/*
Hyejo Seo
Section A
hyejos@andrew.cmu.edu
Final Project
*/
var ang = 0;
var doorX = 180;
var doorY = 55;
var arcX = 500;
var arcY = 85;
let active;
let activeB;
function setup() {
createCanvas(600, 400);
// loading image for green (palace)
green = loadImage("https://i.imgur.com/AA44Ncy.jpg");
// loading image for blue (flower field)
blue = loadImage("https://i.imgur.com/7prQtyF.jpg");
// loading image for red (sophie's hometown)
red = loadImage("https://i.imgur.com/puWmVDz.jpg");
// loading image for yellow (war scene)
black = loadImage("https://i.imgur.com/yZ2mKQJ.jpg");
active = 1;
activeB = 1;
}
function draw() {
angleMode(DEGREES);
activeBackground();
roomSetting();
activeElement();
wheel();
// arrow/pointer
noStroke();
fill('#32303A');
rect(arcX - 4, 0, 8, 30);
triangle(arcX - 8, 30, arcX + 8, 30, arcX, 45);
fill('#727072');
rect(arcX - 2.5, 0, 5, 30);
triangle(arcX - 6, 30, arcX + 6, 30, arcX, 45);
}
function wheel() {
// center of the wheel
fill(250, 204, 107);
circle(arcX, arcY, 17);
// highlight in the middle
fill(255, 233, 150);
noStroke();
beginShape();
curveVertex(494.5, 87);
curveVertex(494, 86);
curveVertex(494.2, 84);
curveVertex(495, 82);
curveVertex(497, 80);
curveVertex(499, 80);
curveVertex(497, 82);
curveVertex(496, 84);
curveVertex(495.5, 85);
curveVertex(495, 87);
curveVertex(495, 85);
endShape();
}
function mousePressed() {
// if user clicks inside of the wheel, it returns true
if ((mouseX >= arcX - 120 & mouseX <= arcX + 120) && (mouseY >= arcY - 120 & mouseY <= arcY + 120)) {
active = ((active % 4) + 1); // for the wheel to turn
activeB = active; // for the background to change
return true
} else {
return false
}
}
function roomSetting() {
//walls
noStroke();
fill("#E8E8E8");
rect(0, 0, width, 53);
rect(0, 53, 120, 107);
rect(0, 53 + 97, 15, 300);
rect(0, 290, 120, 200);
rect(415, 53, 190, 107);
rect(415, 160, 10, 240);
rect(575, 160, 30, 240);
rect(420, 300, 155, 100);
// left window
fill("#4C3D2E");
rect(15, 160, 120, 10);
rect(15, 170, 10, 120);
rect(15, 290, 120, 10);
rect(15, 225, 120, 8);
rect(90, 170, 8, 120);
// right window
rect(425, 160, 140, 10);
rect(425, 170, 10, 120);
rect(425, 290, 140, 10);
rect(565, 160, 10, 140);
rect(495, 170, 10, 120);
rect(435, 225, 140, 10);
// door frame
stroke("#72594E");
strokeWeight(5);
noFill();
rect(175, 55, 240, height);
// opened door
fill("#725B45");
noStroke();
quad(doorX, doorY, doorX - 50, doorY - 30, doorX - 50, height, doorX, height);
stroke("#4C3D2E");
strokeWeight(2);
line(doorX - 10, doorY - 5, doorX - 10, height);
line(doorX - 17, doorY - 9, doorX - 17, height);
