This week’s project asked us to make a wallpaper, so I decided to make my work based on african textiles. Attached is a sketch of my initial idea and my final product. Enjoy!
sketch
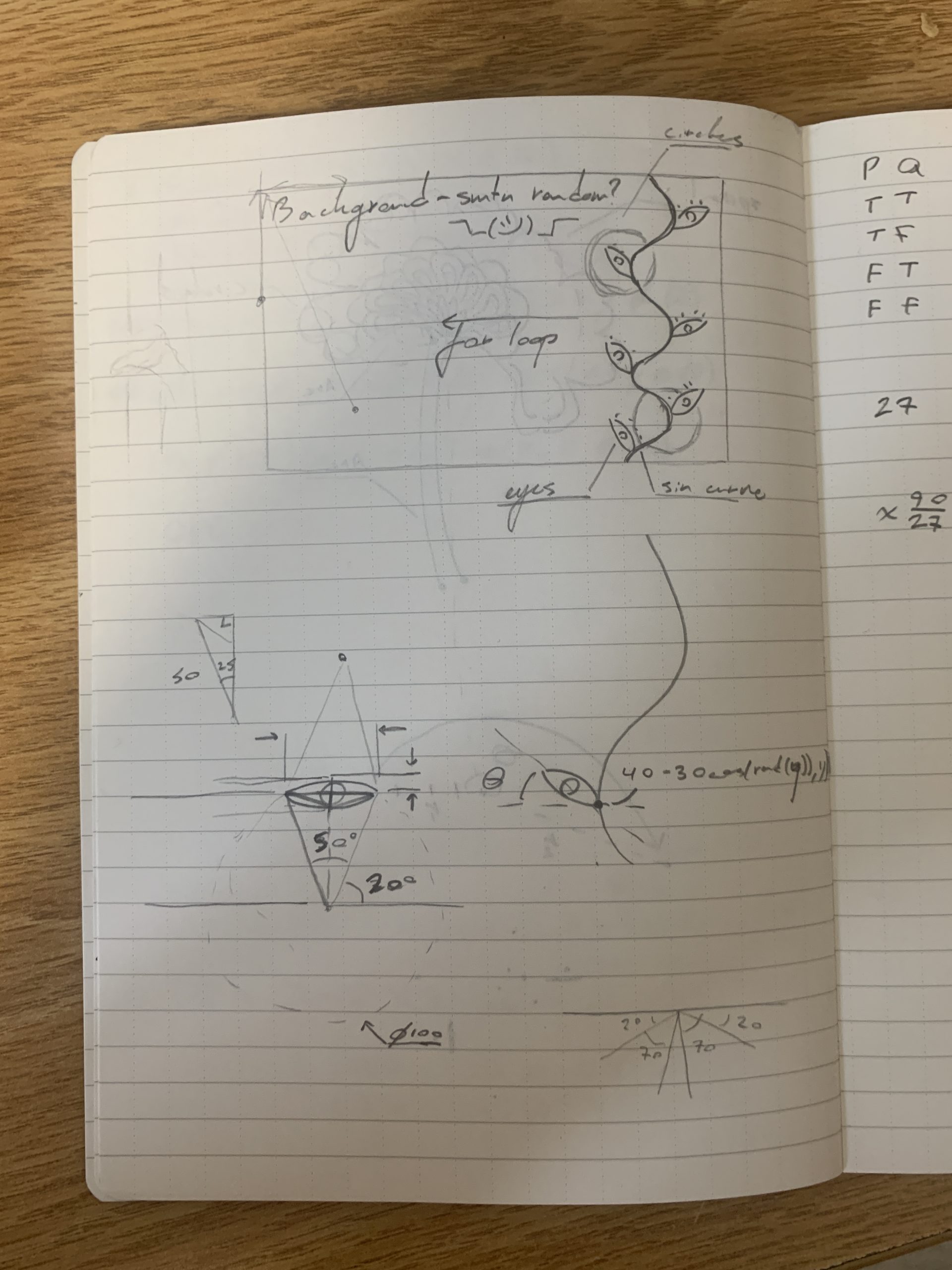
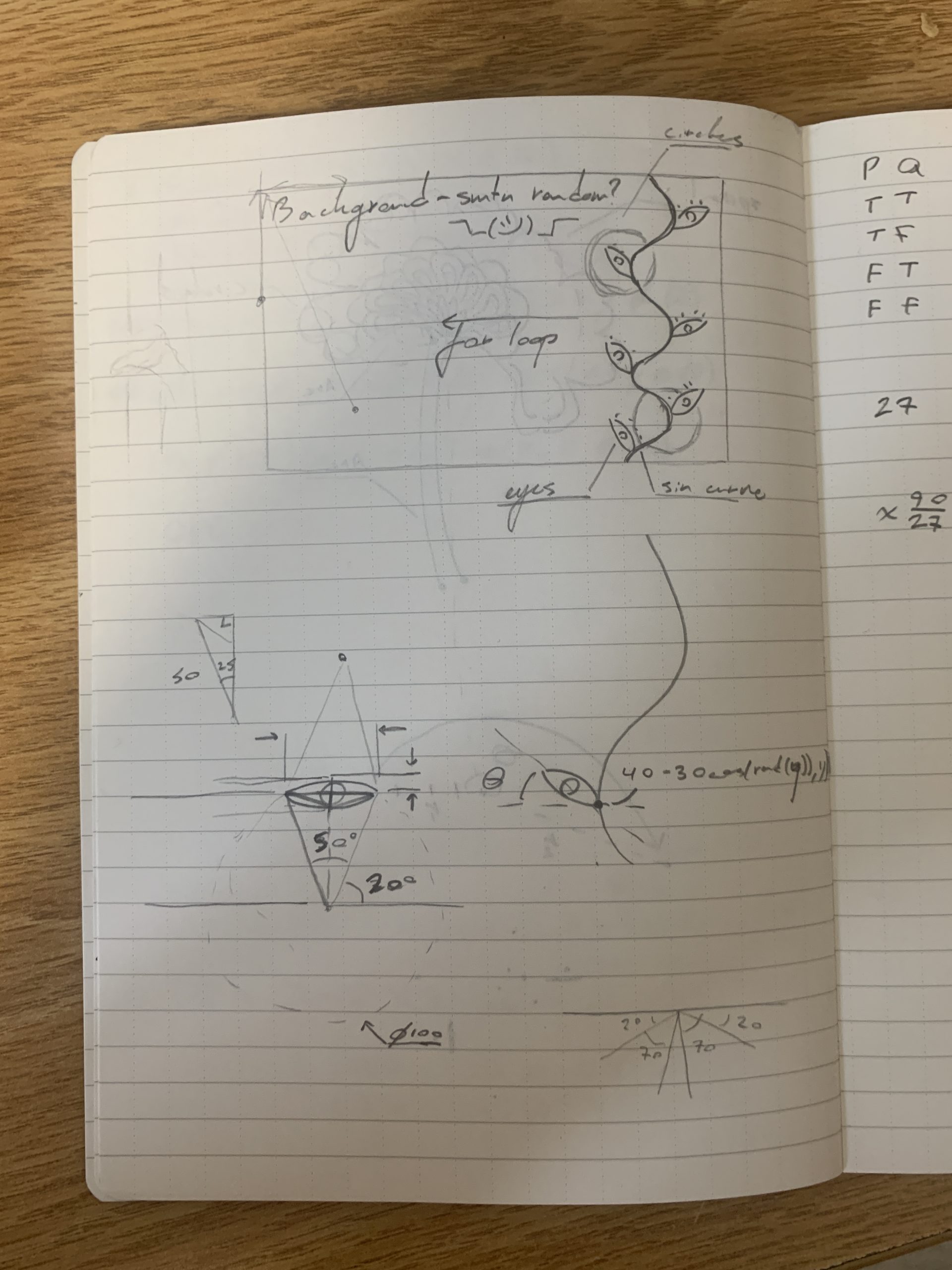
var num = 6; //number of itterations of everything
function setup() {
createCanvas(600, 400);
background(250, 249, 246);
}
/*let eyeBrown = color(165,42,42);
let bgBrown = color(255, 200, 168);
let branchGreen = color(63, 146, 65);
let turq = color(64,224,208);*/
function drawBackground() {
//random lines from ex 4 but in for loop
stroke(255, 200, 168);
strokeWeight(1);
for (var y = 0; y < height; y+=20) {
for (var x = 0; x < width; x+=20) {
if (random(1) > 0.5) {
line(x, y, x+20, y+20);
}
else {
line(x, y+20, x+20, y);
}
}
}
//ornaments
for (var j = 0; j <= width; j+=width/num) {
var colors = [color(145,205,211), color(234,204,84)]
noStroke();
fill(random(colors));
circle(random(j, j+(width/num)), random(height/2), random(40,60));
circle(random(j, j+(width/num)), random(height/2,height), random(40,60));
push();
fill(200,74,55);
translate(random(j,j+(width/num)), random(height));
for (var r = 10; r < 60; r+=10) {
for (var i = 0; i < 360; i+=30) {
push();
scale(0.3);
circle(r*cos(radians(i)), r*sin(radians(i)), r/3);
pop();
}
}
pop();
}
}
function drawEye(a, b, side) {
push();
translate(a, b);
//alternates side of branch
if (side == 1) {
rotate(degrees(-.009));
} else if (side == -1) {
rotate(degrees(.009));
}
//eye arcs
