes*46);
line(0,minutes*47,415,minutes*47);
line(0,minutes*48,415,minutes*48);
line(0,minutes*49,415,minutes*49);
line(0,minutes*50,415,minutes*50);
//seconds
line(seconds,0,seconds,415);
line(seconds*2,0,seconds*2,415);
line(seconds*3,0,seconds*3,415);
line(seconds*4,0,seconds*4,415);
line(seconds*5,0,seconds*5,415);
line(seconds*6,0,seconds*6,415);
// seconds real time line
stroke(0,255,0);
strokeWeight(3);
line(seconds*7,0,seconds*7,415);
stroke(255);
strokeWeight(1);
line(seconds*8,0,seconds*8,415);
line(seconds*9,0,seconds*9,415);
line(seconds*10,0,seconds*10,415);
line(seconds*11,0,seconds*11,415);
line(seconds*12,0,seconds*12,415);
line(seconds*13,0,seconds*13,415);
line(seconds*14,0,seconds*14,415);
line(seconds*15,0,seconds*15,415);
line(seconds*16,0,seconds*16,415);
line(seconds*17,0,seconds*17,415);
line(seconds*18,0,seconds*18,415);
line(seconds*19,0,seconds*19,415);
line(seconds*20,0,seconds*20,415);
line(seconds*21,0,seconds*21,415);
line(seconds*22,0,seconds*22,415);
line(seconds*23,0,seconds*23,415);
line(seconds*24,0,seconds*24,415);
line(seconds*25,0,seconds*25,415);
line(seconds*26,0,seconds*26,415);
line(seconds*27,0,seconds*27,415);
line(seconds*28,0,seconds*28,415);
line(seconds*29,0,seconds*29,415);
line(seconds*30,0,seconds*30,415);
line(seconds*31,0,seconds*31,415);
line(seconds*32,0,seconds*32,415);
line(seconds*33,0,seconds*33,415);
line(seconds*34,0,seconds*34,415);
line(seconds*35,0,seconds*35,415);
line(seconds*36,0,seconds*36,415);
line(seconds*37,0,seconds*37,415);
line(seconds*38,0,seconds*38,415);
line(seconds*39,0,seconds*39,415);
line(seconds*40,0,seconds*40,415);
line(seconds*41,0,seconds*41,415);
line(seconds*42,0,seconds*42,415);
line(seconds*43,0,seconds*43,415);
line(seconds*44,0,seconds*44,415);
line(seconds*45,0,seconds*45,415);
line(seconds*46,0,seconds*46,415);
line(seconds*47,0,seconds*47,415);
line(seconds*48,0,seconds*48,415);
line(seconds*49,0,seconds*49,415);
line(seconds*50,0,seconds*50,415);
pop();
// red reference lines
stroke(255,0,0);
strokeWeight(3);
var y = 0;
var spacing = 7;
var len= 7;
//seconds red lines
for (var x = 0; x <= 415; x += spacing) {
line(x, y, x, y + len);
}
//minutes red lines
for (var y = 0; y <= 415; y += 7) {
for (var x = 0; x <=0; x += 7) {
line(x, y, x + len, y );
}
}
//hours red lines
var w = width/24;
var h = height/24;
var y = 0;
for (var x = 0; x < width; x += w) {
line(x, y, x + len, y );
y += h;
}
}
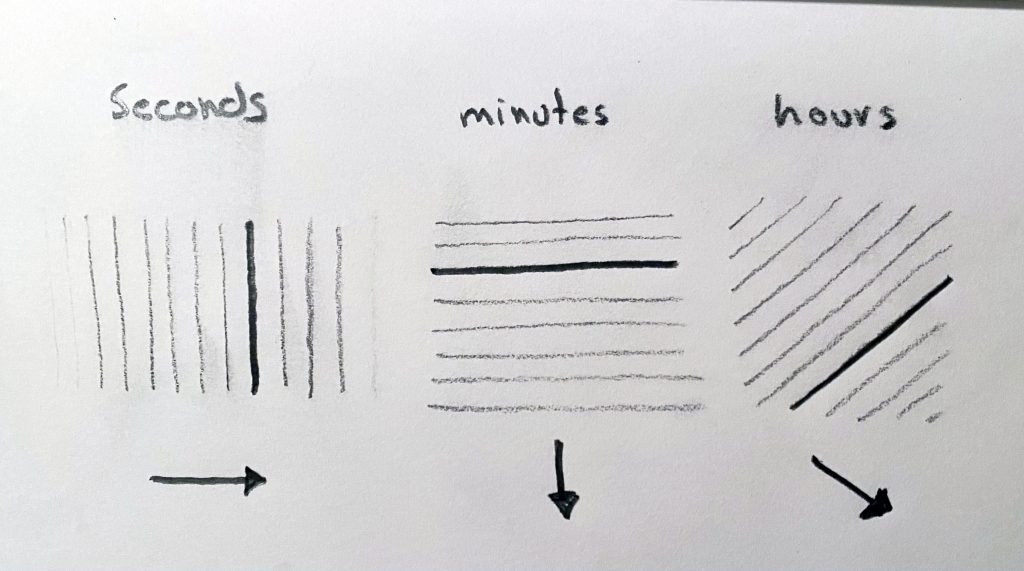
This clock intends to create different forms depending on the time of the day by overlapping different groups of lines oriented by seconds, minutes and hours. Each group of lines would have multiple lines to create a grid but the green lines will determine the time it is.
//Emilio Bustamante
//ebustama@andrew.cmu.edu
//Section D
//Project-06-Abstract clock
function setup() {
createCanvas(415, 415);
}
function draw() {
//variables for the time
var seconds = second();
var minutes = minute();
