/* Rachel Shin
reshin@andrew.cmu.edu
15-104 Section B
Project 11 - Landscape
*/
var terrainSpeed = 0.0005;
var terrainDetail = 0.0195;
var terrainDetail1 = 0.04;
var stars = [];
function setup() {
createCanvas(400, 300);
frameRate(15);
for (var i = 0; i < 70; i++){
var starX = random(width);
var starY = random(0, height/4);
stars[i] = makeStar(starX, starY);
}
}
function draw() {
background(0);
displayStars(); //bottom layer
darkTerrain(); //next layer
terrain(); //second to top layer
bottomTerrain(); //top layer
}
function terrain() {
noStroke();
fill(220);
beginShape();
for (var x = 0; x < width; x ++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, 200, 100);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function darkTerrain() {
noStroke();
fill(17, 25, 36);
beginShape();
for (var x = 0; x < width; x ++) {
var t = (x * terrainDetail1) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 2, 0, 300);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function bottomTerrain() {
noStroke();
fill(255);
beginShape();
for (var x = 0; x < width; x ++) {
var t = (x * terrainDetail1) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, 50, 300);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function drawStar() {
noStroke();
fill(230, 242, 174);
push();
translate(this.x, this.y);
ellipse(5, 10, 5, 5);
pop();
}
function makeStar(starX, starY) {
var makeStar = {x: starX,
y: starY,
speed: -1,
move: moveStar,
draw: drawStar}
return makeStar;
}
function moveStar() {
this.x += this.speed;
if (this.x <= -10){
this.x += width;
}
}
function displayStars() {
for(i = 0; i < stars.length; i ++) {
stars[i].move();
stars[i].draw();
}
}
As a kid, I often went on trips to Reno or Lake Tahoe with my family and family friends. If I wasn’t playing video games in the car, I would stare at the mountains that we drove past and was mesmerized by the stars that I couldn’t see in Cupertino because of all the light pollution there. I decided to create 3 different terrains to create more depth in my production and put many stars in it to represent the countless number of stars that I saw during the drive.
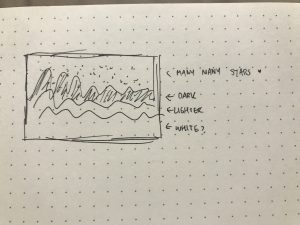