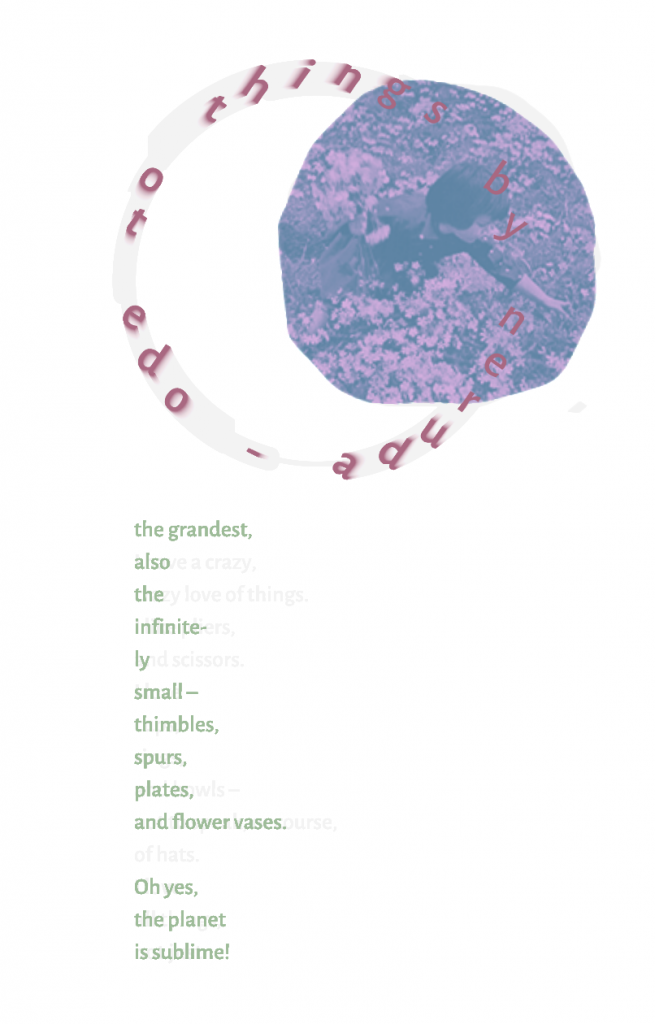
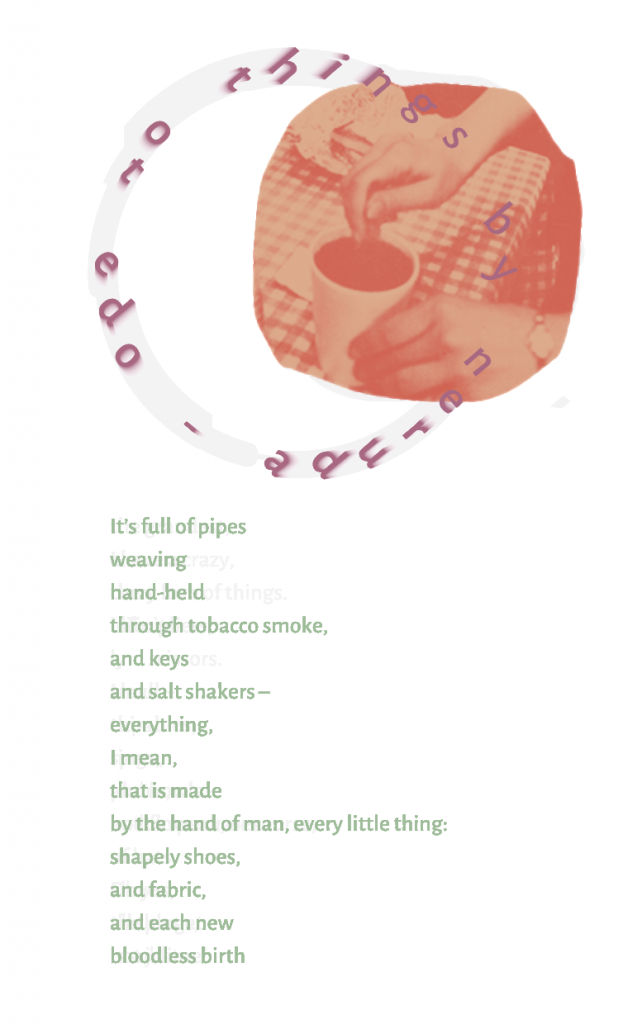
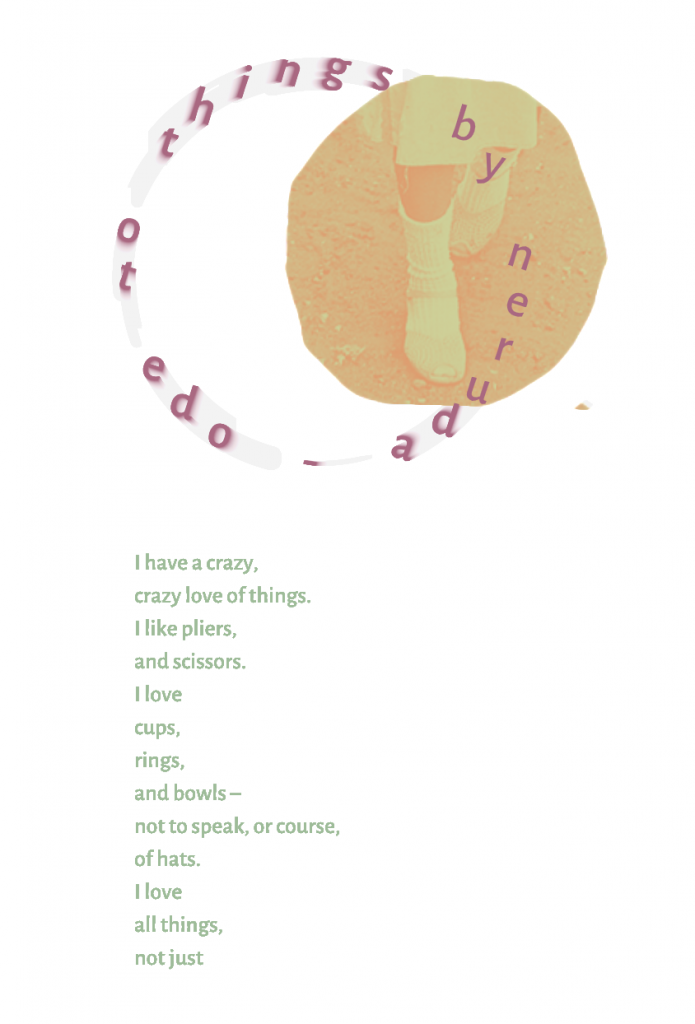
For my final project, I really wanted to work with text and explore alternate ways of moving through a poem. I chose to focus on one of my favorite poets, pablo neruda, and chose his poem, Ode to Things, as it has a lot of beauty to it.
// ilona altman
// final project
// section a
// iea @ andrew.cmu.edu
// this is a poem showcase of
var odetothings;
let fromHere;
let toHere;
let thefont; //allegra sans from GoogleFont
let poemletters = []; //array of letters
let poem = "ode to things by neruda - "; // title of the poem
let reversedpoem = reverseString(poem); // reversed
let nextimageCount; //image counter
let imagelinks= ['https://i.imgur.com/EiIMWxn.png',
'https://i.imgur.com/XCmsYws.png',
'https://i.imgur.com/fQZf2Sc.png'];
let imagesofthings = []; // image array
let currentThingImage;
function preload() {
//loading the text and the poem
odetothings = loadStrings("https://courses.ideate.cmu.edu/15-104/f2019/wp-content/uploads/2019/12/ode-2.txt");
//thefont = loadFont('thefont.ttf'); (cannot figure out hot to upload font for WordPress)
//loading the images
for (l = 0; l < imagelinks.length; l++) {
imagesofthings[l] = loadImage(imagelinks[l]);
}
}
function setup() {
createCanvas(450,640);
//initializing variables
fromHere = 0;
toHere = 14;
nextimageCount = 0;
nextimage = 0;
//initial placement for letters in a circle
for (let i = 0; i < poem.length; i ++) {
var radius = 120;
var angle = i * TWO_PI/poem.length;
var inputX = radius * sin(angle);
