//Jai Sawkar
//Section C
//jsawkar@andrew.cmu.edu
//Project 07: Abstract Clock
function setup() {
createCanvas(480, 480);
frameRate(60);
}
var pts = 200;
function draw(){
background(0);
// using push & pull to orient the Bicorn
push()
translate(width/2, height/2) //center focused
drawCurve();
pop();
push();
rotate(PI); //upside down
translate(-width/2, -height/2);
drawCurve();
pop();
push();
rotate(PI/2); // clokcwise
translate(width/2, -height/2)
drawCurve();
pop();
push();
rotate(-PI/2); //counter clockwise
translate(-width/2, height/2)
drawCurve();
pop();
}
function drawCurve(){
//fill(255)
noFill();
stroke(255)
//noStroke();
var g = map(mouseX, 0, width, 0, width/2); //moving the parameters of mouse x
var g = map(sq(mouseY), 0, height, 0, 1); //moving parameters of mouse y
beginShape();
for (i = 0; i < pts; i++){
var j = map(i, 0, pts, 0, radians(360));
x = g * sin(j); //forumla
y = (g * cos(j) * cos(j) * (2+ cos(j))) / (3 + sin(j) * sin(j)); //formula
var w = map(mouseX, 0, height, 1, 70) //remapping width
var h = map(mouseY, 0, width, 1, 60) //remapping heigth
ellipse(x, y, w, h); //ellipse
}
endShape(CLOSE);
}
For this project, I found the Bicornal Curve interesting, so I decided to explore its possibilities. Once I drew it using the math equation provided, I found it a tad dull. I then decided to create 3 more, creating 4 bicornal curves
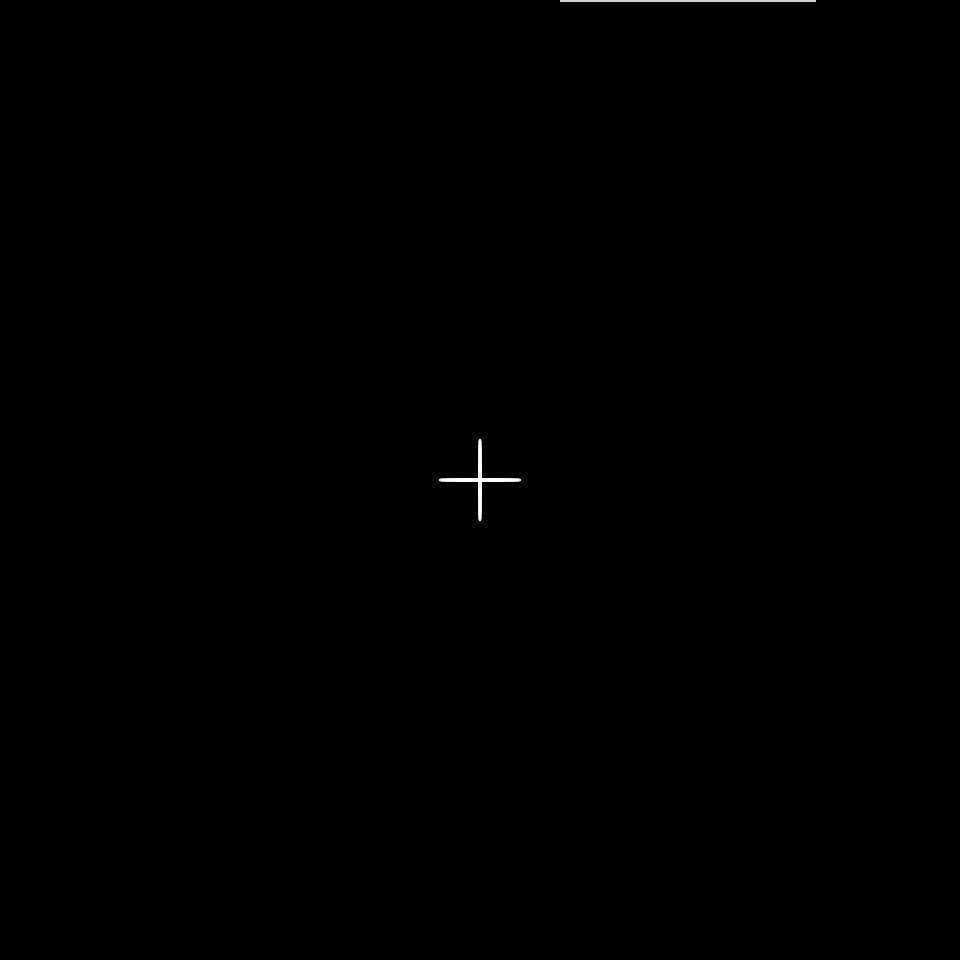
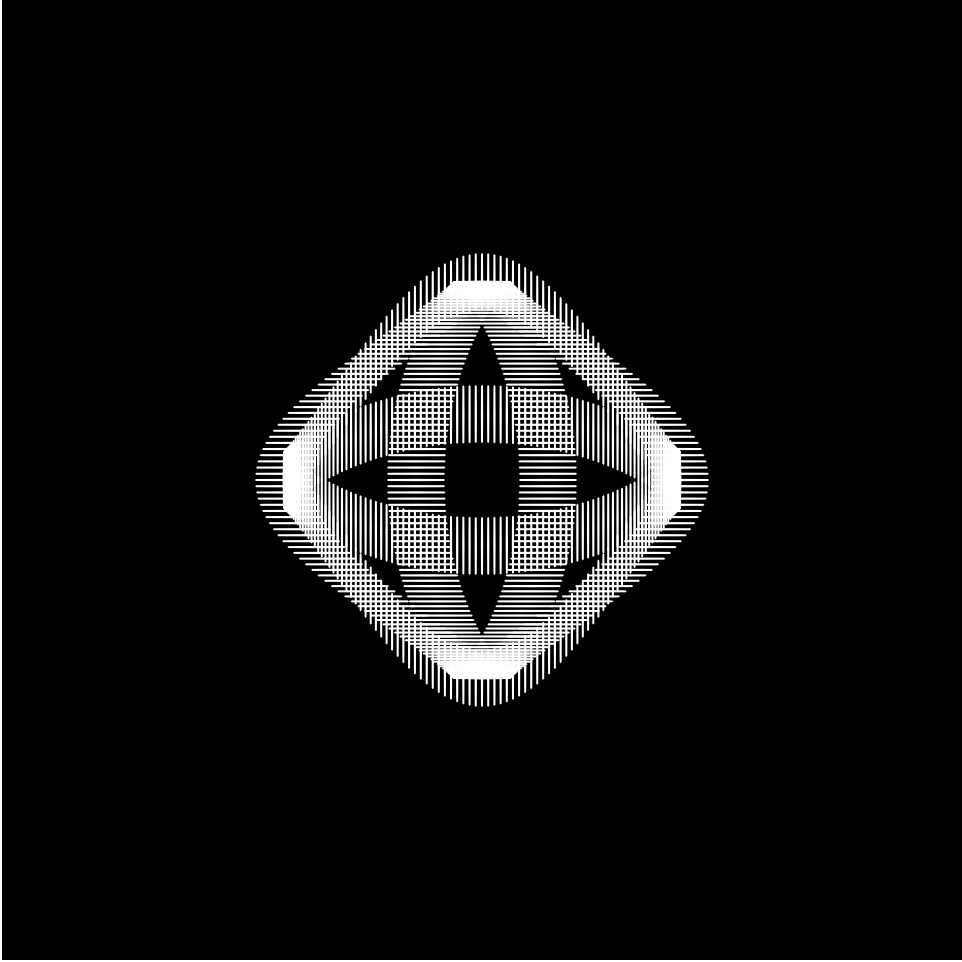
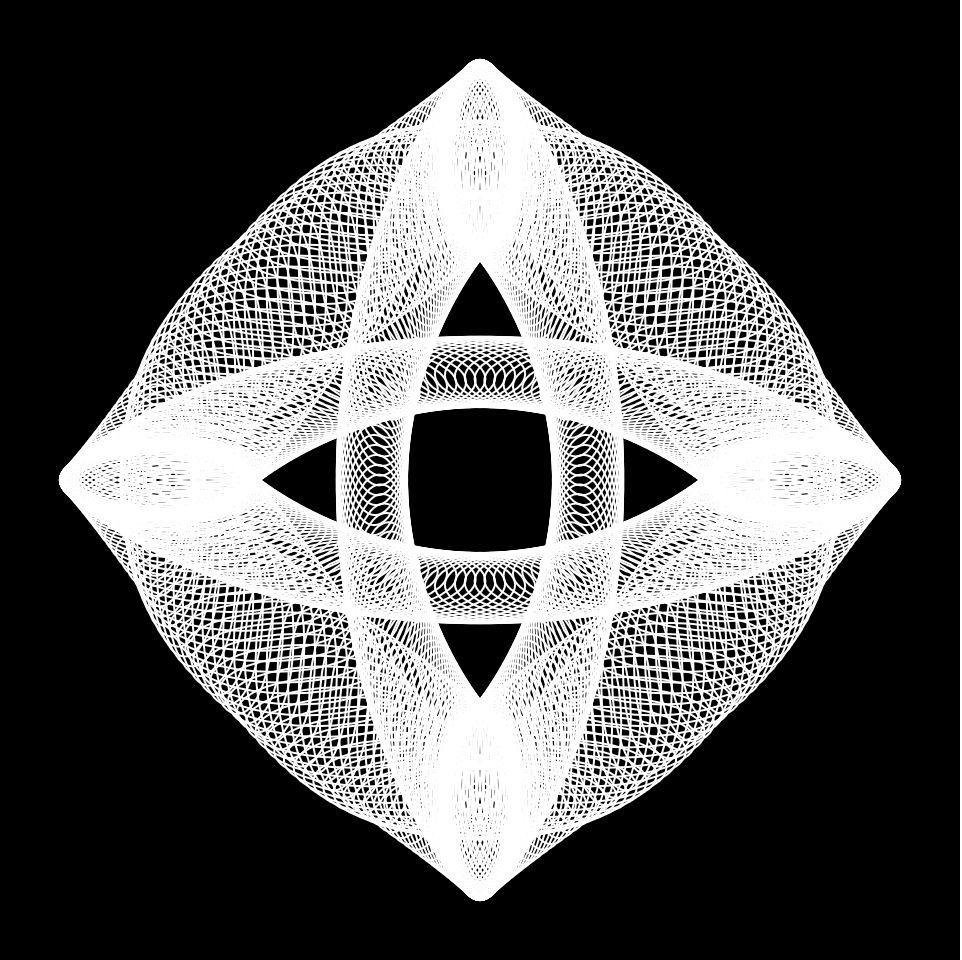
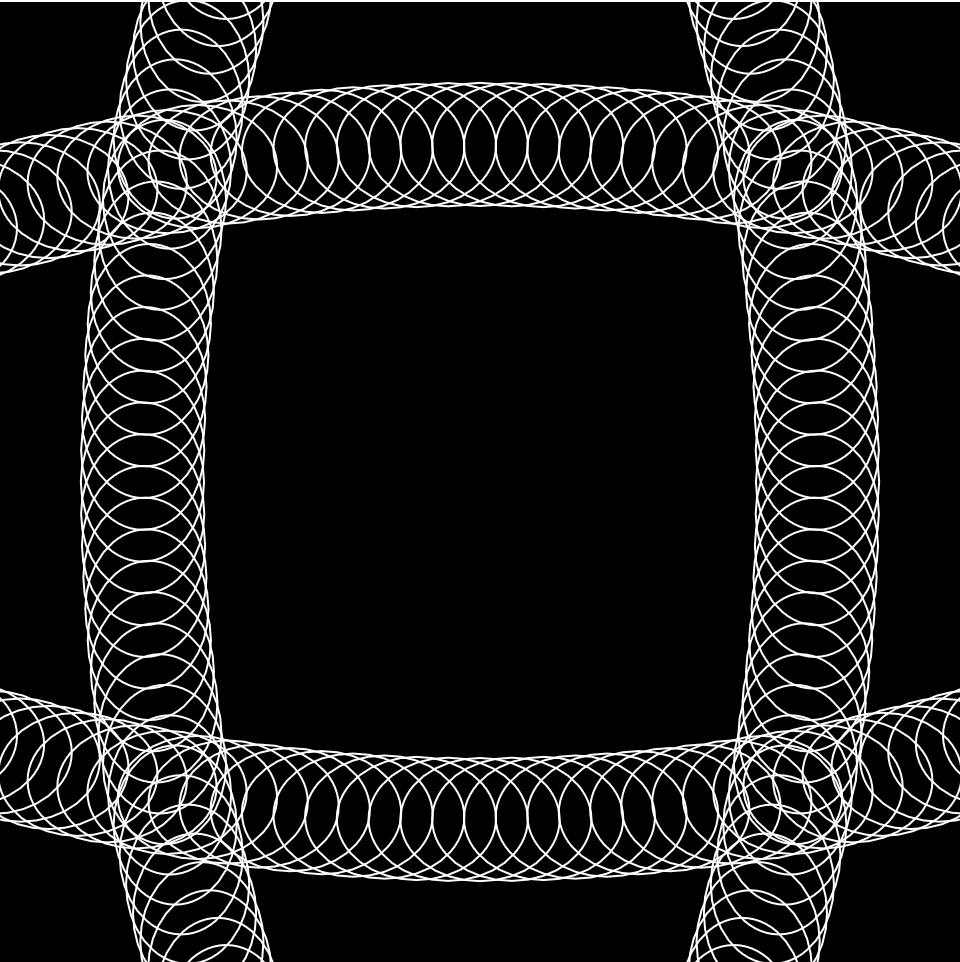