//Anthony Pan
//Section C
var d = 0; //diameter for 24 hr circles
var d1 = 0; //diameter for 24 hr circles
//var d2; //first circle diameter
//var d3; //first cirlce diameter
var c1 = []; //color array for the 24 hrs of a day
var hours = []; //hours in a day
function setup() {
createCanvas(480, 480);
for(var i = 0; i <= 23; i++) {
hours[i] = i;
if(i >= 0 & i <= 8){
c1[i] = color(0, random(256), random(256)); //random green + blue, no red first 8 hrs
}
if(i > 8 & i <= 16) {
c1[i] = color(random(256), 0 , random(256)); //random red + blue, no green between 8-16 hrs
}
if(i >16 & i<=23) {
c1[i] = color(random(256), random(256), 0); //random red + green, no blue 16-24 hrs
}
}
}
function draw() {
background(0);
draw_circle(d, d1);
//fill_circle(d2, d3);
noStroke();
fill(c1[hour()]); //fill color based on the hour
//draw a circle that grows based on the number of minutes that has passed
ellipse(240, 240, 20*hour() + minute()/3, 20*hour() + minute()/3);
//display clock
textSize(15);
strokeWeight(1);
fill(255);
text(hour() + ":" + minute() + ":" + second(), 10, 470); //display time on 24 hr clock at bottom left corner
}
function draw_circle(d, d1){
for(var i = 1; i <= 25; i++) {
noFill();
stroke(255); //create why circle stroke
ellipse(240, 240, d, d1); //create 24 concentric cirlces for 24 hrs in a day
d += 20;
d1 += 20; //d and d1 grow each cirlce's radius
}
}
//process for creating fill circle
/*
function fill_circle(d2, d3) { //fill circle based on minute
for(var i = 0; i <= hour(); i++) {
noStroke();
fill(c1[hour()]); //fill color based on the hour
ellipse(240, 240, 10*hour() + d2*minute(), 10*hour() + d3*minute());
d2 += 1/6; //rate of growth based on time /proportions
d3 += 1/6;
print(d2);
}
}
*/
/*
function fill_circle(d2, d3) { //fill circle based on minute
noStroke();
fill(c1[hour()]); //fill color based on the hour
ellipse(240, 240, 20*hour() + minute()/3, 20*hour() + minute()/3);
}
/*
function draw_circle(d, d1){
for(var i = 0; i <= 23; i++) {
noFill();
stroke(255);
ellipse(240, 240, d, d1); //create 24 concentric cirlces for 24 hrs in a day
d += 20;
d1 += 20; //d and d1 grow each cirlce's radius
}
}
*/
/*
function fill_circle(d2, d3) { //fill circle based on minute
for(var i = 0; i <= minute(); i++) {
noStroke();
fill(c1[hour()]); //fill color based on the hour
ellipse(240, 240, d2, d3);
d2 += 0.33; //rate of growth based on time /proportions
d3 += 0.33;
}
}
*/
Project Description:
This clock has 24 concentric circles representing the 24 hours in a day. A second function creates a circle that is filled with a random color based on the range of time: 0-8, 8-16, and 16-24. The circle fills the concentric circles slowly, growing based on each minute passed. There is a display of the time on a 24-hour clock at the bottom of the canvas to show the exact time as the circle fills the concentric circles very slowly.
Process/Ideation:
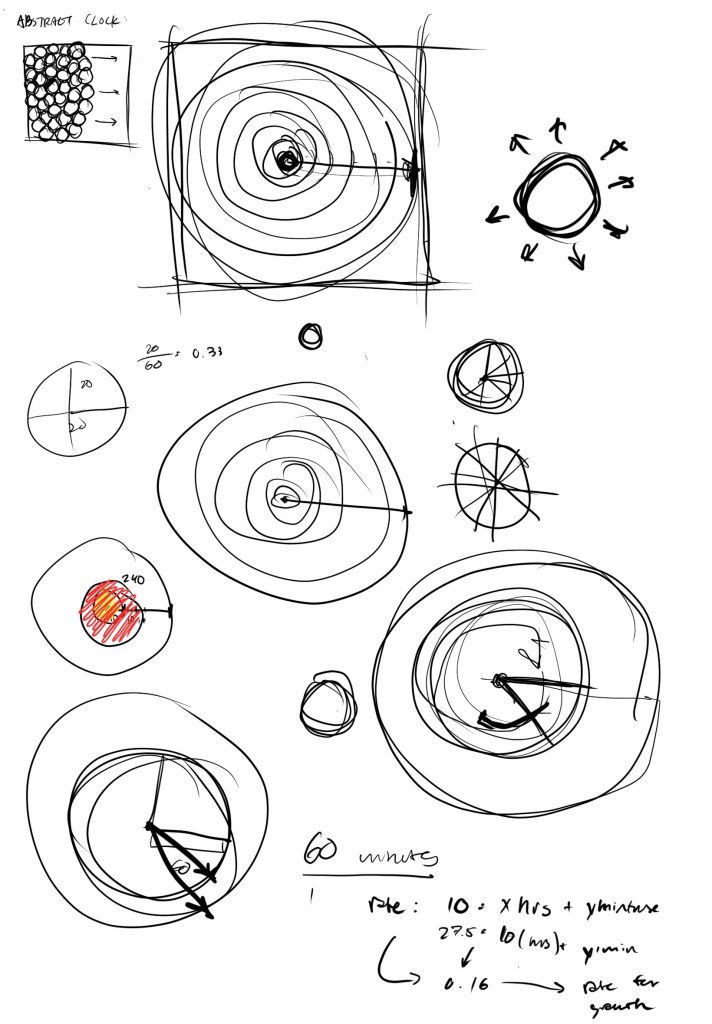
This was some of my process working on creating this clock.