var col1;
var row1;
var col2;
var row2;
var col3;
var row3;
function setup() {
createCanvas(600, 400);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(88,24,69);
for (col2 = 50; col2 <=600; col2 += 100) {
for(row2 = -50; row2 <= 400; row2 += 100) {
stroke(218,247,166);
noFill();
ellipse(col2, row2 + 50, 100, 100);
}
noLoop();
}
for(col1 = 50; col1 <= 600; col1 += 200) {
for(row1 = 50; row1 <=600; row1 += 200) {
flower(col1,row1);
print(row1);
}
print(col1);
}
noLoop();
for(col3 = 150; col3 <= 600; col3 += 200) {
for(row3 = 150; row3 <= 600; row3 += 200) {
flower(col3, row3);
}
}
}
function flower(x,y) {
fill(199,0,57);
stroke(255,131,105);
push();
translate(x,y);
ellipse(25,0,50,30);
rotate(PI/3);
ellipse(25,0,50,30);
rotate(PI/3);
ellipse(25,0,50,30);
rotate(PI/3);
ellipse(25,0,50,30);
rotate(PI/3);
ellipse(25,0,50,30);
rotate(PI/3);
ellipse(25,0,50,30);
fill(199,0,57);
stroke(199,0,57);
ellipse(0,0,10,10);
pop();
}
Author: eomay
Looking Outwards- 3D Rendering
After exploring some different kinds of 3D generated art, I chose to focus on architectural rendering. The image above is from Archicgi, an architectural rendering studio. Here’s a great article about their process: https://archicgi.com/3d-architectural-rendering-101-a-definitive-guide/
These types of renderings are very interesting to me because of the level of detail, as well as the realism that they present. The article mentions their use of various softwares, including Maya and Blender, which are popular 3D rendering tools that I’ve heard of before. But they also mention some that I haven’t heard of such as V-Ray, Cinema 4D, and Revit. I would be interested to explore these a little more.
I’m sure there are teams of artists that go into creating these images, but I think there is a lot to say about artistic vision in the process. The architecture firm can send the studio their draftings of the building, but I believe the studio is responsible for making it look good. For example, the lighting in the image above has a huge impact on the building, and makes it look very dramatic. If the lighting were flat, the building probably wouldn’t look as interesting.
Project 4- String Art
//Eva Oney
//eoney
//section C
var c1 = 0;
var c2 = 255;
function setup() {
createCanvas(400, 300);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(0);
//loop for lines coming off the x axis
for (var i = 1; i <= 500; i += 5) {
stroke(255,0,0);
//I found my x and y values by originally setting x1 and x2 to mouseX
//and mouseY, then when I found a position I liked, I printed the value
//of mouseX and mouseY and got (15,400)
line(400 + i, 0, 15 +i, 150);
line(15 + i, 150, 400 +i, 400);
}
//loop for lines coming off the y axis
for(var i = 0; i <=500; i +=5) {
stroke(0,0,255);
line(0, 400 + i, 200, 15 + i );
line(400, 400 + i, 200, 15 +i);
}
noLoop();
}
I kept my code pretty simple so I could focus on understanding how the loops and parameters actually affect the image. I am not a math wiz by nature, so it is a little difficult for me to understand but I am slowly getting the hang of it.
Looking Outwards- Sound
The project that I decided to look at was Expressions, by Kynd and Yu Miyashita. I found the video to be captivatingly intricate, and I was shocked when I read that it was all digitally rendered. I was even more shocked when I found out it was rendered in 2D, using various layering and shading techniques as opposed to 3D vectors.
The images themselves were really cool, but the soundscape that went with it really elevated the experience. A brief warning if you are going to watch it, make sure to turn your sound down. I got blasted with an intense high pitched screech in my ear right off the bat (which was cool… but scary). I thought the interactivity between the sound and visuals was really compelling.
When reading about the piece at: https://www.creativeapplications.net/sound/expressions-paint-and-pixel-matiere-at-micro-scale/
I learned that the developer used WebGL which is a JavaScript api, made to render 2D and 3D graphics, and then went in on TouchDesigner to add detail. When looking at WebGL samples, I found that they are very similar to what we are doing now. I also know that we can use WebGL in p5.js, so maybe they’re the same thing? It was hard to find information on it. But anyways, it was interesting to see what I could do in the future if I keep up with programming.
Project 3- Dynamic Drawing
var x;
var y;
var strokeValue;
var mx;
var my;
var dragging
function setup() {
createCanvas(600, 450);
background(0);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
//center point variables
let x = width/2;
let y = height/2;
//stroke color variable
let strokeValue = 255;
//map of black screen ellipse
let mx= map(mouseX, 0, width, 0, 600);
//allows for ellipse to drag (which I just realized
//is pointless for this code)
if(dragging == true) {
x=mouseX;
y=mouseY;
}
//redraws ellipse with no background
if (mouseIsPressed) {
stroke(strokeValue);
noFill();
ellipse(x, y, (mouseX), (mouseY));
fill(0);
//draws two ellipses on black screen, one is mapped
} else {
background(0)
stroke(mouseX,0,mouseY);
noFill();
ellipse(mouseX, mouseY, mouseX, mouseY);
ellipse(mx, y, mouseX, mouseY);
text('press :)', mouseX, mouseY);
}
}
//changes background value to current value of mouseX,mouseY
function mousePressed() {
background(mouseX,0,mouseY);
dragging = true;
}
//changes dragging to false
function mouseReleased() {
dragging = false;
}
Originally, I wanted to do something really cool with infinitely generating circles. That idea morphed into this fun, interactive circle drawing. You can click anywhere on the canvas to generate a new background color based on the location of your mouse, and draw cool circles!
