/*
Ellan Suder
15104 1 D
esuder@andrew.cmu.edu
Project-02
*/
var left = 165;
var right = left + 200;
var upDown;
var randomColor;
var randomAlpha = 100;
var randomSize = 50;
function setup() {
createCanvas(600, 450);
background(200,300,40);
randomColor = color(0,0,0);
noStroke();
fill(0,140,230);
ellipse(200,450,300,300);
fill(255); //face
ellipse(200,200,300,390);
ellipse(350, 150, 80, 80);
fill(0,20,30) //dot eyes
ellipse(150,150,40,40);
ellipse(350,150,40,40);
fill(0,20,30); //hat
noStroke();
beginShape();
curveVertex(70, 90);
curveVertex(70, 90);
curveVertex(58, -20);
curveVertex(321, -20);
curveVertex(332, 90);
curveVertex(332, 90);
endShape();
strokeWeight(6); // lines
stroke(0,20,30)
noFill();
arc(150, 150, 80, 80, 3* QUARTER_PI, TWO_PI); //left eye
arc(350, 150, 80, 80, PI, TWO_PI + HALF_PI); //right eye
arc(250, 220, 80, 30, 3 * HALF_PI, QUARTER_PI); //nose
arc(250, 280, 60, 60, 0 , PI); //mouth
strokeWeight(7);
stroke(0,140,230);
rect(80, 100, 140, 120, 20); // left glasses lens
rect(300, 100, 140, 120, 20); // right glasses lens
textSize(10);
noStroke();
fill(0);
text("click to randomize!", 460, 90);
}
function mousePressed() {
noStroke(); //two circles change color every mousepress
randomColor = color(random(230),random(230),random(230));
fill(randomColor);
ellipse(150,220,40,40);
ellipse(350,220,40,40);
var a = 110; //clover
var b = 45;
var r = 10;
var w = 50;
ellipse(a,b,r+10,w+10);
bezier(a,b, //top
a-r+w,b-r+w,
a+r+w,b-r-w,
a,b);
bezier(a,b, //top (left)
a-r-w,b-r-w,
a+r-w,b-r+w,
a,b);
bezier(a,b, //right (top)
a+r-w,b+r-w,
a+r+w,b-r-w,
a,b);
bezier(a,b, //right (bottom)
a+r+w,b+r+w,
a+r-w,b-r+w,
a,b);
bezier(a,b, //left
a-r+w,b-r+w,
a-r-w,b+r+w,
a,b);
bezier(a,b, //left (top)
a-r-w,b-r-w,
a-r+w,b+r-w,
a,b);
bezier(a,b, //bottom (left)
a+r-w,b+r-w,
a-r-w,b+r+w,
a,b);
bezier(a,b, //bottom
a+r+w,b+r+w,
a-r+w,b+r-w,
a,b);
fill(255) //redraw sclera
ellipse(150,150,75,75);
ellipse(350,150,75,75);
left = random(137, 158);
right = left + 200
randomSize = random(10,45);
randomAlpha = random(60,100);
randomColor.setAlpha(randomAlpha);
fill(randomColor);
upDown = random(137,163);
ellipse(left, upDown, randomSize, randomSize); //eyes
ellipse(right, upDown, randomSize, randomSize);
fill(255) //redraw nose area
rect(250,200,45,40);
stroke(0);
noFill();
arc(250, 220, randomSize + 20, 30, 3 * HALF_PI, QUARTER_PI); //nose
noStroke();
fill(255)
ellipse(250,300,85,85);
fill(0);
var oscsize; //mouth
ellipse(250,300,80,60 - sin(millis()/100) * 20);
let letters = ['A', 'B', 'C', 'D', 'E', 'F',
'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P',
'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'];
let letter = random(letters); // select random letter
fill(200,300,40) //redraw background
rect(450,30,100,100)
fill(0);
textSize(62);
text(letter, 470, 90);
}
Author: esuder
Ellan Suder-LookingOutwards-02
Creator’s name: Alfred Hoehn
Title of the work above: Drawing Machine Ptolemaios
Year of creation: 2008
I really enjoy the works of Alfred Hoehn, a Swiss artist who constructs large-scale linkage harmonographs. The simple movements of the pendulums create complex images that can look flat/abstract or look almost 3D, depending on how the artist sets the lengths and the diameters of the spinning circles.
The finished product is fun to look at, but the process of watching the image as it’s being drawn is important to the project as well. It’s very mesmerizing and calming –– a few people in the comments section were comparing it to a computer screensaver.
The artist can probably estimate the finished product to an extent, but I like the element of uncertainty. It’s kind of like plugging a random equation and seeing what kind of graph it’ll make.
