//Patrick Fisher, Section B, wpf@andrew.cmu.edu Assignment -11-project
var trackLines = []; //array for converyer belt
var robots = []; //array for the robots
function setup() {
createCanvas(480,300);
background(219.219,211);
for(var i = 0; i <= 9; i++){
trackLines[i] = i*48; //fills the track array
}
for(var i = 0; i <= 5; i++){
robots[i] = makeRobot(i); //fills the robot array
robots[i].centerX = -50 + (i*-200);
}
}
function draw(){
push();
stroke(0);
strokeWeight(4);
fill(54,54,66);
rect(0,50,width,200);
fill(28,150,195);
rect(0,25,width,25);
rect(0,250,width,25);
stroke(0);
strokeWeight(3); //draws the lines and moves them forward
for(var i = 0; i <= 9; i++){
line(trackLines[i],50,trackLines[i],250);
trackLines[i] += 1;
if(trackLines[i] >= width){ //sends a line back to the start when it gets to big
trackLines[i] = 0;
}
}
pop();
for(var i = 0; i <= 5; i++){
robots[i].draw(); //draws the robots
robots[i].centerX ++; //sends the robots forward
if(robots[i].centerX >= 800){ //deletes a robot from the array when it gets too far off screen and makes a new one
robots.shift();
robots.push(makeRobot(0));
robots[5].centerX = -400
}
}
}
function makeRobot(i) {
var rob = {centerX: 0, //funciton for making the robot
head: floor(random(0,3)),
eyes: floor(random(0,4)),
glowColor: clrSelect(floor(random(0,6))), //sends a random number to the color select function to set the color variable
mouth: floor(random(0,3)), //5
chest: floor(random(0,2)),
chestPiece: floor(random(0,3)), //8
arms: floor(random(0,3)), //4
legs: floor(random(0,3)), //3
draw: drawRobot,
drawHead: drawRobotHead,
drawBody: drawRobotBody,
drawEye: robotEyes,
drawMouth: robotMouth,
drawPiece: robotChestPiece,
drawArms: robotArms,
drawLegs: robotLegs,
}
return rob;
}
function drawRobot(){// draws the robot in fragments
this.drawHead();
this.drawBody();
}
function drawRobotHead(){
fill(101,108,127);
if(this.head == 0){
circle(this.centerX,90,60);
}
if(this.head == 1){
push();
rectMode(CENTER);
rect(this.centerX,90,60,60);
pop();
}
if(this.head == 2){
triangle(this.centerX,120,this.centerX-50,70,this.centerX+50,70);
}
this.drawEye();
this.drawMouth();
}
function drawRobotBody(){
fill(101,108,127);
if(this.chest == 0){
rect(this.centerX-25,120,50,75);
}
if(this.chest == 1){
ellipse(this.centerX, 157.5,50,75);
}
this.drawPiece();
this.drawArms();
this.drawLegs();
}
function robotEyes() {
push();
fill(this.glowColor);
if(this.eyes == 0){
circle(this.centerX + 15,85,20);
circle(this.centerX - 15,85,20);
}
if(this.eyes == 1){
push();
rectMode(CENTER);
rect(this.centerX + 15, 85, 15, 15);
rect(this.centerX - 15, 85, 15, 15);
pop();
}
if(this.eyes == 2){
push();
stroke(this.glowColor);
strokeWeight(3);
line(this.centerX + 20, 80 , this.centerX + 5, 80);
line(this.centerX - 20, 80 , this.centerX - 5, 80);
pop()
}
if(this.eyes == 3){
push();
stroke(this.glowColor);
strokeWeight(3);
line(this.centerX + 15, 90 , this.centerX + 15, 75);
