// CJ Walsh
// Section D
// cjwalsh@andrew.cmu.edu
// Project 11
// establishing variables for movement of environment
var oceanFloor = 0.002;
var oceanFloorSpeed = 0.0001;
var surface = 0.0008;
var surfaceSpeed = 0.0006;
var seaweed = 1;
var seaweedSpeed = 0.0005;
var coral = 0.06;
var coralSpeed = 0.0003;
var fish = [];
var fishNum = 20;
function setup() {
createCanvas(480, 480);
background(220);
for (i=0; i<fishNum; i++) {
f = makeFish(width+random(10, 100));
fish.push(f);
}
}
function draw() {
background('#A5F9F5');
drawNoise();
drawFishy();
updateFish();
}
// remove old fish and add new fish
function updateFish() {
keepFish = [];
for (i=0; i<fishNum; i++) {
f = fish[i];
if (f.x > 0-f.bodyW) {
keepFish.push(f);
}
else {
keepFish.push(makeFish(width+50));
}
}
fish = keepFish;
}
// draw the fish
function drawFishy() {
for (i=0; i<fishNum; i++) {
fish[i].move();
fish[i].display();
}
}
// drawing environment
function drawNoise() {
// Surface
beginShape();
fill('#516FCE');
noStroke();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * surface) + (millis() * surfaceSpeed);
var y = map(noise(t * 0.5), 0, 1, 0, 100);
vertex(x, y);
}
vertex(width, height);
endShape();
// coral
beginShape();
fill('#425689');
noStroke();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * coral) + (millis() * coralSpeed);
var y = map(noise(t * 0.5), 0, 1, 250, 300);
vertex(x, y);
}
vertex(width, height);
endShape();
// Seaweed
beginShape();
fill('#3E7C6A');
noStroke();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * seaweed) + (millis() * seaweedSpeed);
var y = map(noise(t * 0.5), 0, 1, 250, 480);
vertex(x, y);
}
vertex(width, height);
endShape();
// ocean floor
beginShape();
fill('#E5D9A8');
noStroke();
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * oceanFloor) + (millis() * oceanFloorSpeed);
var y = map(noise(t * 0.5), 0, 1, 350, 480);
vertex(x, y);
}
vertex(width, height);
endShape();
}
// creating an object for the fish
function makeFish(fishlx) {
var fsh = {x: fishlx,
y: random(height-350, height-50),
bodyW: random(30, 50),
bodyH: random(15, 40),
speed: random(-2, -0.5),
tail: random(15, 20),
fColor: [random(0,255), random(0,255), random(0,255)],
move: fishMove,
display: fishDisplay
}
return fsh;
}
// displaying the fish
function fishDisplay() {
fill(this.fColor);
noStroke();
tx = this.x + this.bodyW/3;
// drawing fish tail
triangle(tx, this.y, tx+this.tail, this.y+this.tail/2,
tx+this.tail, this.y-this.tail/2);
// drawing fish body
ellipse(this.x, this.y, this.bodyW, this.bodyH);
// drawing fish eye
fill(0);
ellipse(this.x-this.bodyW/4, this.y-this.bodyH/4, 4, 4);
}
// moving the fish across canvas
function fishMove() {
this.x += this.speed;
}
This project was super fun to make. The idea I started out with was wanting to create an underwater scene with fish and seaweed. Using noise, I was able to create the forms for the waterline, the ocean floor, seaweed and a coral/sea mountain background. After playing with the amount of noise and speed, I was able to create really cool movements with both the water and the seaweed. The surface looks like it undulates in waves like the ocean and the seaweed has a fun flowing motion. I then created the fish and randomized their size and color to create some fun forms. Im really happy with how it came together.
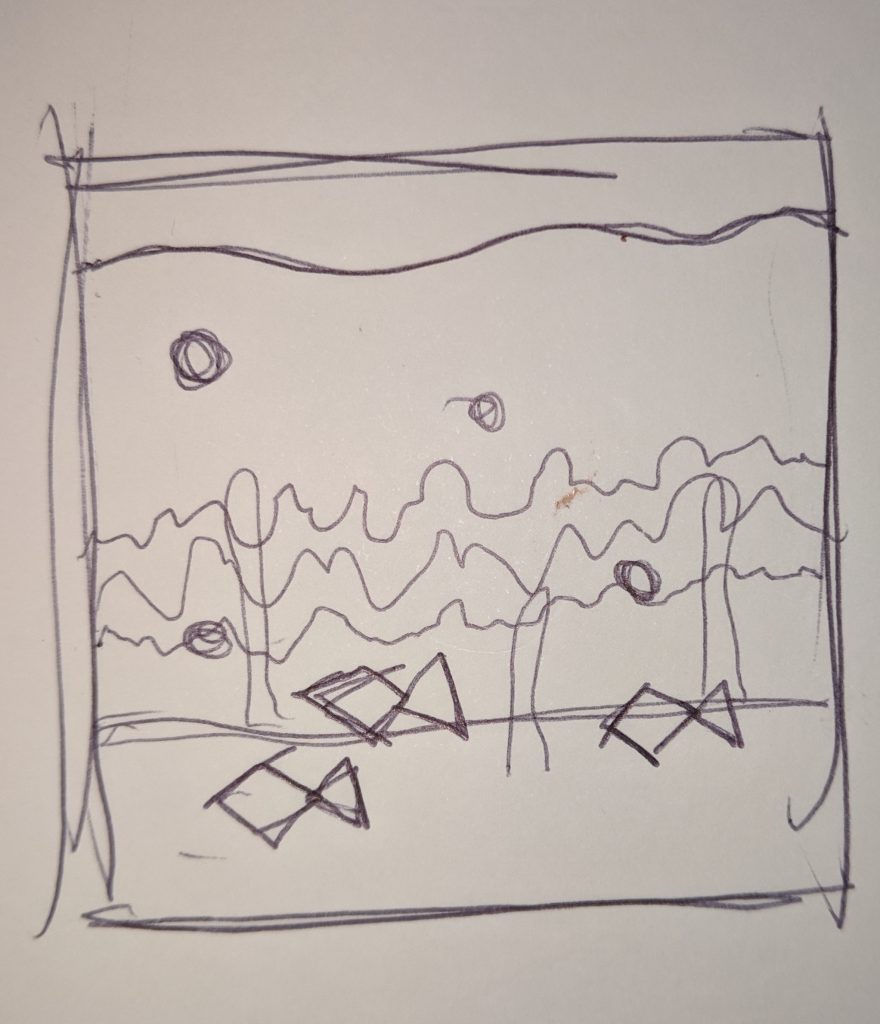