/*
Min Ji Kim Kim
Section A
mkimkim@andrew.cmu.edu
Project-11
*/
var luggage = [];
function setup() {
createCanvas(480,450);
frameRate(50);
// create an initial collection of luggage
for (var i = 0; i < 3; i++) {
var rx = random(width);
luggage[i] = makeLuggage(rx);
}
}
function draw() {
background(225, 225, 218);
baggageClaim(); //background details
updateLuggage(); //update luggage
removeLuggage(); //remove luggage
addLuggage(); //add luggage
}
function updateLuggage() {
// update the luggage's position and display them
for (var i = 0; i < luggage.length; i++) {
luggage[i].move();
luggage[i].display();
}
}
function removeLuggage() {
//remove luggage from array if it's out of sight
var luggageToKeep = [];
for (var i = 0; i < luggage.length; i++) {
if (luggage[i].x + luggage[i].breadth > 0) {
luggageToKeep.push(luggage[i]);
}
}
luggage = luggageToKeep; //remember the surviving luggage
}
function addLuggage() {
//with a very small probability, add a new luggage to the end
var newLuggageProb = 0.008;
if (random(0,1) < newLuggageProb) {
luggage.push(makeLuggage(width));
}
}
// method to update position of luggage every frame
function luggageMove() {
this.x += this.speed;
}
// draw the luggage
function luggageDisplay() {
//bag
noStroke();
fill(this.r, this.g, this.b);
rect(this.x, 360, this.breadth, this.h);
//bag handle
fill("#654321");
var top = 350 + this.h; //top of bag
rect(this.x + 20, top, this.breadth - 45, 5);
rect(this.x + 20, top, 5, 10);
rect(this.x + this.breadth - 25, top, 5, 10);
//bag tag
fill(245);
stroke(this.r, 50, 150);
strokeWeight(3);
rect(this.x + 30, top + 20, 15, 27);
line(this.x + 35, top, this.x + 35, top + 20);
}
// make a luggage bag object
function makeLuggage(beginX) {
var lgg = {x: beginX,
breadth: random(80, 120),
speed: -1.0,
h: -random(50, 100), //luggage height
//set color
r: random(10, 250),
g: random(10, 250),
b: random(10, 250),
move: luggageMove,
display: luggageDisplay}
return lgg;
}
function baggageClaim() { //create background details
noStroke();
//floor
fill(238, 239, 235);
rect(0, 200, width, height);
//windows
fill(236, 236, 230);
rect(0, 50, width, 120);
for(i = 0; i < 6; i++) {
stroke(255);
strokeWeight(4);
strokeCap(SQUARE);
line(i * 85 + 25, 50, i * 85 + 25, 170);
}
line(0, 110, width, 110);
line(0, 140, width, 140);
chair();
//carousel sign
fill(90);
rect(150, 45, 250, 60);
rect(200, 10, 3, 35);
rect(350, 10, 3, 35);
noStroke();
fill(29, 40, 80);
rect(155, 65, 70, 35);
fill(0);
rect(235, 65, 160, 35);
textSize(8);
fill(255);
text("Baggage Claim", 155, 60);
text("Arrival Time", 235, 60);
textSize(15);
text("Delta 2:50", 270, 88);
textSize(35);
text("K", 180, 95);
//conveyor belt
fill(135, 147, 141);
rect(0, 400, width, 20);
fill(67, 67, 67);
rect(0, 420, width, 5);
fill(168, 184, 179);
rect(0, 395, width, 5);
rect(0,305, width, 5);
fill(30);
rect(0, 310, width, 85);
for(j = 0; j < width; j++) { //belt lines
stroke(15);
strokeWeight(3);
line(j * 30, 310, j * 30, 395);
}
}
function chair() { //create a chair
for(x = 0; x < 4; x++) {
noStroke();
fill(196, 197, 190);
rect(80 + x * 22, 155, 20, 12);
rect(80 + x * 22, 168, 20, 2);
stroke(196, 197, 190);
strokeWeight(2);
strokeCap(SQUARE);
line(x * 22 + 90, 167, x * 22 + 90, 172);
line(x * 25 + 85, 172, x * 25 + 85, 180);
strokeWeight(1);
line(84, 172, 161, 172);
}
}
I think this week’s project was the one that I had the most fun making but also the most challenging. Coming up with the idea was easy. I love to travel and I like staring at the baggage claim conveyor belt because if I stare long enough, it makes me feel like I’m moving. I quickly drew a sketch of what I wanted my landscape to look like.
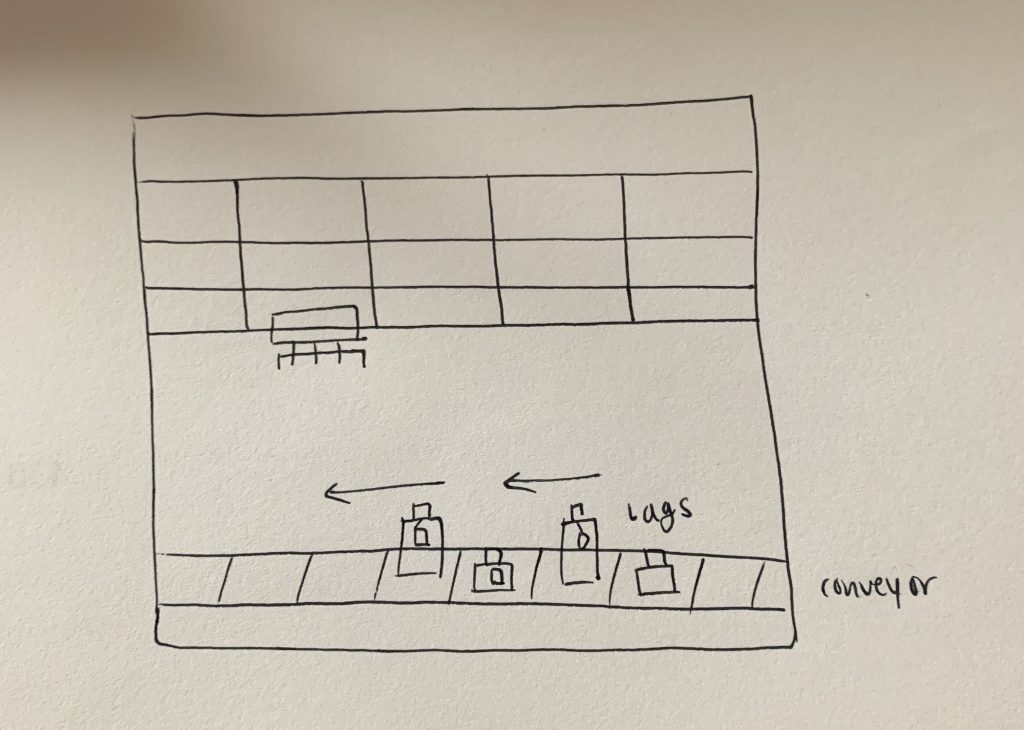
I then moved on to the starter code template and tried to understand what each part of the code was doing and how it was changing the image. This part was a little hard just because there were so many moving parts but I ultimately figured it out and then I tailored it one function at a time to match my sketch and create the final product. The colors and sizes of the bags are generated randomly.