var hours= hour();
background(0)
push();
stroke(255);
strokeWeight(1);
// hours
line(hours*2,0,0,hours*2);
line(hours*4,0,0,hours*4);
line(hours*6,0,0,hours*6);
line(hours*8,0,0,hours*8);
line(hours*10,0,0,hours*10);
line(hours*12,0,0,hours*12);
line(hours*14,0,0,hours*14);
line(hours*16,0,0,hours*16);
line(hours*18,0,0,hours*18);
line(hours*20,0,0,hours*20);
line(hours*22,0,0,hours*22);
line(hours*24,0,0,hours*24);
line(hours*26,0,0,hours*26);
line(hours*28,0,0,hours*28);
line(hours*30,0,0,hours*30);
line(hours*32,0,0,hours*32);
// hours real time line
stroke(0,255,0);
strokeWeight(3);
line(hours*34,0,0,hours*34);
stroke(255);
strokeWeight(1);
line(hours*36,0,0,hours*36);
line(hours*38,0,0,hours*38);
line(hours*40,0,0,hours*40);
line(hours*42,0,0,hours*42);
line(hours*44,0,0,hours*44);
line(hours*46,0,0,hours*46);
line(hours*48,0,0,hours*48);
line(hours*50,0,0,hours*50);
// minutes
line(0,minutes,415,minutes);
line(0,minutes*2,415,minutes*2);
line(0,minutes*3,415,minutes*3);
line(0,minutes*4,415,minutes*4);
line(0,minutes*5,415,minutes*5);
// minutes real time line
stroke(0,255,0);
strokeWeight(3);
line(0,minutes*6,415,minutes*6);
stroke(255);
strokeWeight(1);
line(0,minutes*7,415,minutes*7);
line(0,minutes*8,415,minutes*8);
line(0,minutes*9,415,minutes*9);
line(0,minutes*10,415,minutes*10);
line(0,minutes*11,415,minutes*11);
line(0,minutes*12,415,minutes*12);
line(0,minutes*13,415,minutes*13);
line(0,minutes*14,415,minutes*14);
line(0,minutes*15,415,minutes*15);
line(0,minutes*16,415,minutes*16);
line(0,minutes*17,415,minutes*17);
line(0,minutes*18,415,minutes*18);
line(0,minutes*19,415,minutes*19);
line(0,minutes*20,415,minutes*20);
line(0,minutes*21,415,minutes*21);
line(0,minutes*22,415,minutes*22);
line(0,minutes*23,415,minutes*23);
line(0,minutes*24,415,minutes*24);
line(0,minutes*25,415,minutes*25);
line(0,minutes*26,415,minutes*26);
line(0,minutes*27,415,minutes*27);
line(0,minutes*28,415,minutes*28);
line(0,minutes*29,415,minutes*29);
line(0,minutes*30,415,minutes*30);
line(0,minutes*31,415,minutes*31);
line(0,minutes*32,415,minutes*32);
line(0,minutes*33,415,minutes*33);
line(0,minutes*34,415,minutes*34);
line(0,minutes*35,415,minutes*35);
line(0,minutes*36,415,minutes*36);
line(0,minutes*37,415,minutes*37);
line(0,minutes*38,415,minutes*38);
line(0,minutes*39,415,minutes*39);
line(0,minutes*40,415,minutes*40);
line(0,minutes*41,415,minutes*41);
line(0,minutes*42,415,minutes*42);
line(0,minutes*43,415,minutes*43);
line(0,minutes*44,415,minutes*44);
line(0,minutes*45,415,minutes*45);
line(0,minutes*46,415,minutes*46);
line(0,minutes*47,415,minutes*47);
line(0,minutes*48,415,minutes*48);
line(0,minutes*49,415,minutes*49);
line(0,minutes*50,415,minutes*50);
//seconds
line(seconds,0,seconds,415);
line(seconds*2,0,seconds*2,415);
line(seconds*3,0,seconds*3,415);
line(seconds*4,0,seconds*4,415);
line(seconds*5,0,seconds*5,415);
line(seconds*6,0,seconds*6,415);
// seconds real time line
stroke(0,255,0);
strokeWeight(3);