//blue filling
push();
scale(0.7);
noFill();
strokeWeight(10);
stroke(52,173,193);
arc(0, -45, 100, 100, radians(65), radians(180-65));
push();
rotate(radians(180));
arc(0, -45, 100, 100, radians(65), radians(180-65));
pop();
pop();
//green arcs
strokeWeight(3);
noFill();
stroke(50, 118, 112);
arc(0, -45, 100, 100, radians(65), radians(180-65));
push();
rotate(radians(180));
arc(0, -45, 100, 100, radians(65), radians(180-65));
pop();
//gold arcs
push();
scale(0.9);
noFill();
strokeWeight(2);
stroke(234,178,57);
arc(0, -45, 100, 100, radians(65), radians(180-65));
push();
rotate(radians(180));
arc(0, -45, 100, 100, radians(65), radians(180-65));
pop();
pop();
fill(165,42,42);
noStroke();
circle(0, 0, 10); //eyeball
//lashes
strokeWeight(1);
push();
translate(0, 45);
rotate(radians(-35));
stroke(0);
for (var i = 0; i <= 50; i+=10) {
rotate(radians(10));
line(0, -52, 0, -54);
}
pop();
pop();
}
function drawBranches() {
stroke(63, 146, 65);
for (var x = 0; x <= width; x+= width/num) {
push();
translate(x, random(-50,50));
var side = 1;
for (var y = -100; y < height+100; y += 1) {
//draw alternating eyes
if ((y%50) == 0 & side == 1) {
drawEye(cosy+19,y-10,side);
side = -1*side;
} else if ((y%50) == 0 & side == -1){
drawEye(cosy-19,y-10,side);
side = -1*side;
}
//draw branches (vertical cosine curves)
var cosy = 40-30 * cos(radians(y));
stroke(50, 118, 112);
strokeWeight(5);
point(cosy, y);
}
pop();
}
}
var count = 0;
function draw() {
drawBackground();
drawBranches();
noLoop();
}