I created an interactive clock that includes two modes that the user can switch between: normal, and Christmas. Each of the two modes draws a green tree and a snowy Christmas tree. In the beginning, the user wouldn’t know what the wiggly lines are drawing, but as they wait, the tree images begin to appear. The drawing refreshes when they switch between the two modes.
The time is displayed quantitatively as numbers and more visually with bars on the tree trunk, and mouse movement creates variant colors for certain features.
/* Youie Cho
Section E
minyounc@andrew.cmu.edu
Final-Project*/
// Set up turtles to draw paths. Two draw their own paths simultaneously.
var ttl;
var ttl2;
var currIter = 0;
// Sets color for tree, outside area, text, and background
var rTree = 0;
var gTree = 100;
var bTree = 0;
var rOutside = 64;
var gOutside = 147;
var bOutside = 255;
var rText = 255;
var gText = 255;
var bText = 255;
var backgroundCol = 255;
// Ball colors (Christmas mode)
var ballcolors = ["red", "green", "pink", "orange", 'rgb(18, 89, 52)',
'rgb(140, 0, 0)', 'rgb(252, 186, 3)', 'rgb(217, 86, 30)'];
// Coordinates and opacity for drawing star at top (Christmas mode)
var x = [300, 311, 333, 319, 321, 300, 279, 281, 267, 289];
var y = [48, 67, 73, 90, 112, 103, 112, 90, 73, 67];
// Opacity for star (Christmas mode)
var opacity = 0;
function setup() {
createCanvas(600, 600);
background("white");
// Create turtles
ttl = makeTurtle(0, 300);
ttl.setWeight(1);
ttl2 = makeTurtle(600, 300);
ttl2.setWeight(1);
}
function draw() {
time(); // Displays time witn numbers
trunk(); // Trunk displays time with bars
star(); // Star displays in Christmas mode
ttl1Mvt(); // ttl1 settings
ttl2Mvt(); // ttl2 settings
// Turtle movement per frame
currIter ++;
ttl.forward(10);
ttl.right(random(-50, 50));
ttl2.forward(10);
ttl2.left(random(-50, 50));
buttons(); // Buttons used to switch between modes
}
function time() {
// Text box
noStroke();
fill(rTree, gTree, bTree);
rectMode(CENTER);
rect(width / 2, 293, 140, 32);
// Display hours with AM/PM mode, minutes, and seconds
noStroke();