line(seconds*7,0,seconds*7,415);
stroke(255);
strokeWeight(1);
line(seconds*8,0,seconds*8,415);
line(seconds*9,0,seconds*9,415);
line(seconds*10,0,seconds*10,415);
line(seconds*11,0,seconds*11,415);
line(seconds*12,0,seconds*12,415);
line(seconds*13,0,seconds*13,415);
line(seconds*14,0,seconds*14,415);
line(seconds*15,0,seconds*15,415);
line(seconds*16,0,seconds*16,415);
line(seconds*17,0,seconds*17,415);
line(seconds*18,0,seconds*18,415);
line(seconds*19,0,seconds*19,415);
line(seconds*20,0,seconds*20,415);
line(seconds*21,0,seconds*21,415);
line(seconds*22,0,seconds*22,415);
line(seconds*23,0,seconds*23,415);
line(seconds*24,0,seconds*24,415);
line(seconds*25,0,seconds*25,415);
line(seconds*26,0,seconds*26,415);
line(seconds*27,0,seconds*27,415);
line(seconds*28,0,seconds*28,415);
line(seconds*29,0,seconds*29,415);
line(seconds*30,0,seconds*30,415);
line(seconds*31,0,seconds*31,415);
line(seconds*32,0,seconds*32,415);
line(seconds*33,0,seconds*33,415);
line(seconds*34,0,seconds*34,415);
line(seconds*35,0,seconds*35,415);
line(seconds*36,0,seconds*36,415);
line(seconds*37,0,seconds*37,415);
line(seconds*38,0,seconds*38,415);
line(seconds*39,0,seconds*39,415);
line(seconds*40,0,seconds*40,415);
line(seconds*41,0,seconds*41,415);
line(seconds*42,0,seconds*42,415);
line(seconds*43,0,seconds*43,415);
line(seconds*44,0,seconds*44,415);
line(seconds*45,0,seconds*45,415);
line(seconds*46,0,seconds*46,415);
line(seconds*47,0,seconds*47,415);
line(seconds*48,0,seconds*48,415);
line(seconds*49,0,seconds*49,415);
line(seconds*50,0,seconds*50,415);
pop();
// red reference lines
stroke(255,0,0);
strokeWeight(3);
var y = 0;
var spacing = 7;
var len= 7;
//seconds red lines
for (var x = 0; x <= 415; x += spacing) {
line(x, y, x, y + len);
}
//minutes red lines
for (var y = 0; y <= 415; y += 7) {
for (var x = 0; x <=0; x += 7) {
line(x, y, x + len, y );
}
}
//hours red lines
var w = width/24;
var h = height/24;
var y = 0;
for (var x = 0; x < width; x += w) {
line(x, y, x + len, y );
y += h;
}
}
Abstract Clock
// project 06
// gnmarino@andrew.cmu.edu
// Gia Marino
// Section D
var theta = 0; // references theta in draw function only
var m; // current minute
var h; // current hour
function setup() {
createCanvas(480, 480);
background(255);
}
function draw() {
translate(width/2, height/2);
// rotates whole clock
rotate(radians(theta));
// draws the whole clock
NightAndDay(); // draws the night and day sides of the circle
sun(0, height/2 - 80);
moon(0, -height/2 + 80);
clouds(width/2 - 25, 0);
diamonds(-width/2 + 25, 0);
// takes current time and calculates how much to rotate the clock
// every minute the clock should turn .25 degrees
// every hour the clock should have rotated 15 more degrees
m = minute();
h = hour();
theta = (h * 15) + (m * .25);
}
function sun(sunX, sunY) {
push();
// orangey yellow color for sun
fill(253, 208, 25);
// moves sun to coordinates stated in draw function
translate(sunX, sunY);
circle(0, 0, 50); // middle of sun
