/*
Yanina Shavialenka
Section B
yshavial@andrew.cmu.edu
Project 5: Wallpaper
*/
var x = 100;
var y = 45;
var s = 8;
var xS1 = 193;
var yS1 = 60;
var xS2 = 193;
var yS2 = 115;
function setup() {
createCanvas(600, 600);
background(0, 0, 51);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(0, 0, 51);
//Hexagonal background
for(var xH = 0; xH <= 600; xH += 15){
for (var yH = 0; yH <= 600; yH += 2*s*sqrt(3)/2){
fill(0, 0, 51);
hexagon(xH, yH);
}
}
for (var xH = 10; xH <= 600; xH += 15){
for (var yH = s*sqrt(3)/2; yH <= 600; yH += 2*s*sqrt(3)/2){
fill(0, 0, 95)
hexagon(xH, yH);
}
}
//Solar Systems
for(x = 100; x <= width; x += 200){
for(y = 85; y <=height; y += 300){
solarSystem();
}
}
for(x = 0; x <= width; x += 200){
for(y = 235; y <= height; y += 300){
solarSystem();
}
}
//Yellow Stars
for(xS1 = -7; xS1 <= width; xS1 += 200){
for(yS1 = 45; yS1 <=height; yS1 += 300){
star();
}
}
for(xS1 = 93; xS1 <= width; xS1 += 200){
for(yS1 = 195; yS1 <= height; yS1 += 300){
star();
}
}
//Pink Stars
for(xS2 = -7; xS2 <= width; xS2 += 200){
for(yS2 = 115; yS2 <=height; yS2 += 300){
star();
}
}
for(xS2 = 93; xS2 <= width; xS2 += 200){
for(yS2 = 265; yS2 <= height; yS2 += 300){
star();
}
}
}
function star() {
/*
This function draws yellow and pink stars.
The star is made out of a square in the middle and 4
triangles that surround that square.
*/
//Yellow Star
noStroke();
fill(255, 255, 102);
rect(xS1, yS1, 13, 13);
triangle(xS1, yS1, xS1+6.5, yS1-13, xS1+13, yS1);
triangle(xS1, yS1, xS1-13, yS1+6.5, xS1, yS1+13);
triangle(xS1, yS1+13, xS1+6.5, yS1+26, xS1+13, yS1+13);
triangle(xS1+13, yS1, xS1+26, yS1+6.5, xS1+13, yS1+13);
//Pink Star
noStroke();
fill(255, 178, 153);
rect(xS2, yS2, 13, 13);
triangle(xS2, yS2, xS2+6.5, yS2-13, xS2+13, yS2);
triangle(xS2, yS2, xS2-13, yS2+6.5, xS2, yS2+13);
triangle(xS2, yS2+13, xS2+6.5, yS2+26, xS2+13, yS2+13);
triangle(xS2+13, yS2, xS2+26, yS2+6.5, xS2+13, yS2+13);
}
function solarSystem() {
/*
This function draws Solar System with sun in the middle
and planets rotating around the sun on their orbits.
*/
//Orbits
stroke(255);
fill(0, 0, 0, 0);
ellipse(x, y, 25, 25);
ellipse(x, y, 45, 45);
ellipse(x, y, 65, 65);
push();
strokeWeight(3);
stroke(180);
ellipse(x, y, 85, 85);
pop();
ellipse(x, y, 105, 105);
ellipse(x, y, 125, 125);
ellipse(x, y, 145, 145);
ellipse(x, y, 165, 165);
//Sun
noStroke();
fill(255, 255, 0);
ellipse(x, y, 12, 12);
//Venus
noStroke();
fill(160, 160, 160);
ellipse(x+8, y+10, 8, 8);
//Earth
noStroke();
fill(0, 102, 204);
ellipse(x+10, y-20, 13, 13);
fill(0,220,0);
ellipse(x+11, y-22, 8, 4);
ellipse(x+11, y-17, 4, 2.5);
ellipse(x+7, y-19, 5, 2);
//Mars
noStroke();
fill(204, 65, 0);
ellipse(x-30, y-10, 12, 12);
//Jupiter
noStroke();
fill(255, 155, 0);
ellipse(x+40, y+35, 18, 18);
stroke(195, 0, 0);
line(x+35, y+28, x+48, y+34);
stroke(205, 50, 0);
line(x+32, y+33, x+46, y+40);
//Saturn
stroke(255);
fill(0, 0, 0, 0);
ellipse(x+37, y-50, 30, 9);
noStroke();
fill(190, 190, 190);
ellipse(x+37, y-50, 16.5, 16.5);
//Uranus
noStroke();
fill(153, 204, 255);
ellipse(x-53, y+50, 15, 15);
//Neptune
noStroke();
fill(10, 76, 153);
ellipse(x-45, y-70, 15, 15);
}
function hexagon(xH,yH){
/*
This function draws one hexagon with a side of 8 pixels
that is made out of 8 triangles.
