/*Katrina Hu
15104-C
kfh@andrew.cmu.edu
Project-11*/
var x1 = 100;
var x2 = 400;
var x3 = 30;
var luggage = [];
function setup() {
createCanvas(480, 480);
frameRate(60);
background(191, 233, 255);
//set an initial group of luggage
for (var i = 0; i < 5; i++) {
var rx = random(width);
luggage[i] = makeLuggage(rx)
}
}
function draw() {
//drawing sun
noStroke();
fill(255, 252, 227);
ellipse(80, 40, 80, 80);
fill(255, 246, 179);
ellipse(80, 40, 70, 70);
fill(255, 238, 112);
ellipse(80, 40, 50, 50);
//drawing clouds
drawCloud(x1);
drawCloud(x2);
drawCloud(x3);
//drawing floor & window
fill(100);
rect(97, 0, 10, height);
rect(377, 0, 10, height);
rect(0, 67, width, 10);
rect(0, 294, width, 180);
fill(184);
rect(0, 300, width, 180);
rect(100, 0, 10, height);
rect(380, 0, 10, height);
rect(0, 70, width, 10);
//drawing conveyor belt
fill(50);
rect(0, 372, width, 10);
fill(110);
rect(0, 380, width, 50)
fill(200);
rect(0, 390, width, 30)
for(var dots = 0; dots < 9; dots ++) {
fill(110);
ellipse(dots * 70 + 20, 408, 10, 10);
fill(250);
ellipse(dots * 70 + 20, 403, 10, 10);
}
//drawing luggage
updateLuggage();
removeLuggageFromView();
addLuggage();
}
function drawCloud(x) {
push();
noStroke();
fill(240);
ellipse(x, height / 5, 100, 100);
ellipse(x + 10, height / 5 + 5, 100, 100);
ellipse(x + 80, height / 5, 80, 70);
ellipse(x + 10, height / 5 - 10, 80, 80);
ellipse(x + 70, height / 4 - 55, 60, 50);
pop();
}
function updateLuggage() {
for (var i = 0; i < luggage.length; i++) {
luggage[i].move();
luggage[i].display();
}
}
function removeLuggageFromView() {
//remove luggage from array if it's out of sight
var luggageToKeep = [];
for (var i = 0; i < luggage.length; i++) {
if (luggage[i].x + luggage[i].breadth > 0) {
luggageToKeep.push(luggage[i]);
}
}
luggage = luggageToKeep; //remember the surviving luggage
}
function addLuggage() {
//with a very small probability, add a new luggage to the end
var newLuggageProb = 0.005;
if (random(0,1) < newLuggageProb) {
luggage.push(makeLuggage(width));
}
}
function luggageDisplay() {
var top = 375 + this.height;
//draw luggage
stroke(0);
fill(this.r, this.g, this.b);
rect(this.x, 380, this.breadth, this.height);
//draw handle
fill(50);
rect(this.x + 20, top, this.breadth - 45, 4);
rect(this.x + 20, top, 4, 10);
rect(this.x + this.breadth - 25, top, 4, 10);
}
function luggageMove(){
this.x += this.speed;
}
function makeLuggage(startX){
var myLuggage = {x: startX,
speed: -1.0,
breadth: random(80, 120),
height: -random(50, 80),
r: random(100, 255),
g: random(100, 255),
b: random(100, 255),
move: luggageMove,
display: luggageDisplay}
return myLuggage;
}
My project is of a luggage conveyor belt in an airport. I decided to choose this landscape because of the picture that was posted within the instructions, which showed luggage moving around the belt. Luggage size and color is randomly picked and drawn onto the conveyor belt. This was a very fun project to do, and it was a great opportunity for me to practice using objects. The part I struggled with the most was figuring out how to draw the luggage handles on top of the suitcases. But overall, I learned a lot and am very happy with how the project turned out.
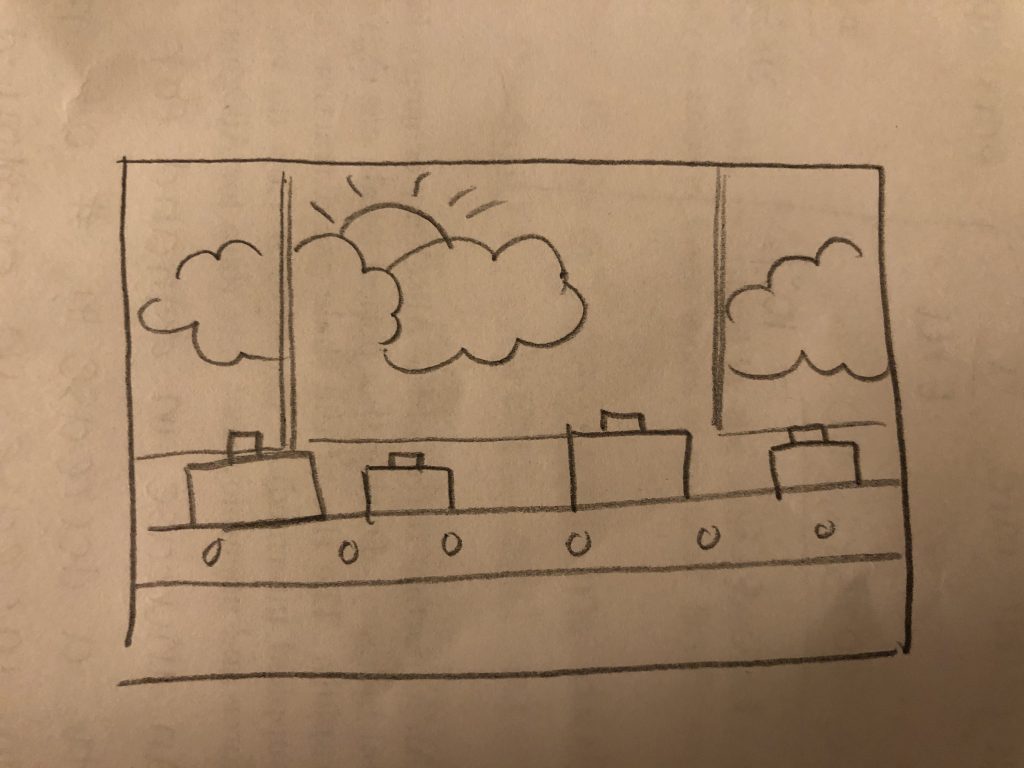