For this project, I wanted to create an interactive portrait. I was inspired by Ryan Alexander’s work and wanted to have the portrait show up based on where you clicked your mouse. I wasn’t able to get that working fully, but I still think the final product is pretty cool as the way the portrait shows up changes based on where you click the mouse. I also added randomness to each pixel width and height to make the portrait more animated.
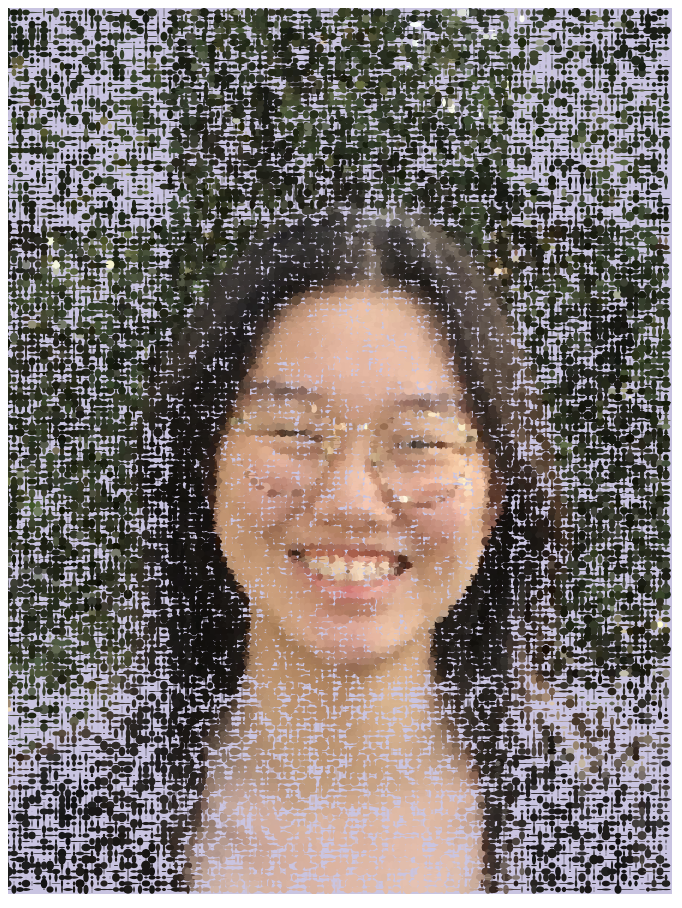
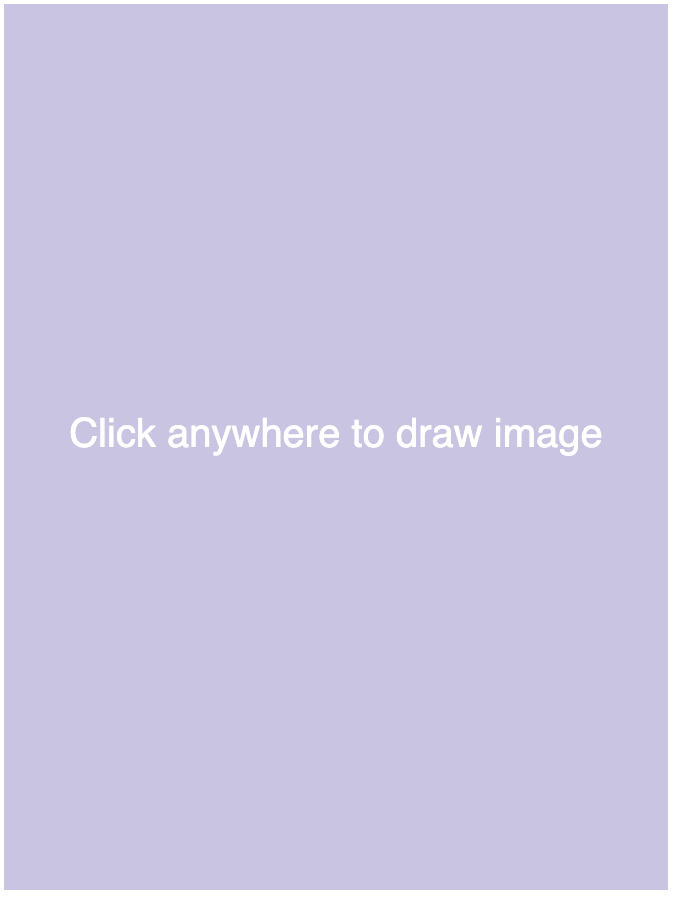
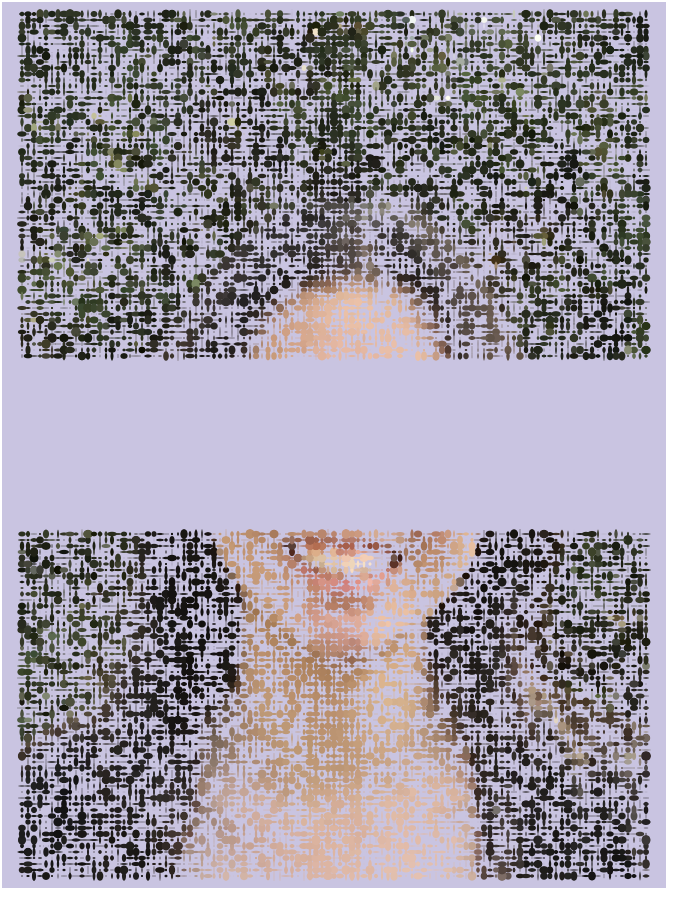
//Catherine Liu
//jianingl_andrew.cmu.edu
//Section D
//project_09
//image shows up in pixels and spreads out on canvas
var img;
var xStart; //stores mouseX
var yStart; //stores mouseY
var click = true; //checks for mousePressed and removes text
var radianSize = 0; //increases area of expansion
var clickCol = false; //checks color of background
function preload() {
img = loadImage("https://i.imgur.com/mzVFTDQ.jpg");
}
function setup() {
img.resize(img.width/2, img.height/2);
createCanvas(img.width, img.height);
frameRate(50);
print (img.width/2);
print(img.height/2)
}
function draw() {
//redraws background every mousePressed