line(this.centerX - 15, 90 , this.centerX - 15, 75);
pop()
}
pop();
}
function robotMouth() {
if(this.mouth == 0){
push();
stroke(this.glowColor);
strokeWeight(4);
noFill();
arc(this.centerX, 100, 20, 20, 0, PI);
pop();
}
if(this.mouth == 1){
push();
stroke(this.glowColor);
strokeWeight(4);
line(this.centerX + 10, 105, this.centerX -10, 105);
pop();
}
if(this.mouth == 2){
push();
fill(this.glowColor);
rect(this.centerX - 10, 101, 20, 8);
line(this.centerX -10, 105, this.centerX + 10, 105);
pop();
}
}
function robotChestPiece() {
if(this.chestPiece == 0){
push();
fill(this.glowColor);
circle(this.centerX, 147,20);
pop();
}
if(this.chestPiece == 1){
push();
fill(this.glowColor);
rectMode(CENTER);
rect(this.centerX, 147,20,20);
pop();
}
if(this.chestPiece == 2){
push();
fill(this.glowColor);
translate(this.centerX,147);
rotate(radians(45));
rectMode(CENTER);
rect(0,0,20,20);
pop();
}
}
function robotArms(){
if(this.arms == 0){
push();
stroke(0);
strokeWeight(2);
line(this.centerX + 25, 147, this.centerX + 50, 147);
line(this.centerX - 25, 147, this.centerX - 50, 147);
pop();
circle(this.centerX - 55, 147, 10);
circle(this.centerX + 55, 147, 10);
}
if(this.arms == 1){
ellipse(this.centerX+37.5,147,25,10);
ellipse(this.centerX-37.5,147,25,10);
}
if(this.arms == 2){
push();
rectMode(CENTER);
square(this.centerX+35,147,20);
square(this.centerX-35,147,20);
pop();
}
}
function robotLegs(){
if(this.legs == 0){
push()
stroke(0);
strokeWeight(2);
line(this.centerX + 20, 195, this.centerX + 20, 225);
line(this.centerX - 20, 195, this.centerX - 20, 225);
pop();
circle(this.centerX + 20, 230, 10);
circle(this.centerX - 20, 230, 10);
}
if(this.legs == 1){
ellipse(this.centerX - 15, 215, 10,40);
ellipse(this.centerX + 15, 215, 10,40);
}
if(this.legs == 2){
triangle(this.centerX-15, 197.5, this.centerX - 10, 235, this.centerX - 20, 235);
triangle(this.centerX+15, 197.5, this.centerX +10, 235, this.centerX + 20, 235);
}
}
function clrSelect(i){
if(i == 0){
var c = color(128,196,99); //green
}
if(i == 1){
var c = color(182,20,29); //red
}
if(i == 2){
var c = color(10,201,239); //blue
}
if(i == 3){
var c = color(217,16,207); //purple
}
if(i == 4){
var c = color(248,241,25); //yellow
}
if(i == 5){
var c = color(244,239,221); //off white
}
return c;
}
For my project, I did a robot factory line, with all the different parts of the robots changing. I started with getting the conveyor belt to look correct. Then I worked on making one look for the robot and getting it to loop continuously. Afterward, I started to separate the body parts into distinct functions and then implements the if statements to draw different types of body parts. I am very happy with how it worked out, I think the robots look kind of cute and I am happy with how seamless the loop is. I also think I have a good amount of variety so the robots do not get immediately boing.
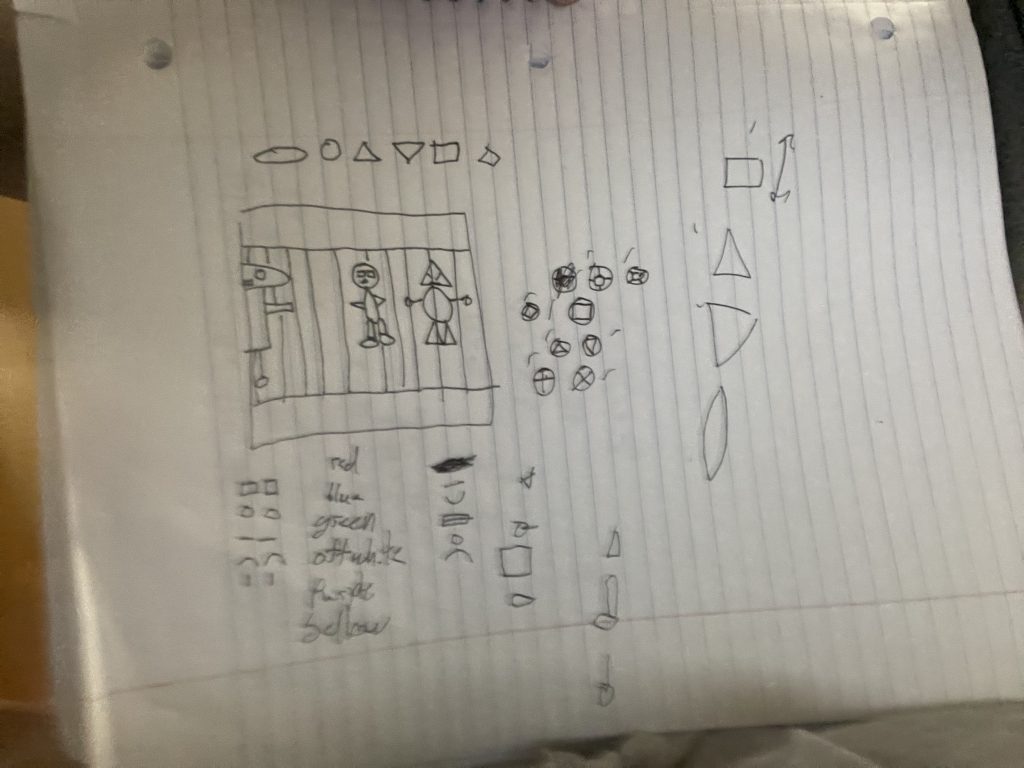