Because of the time of year, I decided to make mine about Halloween. I based my design on the Charlie Brown Halloween special “It’s The Great Pumpkin, Charlie Brown”. I looked up a bunch of photos from this special and started taking what I liked from them and mixing them into my sketch. I had my sketch be of the pumpkin patch where the kids would wait for the Great Pumpkin, and would have the sun and moon tell what time of day it was. I also decided to have the sky change color in response to these. I used if statements and the hour() variable in order to accomplish this. I then went about creating the patch, using for loops to create lots of leaves and also the lines dividing a fence. I individually created each pumpkin in the patch, making the main one the kids are behind the largest and bumpiest.
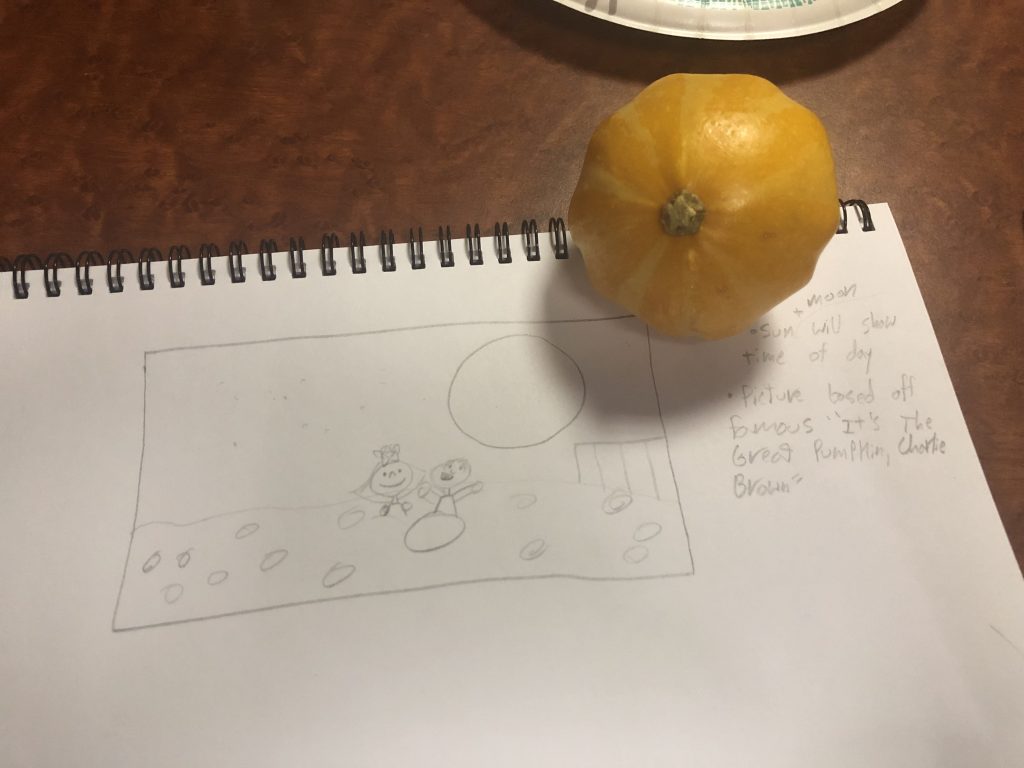
function setup(){
createCanvas(480,480);
}
var backColorR = 168;//red value
var backColorG = 215;//green value
var backColorB = 255;//blue value
var lSW = 10;//leaf width
var lSH = 10;//leaf height
var gSP = 20;//space between columns
var vSP = 20;//space between rows
var gA = 20;//# added to g
var vA = 350;//# added to v
var dotX = 213;//x value of girl's dot
var dotY = 352;//y valye of girl's dot
var lineX = 244;//x value of first stripe point
var lineY = 345;//y value of first stripe point
var bFaceX = 258;//x value of center of boy's head
var bFaceY = 316;//y value of center of boy's head
var gFaceX = 211;//x value of center of girl's head
var gFaceY = 316;//y value of center of girl's head
function draw() {
var H = hour();
//"clock" part
push();
noStroke();
//sky colors
//changes sky color according to real hours
if(H > 20){//black
backColorR = 0;
backColorG = 0;
backColorB = 0;
}
else if(H > 16){//dark blue
backColorR = 1;
backColorG = 25;
backColorB = 145;
}
else if(H > 12){//blue
backColorR = 143;
backColorG = 182;
backColorB = 255;
}
else if(H > 8){//light blue
backColorR = 168;
backColorG = 215;
backColorB = 255;
}
else if(H > 4){//yellow
backColorR = 255;
backColorG = 227;
backColorB = 143;
}
else{//pink
backColorR = 255;
backColorG = 94;
backColorB = 129;
}
pop();
background(backColorR,backColorG,backColorB);//sky
//fence
fill("white");
rect(367,300, 112,70);//fence
for(var i = 0; i < 7; i++){//fence dividers
line((i * 16) + 367,300, (i * 16) + 368, 370);//draws dividers
}
//sun
//changes sun position and color according to real hours
if(H > 12 & H < 19){//13 and above
fill(255, 219, 56);
ellipse(width / 2, (H * 23), (width / 2) - 10,(height / 2) - 10);
}
//moon
else if(H > 18){//19 to 0/24
fill(255, 217, 79);
ellipse(width / 2, height - (H * 15), (width / 2) - 10,
(height / 2) - 10);
}
//sun
else{//0 to 12
fill(255, 219, 56);
ellipse(width / 2, height - (H * 30), (width / 2) - 10,
(height / 2) - 10);
}
pop();
noLoop();
//decorations
push();
strokeWeight(.2);
line(374,308, 374,318);//fence line (FL) 1
line(378,310, 378,330);//FL2
line(390,334, 390,346);//FL3
line(405,305, 405,315);//FL4
line(410,311, 410,330);//FL5
pop();
push();
fill("green");
noStroke();
