Click on a drum to hear its sound!
// Timothy Liu
// 15-104, Section C
// tcliu@andrew.cmu.edu
// OpenEnded-10
// bass drum variables
var bassDrumW = 200;
var bassDrumX;
var bassDrumY;
var rimWidth = 20;
var bassRadius = bassDrumW / 2;
// high tom tom variables
var tomTomW = 100;
var tomTomH = 40;
var tomTomBaseW = 100;
var tomTomBaseH = 50;
var tomTomX1 = 220;
var tomTomY1 = 200;
var tomTomX2 = 380;
var tomTomY2 = 200;
var baseOffset = tomTomW / 2;
var tomTomRodW = 5;
var tomTomRodH = 250;
var httRodOffset = tomTomRodW / 2;
var hTTDRadius = 50;
// floor tom tom variables
var fTomTomW = 150;
var fTomTomH = 60;
var fTomTomBaseW = 150;
var fTomTomBaseH = 100;
var fTomTomX = 135;
var fTomTomY = 300;
var fBaseOffsetX = fTomTomW / 2;
var fBaseOffsetY = 100;
var fTomTomRodW = 8;
var fTomTomRodH = 100;
var fRodOffset = fTomTomRodW / 2;
var fTTDRadius = 95;
// snare drum
var snareW = 120;
var snareH = 40;
var snareBaseW = 120;
var snareBaseH = 30;
var snareX = 450;
var snareY = 275;
var snareOffsetX = snareW / 2;
var snareOffsetY = snareBaseH;
var snareRodW = 8;
var snareRodH = 200;
var snareRodOffset = snareRodW / 2;
var snareRadius = 50;
// cymbals
var cymbalX1 = [100, 130];
var cymbalY1 = [30, 150];
var cymbalZ1 = [170, 150];
var cymbalX2 = [500, 130];
var cymbalY2 = [570, 150];
var cymbalZ2 = [430, 150];
var cymbalRodH = 350;
var cymbalRodW = 4;
var cymbalOffset = cymbalRodW / 2;
var cymbalH = 20;
// variables for sounds
var hTTDSound;
var FTTDSound;
var bassDSound;
var cymbalSound;
var snareDSound;
// preload all the different drum sounds: snare, bass, high tom tom, floor tom tom, and cymbal
function preload() {
snareDSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/wp-content/uploads/2019/11/snare.wav");
snareDSound.setVolume(0.5);
bassDSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/wp-content/uploads/2019/11/bass.wav");
bassDSound.setVolume(0.9);
hTTDSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/wp-content/uploads/2019/11/HTTD.wav");
hTTDSound.setVolume(0.5);
fTTDSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/wp-content/uploads/2019/11/FTTD.wav");
fTTDSound.setVolume(0.5);
cymbalSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/wp-content/uploads/2019/11/cymbal.wav");
cymbalSound.setVolume(0.5);
}
function setup() {
createCanvas(600, 600);
}
function draw() {
background(200, 200, 255); // blue background
noStroke();
highTomTomDrum(tomTomX1, tomTomY1); // calls the high Tom Tom Drum drawing function and places it at the first x, y
highTomTomDrum(tomTomX2, tomTomY2); // calls the high Tom Tom Drum drawing function again and places it at the other x, y
cymbals(cymbalX1, cymbalY1, cymbalZ1); // calls the cymbal drawing function and places it at the first x, y
cymbals(cymbalX2, cymbalY2, cymbalZ2); // calls the cymbal drawing function again and places it at the other x, y
floorTomTomDrum(fTomTomX, fTomTomY); // calls the floor Tom Tom Drum drawing function and places it at the given x, y
snareDrum(snareX, snareY); // calls the snare drum drawing function and places it at the given x, y
bassDrum(); // calls the bass drum drawing function
}
function mousePressed() {
// play the high tom tom drum sound when the mouse is pressed on the left high tom tom drum
if (insideHTTDrumLeft() === true) {
hTTDSound.play();
}
// play the high tom tom drum sound when the mouse is pressed on the right high tom tom drum
if (insideHTTDrumRight() === true) {
hTTDSound.play();
}
// play the floor tom tom drum sound when the mouse is pressed on the floor tom tom drum
if (insideFTTDrum() === true) {
fTTDSound.play();
}
// play the bass drum sound when the mouse is pressed on the bass drum
if (insideBassDrum() === true) {
bassDSound.play();
}
// play the bass drum sound when the mouse is pressed on the snare drum
if (insideSnare() === true) {
snareDSound.play();
}
// play the bass drum sound when the mouse is pressed on the left cymbal
if (insideCymbalLeft() === true) {
cymbalSound.play();
}