// strokeWeight and loop makes the sun rays
strokeWeight(2);
for (theta = 0; theta < 360; theta += 45) {
rotate(radians(theta));
line(30, 0, 37, 0);
}
pop();
}
function moon(moonX, moonY) {
push();
fill(220); // light grey
// moves moon to coordinates stated in draw function
translate(moonX, moonY);
circle(0, 0, 50);
pop();
}
function diamonds(diamondX, diamondY) {
push();
noFill();
strokeWeight(5);
// counter keeps track how many times the for-loop loops
// needs to start at 12 so the diamonds don't fill in when it's before noon
var counter = 12;
// loop rotates and draws diamonds
for (theta = 7; theta < 177; theta += 15) {
push();
rotate(radians(theta));
// moves diamonds to coordinates stated in draw function
translate(diamondX, diamondY)
// scale & rotate are used to make diamonds look better along the border
scale(.25);
rotate(radians(90));
// if statement says the diamonds should be filled in based on the hour
// diamond fills in after every hour passes
// if clock hasn't passed that hour yet then diamond stays unfilled
// diamonds fill in for 1pm to 12 am
if (counter < h) {
fill(255);
} else {
noFill();
}
// draws 1 diamond every time
beginShape();
vertex(-20, 0);
quadraticVertex(-10, -5, 0, -30);
quadraticVertex(10, -5, 20, 0);
quadraticVertex(10, 5, 0, 30);
quadraticVertex(-10, 5, -20, 0);
endShape();
pop();
counter += 1; // adds 1 everytime loop ends
}
pop();
}
function clouds(cloudX, cloudY) {
push();
strokeWeight(5);
noFill();
// counter keeps track how many times the for-loop loops
// counter starts at zero so clouds starts filling after 1st hour has passed
var counter = 0;
// loop rotates and draws clouds
for (theta = 7; theta < 177; theta += 15) {
push();
rotate(radians(theta));
// moves clouds to coordinates stated in draw function
translate(cloudX, cloudY);
// scale & rotate are used to make clouds look better along the border
scale(.25);
rotate(radians(90));
// if statement says the clouds should be filled in based on the hour
// cloud fills in after every hour passes
// if clock hasn't passed that hour yet then cloud stays unfilled
// clouds fill in for 1 am to 12 pm
if (counter <= h) {
fill(255);
} else {
noFill();
}
// draws 1 cloud every time
beginShape();
vertex(0, 0);
quadraticVertex(-45, 5, -40, -10);
quadraticVertex(-35, -30, -15, -20);
quadraticVertex(-20, -40, 0, -40);
quadraticVertex(20, -40, 15, -20);
quadraticVertex(35, -30, 40, -10);
quadraticVertex(45, 5, 0, 0);
endShape();
pop();
counter += 1; // adds 1 everytime loop ends
}
pop();
}
function NightAndDay() {
push();
// yellow half circle represents day time
fill(255, 238, 127); // yellow
arc(0, 0, width, height, radians(0), radians(180));
// navy half circle represents night time
fill(60, 67, 129); // navy
arc(0, 0, width, height, radians(180), radians(360));
pop();
}
For this assignment I actually got inspiration from the Minecraft clock because it is the first example I could think of for a non-western standard clock.
