/* Kimberlyn Cho
Section C
ycho2@andrew.cmu.edu
Project-07 */
function setup() {
createCanvas(480, 480);
};
function draw() {
push();
background(0);
strokeWeight(2);
noFill();
translate(width / 2, height / 2);
stroke(188, 19, 254);
drawHypotrochoidCurve();
stroke(250, 237, 39)
drawEpitrochoidCurve();
pop();
};
//Hypotrochoid Curve
function drawHypotrochoidCurve() {
//http://mathworld.wolfram.com/Hypotrochoid.html
//equations
var x;
var y;
//parameters to control curve
var a = map(mouseX, 0, width, 0, 200)
var b = a / 20
//curve radius
var h = map(mouseY, 0, height, 0, 200)
//drawing curve
push();
beginShape();
for (var i = 0; i < 100; i ++) {
var t = map(i, 0, 100, 0, TWO_PI)
x = (a - b) * cos(t) + h * cos(((a - b) / b) * t)
y = (a - b) * sin(t) - h * sin(((a - b) / b) * t)
vertex(x, y)
};
endShape();
pop();
}
//Epitrochoid Curve
function drawEpitrochoidCurve() {
//http://mathworld.wolfram.com/Epitrochoid.html
//equations
var x;
var y;
//parameters to control curve
var a = map(mouseX, 0, width, 0, 250)
var b = a / 20
//curve radius
var h = map(mouseY, 0, height, 0, 250)
//drawing curve
push();
beginShape();
for (var i = 0; i < 100; i ++) {
var t = map(i, 0, 100, 0, TWO_PI)
x = (a + b) * cos(t) - h * cos(((a + b) / b) * t)
y = (a + b) * sin(t) - h * sin(((a + b) / b) * t)
vertex(x, y)
};
endShape();
pop();
}
I found the flexibility of this project to be fun in the multitude of possible outcomes it allowed for even with the same kind of curves. The initial exploration of the various equations and curves was interesting– especially in discovering cool, interesting shapes or curves with just math equations. Although the complex equations were intimidating in my first approach to the project, it was pretty amusing to see how the curves reacted to changes in the different parameters. I chose to layer two different types of curves to create an illusion of depth and overlap. Overall, I enjoyed this project and think it was a great introduction to a mathematical approach to design through p5js.
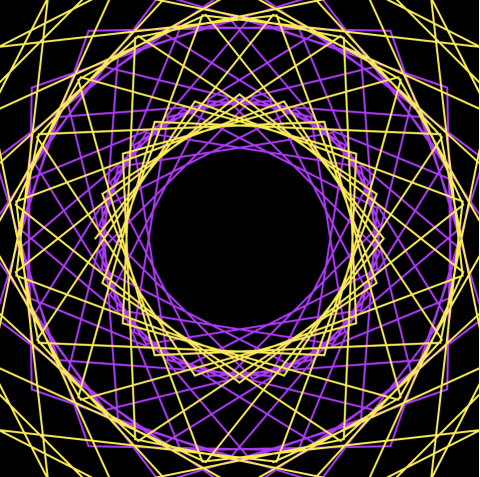
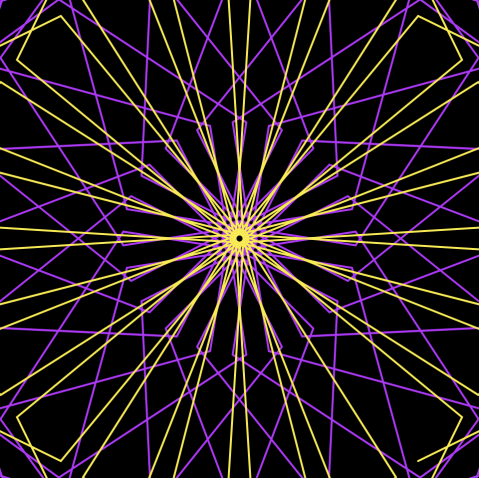