// play the bass drum sound when the mouse is pressed on the right cymbal
if (insideCymbalRight() === true) {
cymbalSound.play();
}
}
function insideHTTDrumLeft() {
var dHTTDrumLeft = dist(mouseX, mouseY, tomTomX1, tomTomY1 + 30); // dist from mouse to center of left HTT Drum
if (dHTTDrumLeft <= hTTDRadius) { // checks if the distance from mouse to the center is less than the radius of the HTT drum (which would mean it's inside the drum)
return true;
}
}
function insideHTTDrumRight() {
var dHTTDrumRight = dist(mouseX, mouseY, tomTomX2, tomTomY2 + 30); // dist from mouse to center of right HTT Drum
if (dHTTDrumRight <= hTTDRadius) { // checks if the distance from mouse to the center is less than the radius of the HTT drum (which would mean it's inside the drum)
return true;
}
}
function insideFTTDrum() {
var dFTTDrum = dist(mouseX, mouseY, fTomTomX, fTomTomY + 75); // dist from mouse to center of FTT Drum
if (dFTTDrum <= fTTDRadius) { // checks if the distance from mouse to the center is less than the radius of the FTT drum (which would mean it's inside the drum)
return true;
}
}
function insideBassDrum() {
var dBass = dist(mouseX, mouseY, bassDrumX, bassDrumY); // dist from mouse to center of bass drum
if (dBass <= bassRadius) { // checks if the distance from mouse to the center is less than the radius of the bass drum (which would mean it's inside the drum)
return true;
}
}
function insideSnare() {
var dSnare = dist(mouseX, mouseY, snareX, snareY + 25); // dist from mouse to center of snare drum
if (dSnare <= snareRadius) { // checks if the distance from mouse to the center is less than the radius of the snare drum (which would mean it's inside the drum)
return true;
}
}
function insideCymbalLeft() { // is the mouse inside the left cymbal?
if (mouseX >= 30 & mouseX <= 170 && mouseY >= 130 && mouseY <= 150) { // checks if the mouse is inside the edges of the cymbal
return true;
}
}
function insideCymbalRight() { // is the mouse inside the right cymbal?
if (mouseX >= 430 & mouseX <= 570 && mouseY >= 130 && mouseY <= 150) { // checks if the mouse is inside the edges of the cymbal
return true;
}
}
function highTomTomDrum(x, y) { // function that draws the high tom tom drums (the 2 small middle ones)
fill(64, 40, 15);
rect(x - httRodOffset, y + baseOffset, tomTomRodW, tomTomRodH);
fill(163, 47, 29);
ellipse(x, y + baseOffset, tomTomW, tomTomH);
rect(x - baseOffset, y, tomTomBaseW, tomTomBaseH);
fill(255, 245, 212);
ellipse(x, y, tomTomW, tomTomH);
}
function floorTomTomDrum(x, y) { // function that draws the floor tom tom drum (the bigger left one)
fill(64, 40, 15);
rect(x - fRodOffset, y + fBaseOffsetY, fTomTomRodW, fTomTomRodH);
fill(163, 47, 29);
ellipse(x, y + fBaseOffsetY, fTomTomW, fTomTomH);
rect(x - fBaseOffsetX, y, fTomTomBaseW, fTomTomBaseH);
fill(255, 245, 212);
ellipse(x, y, fTomTomW, fTomTomH);
}
function bassDrum() { // function that draws the bass drum (the big round middle one)
bassDrumX = width / 2;
bassDrumY = 2 * height / 3;
fill(163, 47, 29);
ellipse(bassDrumX, bassDrumY, bassDrumW + rimWidth, bassDrumW + rimWidth)
fill(255, 245, 212);
ellipse(bassDrumX, bassDrumY, bassDrumW, bassDrumW);
}
function snareDrum(x, y) { // function that draws the snare drum (flatter one on right)
fill(64, 40, 15);
rect(x - snareRodOffset, y + snareOffsetY, snareRodW, snareRodH);
fill(163, 47, 29);
ellipse(x, y + snareOffsetY, snareW, snareH);
rect(x - snareOffsetX, y, snareBaseW, snareBaseH);
fill(255, 245, 212);
ellipse(x, y, snareW, snareH);
}
function cymbals(x, y, z) { // function that draws the cymbals
fill(64, 40, 15);
rect(cymbalX1[0] - cymbalOffset, cymbalX1[1] + cymbalH, cymbalRodW, cymbalRodH);
rect(cymbalX2[0] - cymbalOffset, cymbalX2[1] + cymbalH, cymbalRodW, cymbalRodH);
fill(255, 227, 46);
triangle(x[0], x[1], y[0], y[1], z[0], z[1]);
}
For my sonic sketch, I decided to build an interactive drum set. My drum set is comprised of a bass drum, 2 high tom-tom drums, a snare drum, a floor tom-tom drum, and 2 cymbals (as labeled in the diagram).