*/
push();
translate(xH,yH)
noStroke();
triangle(0,0,-s,0,-s/2,-s*sqrt(3)/2)
triangle(0,0,-s/2,-s*sqrt(3)/2,s/2,-s*sqrt(3)/2)
triangle(0,0,s,0,s/2,-s*sqrt(3)/2)
triangle(0,0,-s,0,-s/2,s*sqrt(3)/2)
triangle(0,0,-s/2,s*sqrt(3)/2,s/2,s*sqrt(3)/2)
triangle(0,0,s,0,s/2,s*sqrt(3)/2)
pop();
}
|
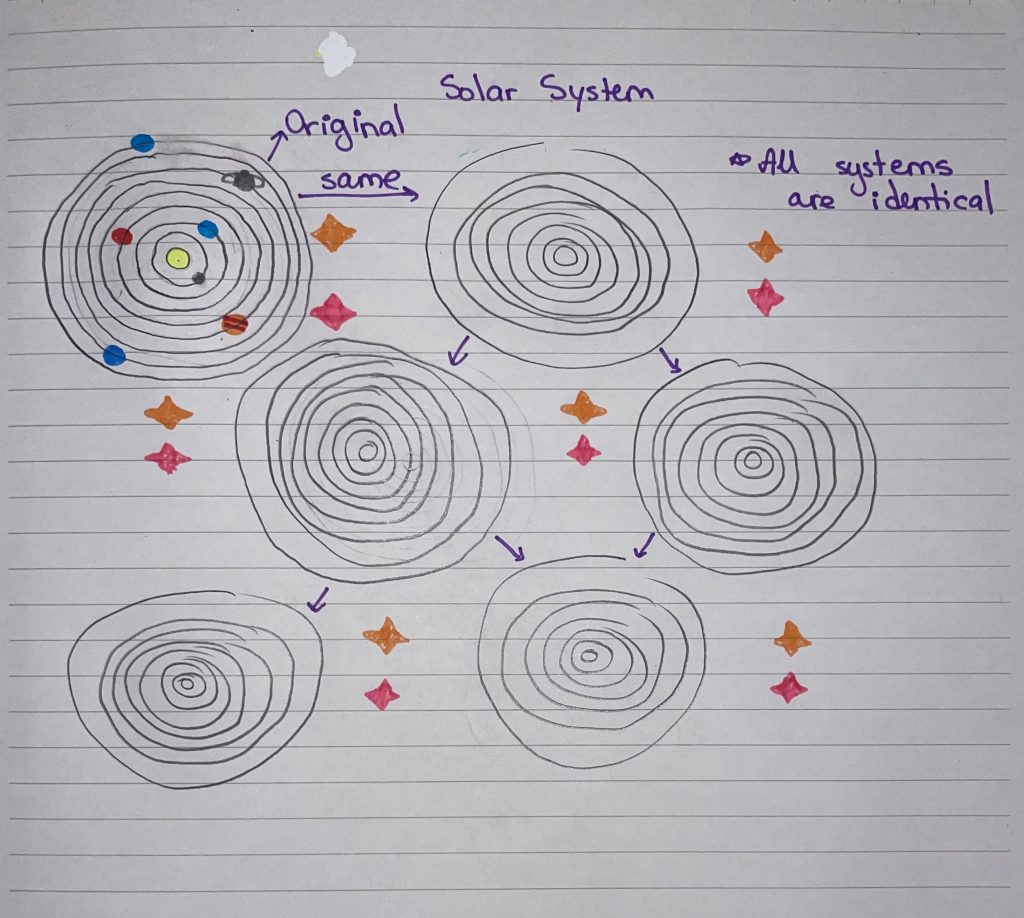
This project was very interesting in the fact that I get to design whatever I want which also made this project hard as if it was hard to stop on one idea only. I came up with the idea of Solar system with planets surrounding a sun in the middle. All of the systems are identical and are separated by yellow and pink stars.