//Jamie Park jiminp@andrew.cmu.edu
//15-104 Section E Project 6
var prevSec;
var millisRolloverTime;
//--------------------------
function setup() {
createCanvas(480, 480);
millisRolloverTime = 0;
}
//--------------------------
function draw() {
background(250); // My favorite pink
noStroke(); //gets rid of stroke of the shapes
fill(255, 223, 212);
for (var x = -10; x < width + 25; x += 50){
for(var y = -10; y < height + 25; y += 50){
ellipse(x, y, 50, 50);
}
}
// Fetch the current time
var H = hour();
var M = minute();
var S = second();
var minRect;
// Reckon the current millisecond,
// particularly if the second has rolled over.
// Note that this is more correct than using millis()%1000;
if (prevSec != S) {
millisRolloverTime = millis();
}
prevSec = S;
var mils = floor(millis() - millisRolloverTime);
var hourColor = map(H, 0, 23, 60, 220);
//changed map from 0 to 120, so the rectangle could increase 2 pixels / min
var minuteIncrease = map(M, 0, 59, 50, 120);
var secondRotation = map(S, 0, 59, 0, width);
//Created two circles that change color depending on the time of the day
fill(61, hourColor, 235); //different shades of blue (dark to light blue)
ellipse(width / 2, height / 2, 325, 325);
fill(235, hourColor, 61); //transition from red to yellow
ellipse(width / 2, height / 2, 300, 300)
//Created a blue rectangle that increases 2 pixels and changes color 2 values every minute
rectMode(CENTER);
fill(51 + minuteIncrease, 111, 184)
rect(width / 2, height / 2, 70 + minuteIncrease, 70 + minuteIncrease, 15, 15);
//Created a hexagon that rotates every second
push();
translate(width / 2, height / 2);
rotate(secondRotation);
fill(116, 232, 228);
polygon(0, 0, 50, 6);
pop();
}
//created function polygon to create a hexagon
function polygon(x, y, radius, nPoints){
var angle = TWO_PI / nPoints
beginShape();
for (var i = 0; i < TWO_PI; i += angle){
var sx = x + cos(i) * radius;
var sy = y + sin(i) * radius;
vertex(sx, sy);
}
endShape(CLOSE);
}
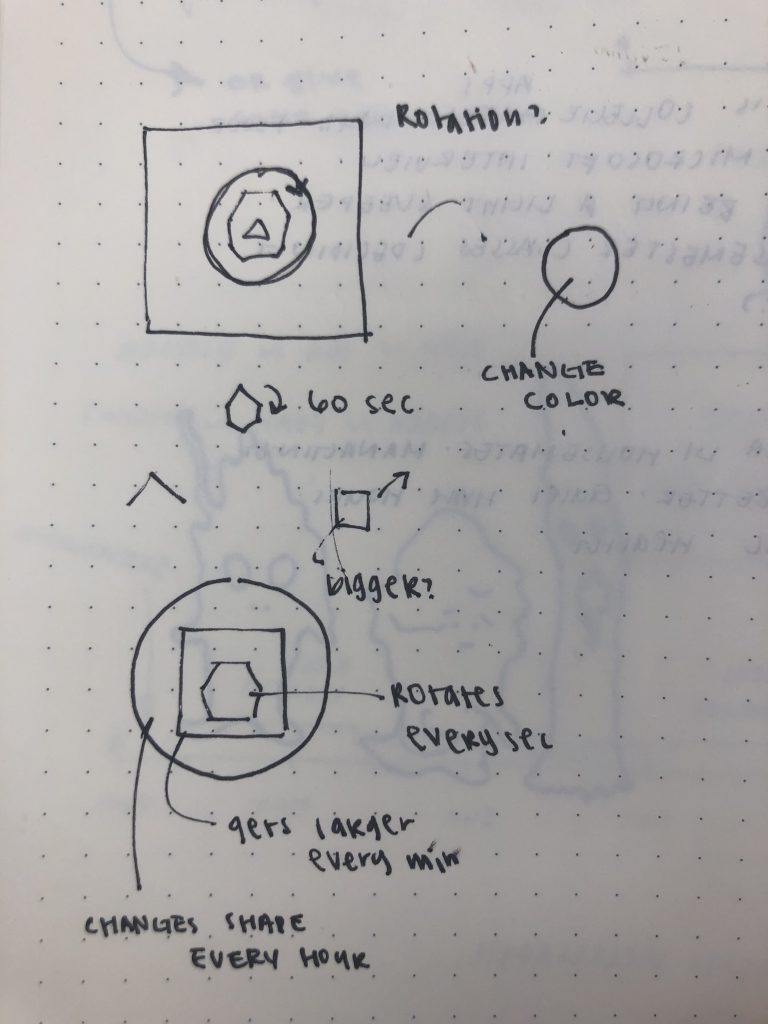
I started the project by writing down the elements I wanted to include in my clock. I then tried to visualize it through sketching. When I had a concrete idea of what i wanted, I coded the information.
And this is my version of an abstract clock that rotates, changes color, and enlarges according to the time of the day. The seconds are marked by rotating hexagon, the minutes are marked by square that increases “r” value of color by 2 and the size of the wall by 2 pixels, and hours by the two circles that change color. I really enjoyed this project as it allowed me to explore the creative side of coding.