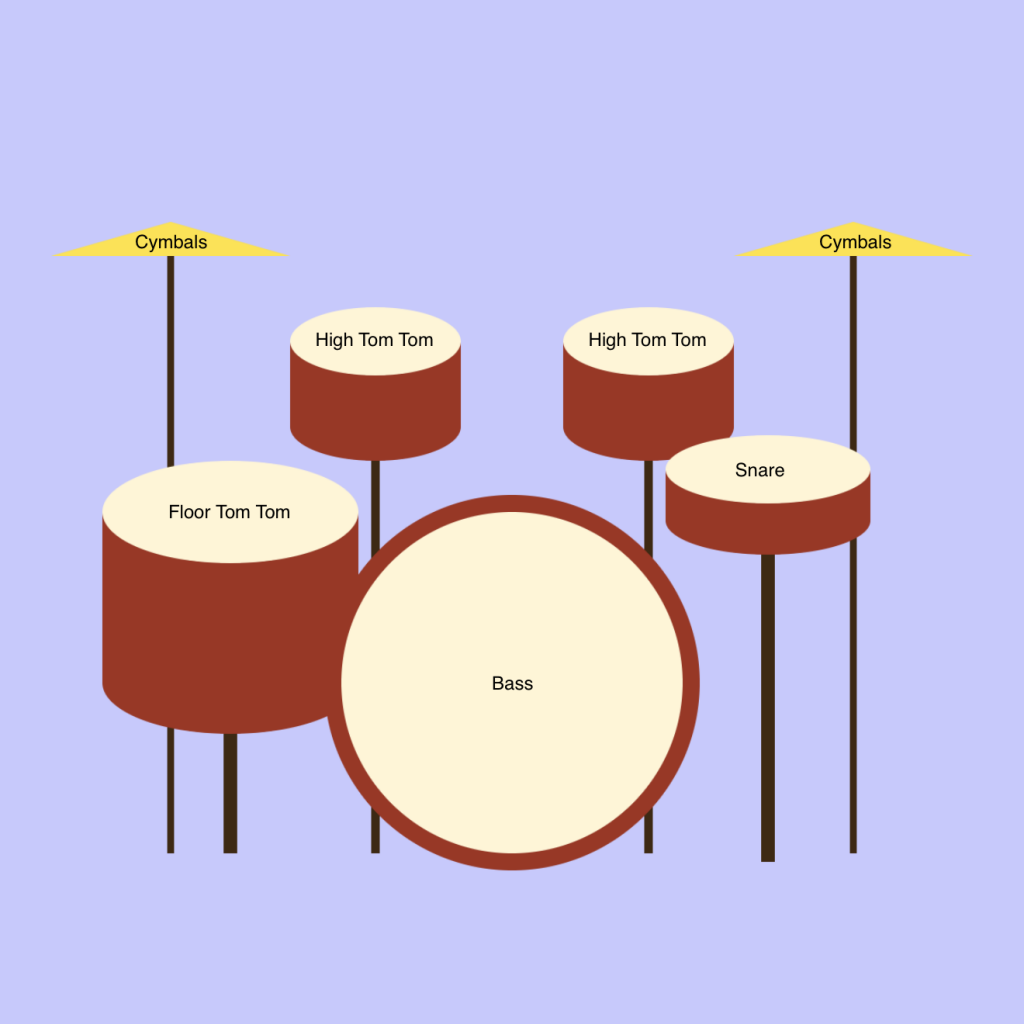
The drum set took a while to create, as I wanted to create separate functions for each drum in order to simplify my draw function. After drawing the drums, I went to freesound.org and found audio files to match each of the drums. I then implemented the logic within mousePressed() to make sure that the sound played when the mouse clicked on the corresponding drum. I really enjoyed this project because it felt very systematic to program and it encouraged me to utilize various functions as well as ample amounts of logic. In addition, getting to learn how to preload sounds was beneficial for my development as a programmer!