// project 06
// gnmarino@andrew.cmu.edu
// Gia Marino
// Section D
var theta = 0; // references theta in draw function only
var m; // current minute
var h; // current hour
function setup() {
createCanvas(480, 480);
background(255);
}
function draw() {
translate(width/2, height/2);
// rotates whole clock
rotate(radians(theta));
// draws the whole clock
NightAndDay(); // draws the night and day sides of the circle
sun(0, height/2 - 80);
moon(0, -height/2 + 80);
clouds(width/2 - 25, 0);
diamonds(-width/2 + 25, 0);
// takes current time and calculates how much to rotate the clock
// every minute the clock should turn .25 degrees
// every hour the clock should have rotated 15 more degrees
m = minute();
h = hour();
theta = (h * 15) + (m * .25);
}
function sun(sunX, sunY) {
push();
// orangey yellow color for sun
fill(253, 208, 25);
// moves sun to coordinates stated in draw function
translate(sunX, sunY);
circle(0, 0, 50); // middle of sun
// strokeWeight and loop makes the sun rays
strokeWeight(2);
for (theta = 0; theta < 360; theta += 45) {
rotate(radians(theta));
line(30, 0, 37, 0);
}
pop();
}
function moon(moonX, moonY) {
push();
fill(220); // light grey
// moves moon to coordinates stated in draw function
translate(moonX, moonY);
circle(0, 0, 50);
pop();
}
function diamonds(diamondX, diamondY) {
push();
noFill();
strokeWeight(5);
// counter keeps track how many times the for-loop loops
// needs to start at 12 so the diamonds don't fill in when it's before noon
var counter = 12;
// loop rotates and draws diamonds
for (theta = 7; theta < 177; theta += 15) {
push();
rotate(radians(theta));
// moves diamonds to coordinates stated in draw function
translate(diamondX, diamondY)
// scale & rotate are used to make diamonds look better along the border
scale(.25);
rotate(radians(90));
// if statement says the diamonds should be filled in based on the hour
// diamond fills in after every hour passes
// if clock hasn't passed that hour yet then diamond stays unfilled
// diamonds fill in for 1pm to 12 am
if (counter < h) {
fill(255);
} else {
noFill();
}
// draws 1 diamond every time
beginShape();
vertex(-20, 0);
quadraticVertex(-10, -5, 0, -30);
quadraticVertex(10, -5, 20, 0);
quadraticVertex(10, 5, 0, 30);
quadraticVertex(-10, 5, -20, 0);
endShape();
pop();
counter += 1; // adds 1 everytime loop ends
}
pop();
}
function clouds(cloudX, cloudY) {
push();
strokeWeight(5);
noFill();
// counter keeps track how many times the for-loop loops
// counter starts at zero so clouds starts filling after 1st hour has passed
var counter = 0;
// loop rotates and draws clouds
for (theta = 7; theta < 177; theta += 15) {
push();
rotate(radians(theta));
// moves clouds to coordinates stated in draw function
translate(cloudX, cloudY);
// scale & rotate are used to make clouds look better along the border
scale(.25);
rotate(radians(90));
// if statement says the clouds should be filled in based on the hour
// cloud fills in after every hour passes
// if clock hasn't passed that hour yet then cloud stays unfilled
// clouds fill in for 1 am to 12 pm
if (counter <= h) {
fill(255);
} else {
noFill();
}
// draws 1 cloud every time
beginShape();
vertex(0, 0);
quadraticVertex(-45, 5, -40, -10);
quadraticVertex(-35, -30, -15, -20);
quadraticVertex(-20, -40, 0, -40);
quadraticVertex(20, -40, 15, -20);
quadraticVertex(35, -30, 40, -10);
quadraticVertex(45, 5, 0, 0);
endShape();
pop();
counter += 1; // adds 1 everytime loop ends
}
pop();
}
function NightAndDay() {
push();
// yellow half circle represents day time
fill(255, 238, 127); // yellow
arc(0, 0, width, height, radians(0), radians(180));
// navy half circle represents night time
fill(60, 67, 129); // navy
arc(0, 0, width, height, radians(180), radians(360));
pop();
}
For this assignment I actually got inspiration from the Minecraft clock because it is the first example I could think of for a non-western standard clock.
