/*
Min Ji Kim Kim
Section A
mkimkim@andrew.cmu.edu
Project-07
*/
function setup() {
createCanvas(480, 480);
}
function draw () {
background(0);
drawHypotrochoid(); //draw the Hypotrochoid
drawHypocycloidPC(); //draw the Hypocycloid Pedal Curve
}
function drawHypotrochoid() { //http://mathworld.wolfram.com/Hypotrochoid.html
var a = int(map(mouseX, 0, width, 100, 200)); //radius of Hypotrochoid
var b = int(map(mouseY, 0, height, 50, 80)); //radius of circle b
var h = int(map(mouseX, 0, width, 40, 50)); //distance between center and point P of circle with radius b
//draw Hypotrochoid
push();
translate(width / 2, height / 2); //move center to middle of canvas
fill(150, mouseX, mouseY); //color changes depending on mouseX and mouseY
beginShape();
for(var i = 0; i < 100; i++) {
var tH = map(i, 0, 100, 0, TWO_PI); //map theta angle
var x = (a - b) * cos(tH) + h * cos((a - b) * tH); //parametric equations
var y = (a - b) * sin(tH) - h * sin((a - b) * tH);
vertex(x, y);
}
endShape();
pop();
}
function drawHypocycloidPC() { // http://mathworld.wolfram.com/HypocycloidPedalCurve.html
var a = int(map(mouseY, 0, height, 30, 80)); //map the radius with mouseX movement
var n = int(map(mouseX, 0, width, 5, 20)); //map the number of cusps with mouseX movement
//draw Hypocycloid Pedal Curve
push();
translate(width / 2, height / 2); //move center to middle of canvas
fill(mouseY, 100 , 120); //color changes depending on mouseY
beginShape();
for(var i = 0; i < 500; i++) {
var t = map(i, 0, 500, 0, TWO_PI); //map theta angle
var x = a * ((n - 1) * cos(t) + cos((n - 1) * t)) / n; //pedal curve equations
var y = a * ((n - 1) * sin(t) + sin((n - 1) * t)) / n;
vertex(x, y);
}
endShape();
pop();
}
I think this was the hardest project to do so far. I didn’t really understand the assignment so I just started by looking at the curves on MathWorld and I was really interested in the Hypotrochoid and the Hypocycloid Pedal Curves. I decided to use those for this project and layer them to create a flower like effect. I think the hardest part of this project was trying to figure out the formulas and how to manipulate the variables but once I got it, it was fun to play around with the shapes and colors. I used mouseX and mouseY to manipulate the variables and the colors to create pretty flowers.

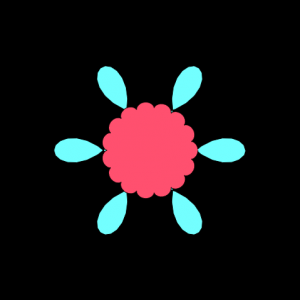