I started off by deciding that I wanted the daylight half circle to be fully on the top and vice versa with the night side.
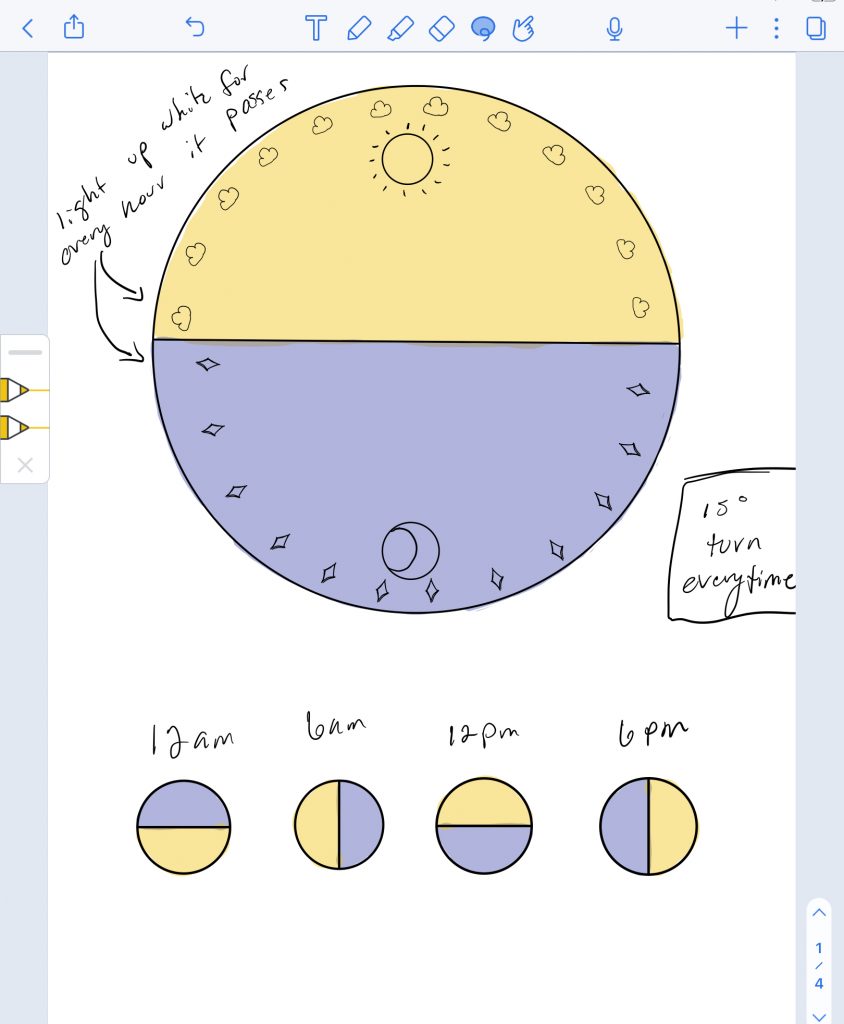
I ended up deciding that I wanted the clock to emulate how the sky typically looks if you are at a reasonable latitude on earth. So, when it is noon and the sun is highest in the sky then I want the clock’s yellow side to be fully on top so it looks like what you’d see if you’d looked outside at noon. It also kinda shows when the sun rises and sets too.
Lastly, I decided that I wanted someone to be looking at my clock and know what time it is and not completely guess. So, I made little hour symbols (the clouds and diamonds) light up every time an hour passes. This makes it when you look at the clock you can count how many clouds or diamonds are white and know it’s been x amount of hours.