/* Chelsea Fan
Section 1B
chelseaf@andrew.cmu.edu
Project-11
*/
//important variables
var fish = [];
var star = [];
function setup() {
createCanvas(480, 480);
frameRate(20);
//initial collection of fish
for (i = 0; i < 30; i++) {
var fishx = random(width);
var fishy = random(300, height);
fish[i] = makeFish(fishx, fishy);
}
//initial collection of stars
for (i = 0; i < 10; i++) {
var starx = random(width);
var stary = random(0, 100);
star[i] = makeStar(starx, stary);
}
}
function draw() {
//black background
background(0);
//draw mountains, water, bed, fish, stars, and moon
drawMountains();
drawWater();
drawBed();
fishies();
stars();
drawMoon();
}
function drawBed() {
stroke(0); //black outline
fill(255, 254, 242); //bed color
rect((width/2)-30, (height/2)-10, 150, 40); //bed shape
fill(255); //pillow color
ellipse(width/2+105, height/2-14, 30, 10); //pillow shape
fill(255, 243, 222); //head color
ellipse(width/2+100, height/2-25, 20, 20); //head shape
noStroke(); //no outline for neck
ellipse(width/2+83, height/2-20, 20, 5); //neck shape
stroke(0); //black outline
fill(203, 202, 204); //blanket color
ellipse(width/2+30, height/2-10, 120, 40); //blanket shape
}
function drawWater() {
fill(66, 112, 128); //blue water color
rect(0, height/2, width, height); //fill bottom half of canvas
}
function drawFish() {
noStroke(); //no outline
fill(255, 160, 122); //orange fish color
push();
translate(this.x1, this.y1); //locate fish at x1, y1
ellipse(10, 10, 10, 5); //fish body
triangle(10, 10, 5, 5, 5, 15); //fish tail
pop();
}
function makeFish(xlocation, ylocation) {
var makeF = {x1: xlocation,
y1: ylocation,
fishx: random(0, width),
fishy: random(300, height),
speed: -3.0,
move: moveFish,
draw: drawFish}
return makeF;
}
function moveFish() {
this.x1 += this.speed; //speed of fish moving
if (this.x1<=-10) { //restart fish at width after it disappears on left
this.x1 += width;
}
}
function fishies() {
for(i=0; i<fish.length; i++) {
fish[i].move();
fish[i].draw();
}
}
function drawStar() {
noStroke();
fill(255, 251, 0); //yellow color
push();
translate(this.x2, this.y2); //draw stars at x2, y2
ellipse(10, 10, 5, 5); //star shape
pop();
}
function makeStar(xlocation, ylocation) {
var makeS = {x2: xlocation,
y2: ylocation,
starx: random(0, width),
stary: random(0, 100),
speed: -3.0,
move: moveStar,
draw: drawStar}
return makeS;
}
function moveStar() {
this.x2 += this.speed; //speed of stars moving
if (this.x2<=-10) { //restart stars on right if it leaves canvas
this.x2 += width;
}
}
function stars() {
for(i=0; i<star.length; i++) {
star[i].move();
star[i].draw();
}
}
function drawMoon(){
fill(255, 253, 184); //yellow moon color
ellipse(400, 50, 50, 50); //moon shape
}
function drawMountains(){
noStroke();
fill(43, 43, 36); //dark gray mountain color
beginShape();
for (i=0; i<width; i++) {
var mountainSpeed = .0007; //speed of mountains moving
var mountainDetail = 0.02; //smoothness of mountains
var t = (i * mountainDetail) + (millis() * mountainSpeed);
//mountain y coord
var y = map(noise(t), 0, 1.8, height/8, height);
//keep drawing mountain
vertex(i, y);
}
//height constriant of mountains
vertex(width, height);
//restart mountains at left side
vertex(0, height);
endShape();
}
This process took me a long time. I spent a while thinking about what I wanted to draw and I decided on a Parent Trap theme with a bed floating in the ocean. The stars, moon, mountains, and fish are meant to resemble an ocean view at night. I had some difficulty making my own object, but once I got the hang of it, it was pretty fun.
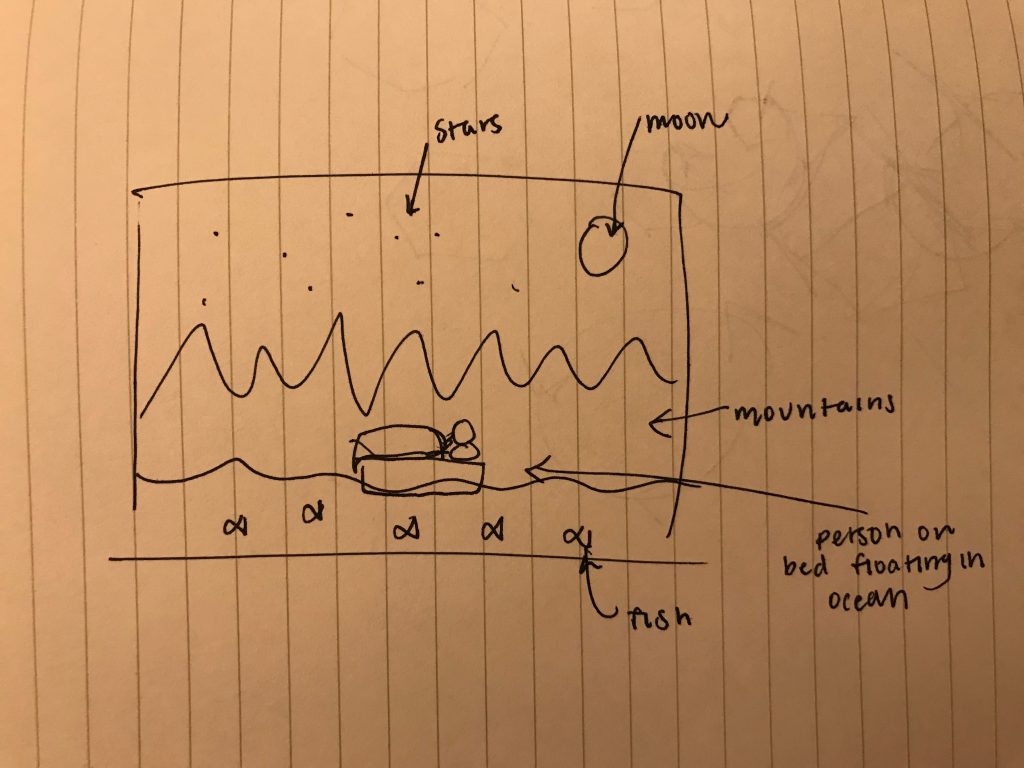