//alcai@andrew.cmu.edu
//alice cai
//section E
//week 11 assignment A
//terrain variables
var terrainSpeed = -0.0003;
var terrainDetail = 0.005;
var terrainSpeed2 = -0.0004;
var terrainDetail2 = 0.007;
//lantern variables
var List = [];
var moving = -5;
var end = -20;
function setup(){
createCanvas (480,480);
frameRate(10);
//call # of lanterns
for (var j = 0; j < 20; j++) {
var px = random(width);
var py = random(height / 3);
List[j] = makeLant(px, py);
}
//define gradient colors
yello=color(255, 187, 0);
orang=color(250, 133, 0);
}
function draw() {
background(100, 50, 122);
//call moon
moon();
//draw dessert mountains
mount();
//water
fill(10, 12, 71);
rect(0, height/3 * 2, width, height);
//render lanterns
lantRender();
}
//draw a mooon
function moon(){
noStroke();
fill(255, 237, 186, 30);
ellipse(width/2, height/3, 200,200);
fill(255, 237, 186);
ellipse(width/2, height/3, 150,150);
}
function mount(){
//two mountain ranges
noStroke();
beginShape();
fill(122, 55, 80);
vertex(0, height);
for (var x = 0; x < width; x++) {
var t2 = (x * terrainDetail2) + (millis() * terrainSpeed2);
var y = map(noise(t2), 0, 1, 100, 300);
vertex(x, y);
}
vertex(width, height);
endShape();
noStroke();
beginShape();
fill(53, 40, 105);
vertex(0, height);
for (var x = 0; x < width; x++) {
var t1 = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t1), 0, 1, 250, 300);
vertex(x, y);
}
vertex(width, height);
endShape();
}
//make lantern with variable parameters, return object lant