var inputY = radius * cos(angle);
var inputL = reversedpoem.charAt([i]);
poemletters[i] = makeLetters(inputL, inputX, inputY);
}
}
function draw() {
background(255,255,255);
//images rotating
push();
imageMode(CENTER);
rotate(frameCount/10000);
currentThingImage = image(imagesofthings[nextimage],width/2,height/3);
pop();
//updating the position of the letters
Update();
// making sure that there is continuous text
if (toHere > odetothings.length){
fromHere = 0;
toHere = 14;
}
// the text on the bottom that runs through the poem
for (var i = fromHere; i < toHere; i++) {
textSize(14);
//textFont(thefont);
fill(150,190,150);
var placeY = 340 + (i%14) * 20
text(odetothings[i], width/4, placeY);
}
}
// reversing the string so the text is easier to read
function reverseString(str) {
var splitting = str.split("");
var reversing = splitting.reverse();
var joined = reversing.join("");
return joined;
}
// the object of each letter
function makeLetters(inputL, inputX, inputY) {
var ltr = {letter: inputL,
px: inputX,
py: inputY,
showing: showLetters}; // draw the letter
return ltr;
}
function showLetters() {
push();
translate(width/2, height/3.5);
rotate(frameCount/1000);
//textFont(thefont);
textSize(30);
textAlign(CENTER);
fill(180,100,130);
text(''+ this.letter, this.px, this.py);
pop();
}
function Update() {
for (var i = 0; i < poemletters.length; i++){
poemletters[i].showing();
}
}
// when the mouse is pressed, the text cycles through
function mouseClicked() {
fromHere = fromHere + 14;
toHere = toHere + 14;
nextimageCount = nextimageCount + 1;
nextimage = nextimageCount%3;
}