/*
Hyejo Seo
Section A
hyejos@andrew.cmu.edu
Project-11-landscape
*/
var c1, c2; // for gradient
var smile = [];
function setup() {
createCanvas(480, 480);
frameRate(50);
//setting up background gradient
c1 = color(15, 113, 115);
c2 = color(240, 93, 94);
}
function draw() {
gradient(c1, c2); // background color
drawMountains();
desert();
drawSun();
updateSmile();
deleteSmile();
addSmile();
}
// setting up gradient for the sky
function gradient(c1, c2) {
noFill();
noStroke();
for (var y = 0; y < height; y++) {
var inter = map(y, 0, height, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(0, y, width, y);
}
}
function drawMountains () { // drawing mountains by using terrain
var terrainSpeed = 0.0005;
var terrainDetail = 0.006;
stroke(47, 57, 94);
noFill();
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, height / 2, height);
line(x, y, x, height); //draws lines from the points of terrain to the bottom of the canvas
}
endShape();
}
function desert () { //drawing the dessert land
fill(216, 164, 127);
noStroke();
beginShape();
vertex(0, height - 60);
vertex(width, height - 60);
vertex(width, height);
vertex(0, height);
endShape(CLOSE);
}
function drawSun() { // drawing sun at a suspended location
fill(216, 30, 91);
circle(400, 200, 50);
}
function updateSmile() {
for(var i = 0; i < smile.length; i++) {
smile[i].move();
smile[i].draw();
}
}
function deleteSmile() {
var smileToKeep = [];
for(var i = 0; i < smile.length; i++) {
if(smile[i].xx + smile[i].w > 0) {
smileToKeep.push(smile[i]);
}
}
smile = smileToKeep;
}
function addSmile() {
var newSmile = 0.008;
if (random(1) < newSmile) {
smile.push(makeSmile(width, random(450, 480)));
}
}
function moveSmile() {
this.xx += this.speed; // moving the smiles to the left
}
function drawSmile() {
// drawing the face
stroke(56, 63, 81);
strokeWeight(2);
fill(255, 120, 79);
push();
translate(this.xx, this.yy);
circle(0, -this.hh, this.w);
pop();
//drawing the eyes
fill(56, 63, 81);
noStroke();
push();
translate(this.xx, this.yy);
ellipse(-5, -this.hh - 5, 6, 13);
ellipse(5, -this.hh - 5, 6, 13);
pop();
//drawing mouth
stroke(56, 63, 81);
strokeWeight(2);
noFill();
push();
translate(this.xx, this.yy);
arc(0, -this.hh + 5, 20, 15, TWO_PI, PI);
pop();
}
function makeSmile(birthLocationX, birthLocationY) {
var sm = {xx: birthLocationX, yy: birthLocationY,
w: random(30, 50), hh: random(10, 30), speed: -1,
move: moveSmile, draw: drawSmile}
return sm;
}
For this project, I wanted to add an element of surprise to my landscape: smiley faces. I roughly sketched out my plan (as seen below) after being inspired by pictures of the desert in Arizona. This is why I chose the light brown for the land – sandy and dry – and darker and colder blue for the mountains. to convey that they are really far away. Overall, this project helped me feel more comfortable with drawing objects.
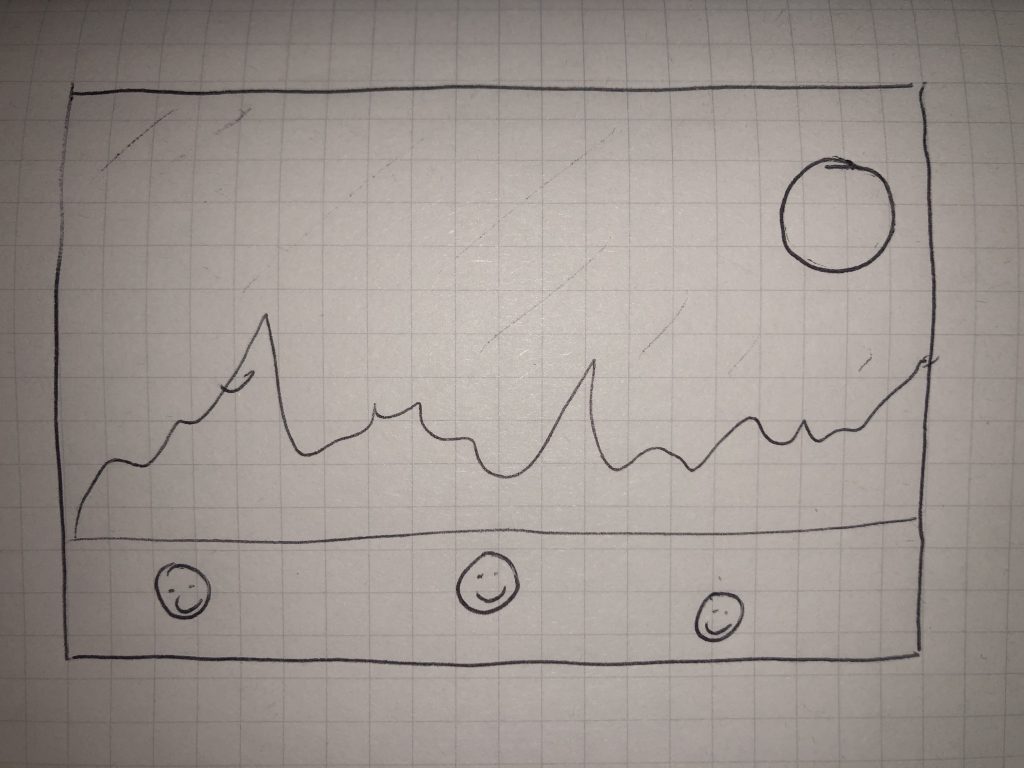
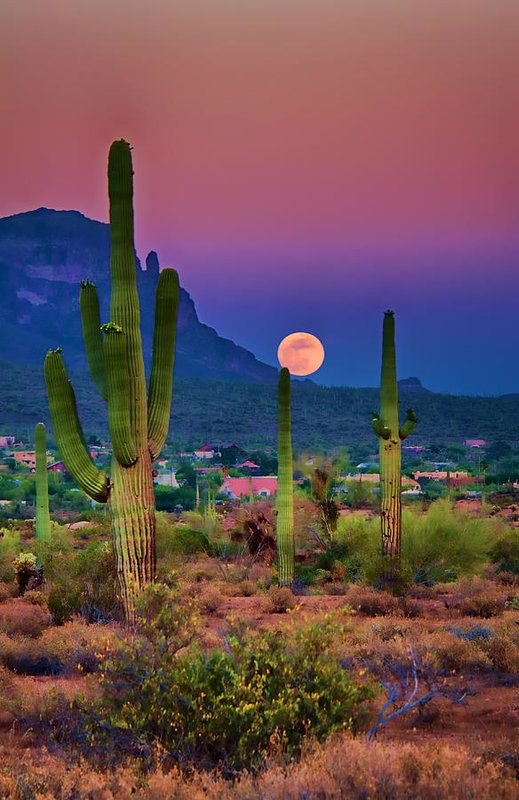