fill(rText, gText, bText);
if (hour() > 12) {
textSize(20);
text(hour() - 12, 245, 300);
textSize(10);
text("PM", 345, 300);
} else {
textSize(20);
text(hour(), 245, 300);
textSize(10);
text("AM", 345, 300);
}
textSize(20);
text(minute(), 280, 300);
text(second(), 315, 300);
}
function trunk() {
rectMode(CORNER);
strokeWeight(0.5);
stroke(128, 70, 13);
fill(backgroundCol, backgroundCol, backgroundCol);
rect(259.5, 370, 81, 120);
fill(128, 70, 13);
// Progression of brown bars display time
var prgH = map(hour(), 0, 23, 0, 120);
rect(259.5, 370, 25, prgH);
var prgM = map(minute(), 0, 59, 0, 120);
rect(287.5, 370, 25, prgM);
var prgS = map(second(), 0, 59, 0, 120);
rect(315.5, 370, 25, prgS);
}
function star() {
var nPoints = x.length;
noStroke();
// Star color changes according to mouseX
var starCol = map(mouseX, 0, width, 130, 220);
fill(255, starCol, 100, opacity);
beginShape();
for (var i = 0; i < nPoints; i++) {
vertex(x[i], y[i]);
}
endShape(CLOSE);
noStroke();
}
function ttl1Mvt() {
// Turtle does not go outside of the canvas
if (ttl.x >= width || ttl.x <= 0 || ttl.y >= height || ttl.y <= 0) {
ttl.penUp();
ttl.goto(300, 300);
ttl.penDown();
}
// Color settings for tree area and outside area
if ((ttl.x > 280 & ttl.x < 320 && ttl.y > 90 && ttl.y < 110) ||
(ttl.x > 260 && ttl.x < 340 && ttl.y > 110 && ttl.y < 150) ||
(ttl.x > 240 && ttl.x < 360 && ttl.y > 150 && ttl.y < 200) ||
(ttl.x > 200 && ttl.x < 400 && ttl.y > 200 && ttl.y < 260) ||
(ttl.x > 150 && ttl.x < 450 && ttl.y > 260 && ttl.y < 370)) {
ttl.setColor(color(rTree, gTree, bTree));
} else {
ttl.setColor(color(rOutside, gOutside, bOutside));
}
}
function ttl2Mvt() {
// Turtle does not go outside of the canvas
if (ttl2.x >= width || ttl2.x <= 0 || ttl2.y >= height || ttl.y <= 0) {
ttl2.penUp();
ttl2.goto(300, 300);
ttl2.penDown();
}
// Color settings for tree area and outside area
if ((ttl2.x > 280 & ttl2.x < 320 && ttl2.y > 90 && ttl2.y < 110) ||
(ttl2.x > 260 && ttl2.x < 340 && ttl2.y > 110 && ttl2.y < 150) ||
(ttl2.x > 240 && ttl2.x < 360 && ttl2.y > 150 && ttl2.y < 200) ||
