My project was pretty tricky to make. Trying to get the ellipses to expand and stop at different points took a while, and I had to settle for them all moving at once, and there was a lot of trial and error. My end result is a Day to Night transition.
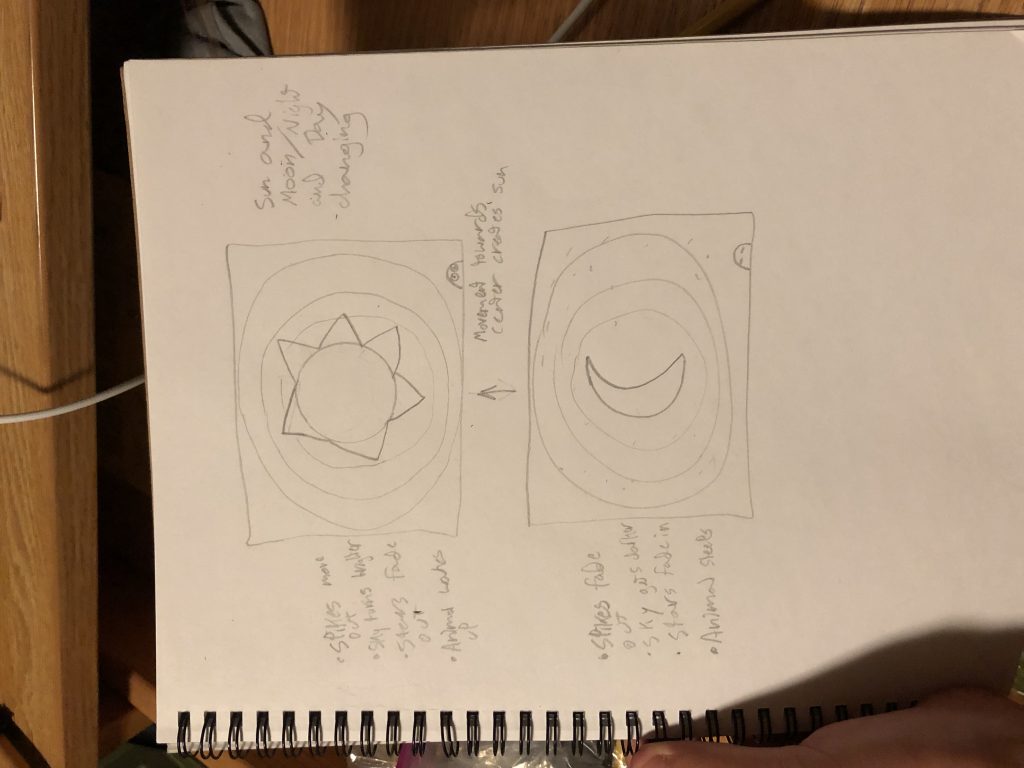
var centX = 320;//center x value
var centY = 240;//center y value
var dTopY = 335;//diamond top
var dBottomY = 145;//diamond bottom
var dRightX = 415;//diamond right
var dLeftX = 225;//diamond left
var x = 13;//slows triangles down through division
function setup() {
createCanvas(640,480);
}
function draw() {
background(6, 2, 240);//blue sky
noStroke();
//sunlight layer variables
var starAngle = mouseX;
var l1 = 190;//layer 1
var l2 = mouseX + 1 * 24;//layer 2
var l3 = mouseX + 1 * 30;//layer 3
var l4 = mouseX + 1 * 18;//layer 4
var l5 = mouseX + 1 * 47;//layer 5
var l6 = mouseX + 1 * 81;//layer 6
var l7 = mouseX + 1 * 106;//layer 7
var l8 = mouseX + 1 * 140;//layer 8
//blue layers behind sunlight layers
fill(25, 22, 240);
ellipse(320,240, 600,480);//outer
fill(38, 35, 235);
ellipse(320,240, 440,410);
fill(45, 71, 237);
ellipse(320,240, 330,320);
fill(67, 99, 240);
ellipse(320,240, 260,260);
fill(77, 137, 240);
ellipse(320,240, 245,245);
fill(103, 164, 245);
ellipse(320,240, 220,220);
//rotating stars
push();
fill(255);
translate(20,20);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(200,360);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(390,90);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(420,300);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(50,80);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(480,220);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(400,400);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(600,470);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(65,300);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(600,60);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
push();
fill(255);
translate(200,30);
rotate(radians(mouseX));
ellipse(0,0, 10,20);
rotate(radians(mouseX + 1));
ellipse(0,0, 20,10);
pop();
//yellow sunlight layers
fill(254, 255, 209);
ellipse(centX,centY, l8,l8);//outer//800
fill(255, 240, 148);
ellipse(centX,centY, l7,l7);//7//600,480
fill(255, 235, 110);
ellipse(centX,centY, l6,l6);//6//440,410
fill(255, 230, 69);
ellipse(centX,centY, l5,l5);//5//330,320
fill(255, 226, 3);
ellipse(centX,centY, l4,l4);//4//260
fill(255, 213, 3);
ellipse(centX,centY, l3,l3);//3//245
fill(255, 205, 3);
ellipse(centX,centY, l2,l2);//210
//triangles expand outward to create Sun rays
fill(252, 132, 33);
triangle(320 + mouseX / x,dTopY, dRightX + mouseX / x,240,
320 + mouseX / x,dBottomY);//triangle right
triangle(320 - mouseX / x,dTopY, dLeftX - mouseX / x,240,
320 - mouseX / x,dBottomY);//triangle left
triangle(dLeftX,240 + mouseX / x, 320,dTopY + mouseX / x,
dRightX,240 + mouseX / x);//triangle top
triangle(dLeftX,240 - mouseX / x, 320,dBottomY - mouseX / x,
dRightX,240 - mouseX / x);//triangle bottom
fill(252, 186, 3);
ellipse(centX,centY, l1,l1);//Sun
//causes gradient shift to Moon
if(mouseX <= 200){
fill(239, 189, 53);
ellipse(centX,centY, l1,l1);//darker Sun
}
if(mouseX <= 160){
fill(226, 197, 103);
ellipse(centX,centY, l1,l1);//darker Sun 2
}
if(mouseX <= 120){
fill(213, 205, 153);
ellipse(centX,centY, l1,l1);//darker Sun 3
}
if(mouseX <= 80){
fill(200, 213, 203);
ellipse(centX,centY, l1,l1);//darker Sun 4
}
if(mouseX <= 40){
fill(187, 221, 253);
ellipse(centX,centY, l1,l1);//darker Sun 5
}
if(mouseX <= 5){
fill(184, 226, 255);
ellipse(centX,centY, l1,l1);//Moon
}
}
A video in case the code doesn’t load