I wanted to make a landscape that gave the illusion of depth, so objects would be moving past at different speeds depending on ‘how far back’ they are. I started by creating the mountains using the noise function. They were initially straight lines (x values i
and i
), but I used i*0.8
and i
instead because the offset created an interesting effect. Then I made a balloon that randomly picked an image from an array. This balloon also randomly generated things like speed and height.
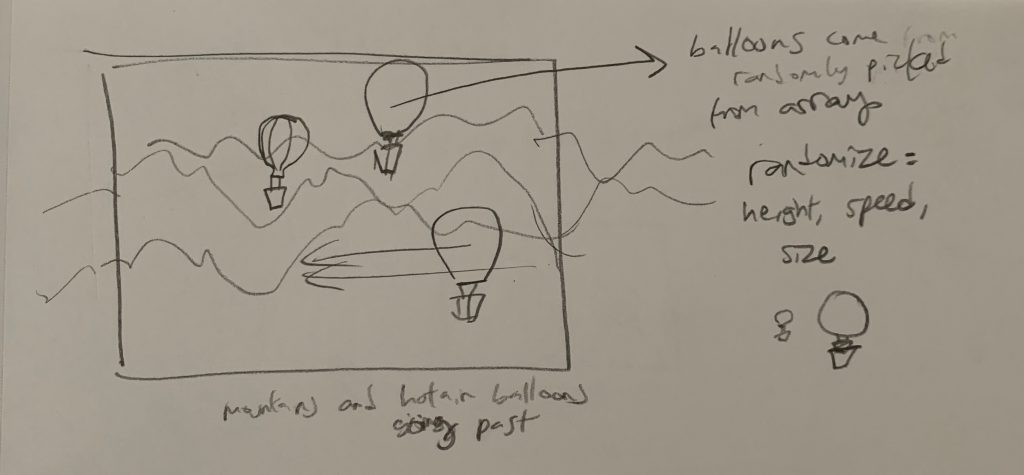
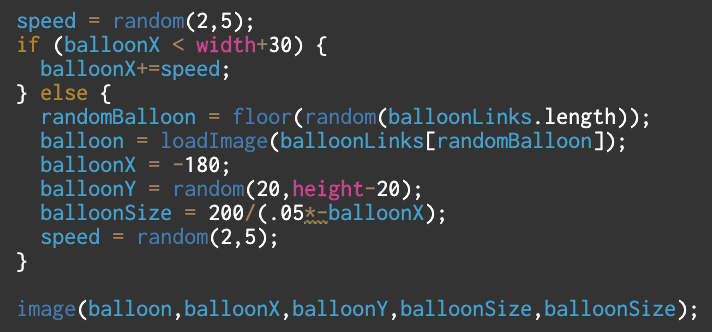
After ironing things out a bit, I used the sample code as a framework for making several balloons in an array. I also made the speed/size more deliberate. Now, the closer the balloon is to the top (the smaller the y-value), the slower it moves, giving the illusion that it is farther away.
/*
Ellan Suder
15104 1 D
esuder@andrew.cmu.edu
Project-11
*/
var balloons = [];
var balloonLinks = [];
var t1 = 0;
var t2= 0;
var t3 = 0;
function preload() {
balloonLinks = [
"https://i.imgur.com/rmLvmIq.png",
"https://i.imgur.com/03Cr2Sx.png",
"https://i.imgur.com/peP166r.png"];
}
function setup() {
createCanvas(480, 480);
frameRate(30);
// create an initial collection of balloons
for (var i = 0; i < 10; i++){
var rx = random(-180, width);
var ry = random(40,height-200);
var rs = 70*(0.005*ry); //make balloons higher up smaller
var spd = ry/70; //make balloons higher up move slower
randomBalloon = floor(random(balloonLinks.length));
balloon = loadImage(balloonLinks[randomBalloon]);
balloons[i] = makeBalloon(balloon, rx, ry, rs, spd);
}
frameRate(10);
}
function draw() {
background(200);
updateAndDisplayBalloons();
removeBalloonsThatHaveSlippedOutOfView();
addNewBalloonsWithSomeRandomProbability();
//the larger t is
//the faster it moves
t1 += 0.01;
t2 += 0.04;
t3 += 0.1;
for(var i=0; i < width*1.3; i++)
{
//the larger the number you divide i by
//the 'faster' the terrain will move
n1 = noise(i/100-t1);
n2 = noise(i/100-t2);
n3 = noise(i/100-t3);
stroke(0,20);
line(i*0.8, height*n1, //top of the line (bumpy randomized part)
i, height); //bottom of line is bottom of canvas
stroke(0,70);
line(i*0.8, height*n2,
i, height);
stroke(0,200);
line(i*0.8, height*n3,
i, height);
}
}
function updateAndDisplayBalloons(){
// Update the balloon's positions, and display them.
for (var i = 0; i < balloons.length; i++){
balloons[i].move();
balloons[i].display();
}
}
function removeBalloonsThatHaveSlippedOutOfView(){
// If a balloon has dropped off the left edge,
// remove it from the array.
var balloonsToKeep = [];
for (var i = 0; i < balloons.length; i++){
if (balloons[i].x + balloons[i].size > 0) {
balloonsToKeep.push(balloons[i]);
}
}
balloons = balloonsToKeep; // remember the surviving balloons
}
function addNewBalloonsWithSomeRandomProbability() {
var newBalloonLikelihood = 0.03;
if (random(0,1) < newBalloonLikelihood) {
rx = random(-60, -50);
ry = random(40,height-200);
rs = 70*(0.005*ry);
spd = ry/70; //make balloons higher up move slower
randomBalloon = floor(random(balloonLinks.length));
balloon = loadImage(balloonLinks[randomBalloon]);
balloons.push(makeBalloon(balloon, rx, ry, rs, spd));
}
}
// method to update position of balloon every frame
function balloonMove() {
this.x += this.speed;
}
// draw the balloon
function balloonDisplay() {
image(this.img,this.x,this.y,this.size,this.size);
}
function makeBalloon(balloon, balloonX, balloonY, balloonSize, balloonSpeed) {
var blln = {img: balloon,
x: balloonX,
y: balloonY,
size: balloonSize,
speed: balloonSpeed,
move: balloonMove,
display: balloonDisplay}
return blln;
}