(ttl2.x > 200 && ttl2.x < 400 && ttl2.y > 200 && ttl2.y < 260) ||
(ttl2.x > 150 && ttl2.x < 450 && ttl2.y > 260 && ttl2.y < 370)) {
ttl2.setColor(color(rTree, gTree, bTree));
} else {
ttl2.setColor(color(rOutside, gOutside, bOutside));
}
}
function buttons() {
noStroke();
fill(mouseY / 5 + 200, mouseX / 5 + 200, mouseX / 5 + 100);
rect(13, 558, 100, 33);
rect(487, 558, 100, 33);
strokeWeight(0.5);
fill(rOutside, gOutside, bOutside);
textSize(15);
text("Normal", 40, 580);
text("Christmas", 505, 580);
}
function mousePressed() {
// NORMAL MODE ////////////////////////////////////////////////////////////
if (mouseX >= 13 & mouseX <= 113 && mouseY >= 558 && mouseY <= 691) {
// Turtle drawing refreshes upon cliking the button
noStroke();
clear();
backgroundCol = 255;
background(backgroundCol, backgroundCol, backgroundCol);
opacity = 0;
// Color settings
rTree = 0; // Green tree
gTree = 100;
bTree = 0;
rOutside = 64; // Blue outside
gOutside = 147;
bOutside = 255;
rText = 240; // Light green text
gText = 247;
bText = 218;
}
// CHRISTMAS MODE /////////////////////////////////////////////////////////
if (mouseX >= 487 & mouseX <= 587 && mouseY >= 558 && mouseY <= 691) {
// Turtle drawing refreshes upon cliking the button
clear();
backgroundCol = 0;
background(backgroundCol, backgroundCol, backgroundCol);
opacity = 100; // Displays star only in Christmas mode
// Color settings
rTree = 255; // White tree
gTree = 255;
bTree = 255;
rOutside = 255; // Yellow outside
gOutside = 213;
bOutside = 28;
rText = 248; // Dark yellow text
gText = 178;
bText = 41;
// Ornaments (Christmas mode)
noStroke();
for (var i = 0; i < ballcolors.length; i++) {
for (var j = 0; j < ballcolors.length; j++) {
fill(ballcolors[i]);
}
ellipse(random(280, 320), random(150, 250), 12, 12);
ellipse(random(170, 450), random(250, 370), 18, 18);
}
}
}
// TURTLE FUNCTIONS ///////////////////////////////////////////////////////////
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
};
return turtle;
}
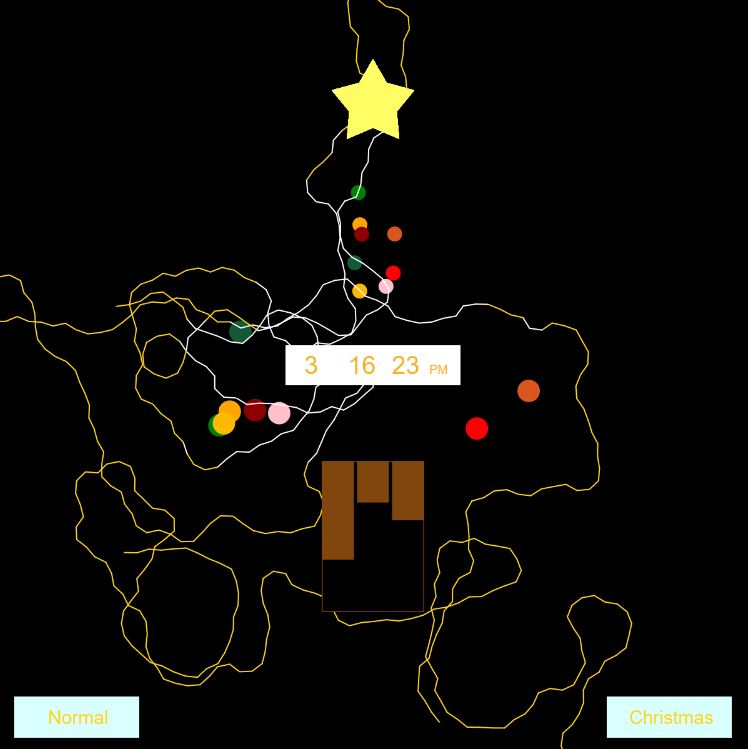
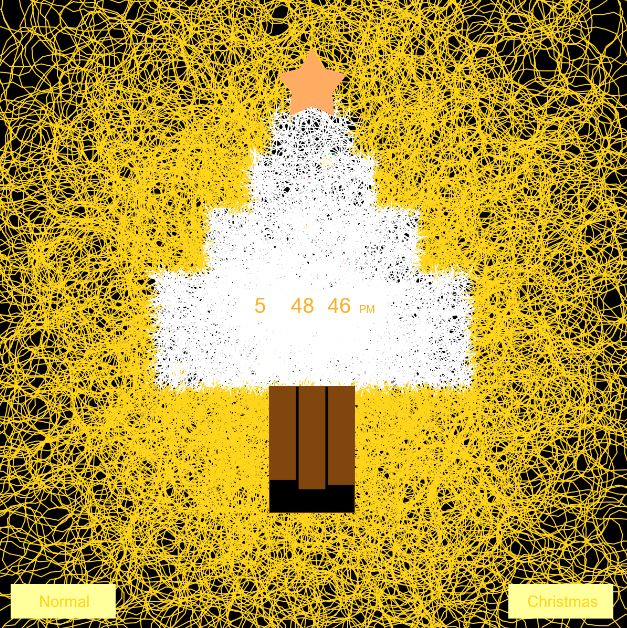
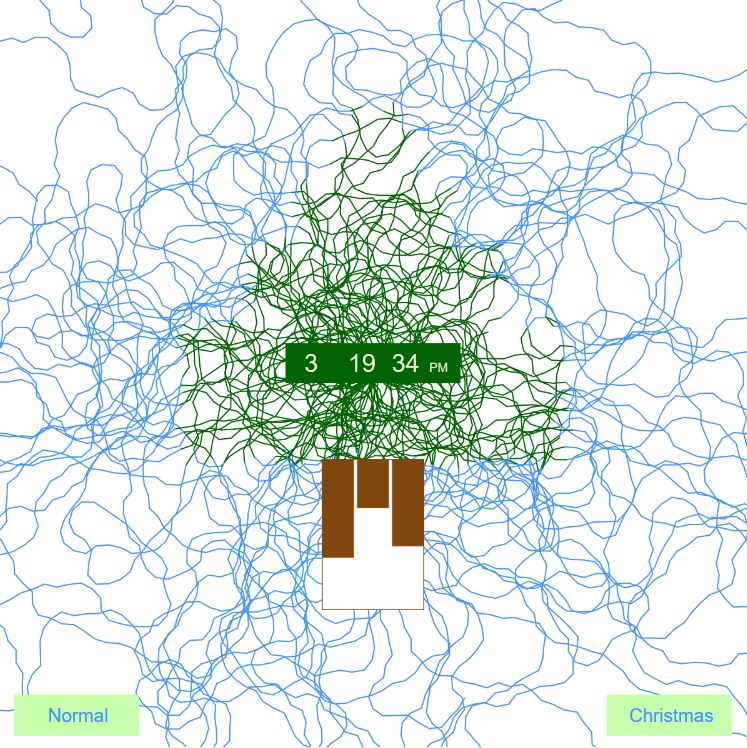