I started off by deciding that I wanted the daylight half circle to be fully on the top and vice versa with the night side.
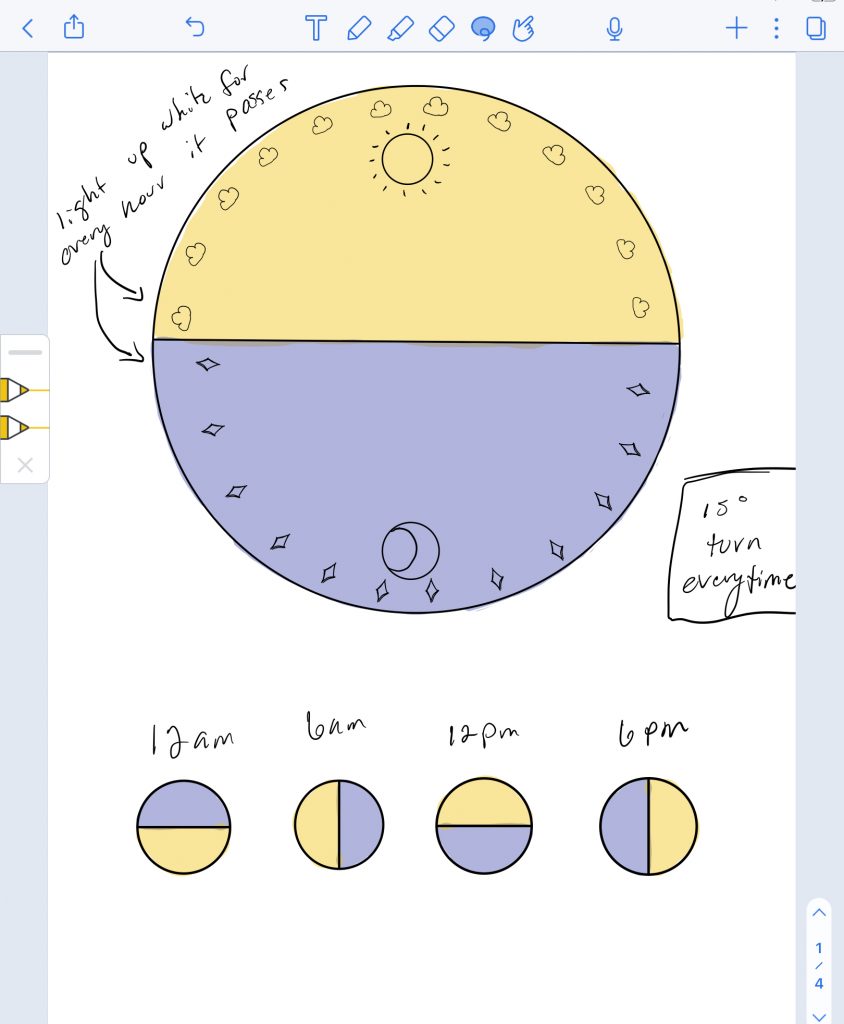
I ended up deciding that I wanted the clock to emulate how the sky typically looks if you are at a reasonable latitude on earth. So, when it is noon and the sun is highest in the sky then I want the clock’s yellow side to be fully on top so it looks like what you’d see if you’d looked outside at noon. It also kinda shows when the sun rises and sets too.
Lastly, I decided that I wanted someone to be looking at my clock and know what time it is and not completely guess. So, I made little hour symbols (the clouds and diamonds) light up every time an hour passes. This makes it when you look at the clock you can count how many clouds or diamonds are white and know it’s been x amount of hours.