// Nadia Susanto
// nsusanto@andrew.cmu.edu
// Section B
// Project-11-Generative Landscape
var terrainSpeed = 0.0005;
var terrainDetail = 0.005;
var boats = [];
var clouds = [];
function setup() {
createCanvas(480, 480);
//initalizing boats
for (var i = 0; i < 3; i++){
var bx = random(width);
boats[i] = makeBoats(bx);
}
//initalizing clouds
for (var x = 0; x < 5; x++) {
var cx = random(width);
clouds[x] = makeClouds(cx);
}
frameRate(20);
}
function draw() {
//pinkish sky
background(254, 165, 159);
//show mountains and waves
makeMountains();
makeWaves();
//show boats
addNewBoats();
updateAndDisplayBoats();
removeBoatsOutofView();
//show clouds
addNewClouds();
updateAndDisplayClouds();
removeCloudsOutofView();
}
function updateAndDisplayClouds() {
//constantly calling the clouds
for (var x = 0; x < clouds.length; x++){
clouds[x].move();
clouds[x].draw();
}
}
function removeCloudsOutofView() {
var cloudsKeep = [];
for (var x = 0; x < clouds.length; x++) {
if (clouds[x].x > 0) {
cloudsKeep.push(clouds[x]);
}
}
clouds = cloudsKeep; //remember the clouds
}
function addNewClouds() {
var cloudProb = 0.01;
//if random number less than probability then a new cloud is shown
if (random(0, 1) < cloudProb) {
clouds.push(makeClouds(width));
}
}
function cloudsMove() {
//move the clouds from right to left
this.x -= this.speed;
}
function drawClouds() {
//draw the white clouds
fill("white");
ellipse(this.x, this.y, this.width, 10);
}
function makeClouds(cloudX) {
//creating object for cloud
var cloud = {x: cloudX,
y: random(10, 100),
width: random(50, 100),
speed: 0.50,
move: cloudsMove,
draw: drawClouds}
return cloud;
}
function updateAndDisplayBoats() {
//constantly calling the boats
for (var i = 0; i < boats.length; i++){
boats[i].move();
boats[i].draw();
}
}
function removeBoatsOutofView() {
var boatsKeep = [];
for (var i = 0; i < boats.length; i++) {
if (boats[i].x > 0) {
boatsKeep.push(boats[i]);
}
}
boats = boatsKeep; //remember the boats
}
function addNewBoats() {
//if random number less than probability then a new boat is shown
var boatProb = 0.005;
if (random(0, 1) < boatProb) {
boats.push(makeBoats(width));
}
}
function boatsMove() {
//move the boats from right to left
this.x -= this.speed;
}
function drawBoats() {
//random color for boats
fill(this.colorR, this.colorG, this.colorB);
//base of boat
arc(this.x, 350, 75, 50, 0, PI, CHORD);
//pole holding the sail
ellipse(this.x, 330, 10, 80);
//sail of boat
triangle(this.x, 290, this.x, 330, this.x + 15, 310);
}
function makeBoats(boatX) {
//creating object for boat
var boat = {x: boatX,
colorR: random(0, 255),
colorG: random(0, 100),
colorB: random(0, 200),
speed: 1,
move: boatsMove,
draw: drawBoats}
return boat;
}
//adopted the terrain starter code to make mountains in background
function makeMountains() {
noStroke();
fill(35, 144, 79);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail*3) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, height/4, height/2);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
//adopted the terrain starter code to make ocean waves
function makeWaves() {
noStroke();
fill(1, 108, 194);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail/3) + (millis() * terrainSpeed/2);
var y = map(noise(t), 0, 1, height/2, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
For this project I went back to a time when I was in Banff, Canada. I would canoe or ride in a boat on the lake with the mountains behind me. I created moving mountains and moving ocean waves with boats of random colors popping in and out. It was tough at first to figure out how to code the objects, but it was a fun project and I am happy with the final product.
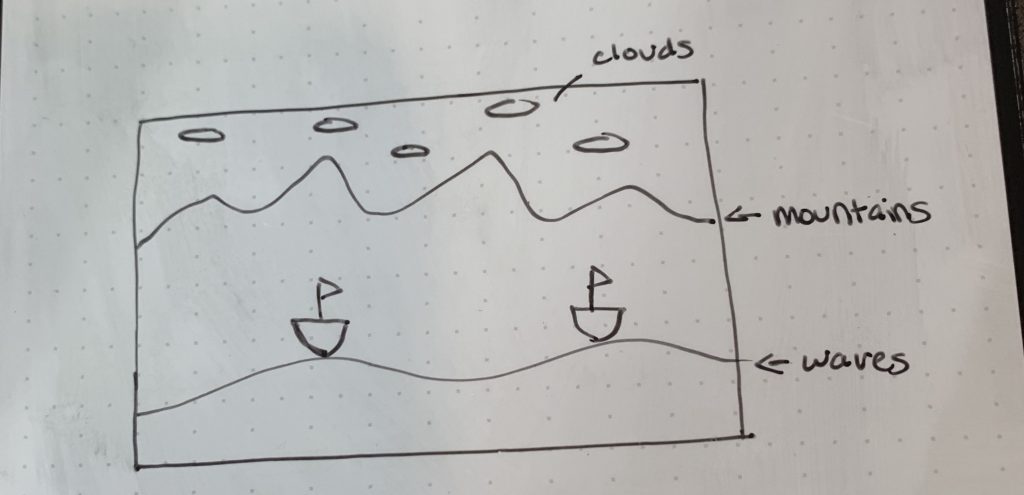