The first thing that came to mind when thinking of portrait is Warhol’s Mao. I radially sampled a portrait of Mao and mapped the sum of each sampled pixel’s red and blue values from 0 to 5, and used that as the size of each dot to make a pop-art-esk halftone effect. I attached a photo because the actual output is too big. Enjoy!!
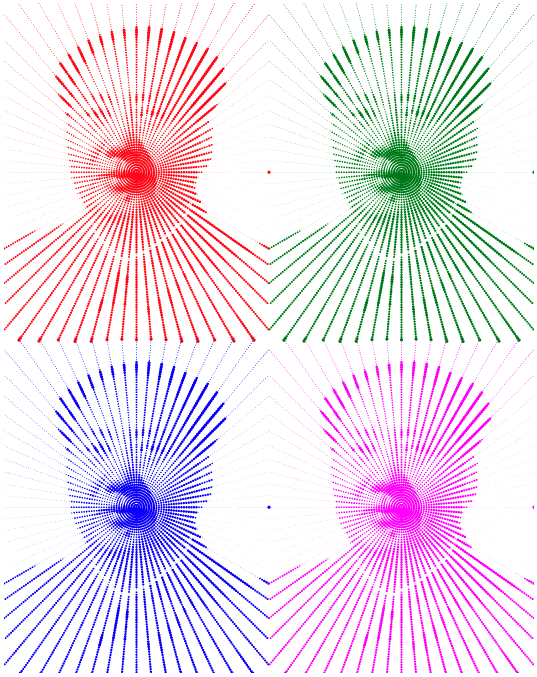
sketch
var img;
var step = 5;
var rmax = 6;
var xpos = [];
var ypos = [];
var rad = [];
function preload() {
img = loadImage('https://i.imgur.com/R13OPCr.jpeg');
}
function setup() {
createCanvas(2*530, 2*670);
image(img, 0, 0, width/2, height/2);
}
function draw() {
rectMode(CENTER);
noStroke();
var xposNum;
var yposNum;
for (var r = 0; r < img.width; r+=step) {
for (var theta = 0; theta < img.height; theta+=step) {
xposNum = img.width/2+r*cos(radians(theta));
yposNum = img.height/2+r*sin(radians(theta));
var c = get(xposNum,yposNum);
fill(c);
xpos.push(xposNum);
ypos.push(yposNum);
c = red(c)+blue(c);
c = map(c, 0, 510, 0, rmax);
if (xposNum > img.width || yposNum > img.height || xposNum < 0 || yposNum < 0) {
c = rmax;
}
rad.push(rmax - c);
}
}
background(255);
push();
for (var i = 0; i < xpos.length; i++) {
fill('RED');
ellipse(xpos[i], ypos[i], rad[i]);
}
translate(width/2, 0);
for (var i = 0; i < xpos.length; i++) {
fill('GREEN');
ellipse(xpos[i], ypos[i], rad[i]);
}
pop();
push();
translate(0, height/2);
for (var i = 0; i < xpos.length; i++) {
fill('BLUE');
ellipse(xpos[i], ypos[i], rad[i]);
}
pop();
push();
translate(width/2, height/2);
for (var i = 0; i < xpos.length; i++) {
fill('MAGENTA');
ellipse(xpos[i], ypos[i], rad[i]);
}
noLoop();
}