// Joseph Zhang
// Section E
// haozhez@andrew.cmue.edu
// Project-11: Generative Wallpaper
var snowballs = [];
var moons = [];
function setup() {
createCanvas(480,300);
// display initial Snowballs
for( i = 0; i < 3; i++){
var snowBallsInitialX = random(width);
snowballs[i] = drawSnowballs(snowBallsInitialX);
}
var moonInitialX = random(0, width / 3);
moons[0] = drawMoon(moonInitialX);
}
function draw() {
background(28,28,45);
//moon
updateAndDisplayMoon();
addMoon();
//makeTerrain
makeTerrain();
updateAndDisplaySnowballs();
addSnowballs();
}
// all the details of the moon
function addMoon() {
var prob = .07;
if(moons[moons.length-1].xPosMoon < -30){
moons.push(drawMoon(width));
}
}
//render moon on screen
function updateAndDisplayMoon() {
for( i = 0; i < moons.length; i++){
moons[i].displayMoon();
moons[i].moveMoon();
}
}
function drawMoon(xPosM) {
var moon = {
xPosMoon: xPosM,
yPosMoon: random(30, 60),
moonSize: random(30, 50),
moonSpeed: -.2,
//color
r: 255,
g: random(180, 255),
b: random(100, 255),
//moveMoon
moveMoon: function(){
this.xPosMoon += this.moonSpeed;
},
//displayMoon
displayMoon: function() {
noStroke();
fill(this.r, this.g, this.b);
ellipse(this.xPosMoon, this.yPosMoon, this.moonSize, this.moonSize);
fill(28,28,45);
ellipse(this.xPosMoon - 20, this.yPosMoon, this.moonSize, this.moonSize);
},
}
return moon;
}
// draw terrain
var terrainSpeed = 0.00019;
var terrainDetail = 0.008;
function makeTerrain() {
// noFill();
fill(30, 40, 50);
beginShape();
for( x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0 , 1, 0, height);
vertex(x, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
// add snowBalls
function addSnowballs() {
var prob = .07;
if( random(1) < prob) {
snowballs.push(drawSnowballs(width));
}
}
// RENDER onto canvas
function updateAndDisplaySnowballs() {
for ( i = 0; i < snowballs.length; i++) {
snowballs[i].displaySnowBalls(); //displayCircle();
snowballs[i].moveSnowBalls(); //moveCircle();
}
}
// create the actual Snowballs
function drawSnowballs(xPosB) {
var sb = {
xPosSnowBalls: xPosB,
yPosSnowBalls: random(height), // randomize yPossnowBalls of snowBallss
radius: random(5,15),
snowBallsSpeed: random(.5,1),
// move snowBalls
moveSnowBalls: function() {
this.xPosSnowBalls -= this.snowBallsSpeed;
this.yPosSnowBalls += this.snowBallsSpeed * random(.2, .4);
},
// display SnowBalls
displaySnowBalls: function() {
fill('white');
ellipse(this.xPosSnowBalls,this.yPosSnowBalls, this.radius, this.radius);
},
}
return sb;
}
As winter approaches, I wanted to create a landscape that reflected the brisk temperature. In this code, there are two arrays, on that randomly generates snowballs and one that generates a new moon form every time it runs across the screen. Objects were created for both the moon and for the snowballs, where parameters such as color, size, and position were dynamically varied.
Below are sketches of my idea, I wanted to create a sense of time of day, sense of direction, and sense of environment. Much of this was done through the use of color which is made of entirely of cool tones and white.
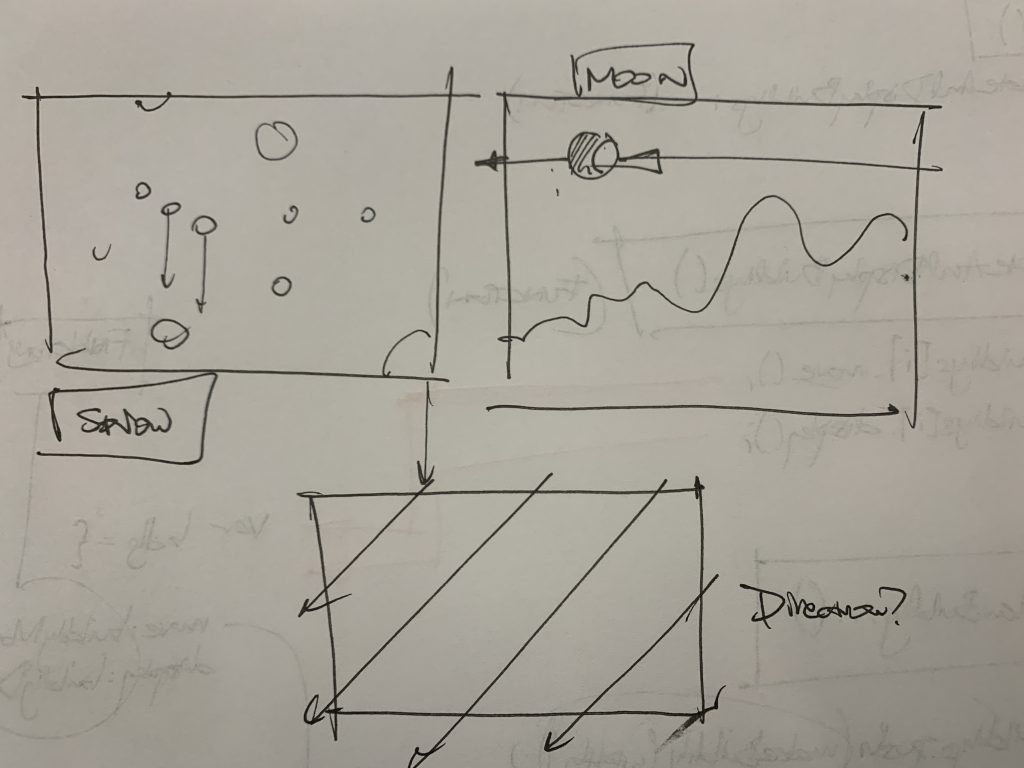