Learning Objectives
- Implement a recursive function to draw a Sierpinski triangle.
- Draw a set of nine cubes in three-dimensional space as a 3 X 3 X 3 set of cubes.
- Draw a Rubik’s Cube in three-dimensional space by creating 9 cubes using six faces each of different colors.
Participation
In this lab/recitation, you will write some p5.js programs that use the techniques you’ve learned in class so far. The goal here is not just to get the programs done as quickly as possible, but also to help your peers if they get stuck and to discuss alternate ways to solve the same problems. You will be put into breakout rooms in Zoom to work on your code and then discuss your answers with each other, or to help each other if you get stuck. Then we will return to discuss the results together as a group.
For each problem, you will start with a copy of the uncompressed template-p5only.zip in a folder named lab-13. Rename the folder as andrewID-13-A, andrewID-13-B, etc. as appropriate.
A. Sierpinski Triangle
This is an example of a fractal, an image that is self-similar. When you look at parts of the image, you see the original image.
One of the most famous fractals is the Sierpinski Triangle which is a triangle that is divided up into three triangles which are divided up into three triangles each, which are divided up into… you get the idea.
To draw this sketch, we start with a large triangle of blue. We then find the midpoints of each of the three sides and draw a triangle between these points in the background color. Finally, we repeat the midpoint process with each of the three remaining blue triangles that are formed when we draw the triangle in the background color. Each of these triangles is “split” into three smaller triangles. This repeats until we reach the number of levels of repetition.
Complete the function splitIntoThree based on the comments to guide you.
B. Cube of Cubes
Write a program that creates a 3 X 3 X 3 set of cubes. each of size 100 X 100 X 100 in three dimensional space. Remember that you need to use the WEBGL renderer, and that the camera is initially facing in the -z direction, with x increasing toward the right and y increased downward and the origin (0,0,0) is initially in the center of the canvas. Use the orbitControl function in the draw function to allow you to use the mouse to move around the set of cubes once you’re done to check your work. Here is a picture of the expected result, once the mouse is used to move around in 3D space a little.
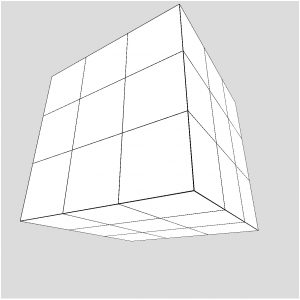
You should use the box function to create each cube, remembering that this function will draw the shape centered at the origin, so to draw all nine boxes, you will need to use transformations to move the origin.
LOOK FOR PATTERNS HERE! Where is the center of each of the 9 cubes? Do you see the pattern? You should be able to use a set of three nested loops to solve this problem, and if so, you will only need to write a call to the box function once.
C. Rubik’s Cube
Make a copy of the code for the previous problem and modify the copy so that the 3 X 3 X 3 cube you draw looks like a Rubik’s Cube in a solved state (ie. each side is all of one color).
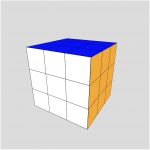
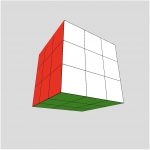
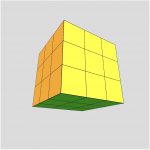
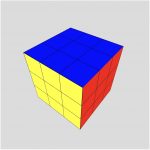
First, add a global variable size that represents the size of the box so that you can use other values other than 100. Update your program to use size instead of 100.
Now, think about drawing the 9 boxes in color. This is a bit more challenging than it might seem. When you draw each of 9 boxes, you need to color each side a different color,
Front: white
Back: yellow
Left: red
Right: orange
Top: blue
Bottom: green
but the box function won’t let you do this.
Instead, replace the box call in your draw function with a call to a separate function drawColorBox that you will write. In this helper function, you should draw a box centered at the origin that has 6 faces (but you won’t use the box function anymore). Draw a wire view of a box on a piece of paper and determine the coordinates of the 8 corners. HINT: Let sz2 be half the size variable; your coordinates should all be in terms of sz2. To draw each face, you will fill with the appropriate color, and then use beginShape, vertex (4 times) with the appropriate coordinates and endShape to create a square. (You can’t use the square function in 3D space since it doesn’t have a z coordinate parameter.) You will need to draw 6 squares (faces) to make the box.
Here’s code for the front face to get you started:
Your draw function will then call this drawColorBox function 9 times to create the 9 boxes. When you’re done, you should be able to see something that looks like a Rubik’s Cube.
Change the size to 50 for a smaller cube. Use the mouse to look around the Rubik’s Cube to make sure you correctly programmed all sides. If your mouse has a scroll wheel, scroll in and see what happens when you get too close.
D. Recursive Tree (Optional challenge)
Try to draw this picture recursively:
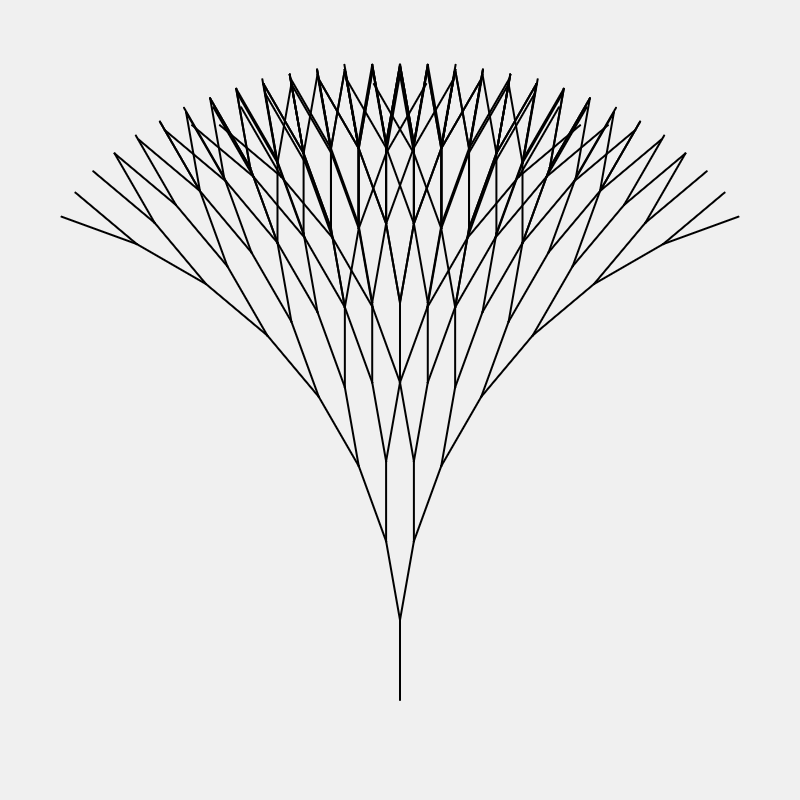
Handin
At the end of the lab, zip the lab-13 folder (whatever you got done) and submit it to Autolab. Do not worry if you did not complete all of the programming problems but you should have made it through problems A and B, and you should have some attempt at problem C. Problem D is not required, but it is given for those who want a challenge.