/* Kimberlyn Cho
Section C
ycho2@andrew.cmu.edu
Project 11 */
var gifts = [];
function setup() {
createCanvas(480, 300);
//gifts already on conveyor at start
for (var i = 0; i < 10; i++) {
var rx = random(width);
gifts[i] = makeGifts(rx);
}
frameRate(15);
}
function draw() {
background(240);
//draw gifts
updateGifts();
addGifts();
removeGifts();
//draw conveyor
drawConveyor();
}
//update gift location
function updateGifts() {
for (var i = 0; i < gifts.length; i++) {
gifts[i].move();
gifts[i].display();
}
}
//remove gifts after conveyor from array
function removeGifts() {
var giftsToKeep = [];
for (var i = 0; i < gifts.length; i++) {
if (gifts[i].x + gifts[i].breadth > 0) {
giftsToKeep.push(gifts[i]);
}
}
gifts = giftsToKeep;
}
//add new gifts to array
function addGifts() {
var newGiftLikelihood = 0.04;
if (random(0,1) < newGiftLikelihood) {
gifts.push(makeGifts(width));
}
}
//move gifts per frame
function giftMove() {
this.x += this.speed;
}
//draw each gift
function giftDisplay() {
//giftbox
var py = 150;
noStroke();
fill(this.r, this.g, this.b);
rect(this.x, py, this.breadth, this.h);
//ribbons
fill(255);
rect(this.x + this.breadth / 4, py, this.ribbon, this.h);
rect(this.x, py + this.h / 2, this.breadth, this.ribbon);
}
//gift Object
function makeGifts(birthLocationX) {
var gift = {x: birthLocationX,
h: -random(10, 40),
ribbon: random(1, 5),
r: random(100, 255),
g: random(100, 255),
b: random(100, 255),
breadth: random(20, 80),
speed: -1.0,
move: giftMove,
display: giftDisplay}
return gift;
}
function drawConveyor() {
//conveyor
strokeWeight(0);
fill(220);
rect(0, height / 2, width, 30, 25);
strokeWeight(2);
stroke(255);
rect(4, (height / 2) + 4, width - 8, 30 - 8, 25);
//conveyor gears
for (var y = 0; y < 18; y++) {
var ny = 15 + y * 20;
strokeWeight(2);
stroke(200);
noFill();
ellipse(ny, height / 2 + 15, 18);
strokeWeight(0);
fill(255);
ellipse(ny, height / 2 + 15, 8);
}
//ground
fill(150);
strokeWeight(0);
rect(0, height * 4 / 5, width, height / 5);
//legs
strokeWeight(0);
fill(190);
rect(100, height / 2 + 30, 12, 80);
rect(88, height / 2 + 110, 35, 15, 2);
//scanning machine
rect(350, 145, 200, 130, 8);
rect(350, 50, 10, 110, 8);
rect(350, 50, 135, 15, 8);
fill(255, 0, 0, 50);
rect(360, 65, 130, 80);
}
For this project, I was interested in the purpose of a conveyor belt and how it is able to move a group of objects at a set rate. I used objects to randomize the size and color of gifts that would pass through the conveyor belt. I kept the background graphics to a grayscale to emphasize the gifts that pass through the screen. I found this assignment to be very helpful in understanding how objects work since we had to create our own objects as opposed to being given an object and just using it in the draw function.
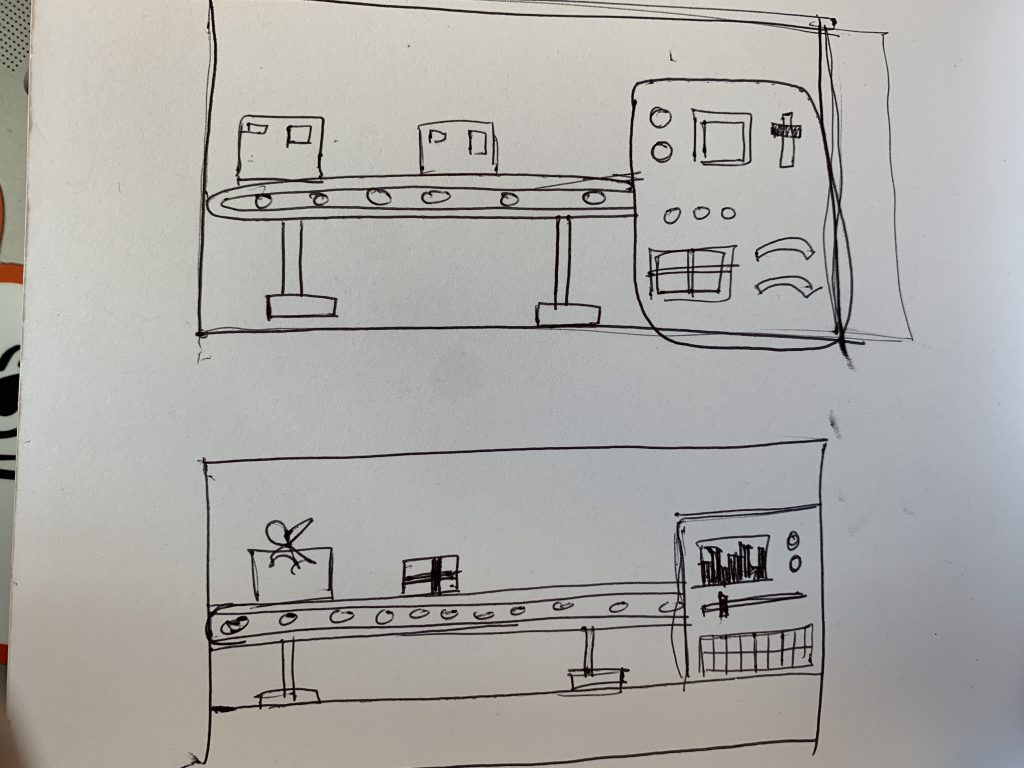