rect(0,360, 480,120);//grass background
ellipse(width / 2,393, 640,100);//grass hill
pop();
//leaves on the ground
push();
noStroke();
for(var g = 0; g < 20; g++){//increments elements
for(var v = 0; v < 10; v++){//draws ellipses
fill(2, 84, 24);//darkest green
ellipse((g * (gSP + 7)) + (gA - 10),(v * (vSP - 5)) + (vA + 7),
lSW,lSH + 2);
ellipse((g * (gSP + 7)),(v * (vSP - 5)) + (vA + 20), lSW,lSH - 4);
ellipse((g * (gSP + 7)) + gA,(v * (vSP - 5)) + (vA + 15),
lSW,lSH + 1);
ellipse(((g + (gA - 1)) * (gSP - 3)) - (gA * 12.5),(v * (vSP - 5))
+ vA, lSW,lSH + 1);
fill(15, 99, 56);//dark green
ellipse((g * (gSP + 9)) + gA,(v * (vSP - 5)) + (vA + 7),
lSW,lSH + 2);
ellipse((g * (gSP * 2.25)),(v * (vSP - 5)) + (vA + 20), lSW,
lSH + 3);
ellipse((g * (gSP * 1.75)) + gA,(v * (vSP - 5)) + (vA + 15),
lSW,lSH);
fill(158, 83, 2);//dark orange
ellipse((g * (gSP * 2.5)) + (gA + 7),(v * (vSP * 2)) + (vA + 7),
lSW + 3,lSH);
ellipse((g * (gSP * 1.5)) - (gA - 5),(v * (vSP * 2.25)) +
(vA + 25), lSW,lSH - 3);
fill(2, 158, 43);//green
ellipse((g * (gSP * 1.75)) + (gA + 7),(v * vSP) + (vA + 7),
lSW,lSH);
ellipse((g * (gSP * 2)) - (gA / 4),(v * (vSP * 2.5)) + (vA + 20),
lSW,lSH);
fill(158, 18, 2);//red
ellipse((g * (gSP * 3.4)) + (gA - 10),(v * (vSP + 5)) + (vA + 4),
lSW,lSH - 1);
fill(122, 108, 26);//olive green
ellipse((g * (gSP * 2.25)) + (gA + 7),(v * vSP) + (vA + 7),
lSW,lSH);
ellipse((g * (gSP * 1.5)) - (gA / 4),(v * (vSP + 15)) + (vA + 3),
lSW,lSH - 2);
}
}
pop();
//characters
push();
noStroke();
//_girl's hair
fill("yellow");
ellipse(gFaceX,gFaceY - 1, 26,26);//main hair
ellipse(gFaceX + 5,gFaceY, 28,20);////right hair
ellipse(gFaceX - 5,gFaceY + 2, 28,20);//left hair
ellipse(gFaceX - 2,gFaceY - 17, 10,10);//main bun
ellipse(gFaceX - 2,gFaceY - 16, 15,7);//left and right buns
//-skin-
fill(250, 217, 162);//skin color
//boy skin
quad(bFaceX - 5,330,bFaceX - 3.5,328, bFaceX + .5,328,
bFaceX + 1,331);//boy's neck
ellipse(bFaceX,bFaceY, 22,25);//boy's head
ellipse(bFaceX - 3,bFaceY + 2, 24,20);//boy's cheek
quad(268,332,275,331, 276,335,269,338);//boy's right arm
quad(244,330,236,328, 235,332,241,336);//boy's left arm
ellipse(278,332, 7,7);//boy's right hand
ellipse(233,329, 7,7);//boy's left hand
ellipse(bFaceX + 11,bFaceY + 4, 5,5);//right ear
ellipse(bFaceX - 13,bFaceY - 1, 5,5);//left ear
//girl skin
quad(gFaceX + 1.5,332,gFaceX + 2,327, gFaceX + 4,327,
gFaceX + 6.5,331);//girl's neck
ellipse(gFaceX,gFaceY, 24,24);//girl's head
triangle(206,338, 222,370, 226,336);//right and left arm
ellipse(gFaceX + 12,gFaceY - 1, 5,5);//right ear
ellipse(gFaceX - 12,gFaceY + 2, 5,5);//left ear
pop();
//-clothes-
//boy clothes
fill("red");
quad(238,355,252,331, 260,332,266,355);//boy's shirt
quad(260,332,267,332, 268,338,263,342);//right shirt sleeve
quad(252,331,245,330, 242,336,246.5,340);//left shirt sleeve
//girl clothes
fill("lightBlue");
ellipse(222,336, 7,7);//right shirt sleeve
ellipse(210,338, 7,7);//left shirt sleeve
quad(209,360,212,333, 218,332,232,354);//girl's shirt
ellipse(212,302.5, 5,8);//right side of bow
ellipse(206,303, 5,8);//left side of bow
