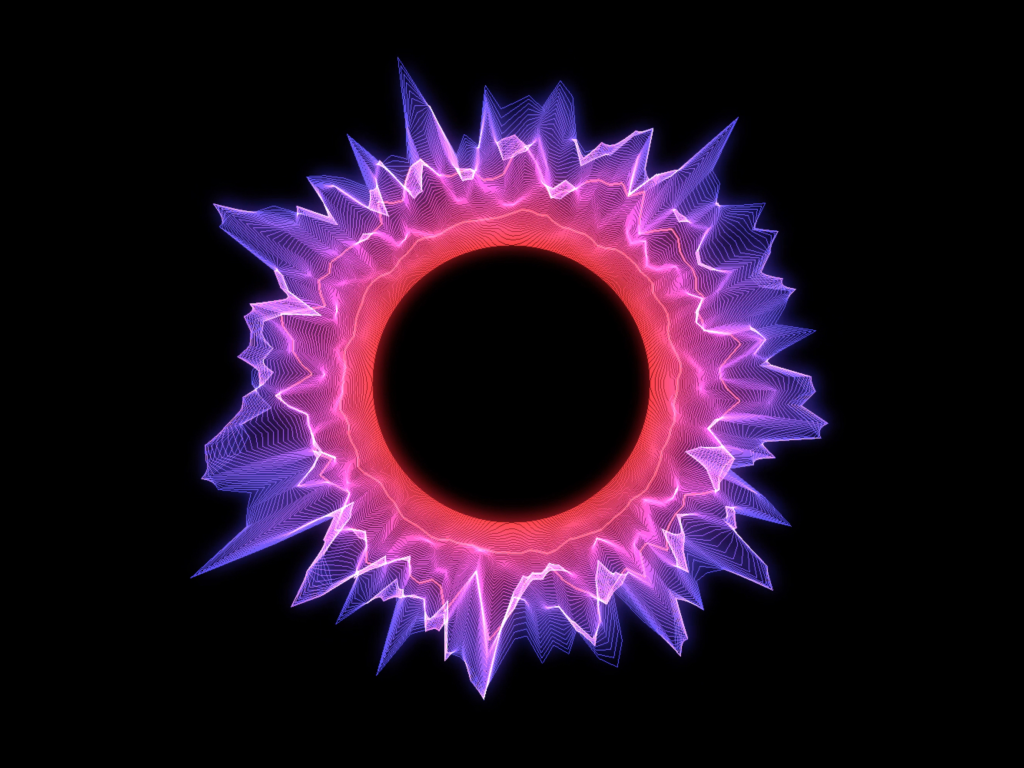
Created by Ora Systems, the project “Halo” is a visualization platform that can combine up to ten different data sets into a fluid multi-dimensional band of light. Ora currently works to develop “halo”s that represent patient’s health status, working with health companies such as Mayo Clinic to enhance the way we look at the health data of patients by creating responsive wearable-driven health identities through an app. Through familiarizing yourself with the visualization, the user should be able to easily understand and compare vast data sets. I appreciate the way this project combines both a creative and artistic approach to the output of the visualization with a technical advancement in its combination of so many different data inputs/sets. I also appreciate that this project can be used to solve problems through its design and not just be a visual.
reated by Ora Systems, the project “Halo” is a visualization platform that can combine up to ten different data sets into a fluid multi-dimensional band of light. Ora currently works to develop “halo”s that represent patient’s health status, working with health companies such as Mayo Clinic to enhance the way we look at the health data of patients by creating responsive wearable-driven health identities through an app. Through familiarizing yourself with the visualization, the user should be able to easily understand and compare vast data sets. I appreciate the way this project combines both a creative and artistic approach to the output of the visualization with a technical advancement in its combination of so many different data inputs/sets. I also appreciate that this project can be used to solve problems through its design and not just be a visual.
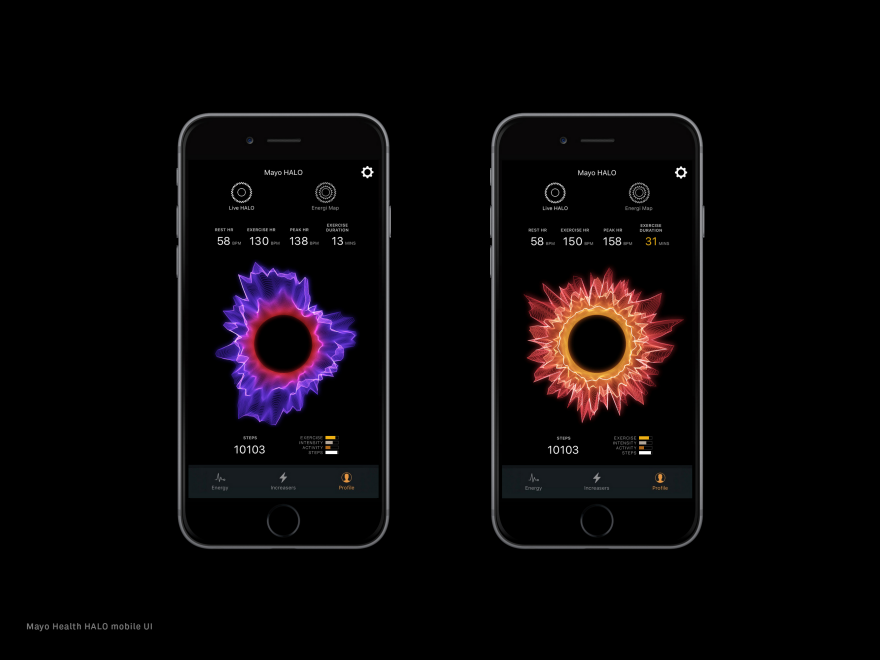