line(doorX - 27, doorY - 15, doorX - 27, height);
line(doorX - 38, doorY - 21, doorX - 38, height);
noStroke();
// thickness of the door
fill("#4C3D2E");
rect(doorX - 60, doorY - 30, 10, height);
}
function blueTop() { // wheel with blue being pointed
stroke(0);
strokeWeight(1);
// blue
fill(39, 154, 241);
arc(arcX, arcY, 120, 120, 225, 315);
// black
fill(52, 46, 55);
arc(arcX, arcY, 120, 120, 315, 405);
//red
fill(255, 50, 50);
arc(arcX, arcY, 120, 120, 45, 135);
// green
fill(159, 211, 86);
arc(arcX, arcY, 120, 120, 135, 225);
}
function greenTop() { // wheel with green being pointed
stroke(0);
strokeWeight(1);
//green
fill(159, 211, 86);
arc(arcX, arcY, 120, 120, 225, 315);
// blue
fill(39, 154, 241);
arc(arcX, arcY, 120, 120, 315, 405);
//black
fill(52, 46, 55);
arc(arcX, arcY, 120, 120, 45, 135);
// red
fill(255, 50, 50);
arc(arcX, arcY, 120, 120, 135, 225);
}
function redTop() { // wheel with red being pointed
stroke(0);
strokeWeight(1);
//red
fill(255, 50, 50);
arc(arcX, arcY, 120, 120, 225, 315);
// green
fill(159, 211, 86);
arc(arcX, arcY, 120, 120, 315, 405);
//blue
fill(39, 154, 241);
arc(arcX, arcY, 120, 120, 45, 135);
// black
fill(52, 46, 55);
arc(arcX, arcY, 120, 120, 135, 225);
}
function blackTop() { // wheel with black being on pointed
stroke(0);
strokeWeight(1);
//black
fill(52, 46, 55);
arc(arcX, arcY, 120, 120, 225, 315);
// red
fill(255, 50, 50);
arc(arcX, arcY, 120, 120, 315, 405);
//green
fill(159, 211, 86);
arc(arcX, arcY, 120, 120, 45, 135);
// blue
fill(39, 154, 241);
arc(arcX, arcY, 120, 120, 135, 225);
}
function activeElement() {
if (active === 1) { // wheel starts with blue being pointed
return blueTop();
}
else if (active === 2) {
return greenTop();
}
else if (active === 3) {
return redTop();
}
else if (active === 4) {
return blackTop();
}
}
function activeBackground() {
if (activeB === 1) { //starts with the background for blue
return background(blue);
}
else if (activeB === 2) {
return background(green);
}
else if (activeB === 3) {
return background(red);
}
else if (activeB === 4) {
return background(black);
}
}
For this project, I recreated Howl’s door in the animation film, Howl’s Moving Castle. In this movie, the door leads to different countries/places based on the color the pointer is at (wheel). In order to see where this door can lead you to, you click anywhere inside the wheel. As the color the pointer is pointing at in the wheel changes, the background changes.
Blue – flower field
Green – palace
Red – Sophie’s hometown (train)
Black – a war scene
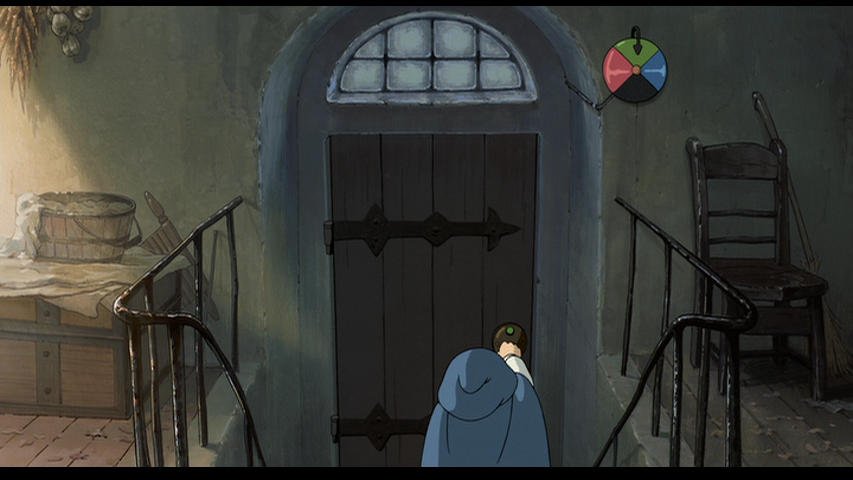