// Nawon Choi
// Section C
// nawonc@andrew.cmu.edu
// Project-11 Landscape
// var buildings = [];
var trainCars = [];
var shapes = [];
// canvas height - 50
var yHorizon = 200 - 50;
function setup() {
createCanvas(480, 200);
// create an initial train car
var rw = random(100, 150);
trainCars.push(makeTrainCar(-50, rw));
frameRate(10);
}
function draw() {
background("#A1C6EA");
displayHorizon();
updateAndDisplayTrainCars();
removeTrainCarsThatHaveSlippedOutOfView();
addNewCars();
// railroad sign
stroke(0);
strokeWeight(2);
line(width - 50, height / 3, width - 50, yHorizon);
fill("yellow");
ellipse(width - 50, height / 3, 40, 40);
fill(0);
textSize(40);
text("X", width - 62, height / 2.5);
}
function updateAndDisplayTrainCars(){
// Update the building's positions, and display them.
for (var i = 0; i < trainCars.length; i++){
trainCars[i].move();
trainCars[i].display();
}
}
function removeTrainCarsThatHaveSlippedOutOfView(){
// If a building has dropped off the left edge,
// remove it from the array. This is quite tricky, but
// we've seen something like this before with particles.
// The easy part is scanning the array to find buildings
// to remove. The tricky part is if we remove them
// immediately, we'll alter the array, and our plan to
// step through each item in the array might not work.
// Our solution is to just copy all the buildings
// we want to keep into a new array.
var carsToKeep = [];
for (var i = 0; i < trainCars.length; i++){
if (trainCars[i].x + trainCars[i].w > 0) {
carsToKeep.push(trainCars[i]);
}
}
trainCars = carsToKeep; // remember the surviving buildings
}
function addNewCars() {
var l = trainCars.length - 1;
var newW = floor(random(100, 150));
var newX = 0 - newW;
// only add a new car after the last one is completely revealed
if (trainCars[l].x >= 0) {
trainCars.push(makeTrainCar(newX, newW));
}
}
// method to update position of the car every frame
function carMove() {
this.x += 3;
}
function carDisplay() {
// draw car body
fill(this.cr, this.cg, this.cb);
rect(this.x, yHorizon - (this.h + 3), this.w, this.h);
// draw wheels
fill("#6b6e6a");
if (this.nWheels >= 2) {
ellipse(this.x + 5, yHorizon, 10, 10);
ellipse(this.x + this.w - 5, yHorizon, 10, 10);
if (this.nWheels == 4) {
ellipse(this.x + 15, yHorizon, 10, 10);
ellipse(this.x + this.w - 15, yHorizon, 10, 10);
} else if (this.nWheels == 3) {
ellipse(this.x + (this.w / 2), yHorizon, 10, 10);
}
}
}
function makeTrainCar(birthLocationX, carWidth) {
var car = {x: birthLocationX,
w: carWidth,
h: floor(random(50, 70)),
nWindows: floor(random(0, 3)),
nWheels: floor(random(2, 5)),
cr: floor(random(0, 255)),
cg: floor(random(0, 255)),
cb: floor(random(0, 255)),
move: carMove,
display: carDisplay}
return car;
}
function displayHorizon(){
noStroke();
// grass
fill("#3a6933");
rect(0, yHorizon, width, 50);
// train track
fill("#35524A");
rect (0, yHorizon, width, 5);
}
For this project, I tried to create a moving landscape in which the subject was moving, but the viewer was still. I depicted a railroad crossing of train cars with varying widths, heights, colors, and wheel numbers. It was interesting to play with random vs fixed variables. For instance, the train cars had to generate right after the last car, instead of at a random frequency. I also tried to create more visual interest by adding a railroad crossing sign in the foreground. I think if I had more time, I would have added interesting patterns to each car, such as windows or texture to the cars.
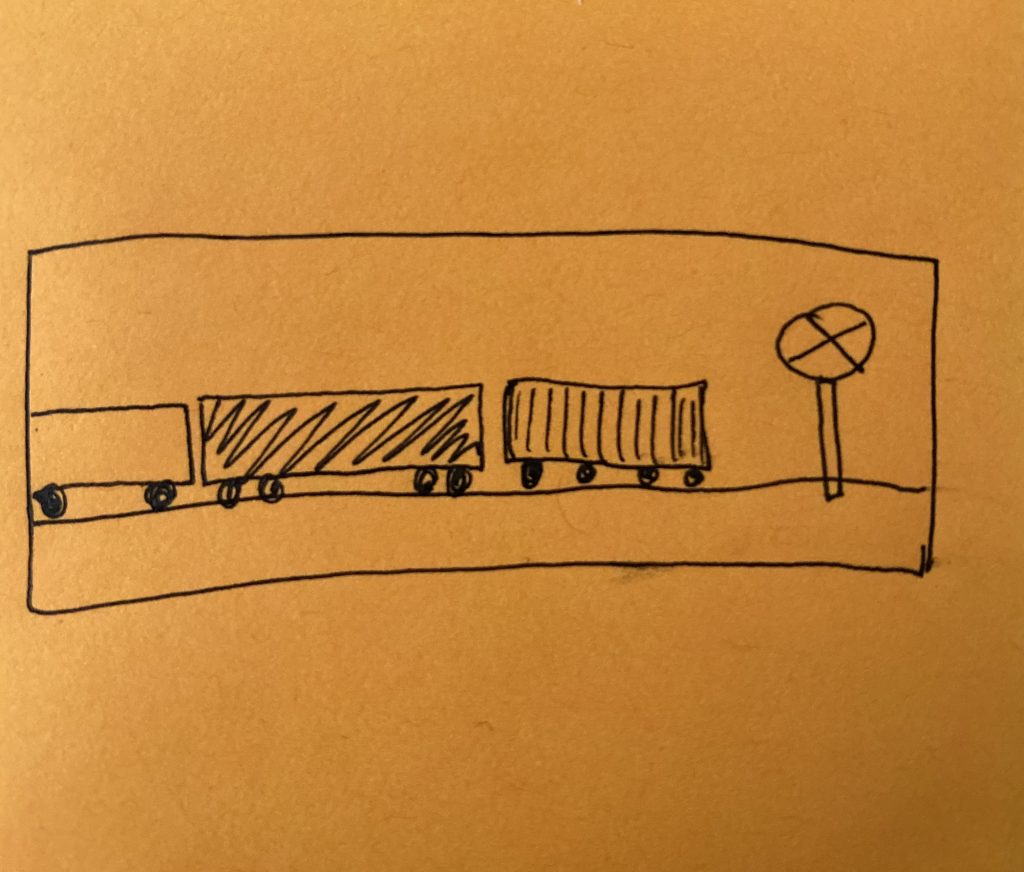