For my landscape project, I chose to make a basic underwater scene containing water, sand, bubbles, and fish. I think this project was a great opportunity to play around with objects and setting different types of parameters. I definitely came out of this with a much better understanding of how to use objects in code. One of the elements that I had trouble with is setting the color using the color() function (which is why I ended up using grayscale). Some things I wish I could have added are: coral/seaweed, other types of animals, and lines to represent water flow.
/* Claire Lee
15-104 Section B
seoyounl@andrew.cmu.edu
Project-11 */
var bubbles = [];
var seaweed = [];
var fish = [];
function setup() {
createCanvas(480, 240);
frameRate(10);
for (i = 0; i < 50; i++) {
bubbleX = random(width);
bubbleY = random(height);
bubbles[i] = blowBubbles(bubbleX, bubbleY);
}
for (k = 0; k < 30; k++) {
fishX = random(width);
fishY = random(height);
fish[k] = makeFish(fishX, fishY);
}
}
function draw() {
background(140, 225, 255);
showBubbles();
showFish();
showSand();
}
var sandSpeed = 0.0004;
function showSand() {
var sand = 0.01;
stroke(235, 220, 180);
strokeWeight(2)
beginShape();
for (var i = 0; i < width; i++) {
var x = (i * sand) + (millis() * sandSpeed);
var s = map(noise(x), 0, 1, 150, 200);
line(i, s, i, height);
// using map() and noise() function to generate randomized sand terrain
}
endShape();
}
function blowBubbles (bubbleX, bubbleY) {
// making bubble object
var bubble = {
x: bubbleX,
y: bubbleY,
radius: random(8, 12),
speed: -0.5,
float: floatBubbles,
draw: drawBubbles
}
return bubble;
}
function floatBubbles() {
// making the bubbles move horizontally and float
this.x += (this.speed * 2);
this.y += this.speed;
if (this.x <= 0) {
this.x = width;
}
if (this.y <= 0) {
this.y = height;
} // bubbles "pop" when they reach top of screen
}
function drawBubbles() {
push();
translate(this.x, this.y);
strokeWeight(1);
stroke(255, 255, 255, 90);
fill(160, 245, 255, 90);
ellipse(1, 10, this.radius, this.radius);
noStroke();
fill(255, 255, 255, 90);
ellipse(-1, 8, 2, 2);
pop();
}
function showBubbles() {
for (i = 0; i < bubbles.length; i++) {
bubbles[i].float();
bubbles[i].draw();
}
}
function makeFish(fishX, fishY) {
var fish = {
fx: fishX,
fy: fishY,
fishspeed: random(-3, -8),
fishmove: moveFish,
fishcolor: random(100, 200),
fishdraw: drawFish
}
return fish;
}
function moveFish() {
this.fx += this.fishspeed;
if (this.fx <= -10) {
this.fx += width;
}
}
function drawFish() {
var tailLength = 8;
noStroke();
fill(this.fishcolor);
ellipse(this.fx, this.fy, 10, 5);
triangle(this.fx + (tailLength / 2), this.fy, this.fx + tailLength, this.fy - 3, this.fx + tailLength, this.fy + 3);
}
function showFish() {
for (i = 0; i < fish.length; i++) {
fish[i].fishmove();
fish[i].fishdraw();
}
}
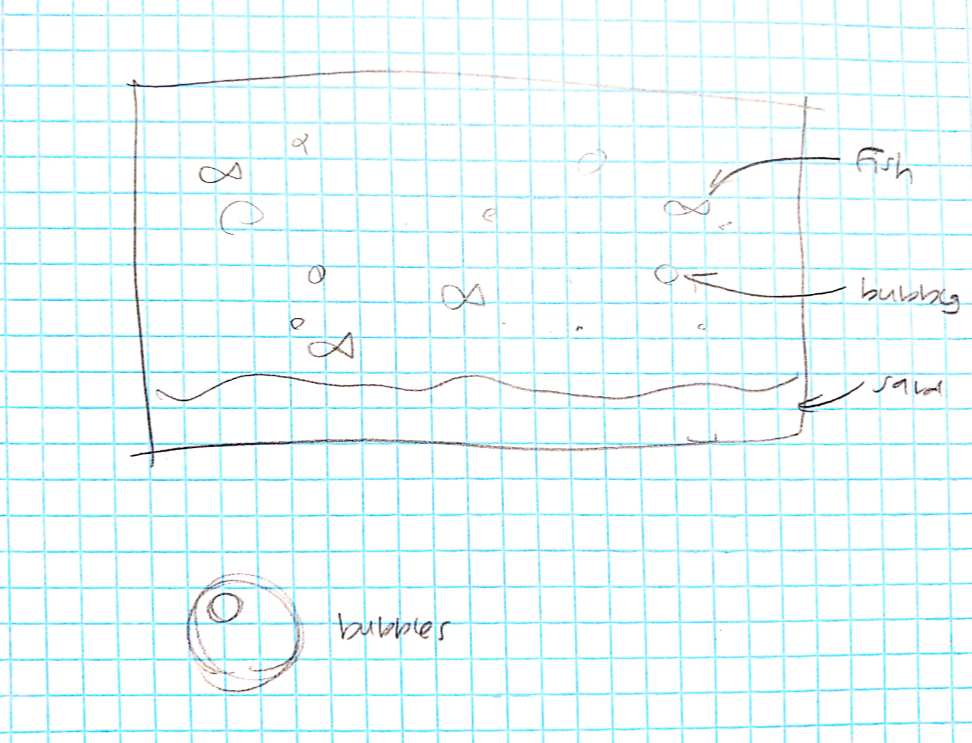
** I’m using a grace day for this project!