Ellan Suder-Project01-Face
I had a bit of trouble sorting out which values corresponded with which points, especially with the quadrilaterals, but I eventually worked it out!
function setup() {
createCanvas(500, 500);
background(93, 203, 255);
stroke(250, 50, 56);
strokeWeight(20);
line(60, 360, 67, 345); //thumb
line(160, 475, 40, 340); //leftarm
line(450, 360, 435, 345); //thumb
line(440, 475, 460, 340); //rightarm
stroke(0, 0, 255)//flag
strokeWeight(5)
line(60,420,60,70)
fill(255);
rect(60, 70, 400, 100, 20);
noStroke(); //face
fill(250, 50, 56);
ellipse(250,350,200,170);
let s = 'hello! nice to meet you.';
fill(255, 0, 10);
textSize(35)
text(s, 80, 85, 400, 100)
noStroke(); //face
fill(250, 41, 56);
ellipse(300,500,300,260);
stroke(255, 0, 10); //nose
strokeWeight(5);
fill(0, 240, 205);
triangle(40+270, 75+300, 58+270, 20+300, 86+270, 65+300);
fill(0, 255, 0); //eyebrows
quad(38+180, 31+240, 86+180, 20+240, 90+180, 40+240, 30+180, 76+240);
fill(0, 255, 0);
quad(38+270, 21+240, 86+270, 20+240, 90+270, 40+240, 20+270, 30+240);
stroke(0, 0, 255); //left eye
strokeWeight(5);
fill(255);
ellipse(250, 340, 30, 50);
stroke(10, 10, 255); //right eye
strokeWeight(5);
fill(255);
ellipse(300, 340, 50, 30);
arc(300, 400, 60, 20, 0, PI + QUARTER_PI, PIE); //mouth
strokeWeight(5);
fill(255);
ellipse(300, 340, 50, 30);
}
function draw() {
}
Ellan Suder-LookingOutwards-01
One of my current favorite video games is Breath of the Wild from Nintendo’s Legend of Zelda series, a nonlinear and open world action-adventure game. It’s the first game of its kind in the series and inspired by other open-worlds such as Skyrim. Besides the beautiful visuals, it’s just a really fun game to play.
I can’t claim to know much about game systems, but I’ve heard how BOTW’s physics system has impressed many. The game was built in a modified version of the Havok Physics Engine. The game developers had to work to create a new physics engine that was consistent across the game world and worked logically without negatively affecting gameplay. (One of the game developers recounts a time where during testing, he entered an area only to find that all the objects normally there had been blown away by the wind.) The engine rewards exploration and experimentation. ‘For instance, in Breath of the Wild you might have a puzzle where making use of the physics, there’ll be various ways you can solve that puzzle’ (Aonuma, series producer).
As well as being a new direction for Zelda, the game is a popular innovation on the open-world genre because it simplifies the needless complexity of similar games in its genre and introduces new ways to interact with the environment. Instead of following a list of to-do list of quests, the players are allowed to focus on the expansive world and direct themselves. I really admire how they were able to create a game that encourages the players to move all across the map and gives them almost complete freedom. BOTW’s greatest success was in capturing the spirit of adventuring into the wild.
Test 1
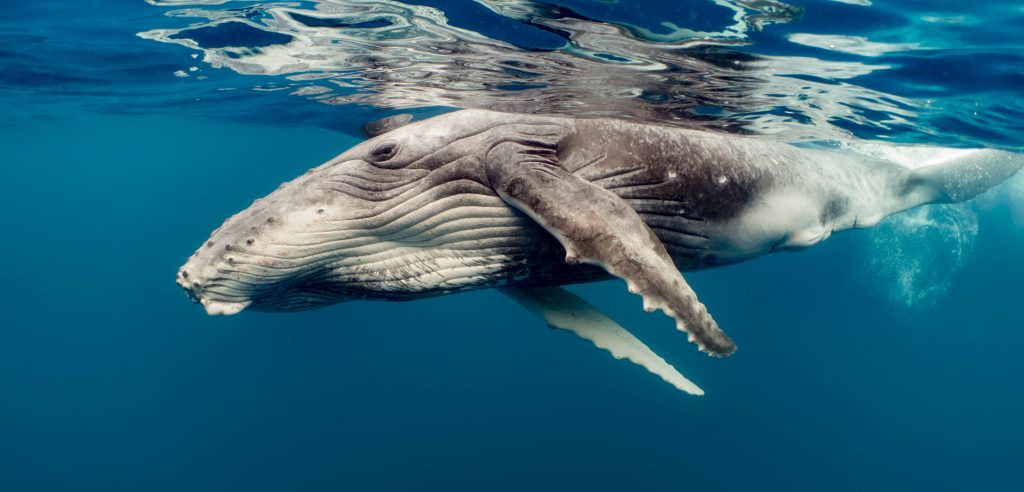
Sample text
function setup() {
createCanvas(200, 20);
background(220);
}
function draw() {
createCanvas(300, 300);
background(200);
ellipse(150, 150, 100, 70);
}