I’ll admit I struggled a lot with this project, but overall I am happy with what I came up with. I know it’s maybe not as complex as what we’re aiming for, however I learned a lot by creating it.
Generative Art
I really like this piece by Sawako & Panagiotis. I found them from the Scripted By Purpose page. https://scriptedbypurpose.wordpress.com/participants/akt/
They are computational design researchers, who tie programming and architecture to generate infrastructure. I picked this image in particular because it looks like something I could try to recreate in p5.js if I spent a good amount of time working on it.
The piece seems like it consists of one string of a manipulated shape being repeated over and over again, similarly to if you were to draw a series of ellipses or rectangles without redrawing the background. I’m sure a lot more went into rendering this image than just that, but those are my initial thoughts. I’m curious about what sort of functions would create the manipulation of the shape? I am not a numbers person, so navigating this type of computational art is a challenge.
Generative Art: Meet Your Creator (2012)
The project that caught my eye is Meet Your Creator, which was a live performance at the Cannes International Festival of Creativity in 2012. As a student in stage design, I found this project particularly interesting, and a little easier to understand from a programming perspective.
When programming stage lights, we use certain softwares that already contain code for our specific needs, and program individual instructions for each light. These instructions are called cues, and during a live performance, there will be a person operating a console that runs the software, launching each cue throughout the performance.
Meet Your Creator is a little more complex from a design standpoint because this show involved the use of several drones which were programmed to execute aerial movements. I’m not familiar with how these would be programmed, however there is a screenshot from this project showing the software used for the drones, and you can see some ‘else if’ statements!
http://www.memo.tv/works/meet-your-creator/
Memo Akten: Show Director
Project 1: Self Portrait
function setup() {
createCanvas(1000, 800);
background(255, 255, 0);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
stroke(255, 255, 0);
strokeWeight(8);
fill(255,153,153)
square(225,100,600); //hair
stroke(191,206,255)
fill(191,206,255) ;
ellipse((width/2),410 ,500,550); //head
fill(255,71,154);
circle(350,400,100);
circle(600,400,110); // eyes
fill(255,255,255);
ellipse(340,375,100,75);
ellipse(590,375,110,80);
arc((width/2), 525, 100, 100, 2 * PI, PI); //mouth
stroke(0)
noFill();
square(275,325,150,20)
square(525,325,150,20) //glasses
line(425, 400, 525, 400)
noLoop()
}
LO Inspiration
When thinking about computational art, the first project that comes to mind is the Immersive Van Gogh exhibit that is travelling around the world. It is a digital exhibit comprised of video and light that brings the work of Van Gogh to life.
The exhibit was created by Massimiliano Siccardi, a video artist. The creative team also consists of animators, graphic designers, and producers. It has been showing since 2008, with many evolutions and upgrades throughout the years. It is hard to find information on how the show was programmed, but I assume it took a lot of different programs and media to create since it involves video, photography, sound, lighting, virtual reality, and animation. Different rooms of the exhibit even have different smells.
So far, the exhibit continues to travel. There are also rumors that Massimiliano Siccardi is developing a similar exhibit inspired by Monet.
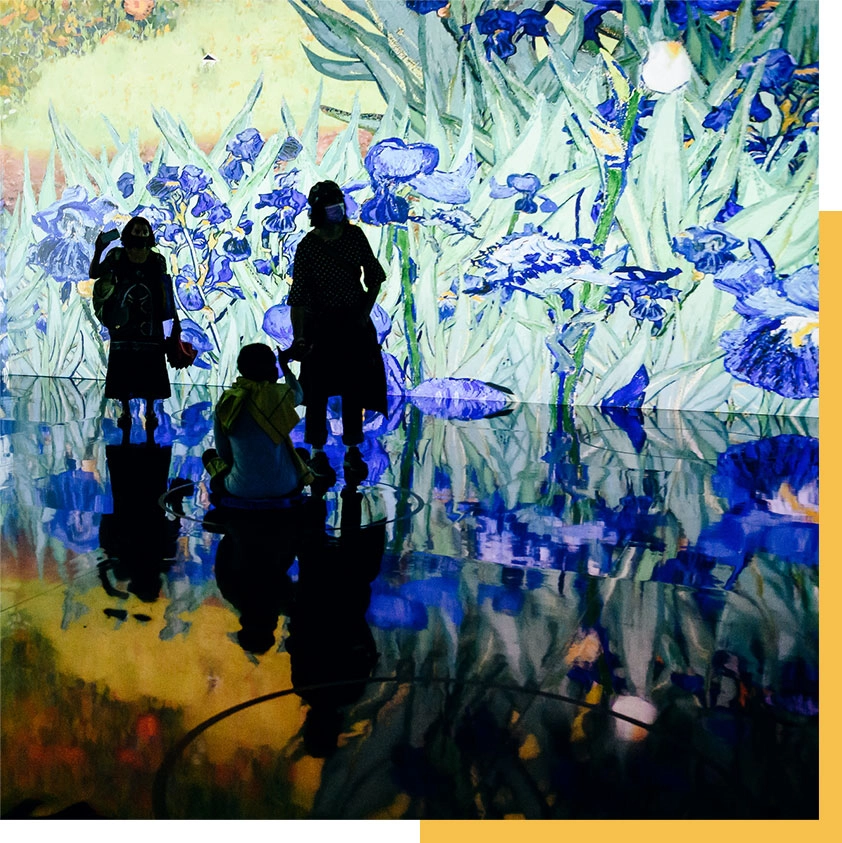