//_boy's hair
line(bFaceX - 3,bFaceY - 13, bFaceX - 6,bFaceY - 10);
line(bFaceX,bFaceY - 13, bFaceX - 3,bFaceY - 10);
line(bFaceX + 3,bFaceY - 12, bFaceX,bFaceY - 9);
line(bFaceX + 6,bFaceY - 10, bFaceX + 3,bFaceY - 7);
line(bFaceX + 9,bFaceY - 7, bFaceX + 6,bFaceY - 5);
line(bFaceX + 10,bFaceY - 4, bFaceX + 7,bFaceY - 2);
line(bFaceX + 10,bFaceY - 1, bFaceX + 8,bFaceY);
push();
//-small details-
strokeWeight(1.5);
//_eyes
//boy
point(bFaceX + 2,bFaceY - 2);//boy left eye
point(bFaceX - 6,bFaceY - 4);//boy right eye
//girl
point(gFaceX - 5,gFaceY - 4);//girl left eye
point(gFaceX + 3,gFaceY - 5);//girl right eye
//_on clothes
//boy's stripes
line(244,345, 264,350);//bottom
line(lineX + 2,lineY - 4, lineX + 19,lineY + 1);//2
line(lineX + 5,lineY - 7, lineX + 18,lineY - 3);//3
line(lineX + 7,lineY - 11, lineX + 17,lineY - 8);//top
line(lineX + 20,lineY - 12, lineX + 21,lineY - 5);//right sleeve
line(lineX + 5,lineY - 14, lineX + .5,lineY - 7);//left sleeve
//girl's dots
point(dotX,dotY);//left
point(dotX + 6, dotY - 6);//middle
point(dotX + 7, dotY - 12);//right
point(dotX + 2, dotY - 17);//right
point(dotX + 10, dotY - 18);//right sleeve
point(dotX - 3, dotY - 14);//left sleeve
point(dotX - 8, dotY - 50);//bow left
point(dotX, dotY - 48);//bow right
point(dotX - 2, dotY - 52);//bow right top
push();
//_smiles
//boy's
fill("red");
arc(255,320, 7, 6, 0, PI, CHORD);
//girl's
noFill()
arc(211,319, 8, 6, 0, PI, OPEN);
pop();
pop();
//-pumpkins-
push();
fill("orange");
//main pumpkin
ellipse(width / 2, (height / 2) + 132, 40,47);//middle bump
ellipse((width / 2) - 11, (height / 2) + 133, 47,46);//left bump
ellipse((width / 2) + 11, (height / 2) + 133, 47,46);//right bump
ellipse(width / 2, (height / 2) + 135, 55,45);//big pumpkin base
ellipse((width / 2) - 10, (height / 2) + 135, 35,45);//left inner gump
ellipse((width / 2) + 10, (height / 2) + 135, 35,45);//right inner gump
ellipse((width / 2), (height / 2) + 137, 20,45);//most inner bump lines
//other pumpkins
fill(224, 132, 4);
ellipse(width - 140,height - 30, 20,18);//1 (medium)
ellipse(width - 140,height - 30, 10,18.5);//1 middle part
fill(227, 127, 27);
ellipse((width / 2) - 40,height - 30, 40,48);//2 (long / big)
ellipse((width / 2) - 40,height - 30, 20,48);//2 middle part
fill(227, 104, 27);
ellipse((width / 2) - 50,height - 100, 9,7);//3 (small)
ellipse((width / 2) - 50,height - 100, 5,7);//3 middle part
fill(255, 81, 0);
ellipse(width - 25,height - 130, 30,35);//4 (upper right)
ellipse(width - 25,height - 130, 20,35);//4 middle part
fill(224, 96, 4);
ellipse(width / 8,height - 60, 40,30);//5 (left)
ellipse(width / 8,height - 60, 20,30);//5 middle part
fill(255, 86, 48);
ellipse(width / 12,height - 110, 20,17);//6 (upper left)
ellipse(width / 12,height - 110, 10,17);//6 middle part
fill(242, 59, 22);
ellipse(width - 180,height - 70, 15,15);//7 (right of main)
ellipse(width - 180,height - 70, 10,15);//7 middle part
fill(227, 98, 7);
ellipse(width - 15,height - 15, 20,20);//8 (left corner)
ellipse(width - 15,height - 15, 12,20.5);//8 middle part
pop();
}