function makeLant(px,py) {
var lant = {x: px,
y: py,
width: random(0,50),
thic: random(20,30),
speed: moving,
update: lantUpdate,
draw: lantDraw}
return lant;
}
//draw lantern
function lantDraw() {
noFill();
noStroke();
//random size adds flickering
var size = this.thic + random(0,5);
var thic = this.width;
push();
translate(this.x, this.y * 3);
//gradient line
for (var i = 0; i < size; i++) {
var inter = map(i, 0, size, 0, 1);
var c = lerpColor(orang, yello, inter);
stroke(c);
strokeWeight(thic);
line(0, i, 20, i);
}
pop();
}
//move the lanters and have them start over when they hit the end
function lantUpdate() {
//speed of moving but with random to make wind/random travel speed effect
this.x += this.speed + random(-5,5);
if (this.x <= end) {
this.x += width - end;
}
}
//render lantern, add to list array
function lantRender() {
for (var i = 0; i < List.length; i++) {
List[i].update();
List[i].draw();
}
}
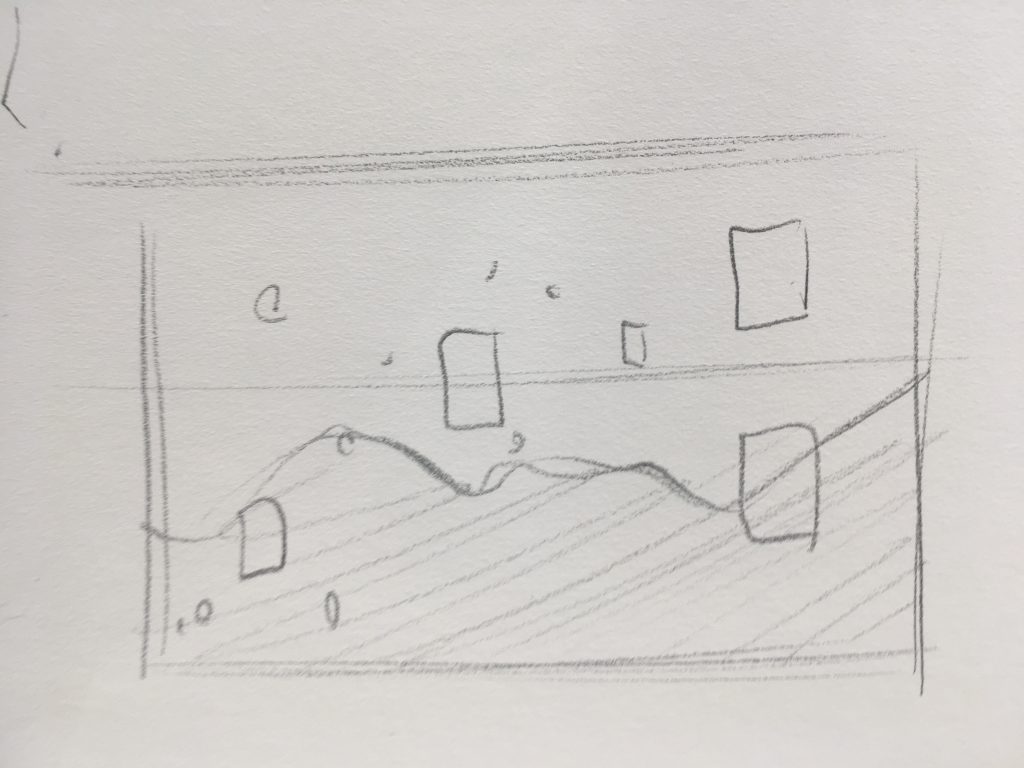
This project took inspiration from the Disney movie Tangled, specifically from the final, super romantic scene of the lantern lighting. I wanted to recreate this scene with the warm colors and floating lanterns. The randomized sizes created flickering and the size varation created depth to the lanters that lit up the sky. The lanterns float across the screen like they are in the sky.
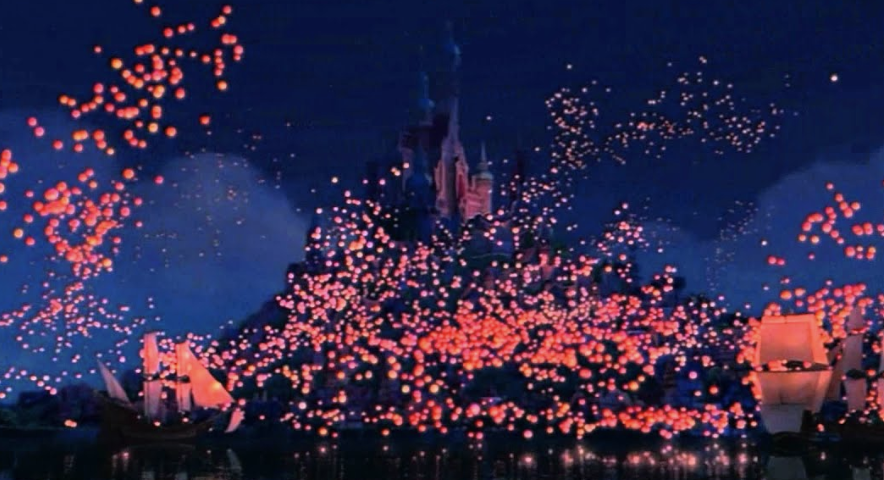
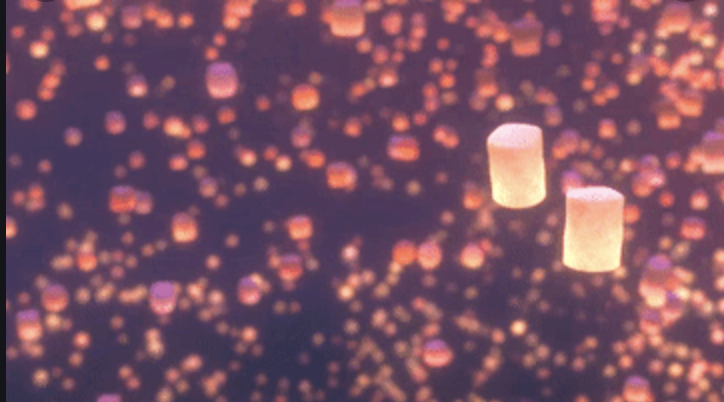