//Jamie Park jiminp@andrew.cmu.edu
//15-104 Section E Project 11
var xCirc = 100;
var yCirc = 120;
var moonX = 270;
var airplaneSound;
var airplanePic;
function preload(){
var airplaneURL = "https://i.imgur.com/4Vw2nUw.png"
airplanePic = loadImage(airplaneURL);
airplaneSound = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/wp-content/uploads/2019/11/325845__daveshu88__airplane-inside-ambient-cessna-414-condenser.mp3");
}
function setup(){
createCanvas(450, 450);
frameRate(9.5);
useSound();
}
function soundSetup(){
airplaneSound.setVolume(0.2);
airplaneSound.play();
airplaneSound.loop();
}
function draw(){
background('#1b2c4d');
stars();
moon();
cloud();
canyons();
canyons2();
canyons3();
image(airplanePic, 0, 0);
}
function canyons(){
var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
noStroke();
fill('#c96e57');
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 0.85, 0, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function canyons2(){
var terrainSpeed = 0.0005;
var terrainDetail = 0.0059;
noStroke();
fill('#913e29');
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 0.65, 0, height - 5);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function canyons3(){
var terrainSpeed = 0.0005;
var terrainDetail = 0.004;
noStroke();
fill('#703223');
beginShape();
for(var x = 0; x < width; x++){
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 0.5, 0, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function cloud(){
noStroke();
fill('#2f446b');
ellipse(xCirc, yCirc + 20, 40, 40);
ellipse(xCirc + 30, yCirc + 10, 60, 60);
ellipse(xCirc + 60, yCirc, 50, 50);
ellipse(xCirc + 70, yCirc + 15, 70, 60);
ellipse(xCirc + 100, yCirc + 10, 60, 60);
ellipse(xCirc + 130, yCirc + 10, 45, 45);
xCirc = xCirc - 5;
if (xCirc === -100){
xCirc = width;
}
}
function stars(){
starX = random(width);
starY = random(height / 2);
for (var i = 0; i < 50; i++){
strokeWeight(2);
stroke("#e8e8cc");
point(starX, starY);
}
}
function moon(){
noStroke();
fill("#f0e3cc");
ellipse(moonX, 180, 100, 100);
fill("#edd4a8");
ellipse(moonX, 180, 70, 70);
moonX = moonX - 0.15;
if (moonX === 0){
moonX = 400;
}
}
I approached this project by brainstorming transportations with windows and ended up landing upon airplanes. The code I have created is a visual of red canyons beyond an airplane window. The stars sparkle at random points of the canvas, and the cloud and the moon shifts left towards the canvas. I had added airplane noise to the file to hint that this is a point of view from an airplane. I really enjoyed the process of visualizing my ideas and I feel like I am becoming more confident in coding.
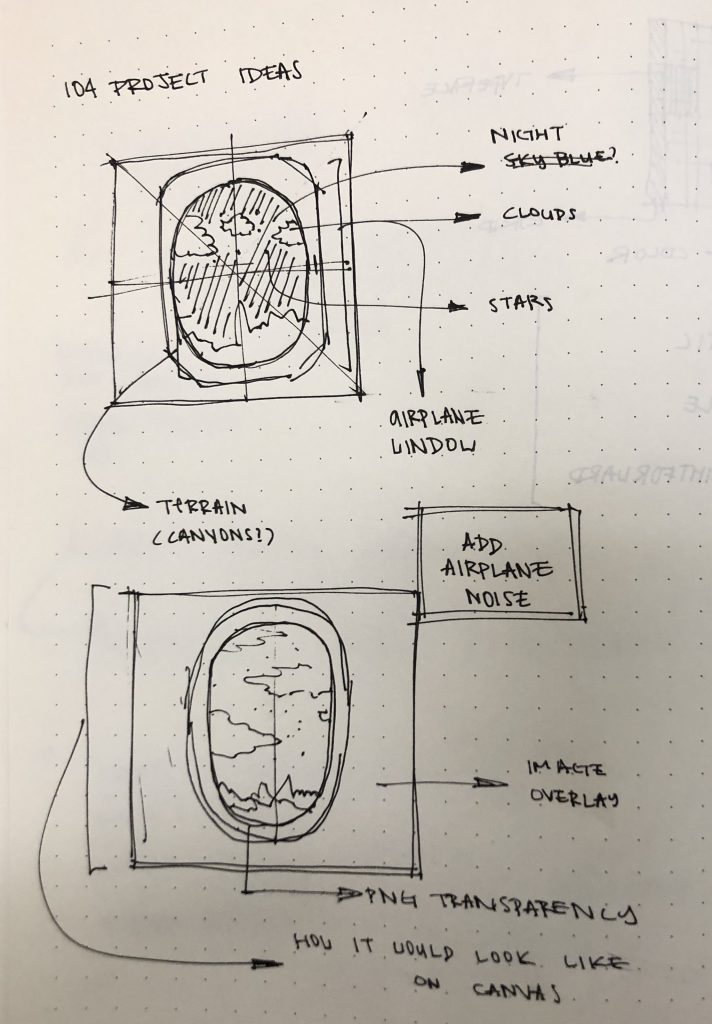