if (clickCol) {
fill(203, 195, 227);
rect(0,0,width,height);
clickCol == false;
}
//sets up text that only shows up at beginning
if(click) {
background(203, 195, 227)
push();
fill(255);
textAlign(CENTER);
textSize(20)
text("Click anywhere to draw image",width/2, height/2)
pop();
}
pixelExpand();
}
function pixelExpand() {
for (var vert = yStart; vert < radianSize; vert += 3) {
for (var hor = xStart; hor < radianSize; hor += 3) {
noStroke();
//expands right downwards
var c = img.get(hor, vert);
fill(c);
ellipse(hor, vert, random(5), random(5));
//expands right upwards
var c2 = img.get(hor, height-vert);
fill(c2);
ellipse(hor, height-vert, random(5), random(5));
//expands left upwards
var c3 = img.get(width-hor, height-vert);
fill(c3);
ellipse(width-hor, height-vert, random(5), random(5));
//expands left downwards
var c4 = img.get(width-hor, vert);
fill(c4);
ellipse(width-hor, vert, random(5), random(5));
}
}
radianSize += 5; //increase expansion size
}
//returns starting point for pixels to show
function mousePressed() {
radianSize = 0;
clickCol = true;
click = false; //text disappears
xStart = constrain(mouseX, 0, width/4);
yStart = constrain(mouseY, 0, height/4);
}